Welcome to
jQuery 110
Day 1
Hello World!
I am Alex Rodriguez

I work at Integrity as a Project and Engineering Lead
I've been doing web development professionally for 5+ years
JS
PHP
Java
Objective - C
I Love Rock Climbing
This is my dog Dexter...

console.log($person.info);
What is your name?
Where do you work?
What kind of programming experience do you have?
What are you looking to get out of this class?
Course Topics
- jQuery Selectors
- DOM traversal and modification
- Animation Effects
- Interaction Helpers
- Interface components like Datepickers and Accordions
- How to use plugins to extend jQuery’s capabilities
- How to make asynchronous JavaScript and XML calls
Prerequisites
-
HTML5 and CSS3: Essentials
-
HTML5 and CSS3: Advanced
-
Using JavaScript or equivalent experience
To learn jQuery, we must learn JavaScript...
What is it?
-
JavaScript (JS) is a dynamic computer programming language.
-
It is most commonly used as part of web browsers, whose implementations allow client-side scripts to interact with the user, control the browser, communicate asynchronously, and alter the document content that is displayed.
-
It is also being used in server-side programming, game development and the creation of desktop and mobile applications.
Definition:
JavaScript is a prototype-based scripting language with dynamic typing and has first-class functions.
What!?
Prototype-Based...
A style of object-oriented programming in which behavior reuse (known as inheritance) is performed via a process of cloning existing objects that serve as prototypes.
This model can also be known as prototypal, prototype-oriented, classless, or instance-based programming.
Delegation is the language feature that supports prototype-based programming.
In javascript, every object has a link to the object which created it, forming a chain. When an object is asked for a property that it does not have, its parent object is asked continually up the chain until the property is found or until the root object is reached.
Scripting Language...
-
A scripting language is a programming language that allows control of one or more software applications.
-
"Scripts" are distinct from the core code of the application, as they are usually written in a different language.
-
Scripts are often interpreted from source code or bytecode, whereas the applications they control are traditionally compiled to native machine code.
-
Scripting languages are nearly always embedded in the applications they control.
Dynamic...
A class of high-level programming languages which, at runtime, execute many common programming behaviors that static programming languages perform during compilation.
These behaviors could include extension of the program, by adding new code, by extending objects and definitions, or by modifying the type system.
This allows us to dynamically infer the types of objects, instead of forcing you to define them, and then enforce those types when the program runs in the interpreter.
In JavaScript, we define a variable with the keyword var, instead of a type like int or char. We don’t know the type of this variable and we don’t need to until we actually want to access it.
If it walks like a duck and it quacks like a duck, then it is a duck.
First-class Functions...
-
Treats functions as first-class citizens.
- This means the language supports:
- Passing functions as arguments to other functions.
- Returning them as the values from other functions.
- Assigning them to variables or storing them in data structures.
- Anonymous function (AKA function constant, function literal, or lambda function)
- A function defined, and possibly called, without being bound to an identifier.
Most Importantly...
JavaScript != Java

Similar but different...
Some of the main differences:
-
Java is a statically typed language; JS is dynamic.
- Java is class-based; JS is prototype-based.
- Java uses block-based scoping; JavaScript uses function-based scoping.
- Java is a full feature, robust, LARGE, programming language. JS is a scripting language.
This sounds hard...
Don't be scared!
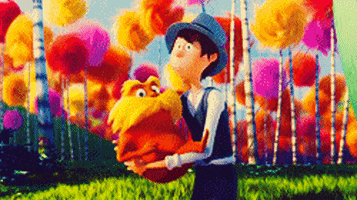
TO THE RESCUE!
What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers. With a combination of versatility and extensibility, jQuery has changed the way that millions of people write JavaScript.
Benefits of jQuery
- It dramatically reduces the amount of code that needs to be written compared to pure Javascript.
- Less development time
- More readable code
- It is easier to understand than scripting with pure Javascript.
- The documentation is extremely well organized and the community is very active with helping out anyone who may be struggling with a snippet of code.
- It makes using Ajax extremely easy.
- Handles a lot of cross browser JS compatibility issues for you.
Common Usages
Find something, then do something...
- Find - HTML elements on a page
- Change - HTML elements on a page
- Listen - To user actions
- Animate - Content on a page
- Communicate - Over the network to fetch and exchange data
Document Object Model
- Commonly referred to as "The DOM"
- A tree-like structure generated by the browser
- Describes relationships in HTML
- Nodes
- Parent
- Child
- Sibling
- API for CSS & JS
- Allows us to find and manipulate HTML elements
The DOM
DOM Nodes

- Element's text and attributes are considered nodes
- Nodes can have Parent and Child relationships
- Special Relationships
- First Child
- Last Child
Generating the DOM...
Issues with the DOM
Each browser has a slightly different DOM interface...

