Proyecto guifOS

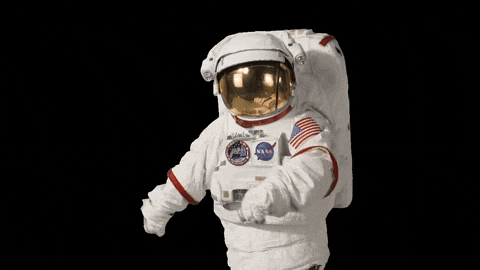
Arquitectura
Arquitectura General
guifOS
SPA
HTML
CSS
JS
Layout
Theme Day
Theme Night
API Services
DOM Handling
GIF Rendering
Style Handling
Video Recorder
Visit Counter
Init
Arquitectura Javascript
Punto de Partida
Pensar el programa como un flujo de información que parte desde un Input y busca un resultado (Output)
Input
Output
Programa
Como lo utilizo?
Que resultado espero?
Arquitectura Javascript
Reglas
Evitar variables globales
DRY (Don't Repeat Yourself)
SRP (Single Responsibility Principle)
Arquitectura Javascript
Funciones
Input
unaFuncion()
otraFuncion()
otraMas()
yOtra()
laUltima()
Output
Arquitectura en base a objetos
const giphyApi = {
searchEndpoint: "https://api.giphy.com/v1/gifs/search",
trendEndpoint: "https://api.giphy.com/v1/gifs/trending",
uploadEndpoint: "https://upload.giphy.com/v1/gifs?",
idEndpoint: "https://api.giphy.com/v1/gifs/",
apiKey: "eiVo3MScNwrZJfkUOIP0WHzIV8uOQesx",
abortController: new AbortController(),
getSearchResults: (url, requestType, limit = 20) => {
const API_KEY = giphyApi.apiKey;
const found = fetch(
url + requestType + "&api_key=" + API_KEY + "&limit=" + limit
).then(response => {
return response.json();
});
return found;
},
postGif: async () => {
try {
const API_ENDPOINT = giphyApi.uploadEndpoint;
const API_KEY = giphyApi.apiKey;
const heading = new Headers();
const uploadFile = createGif.createFormData();
const response = await fetch(API_ENDPOINT + "api_key=" + API_KEY, {
method: "POST",
headers: heading,
body: uploadFile,
cors: "no-cors",
signal: giphyApi.abortController.signal
});
const json = await response.json();
const gifId = json.data.id;
giphyApi.getUploadedGif(gifId);
} catch (error) {
domHandling.showErrorMsg(error);
domHandling.handleUploadWindows();
domHandling.handleRecordField();
domHandling.handleStartWindows();
domHandling.handleGifsSection();
myGuifos.renderMyGuifos();
}
},
getUploadedGif: async id => {
const API_ENDPOINT = giphyApi.idEndpoint + id + "?";
const API_KEY = giphyApi.apiKey;
const response = await fetch(API_ENDPOINT + "&api_key=" + API_KEY);
const json = await response.json();
const gifUrl = await json.data.images.original.url;
createGif.saveGif(gifUrl);
domHandling.handleSuccessWindows(gifUrl);
}
};
Ejemplo 1
Busqueda de Gifs

