Getting Started with RICH
Almog Yalinewich
CITA
15.8.18
Preparation
Useful links
Installation
git clone https://github.com/bolverk/huji-rich.git
make set_environ_vars.sh && `cat set_environ_vars.sh`
Tests
cd tests && make
Installation can take 20m - 1h
you should already have RICH installed
Prerequisits
Object oriented programming
Encapsulation
Inheritence
Polymorphism
Abstraction
classes - objects with their own properties and methods
Numerics
Voronoi Tessellation

Voronoi cell - loci of points closest to a certain mesh generating point than any other mesh generating point
Hydro values at MSG
fluxes on sides
Moving Tessellation

Further reading
Showcase
Into the Matrix
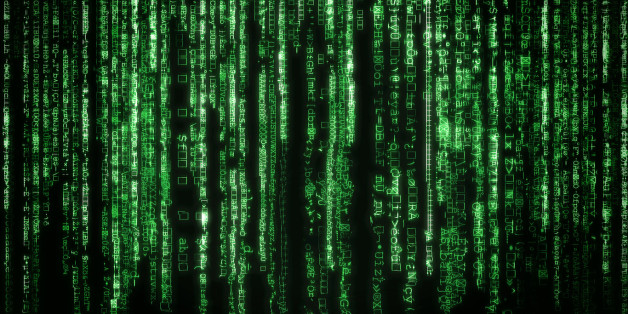
The main file
Let's look at the sedov taylor test
int main(void)
{
SimData sim_data;
hdsim& sim = sim_data.getSim();
for (size_t i = 0; i < 100; ++i)
{
sim.TimeAdvance();
}
write_snapshot_to_hdf5(sim, "final.h5");
return 0;
}
intialisation
time evolution
output
ignore extra code for parallel execution
Simulation Class
class SimData
{
public:
SimData(void):
pg_(),
width_(1),
outer_(0,width_,width_,0),
init_points_(cartesian_mesh(50,50,outer_.getBoundary().first,
outer_.getBoundary().second)),
tess_(init_points_, outer_),
eos_(5./3.),
point_motion_(),
sb_(),
rs_(),
force_(),
tsf_(0.3),
fc_(rs_),
eu_(),
cu_(),
sim_(tess_,
outer_,
pg_,
calc_init_cond(tess_),
eos_,
point_motion_,
sb_,
force_,
tsf_,
fc_,
eu_,
cu_) {}
hdsim& getSim(void)
{
return sim_;
}
private:
const SlabSymmetry pg_;
const double width_;
const SquareBox outer_;
const vector<Vector2D> init_points_;
VoronoiMesh tess_;
const IdealGas eos_;
Lagrangian point_motion_;
const StationaryBox sb_;
const Hllc rs_;
ZeroForce force_;
const SimpleCFL tsf_;
const SimpleFluxCalculator fc_;
const SimpleExtensiveUpdater eu_;
const SimpleCellUpdater cu_;
hdsim sim_;
};
}
Visualisation
see vis_demo.ipynb in the tests folder
tricontourf - faster but smear features
PatchCollection - slower, but shows tessellation
Running your own simulation
Makefile uses RICH_ROOT to find dependencies
LINT_FLAGS = -Werror -Wall -Wextra -pedantic -Wno-long-long -Wfatal-errors -Weffc++ -Wshadow -Wmissing-declarations -Wconversion -O3 -march=native
CXX = g++
build/rich.exe: build/rich.o
$(CXX) $< $(RICH_ROOT)/library_production/librich.a -L $(HDF5_LIB_PATH) -lhdf5 -lhdf5_cpp -lz -o $@
build/rich.o: source/rich.cpp build
$(CXX) -c $(LINT_FLAGS) $< -o $@ -I $(RICH_ROOT)
build:
mkdir build
Questions?
getting started with rich
By almog yalinewich
getting started with rich
- 358