How jQuery accesses The DOM
- A page can't be manipulated safely until the document is "ready."
jQuery(document).ready(function(){
// Your code...
});
// OR
$(function() {
// Your code...
});
-
jQuery detects this state of readiness for you.
- Code included inside $( document ).ready() will only run once the page Document Object Model (DOM) is ready for JavaScript code to execute
Huh?
jQuery(document).ready(function(){
// Your code...
});
// OR
$(function() {
// Your code...
});
window.jQuery = window.$ = jQuery;
http://code.jquery.com/jquery-1.11.0.js
Getting Started with jQuery
- Download jQuery @ http://jquery.com/ (OR use a CDN)
- Load it into your HTML document
3. Start using it!
<script src="application.js"</script>
<script src="application.js"</script>
- Using a CDN can provide decreased latency, increased parallelism and better caching
Demo
Setting Up & Using jQuery
FIND Something...
Using jQuery Selectors!
Selectors

A pure-JavaScript CSS selector engine
jQuery uses Sizzle, an engine also created by the jQuery Foundation (but available as a standalone) to use as it's selector engine.
Basic Selectors
With jQuery you can query the DOM using the CSS selectors that we’re already familiar with...
- ID
- Class
- Element
- Attribute
- Compound CSS Selector
ID Selectors
// jQuery
$('#myID');
// JavaScript
document.getElementById('myID');
Returns a single Node of the DOM.
Class Selectors
// jQuery
$('.myClass');
// JavaScript
document.getElementsByClassName('myClass');
The difference between jQuery and native JavaScript when using selectors like these, is that they return a NodeList that you then have to deal with.
jQuery takes care of all this for you, covering up what's really happening...
Whats really happening...
-
A NodeList is a host object and is not subject to the usual rules that apply to native JavaScript objects.
-
The NodeList object consists of a length property and access to its members via square bracket property access syntax. Similar to and Array but NOT an Array.
-
You can use this API to create an Array containing a snapshot of the NodeList's members
var nodeList = document.getElementsByTagName('myClass');
var nodeArray = [];
for (var i = 0; i < nodeList.length; ++i) {
nodeArray[i] = nodeList[i];
}
Element Selectors
// jQuery
$('div');
// JavaScript
document.getElementsByTagName('div');
Attribute Selectors
// jQuery
$("input[name='first_name']");
// JavaScript
function getElementByAttributeValue(attribute, value) { var allElements = document.getElementsByTagName('*'); for (var i = 0; i < allElements.length; i++) { if (allElements[i].getAttribute(attribute) == value) { return allElements[i]; } } }
Compound CSS Selector
// jQuery
$("#contents ul.people li");
// JavaScript
// Would be too long to fit onto a slide...
Exercise 1
<h1></h1>
<ul id="places">
<li>Chicago, IL</li>
<li>Boulder, CO</li>
<li class="promo">New York, NY</li>
</ul>
Objectives:
-
Find the <h1> element and change its text to say "Where do you want to go?"
-
Find all of the <li> elements and modify their text to say "St. Louis, MO"
- Find the element with the class of "promo" and make its text red
Pseudo Selectors
CSS style pseudo-selectors are available to you as well as attribute selectors.
Attribute selectors in jQuery offer regular expression-like functionality and can even be combined in ways that provide conditional functionality.
Some things to note...
Choosing Selectors
- Choosing good selectors is one way to improve JavaScript's performance.
- A little specificity – for example, including an element type when selecting elements by class name – can go a long way.
- On the other hand, too much specificity can be a bad thing.
- A selector such as #myTable thead tr th.special is overkill if a selector such as #myTable th.special will get the job done.
- Wherever possible, make selections using IDs, class names, and tag names.
Does My Selection Contain Any Elements?
Once you've made a selection, you'll often want to know whether you have anything to work with.
A common mistake is to use:
if ( $( "div.foo" ) ) {
...
}
This won't work. When a selection is made using $(), an object is always returned, and objects always evaluate to true. Even if the selection doesn't contain any elements, the code inside the if statement will still run.
The best way to determine if there are any elements is to test the selection's .length property, which tells you how many elements were selected.
If the answer is 0, the .length property will evaluate to false when used as a boolean value:
// Testing whether a selection contains elements.
if ( $( "div.foo" ).length ) { ... }
Saving Selections
- jQuery doesn't cache elements for you. If you've made a selection that you might need to make again, you should save the selection in a variable rather than making the selection repeatedly.
- Once the selection is stored in a variable, you can call jQuery methods on the variable just like you would have called them on the original selection.
- A selection only fetches the elements that are on the page at the time the selection is made. If elements are added to the page later, you'll have to repeat the selection or otherwise add them to the selection stored in the variable.
Traversing the DOM
At this point, we know that we can search, or query the DOM using the CSS selectors that we’re already familiar with, but what about moving around the DOM after we've made a selection?
Traversing can be broken down into three basic parts: parents, children, and siblings.
Basic Relationships
<ul>
<li>
<span>
<i>Foo</i>
</span>
</li>
<li>Bar</li>
</ul>
-
The first list item is a child of the unordered list.
-
The unordered list is the parent of both list items.
-
The span is a descendant of the unordered list.
-
The unordered list is an ancestor of everything inside of it.
-
The two list items are siblings.
Parents
<div class="grandparent">
<div class="parent">
<div class="child">
<span class="subchild"></span>
</div>
</div>
<div class="surrogateParent1"></div>
<div class="surrogateParent2"></div>
</div>
The methods for finding the parents from a selection include .parent(), .parents(), .parentsUntil(), and .closest()
jQuery 110 Day 1
By Alex Rodriguez
jQuery 110 Day 1
- 2,042