Welcome to iOS100
iOS Platform Development Introduction
Hello World!

I work at Integrity as a Project and Engineering Lead
I've been doing mobile development for 5 years...
iOS
Android
HTML5,CSS3,JS
I Love Rock Climbing
This is my dog Dexter...

NSLog(@"I am %@", Person.info);
What is your name?
What do you do?
What kind of programming experience do you have?
What is your favorite app?
Course Description
- As you go though this class, you will learn Xcode, Apple’s integrated development environment to create a few simple iOS apps.
- You will learn the basics of programming with Objective-C, the language that powers all Mac and iOS apps and frameworks.
- You will also explore the Cocoa Touch framework and learn how to build and test your apps in the iOS Simulator.
Course Topics
The Apple Ecosystem

Macs
- MacBook Air
- MacBook Pro
- Mac mini
- Mac mini server
- iMac
-
Mac Pro
These systems use Mac OSX as the native operating system
iDevices
- iPhone (5C, 5S, 6, 6 +)
- iPod (shuffle, nano, touch)
- iPad (retina, mini retina, mini, air)
These devices use an OS that is derived from OS X, however it has functionalities that are different enough and specific enough only to these touch devices and is named iOS.
Different, but the same...

Xcode
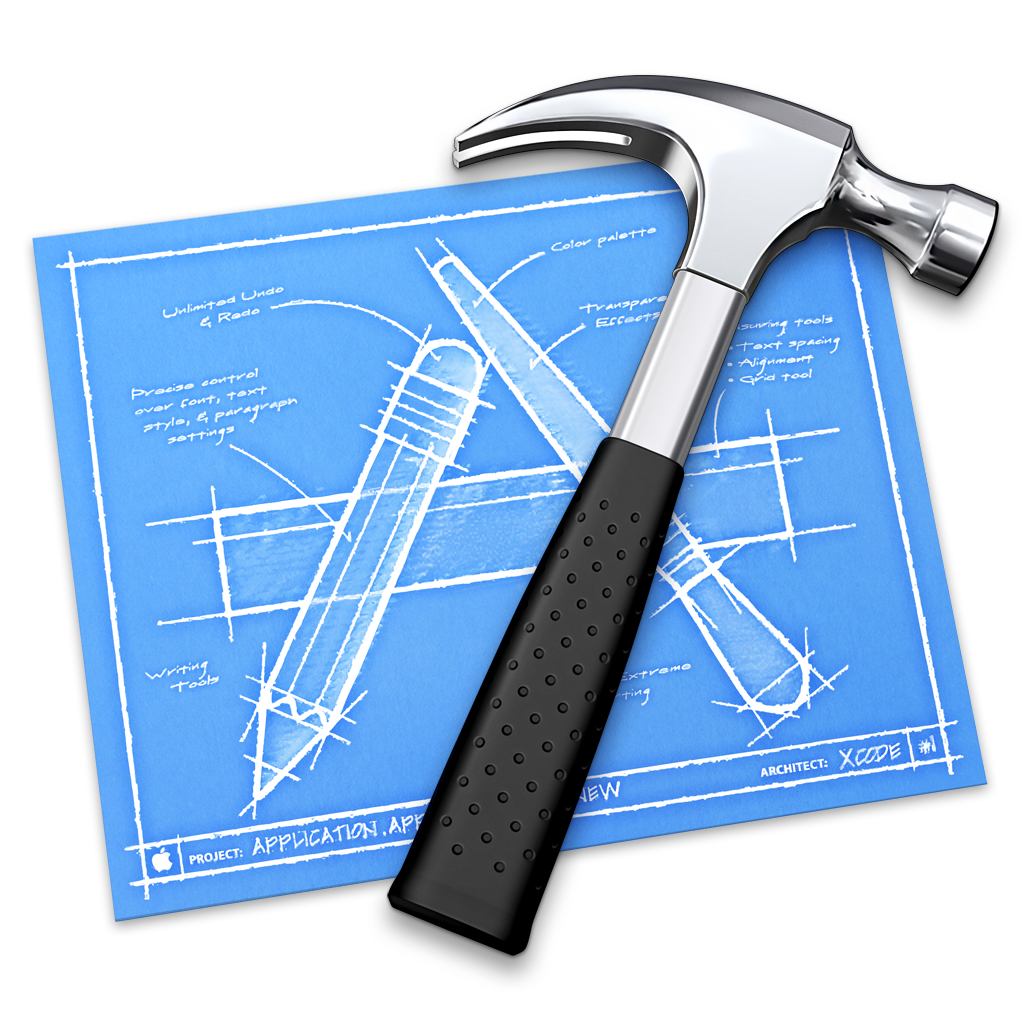
Some of the latest features in Xcode 6
- Swift
- Live Rendering
- View Debugging
- Performance Testing Improvements
- Storyboards for OS X
- Extensions and Frameworks
- Game Building
- Localization Improvements
- Xcode Server
Getting Around in Xcode 6
iOS Simulator
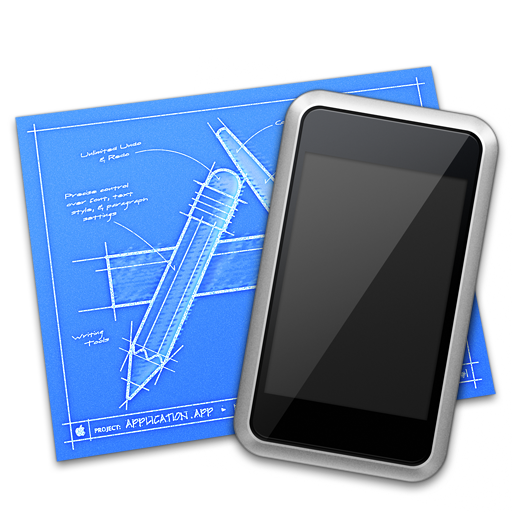
Limitations
-
It uses the Mac OS X versions of the low-level system frameworks, instead of the actual frameworks that run on the device.
-
It uses your Mac's hardware and memory. (Does not provide fully accurate performance metrics)
-
Xcode automatically installs your app onto the simulator but does not let you install apps from the app store.
Limitations
- Certain frameworks are not available:
- External Accessory
- Media Player
- Message UI
- Event Kit
- Store Kit
- No support for:
- Apple Push Services
- Reminders
-
Maximum of two finger gestures supported.
iOS Developer Program

