Serverless
Server Side Rendering
Who cares about server side rendering?
You don't even need that, just call the APIs from client side code
Purely client side rendering is not fast enough
Serverless? Yeah right.
You aren't fooling anybody, it is servers all the way down
Right there are servers
- But we do not manage them at all
- AWS Lambda outsources the Docker/Kubernetes level setup and management
- Your function will scale up and down automatically
- We do not configure containers
- We do not configure container orchestration
- We do not manage ingress controllers
- Our codebase contains less environment management code
- New projects aren't gated by requiring expert setup

But coldstarts are scary
Oh no! Your code might have to start from scratch and be parsed before responding to a request
Small apps start fast
- The smaller your Lambda endpoint bundle the faster it starts.
- Keep your dependency chain short, node.js Lambda bundles under 5MB can startup in <200ms
- Add caching by making your Lambda operate by a trigger from a CloudFront request
- A Lambda will stay alive for 4:30 after the last request
Why is there so much motherflippin javascript?
import styles from './_styles.scss'; // universal zap component styles
import parse from 'url-parse';
import buttonData from './link-data.json';
import ZapDropDown from '../zap-dropdown/index.js';
import brandLogos from './logos.json';
class ZapHeader extends HTMLElement {
connectedCallback() {
const url = parse(window.location.toString(), true);
const self = this;
fetch(url).then(function(response) {
return response.json();
}).then(function(json) {
self.data = json;
self.data.agentBox = require('./agentbox.html')(self.data);
const html = require('./index.html')(self.data);
self.innerHTML = html;
self.dropDownSetup();
self.hamburgerHelper();
self.agentInfoDisplay();
});
}
dropDownSetup() {
const dropdowns = document.querySelectorAll('.menu-container zap-dropdown');
const self = this;
dropdowns.forEach(function(menu,i) {
menu.data = self.data.buttons[i];
})
}
hamburgerHelper() {
const hamburger = document.querySelector('.hamburger-container');
hamburger.addEventListener('click',function(e) {
e.preventDefault();
const menuC = document.querySelector('.menu-container');
if(menuC.style.left !== '0px') {
document.querySelector('.agent-profile').style.display = 'block';
menuC.style.left = '0px';
} else {
menuC.style.left = '-100%';
setTimeout(function() {
document.querySelector('.agent-profile').style.display = 'none';
},300)
}
})
}
agentInfoDisplay() {
const agentLink = document.querySelector('.agent-link');
if(agentLink) {
const agentContainer = document.querySelector('.agent-container');
agentContainer.addEventListener('mouseenter',function(e) {
agentContainer.querySelector('.agent-profile').style.display = 'block';
})
agentContainer.addEventListener('mouseleave',function(e) {
agentContainer.querySelector('.agent-profile').style.display = 'none';
})
}
}
}
window.customElements.define('zap-header', ZapHeader)
Our Lambda plans are the perfect use case for node.js
- We are aggregating API responses
- Most of the execution time is spent waiting for an external API response
- node.js is extremely efficient at this type of operation, able to deliver high capacity with low-end hardware
- We aren't managing threads in node.js, callback responses are handled by the node.js event loop
- JSON is understood without additional libraries
Too much
For every endpoint I have to setup the Lambda function, then to call it I need to setup a trigger so I need CloudFront or API Gateway...
