React, Redux
and
Chrome DevTools
Amanpreet Singh
@apsdehal
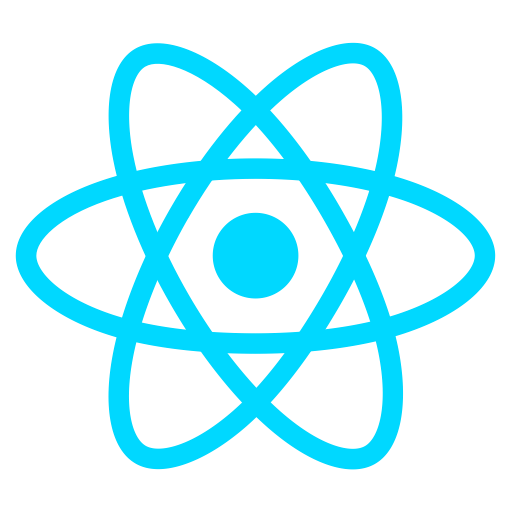
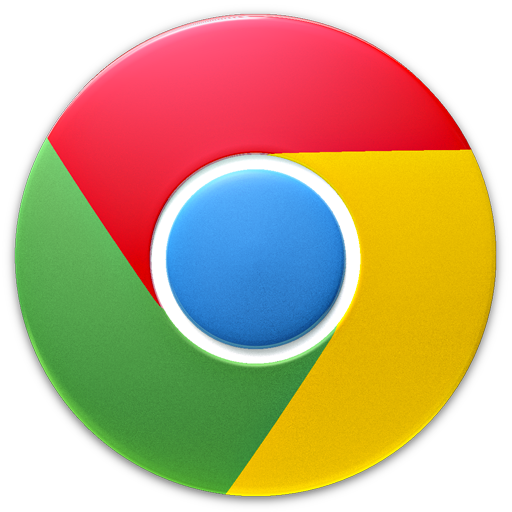
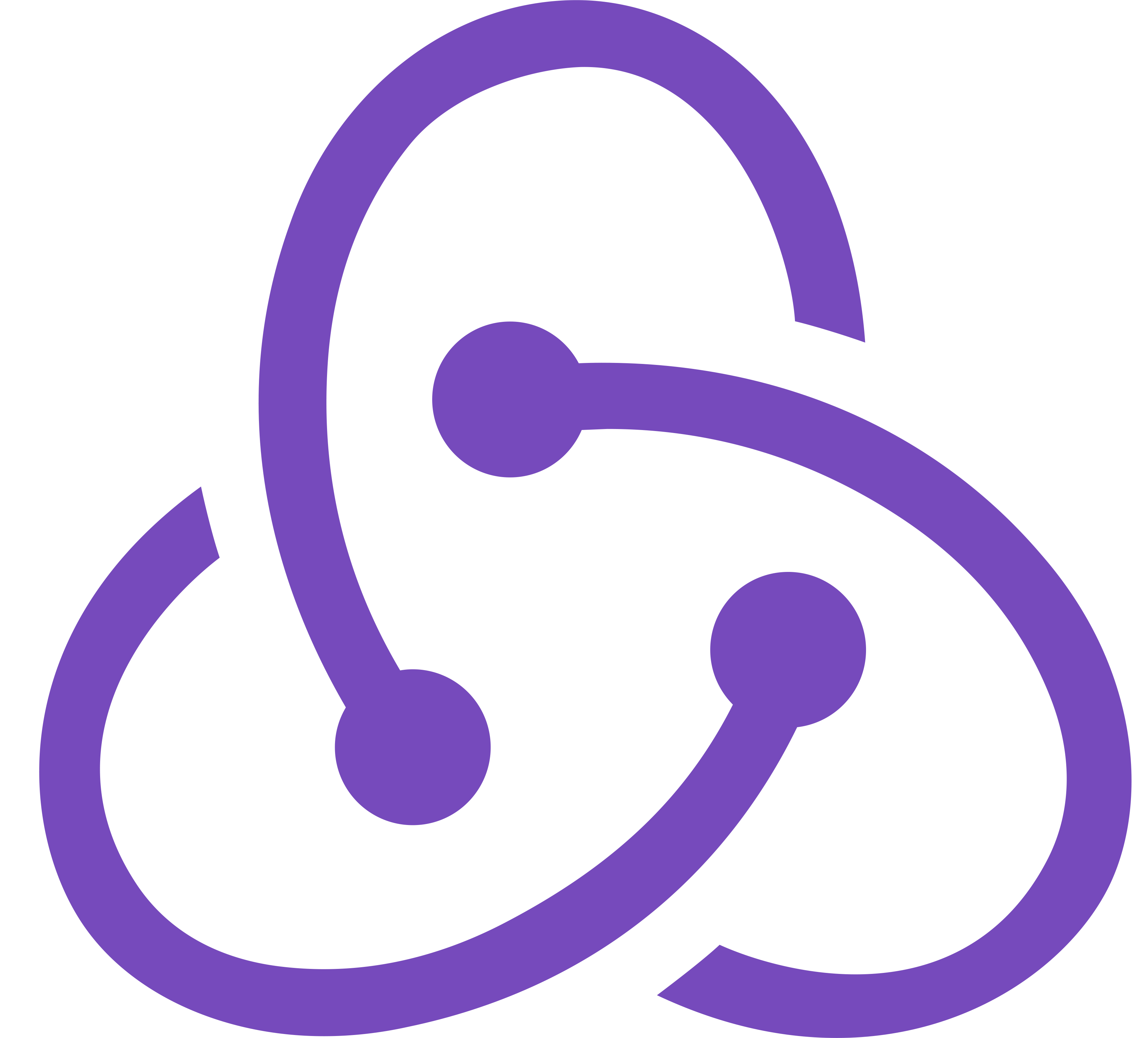
Agenda
- React and its usage
- Demo for React
- How Redux comes into play
- Demo for Redux
- Demo for using Chrome DevTools
A library for creating user interfaces
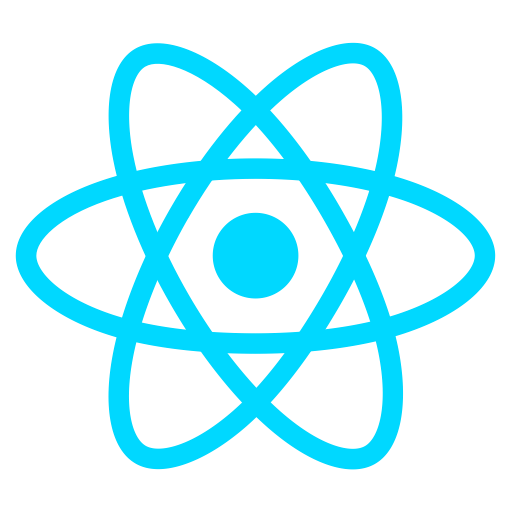
Model
View
Virtual DOM
React
Rerender everything on every update
One-way Data Binding
Title text field
let title = "";
Event
Watcher
Virtual DOM
JSX
-
JavaScript syntax that looks like XML
const SpecTile = props => {
let originalBackgroundTitle = 'rgba(0, 0, 0, 0.4)';
let { keyProp, selectedKey, handleGridTileClick, image, images } = props;
return (
<GridTile
style={styles.hoverCursorPointer}
titleBackground={
selectedKey === keyProp ? blue500 : originalBackgroundTitle
}
title={image.gameName}
