Web components are great right?
You get easily shareable custom elements that neatly encapsulate your code and can isolate your CSS via shadow DOM, this is all free in modern browsers. The API with connected and attribute change callbacks is easy to understand. Well tested polyfills are available...
But
How do I pass complex objects into my custom elements?
<script type="text/javascript">
class HelloElement extends HTMLElement {
static get observedAttributes() {return ['name']; }
attributeChangedCallback(attr, oldValue, newValue) {
if (attr == 'name') {
console.log(this.myObj);
this.textContent = `Hello, ${newValue}`;
}
}
}
customElements.define('hello-element', HelloElement);
</script>
<hello-element name="Anita"></hello-element>
<script type="text/javascript">
setTimeout(function() {
let he = document.querySelector('hello-element');
he.myObj = { myArr:[1,2,3,4,5]}
he.setAttribute('name','Aaron');
},1000)
</script>
Geez
Manually triggering rerender is too much work
Use a tiny component automatic re-rendering library like
https://github.com/mikeal/rza
http://stash.aur.ziprealty.com:7990/users/ahans/repos/component-header/browse/src/zap-header
New design of site header with dynamically re-rendering menu contents
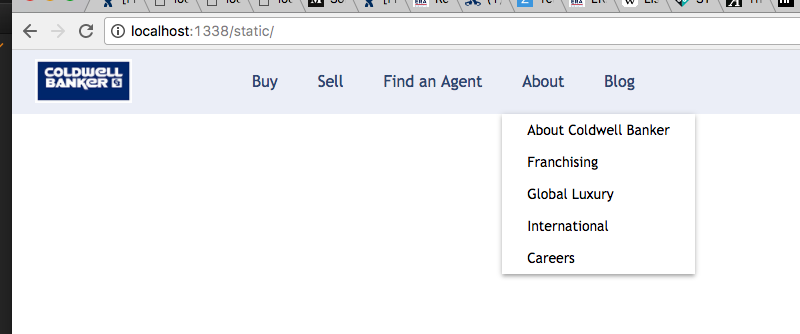
import styles from './_styles.scss';
import RZA from 'rza';
class ZapDropDown extends RZA {
get defaults () {
return {
data: []
}
}
render (settings, innerHTML) {
console.log(settings)
return `<ul class="submenu-container">
${(() => {
let output = '';
if(settings.data.submenu) {
settings.data.submenu.forEach(function(item) {
output += `<li class="menu-item submenu">
<a href="${item.link}">${item.name}</a>
</li>`;
})
}
return output;
})()}
</ul>
<style type="text/css">${styles}</style>`
}
}
window.customElements.define('zap-dropdown', ZapDropDown)
But
It doesn't even have virtual DOM
Choose a tiny, cached, tagged template literal render library
hyperHTML
lit-html
But
I don't want to write any code
Use polymer
Get access to tons of pre-built, accessibility tested components, polymer 3 uses ES6 modules
https://aaronhans.github.io/polymer3-test-drive/index-bundle.html
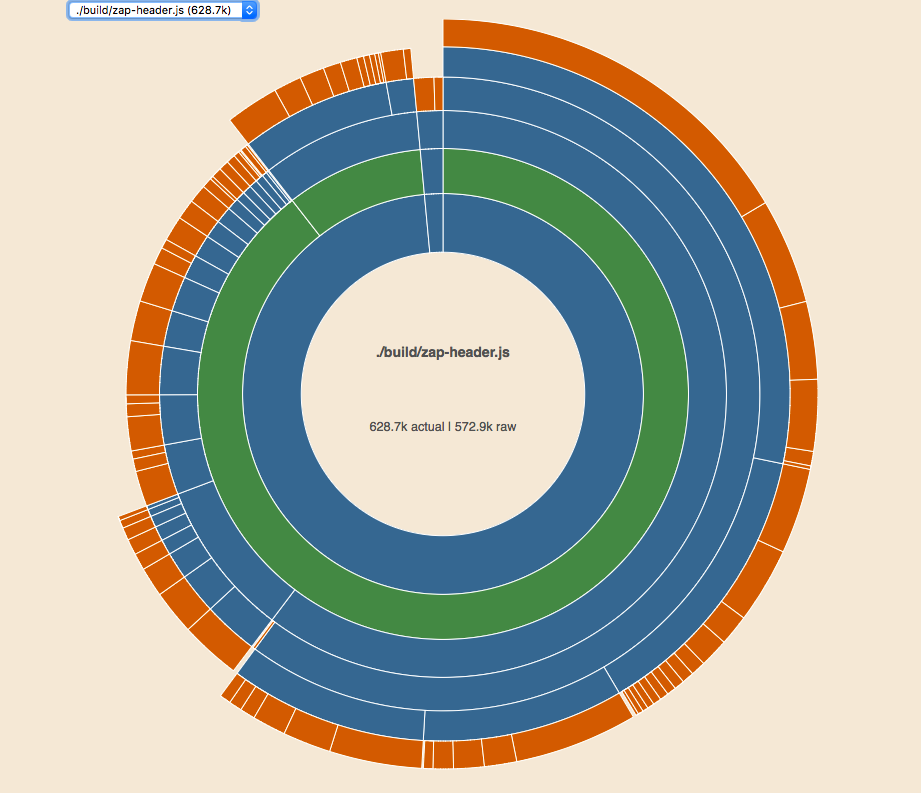
Careful though, the polymer base library is slim but their component weight can add up. This beautiful responsive header can be yours without writing code but their backwards compatible components are heavy
Web components are becoming a standard output target of larger frameworks.
The https://stenciljs.com/ project from https://ionicframework.com/ is a good example, use a fancy framework to build component code but export a plain vanilla web component
I need server side rendering
But
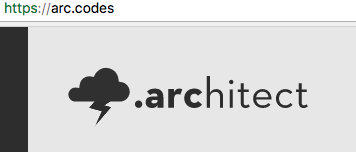
The arc.codes aws lambda management tool
- let me setup and deploy a headless chrome web component renderer in minutes
- local dev is identical to cloud
- staging env setup automatically
- command line deploy
http://stash.aur.ziprealty.com:7990/users/ahans/repos/ssr-lambda/browse
web components?
By Aaron Hans
web components?
- 32