-
iOS Developer Center
- Test apps on your own devices
- Distribute your apps in the app store
iOS Developer Enterprise Program

Sounds Simple...
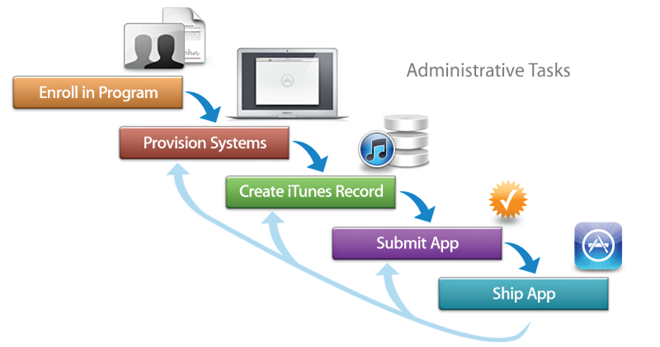
Well, not quite...
App Review Guidelines
- We have lots of kids downloading lots of Apps. Parental controls work great to protect kids, but you have to do your part too. So know that we're keeping an eye out for the kids.
- We have hundreds of thousands of Apps in the App Store. If your App doesn't do something useful, unique or provide some form of lasting entertainment, it may not be accepted.
- If your App looks like it was cobbled together in a few days, or you're trying to get your first practice App into the store to impress your friends, please brace yourself for rejection. We have lots of serious developers who don't want their quality Apps to be surrounded by amateur hour.
App Review Guidelines Continued...
- We will reject Apps for any content or behavior that we believe is over the line. What line, you ask? Well, as a Supreme Court Justice once said, "I'll know it when I see it". And we think that you will also know it when you cross it.
- If your App is rejected, we have a Review Board that you can appeal to. If you run to the press and trash us, it never helps.
- If you attempt to cheat the system (for example, by trying to trick the review process, steal data from users, copy another developer's work, or manipulate the ratings) your Apps will be removed from the store and you will be expelled from the developer program.
App Review Guidelines Continued...
... We love this stuff too, and honor what you do. We're really trying our best to create the best platform in the world for you to express your talents and make a living too. If it sounds like we're control freaks, well, maybe it's because we're so committed to our users and making sure they have a quality experience with our products. Just like almost all of you are too.
iOS Human Interface Guidelines
These are important too!
iOS SDK Features
Cocoa Touch
- UIKit
- Foundation
- Multi-Touch Gestures
iOS SDK Features
Networking Services
- Store Kit
- Push Notifications
- Game Kit
- iAd
- Newsstand
- Core Location
- Map Kit
- Networking
- Accelerate
iOS SDK Features
Media
- Graphics & Animation
- Audio
- Built-in Cameras
- Photo Library
- Media Library
iOS SDK Features
Core Services
- Inter-app Messaging
- Messaging
- In-app SMS
- Cut, Copy, and Paste
- Gyro + Accelerometer
- Multitasking
- Address Book
- Compass
- Electronic Accessories
- Quick Look
How do we access these features?
A framework is a directory that includes a shared library, header files to access the code stored in that library, and other resources such as image and sound files. A shared library defines methods that apps can call.
Most apps link to the Foundation, UIKit, and Core Graphics frameworks
The Foundation Framework
- Provides many primitive object classes and data types
- Almost all other frameworks are built off of it
- You can use Foundation to:
- Create and manage collections such as arrays and dictionaries
- Create and manage strings
- Access images and other resources stored in your app
- Post and observe notifications
- Create date and time objects
- Automatically discover devices on IP networks
- Manipulate URL streams
- Execute code asynchronously
UIKit
- Provides Classes to Create a Graphical, Event-Driven User Interface
- All iOS apps are based on UIKit. You can’t ship an app without this framework.
- You can use UIKit to:
- Construct and manage your user interface
- Handle user events
- Present fonts, colors, and images
- Perform basic animation
- Create custom user interface elements
Other Important Frameworks
-
Core Animation
- Use Core Animation to create rich user experiences from an easy programming model based on compositing independent layers of graphics. Learn more
-
Core Audio
- Core Audio is the professional-grade technology for playing, processing and recording audio, making it easy to add powerful audio features to your application. Learn more
-
Core Data
- Core Data provides an object-oriented data management solution that is easy to use and understand, yet is built to handle the data model needs of any application, large or small.
Note
-
Each framework corresponds to a layer of the iOS stack.
-
Each layer builds on the ones below it.
Always use higher-level frameworks instead of lower-level frameworks whenever possible.
Higher-level frameworks provide object-oriented abstractions for lower-level constructs.
These abstractions usually reduce the amount of code you have to write!
Object Oriented Programming
(OOP)
Objects
- Combines
- Properties and behavior
- Data and operations on that data
- Objects contain a group of related functions and a data structure that serves those functions.
- Functions are known as the object’s methods, and the fields of it's data structure are it's instance variables.
- Objects exist as instances of a class.
Class
- A blueprint of an object.
- Defines an objects methods and instance variables.
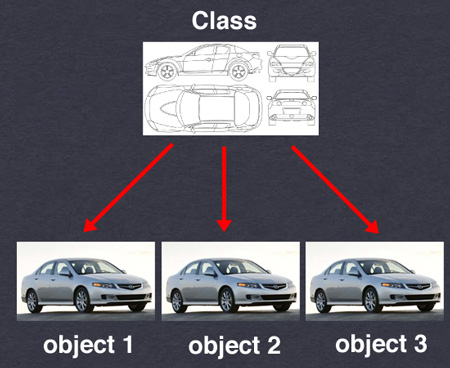
Messaging
- Objects communicate with each other through messaging.
- Messages are requests to perform a specific method of an object.