1. Evento Onsubmit
const domHandling = {
// ASINGACION DEL ELEMENTO HTML DENTRO DEL OBJETO.
searchForm: document.querySelector("#search-form"),
....
// CREO UN METODO PARA MANEJAR LOS EVENTOS.
handlerEvents: () => {
domHandling.searchForm.onsubmit = event => {
renderGifs.cleanRenderedGifs(); // LIMPIA LOS GIFS RENDERIZADOS ANTERIORMENTE
domHandling.removeRelatedButtons(); // REMUEVE LOS BOTONES
//AZULES DE LA BUSQUEDA ANTERIOR
domHandling.printSearchTitle(domHandling.getUserInput()); // IMPRIME EL
//TITULO DE LA BUSQUEDA EN EL ENCABEZADO
renderGifs.renderResultGifs(
giphyApi.searchEndpoint, // LO VEMOS A CONTINUACION
`?q=${domHandling.getUserInput()}`
);
event.preventDefault(); //IMPIDE QUE SE RECARGUE LA PAGINA
};
}
};
2. Function renderResultGifs( )
renderResultGifs: async (url, requestType) => {
domHandling.resetSearchField(); // LIMPIA EL INPUT DE BUSQUEDA
const gifsObject = await giphyApi.getSearchResults(url, requestType); // FETCH A GIPHY.
const printedTags = []; // ALMACENA LOS TITULOS YA IMPRESOS
//COMO TAGS RELACIONADOS PARA QUE NO SE REPITAN
let gifCounter = 0; // ESTE COUNTER ME PERMITE ASIGNARLE ID A LOS
//ELEMENTOS DINAMICOS PARA MANIPULARLOS EN EL DOM.
let spanCounter = 0; // CON ESTO SE LIMITA EL NUMERO DE
//IMAGENES DOBLES A UNA CANTIDAD MAXIMA (LINEA 46)
gifsObject.data.forEach(data => {
const gifUrl = data.images.original.url;
const gifWidth = data.images.original.width; /// OBTENGO DATOS DEL OBJETO
const gifHeigth = data.images.original.height;
const gifDescription = data.title.toLowerCase();
renderGifs.printGifs(gifUrl, gifCounter); //IMPRIME GIFS
renderGifs.printGifTags(gifDescription, gifCounter); // IMPRIME LOS TAGS
if (
url === giphyApi.searchEndpoint &&
!printedTags.includes(gifDescription) &&
gifDescription.length > 3
) {
domHandling.printRelatedButtons(gifDescription); // IMPRIME BOTONES AZULES
printedTags.push(gifDescription);
domHandling.resultsTitle.scrollIntoView();
}
if (spanCounter < 4 && +gifWidth > +gifHeigth * 1.2) {
renderGifs.applySpan(gifCounter); // APLICA SPAN DOBLE
spanCounter++;
}
gifCounter++;
});
},
renderResultGifs( )
resetSearchField( )
getSearchResults( )
printGifs( )
printGifsTags( )
printRelatedButtons( )
applySpan( )
Flujo de la Función

printGifs( )
printGifsTags( )
applySpan( )

printRelatedButtons( )
resetSearchField( )
Output

Ejemplo 2
Crear Gifs
(DOM)

1. Evento Onclick
const domHandling = {
createBtn: document.querySelector("#create-gif"),
....
handlerEvents: () => {
.....
domHandling.createBtn.onclick = () => {
domHandling.handleCreateSection();
domHandling.handleStartWindows();
domHandling.handleSuggestedGifs();
domHandling.handleSearchSection();
domHandling.handleNavBar();
domHandling.handleArrowBack();
myGuifos.renderMyGuifos();
};
}
}
2. Handlers
handleSuggestedGifs: () => {
const $SUGGESTED_SECTION = document.querySelector("#gifs-suggested");
$SUGGESTED_SECTION.className !== "hidden"
? ($SUGGESTED_SECTION.className = "hidden")
: ($SUGGESTED_SECTION.className = "suggested-gifs");
},
handleCreateSection: () => {
const $CREATE_SECTION = document.querySelector("#create-gif-section");
$CREATE_SECTION.className !== "hidden"
? ($CREATE_SECTION.className = "hidden")
: ($CREATE_SECTION.className = "create-gifos");
},
handleSearchSection: () => {
const $SEARCH_SECTION = document.querySelector("#search-section");
$SEARCH_SECTION.className !== "hidden"
? ($SEARCH_SECTION.className = "hidden")
: ($SEARCH_SECTION.className = "gifs-search");
},
handleNavBar: () => {
const $NAV_BAR = document.querySelector("nav");
$NAV_BAR.className !== "hidden"
? ($NAV_BAR.className = "hidden")
: ($NAV_BAR.className = "gifs-search");
},

handleSuggestedGifs( )
handleSearchSection( )
handleNavbar( )
3. Output (Ocultar)

handleCreateSetion( )
handleStartWindows( )
handleArrowback( )
handleMyGuifos( )
3. Output (Mostrar)
Proyecto guifOS
By Alexis Lazzuri
Proyecto guifOS
- 2,271