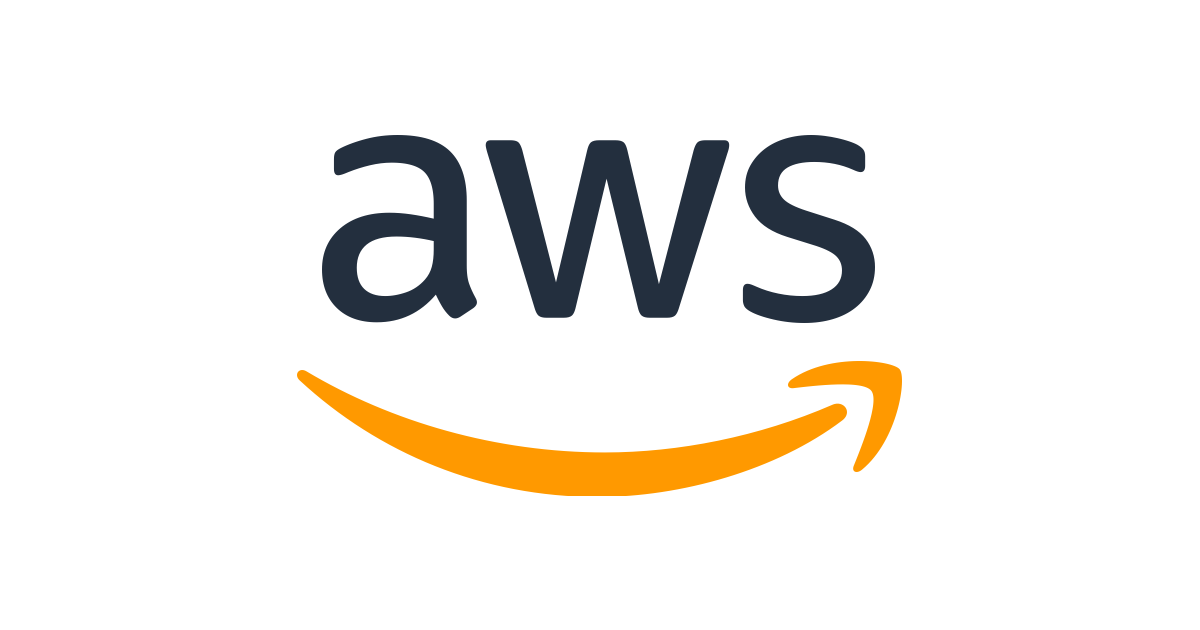
Use a deployment system
- New Lambda creation can be a single command
- Serverless is the name of the most popular Lambda configuration repository. It started with node.js but now supports other languages
- My favorite Lambda setup system is arc.codes
- You can create your own system with some aws cli commands setup as npm run scripts in your package.json
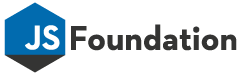
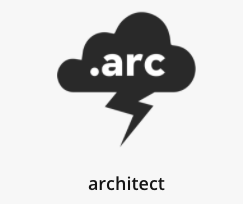
New project setup from scratch through deploying staging and production environments including Lambda's, API Gateway, dynamoDb using arc.codes for both an HTML and JSON endpoint
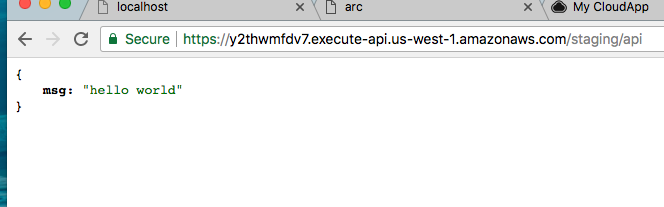