Methods of Abstraction
Encapsulation
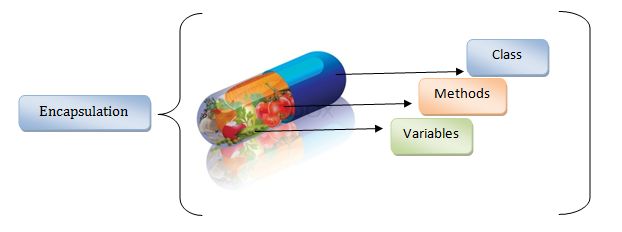
In Objective-C, method implementations and instance variables are hidden inside the object and are not visible outside of it.
Polymorphism
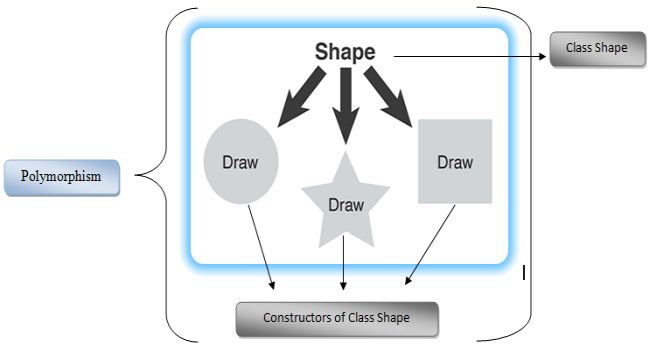
The ability of different objects to respond, each in its own way, to identical messages is called polymorphism.
Inheritance

Class Hierarchies
-
Any class can be used as a superclass for a new class definition.
A class can simultaneously be a subclass of another class, and a superclass for its own subclasses.
Any number of classes can thus be linked in a hierarchy of inheritance.
Every class inherits from the root class and is the accumulation of all the class definitions in its inheritance chain.
Cocoa & Cocoa Touch Root Class
NSObject
Everything else is a subclass
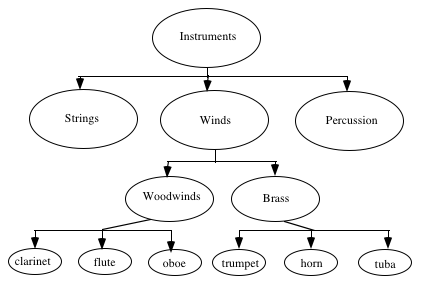
-
Subclasses tend to fill out a superclass definition, making it more specific and specialized.
-
They add, and sometimes replace, code rather than subtract it.
Model-View-Controller
(MVC)
Benefits of MVC
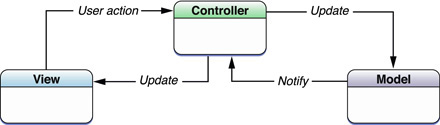
- Many objects in these programs tend to be more reusable.
- Their interfaces tend to be better defined.
- The programs overall are more adaptable to changing requirements.
- They are more easily extensible than programs that are not based on MVC.
Model Objects
-
Models represent knowledge.
- Data
- Model objects encapsulate data and basic behaviors
- A model could be a single object or it could be some structure of objects.
- Classe(s)
- File(s)
- Database(s)
- A model object should never be concerned with interface and presentation issues.
View Objects
- View objects know how to display information though the user interface.
- A view should not be responsible for storing the data it is displaying.
- A view object can be in charge of displaying just one part of a model object, or a whole model object, or even many different model objects.
- The UIKit framework defines a large number of view objects and provides many of them in the Interface Builder library.
- Using UIKit view objects ensures a high level of consistency in behavior across applications. (A button always behaves like a button! )
Controller Objects
- A controller object acts as the intermediary between the application's view objects and its model objects.
- Typically performs one or all of the following...
- Establishing connections between objects and performing other set-up tasks.
- Manage the life cycles of other objects.
- Decides what to do with a users input from the view.
- Updates the view when a model's data has changed
Objective-C Basics
iOS100 - iOS Platform Development Introduction - Day 1
By Alex Rodriguez
iOS100 - iOS Platform Development Introduction - Day 1
Today we will cover: How to setup an iOS project, How to create a ViewController & View (XIB), How to setup up and configuring view objects, How to setup and connect outlets, How to setup and connect actions, Created model objects using NSArrays, How to set the root ViewController to display our view.
- 2,707