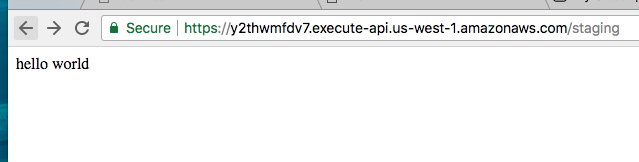
This yaml file is the configuration to create the html and json endpoints below accessible via API Gateway with sessions recorded in dynamoDB
@app
zap-lambda-helloWorld
@html
get /
@json
get /api
Um, being tied to a single cloud vendor is risky
- Currently AWS has the superior function as a service offering
- Libraries like arc.codes are already beginning to support alternate cloud vendors
- You can setup redundancy across regions using AWS today which will insulate you from entire datacenter outages
- We are also already using lambdas@edge where your code executes at the edge caches
Rendering web components
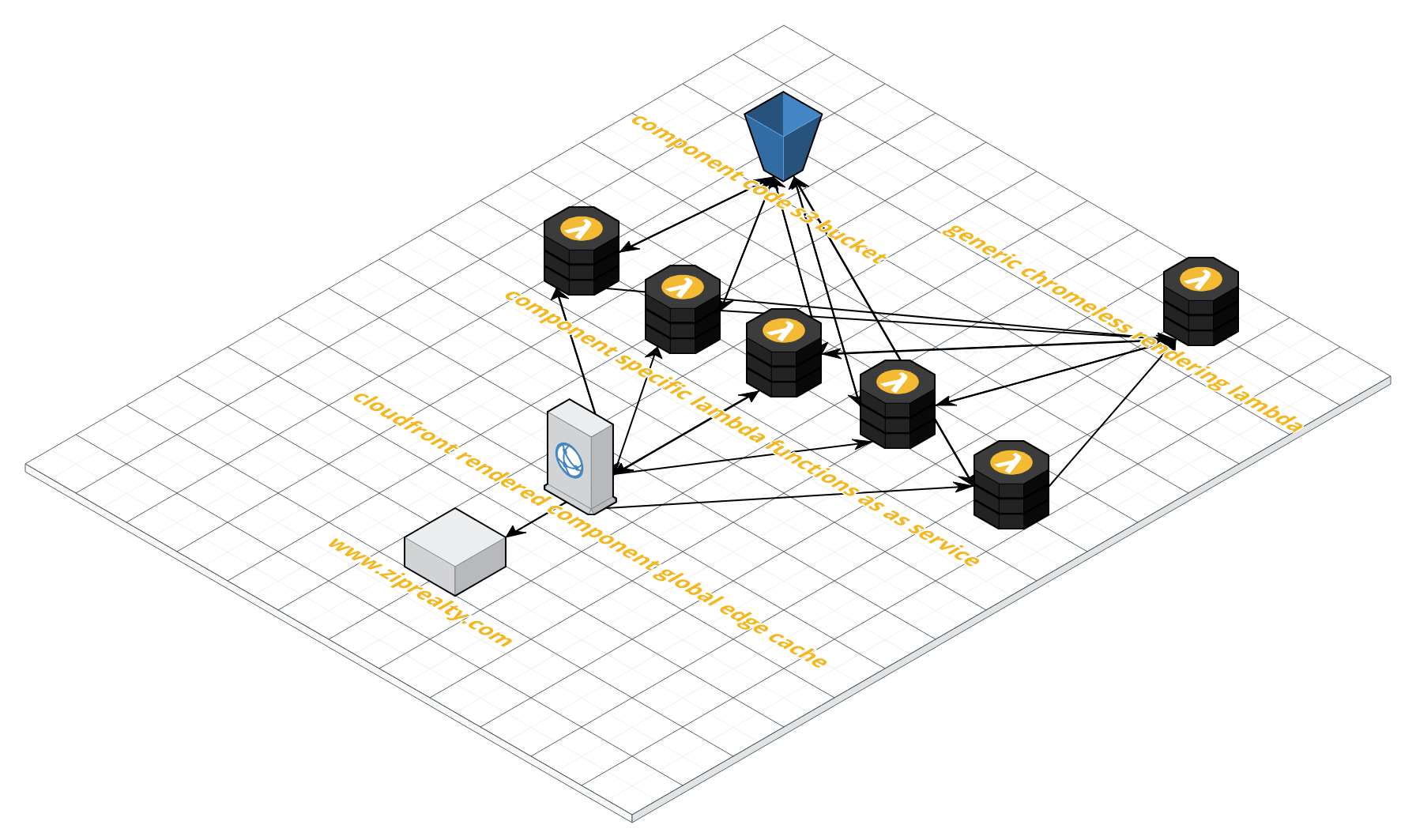
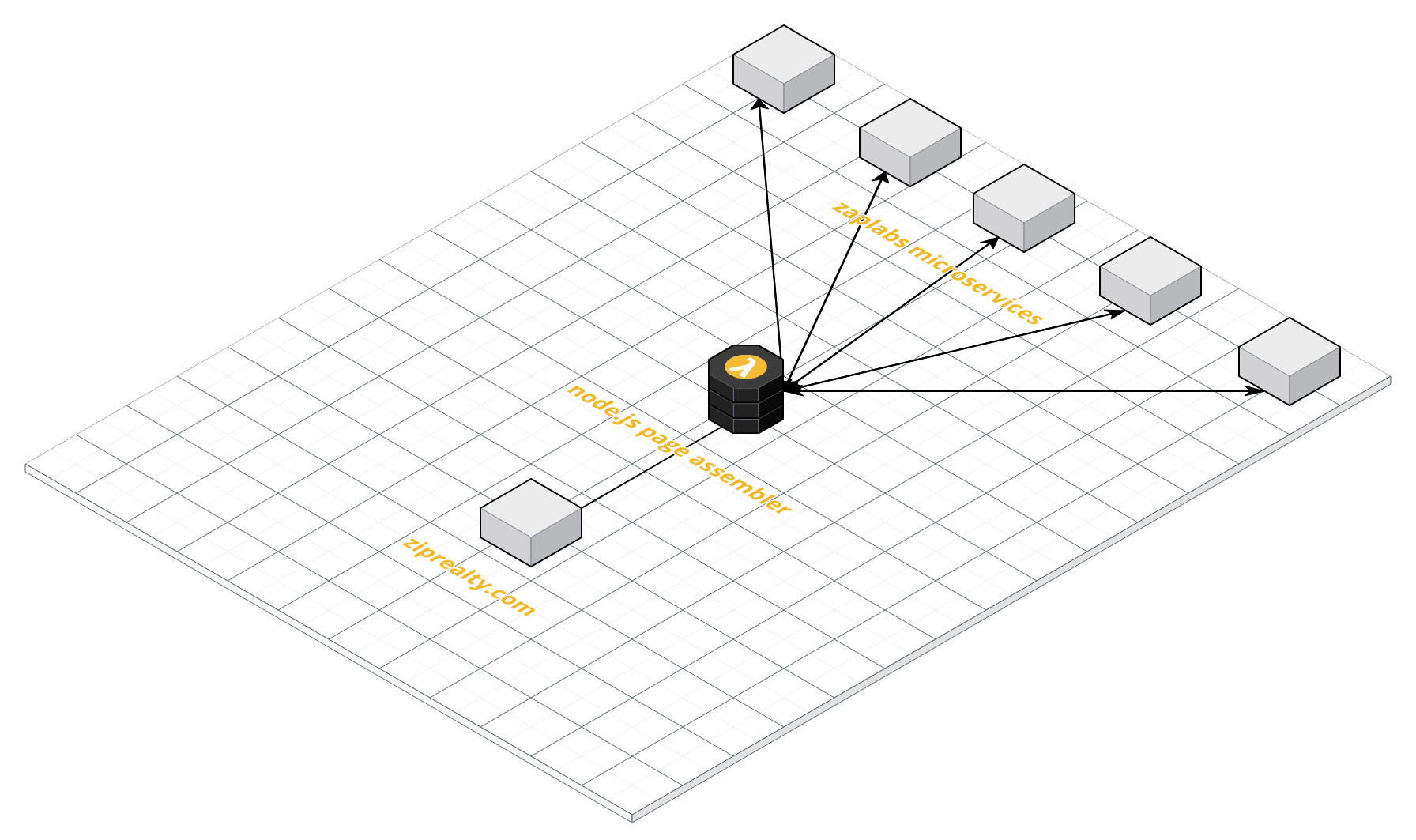
Serving web pages
How do you use it?
Benefits
- Fast new environment standup
- Fast deploys
- Efficient node.js page assembly from API calls
- Render Web Components on server
- Create basic APIs serving json via dynamoDB in few steps
- Ability to execute node.js code at edge cache nodes
- Quickly integrate with CloudFront edge caches
- Auto-scales up and down so traffic bursts are handled
- This is the beginning of a new ecosystem: AWS Serverless Application Repository may become the npm of functions as a service
Serverless Server Side Rendering
By Aaron Hans
Serverless Server Side Rendering
- 44