onClick={e => {
handleGridTileClick(keyProp, image);
}}
>
<img
src={images[image.gameIcon512x512].downloadURL}
alt={images[image.gameIcon512x512].id}
/>
</GridTile>
);
};
Demos & Code
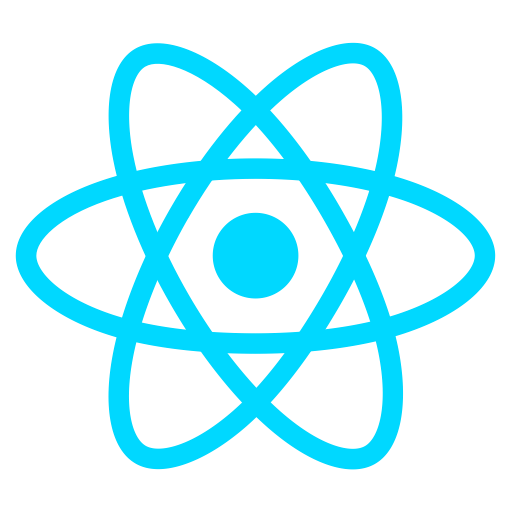
Four Things
- Components
- Props
- State
- Lifecycle Events
Presentational and Container Components
-
Better separation of concerns
- Skinny vs Fat controllers
- Eventually, we end up we too much props passing
- This helps with that
- Read more at https://goo.gl/ypJ1TP
Presentational and Container Components
const SearchBar = (props) => {
return <input type="search"
onChange={(e, newValue) => {props.handleSearch(e, newValue)}}/>
}
class App extends React.Component {
state = {
searchText: ''
};
handleSearch(e, newValue) {
this.setState({...this.state, searchText: newValue});
}
render() {
return (<SearchBar handleSearch={this.handleSearch.bind(this)} />);
}
}
Redux
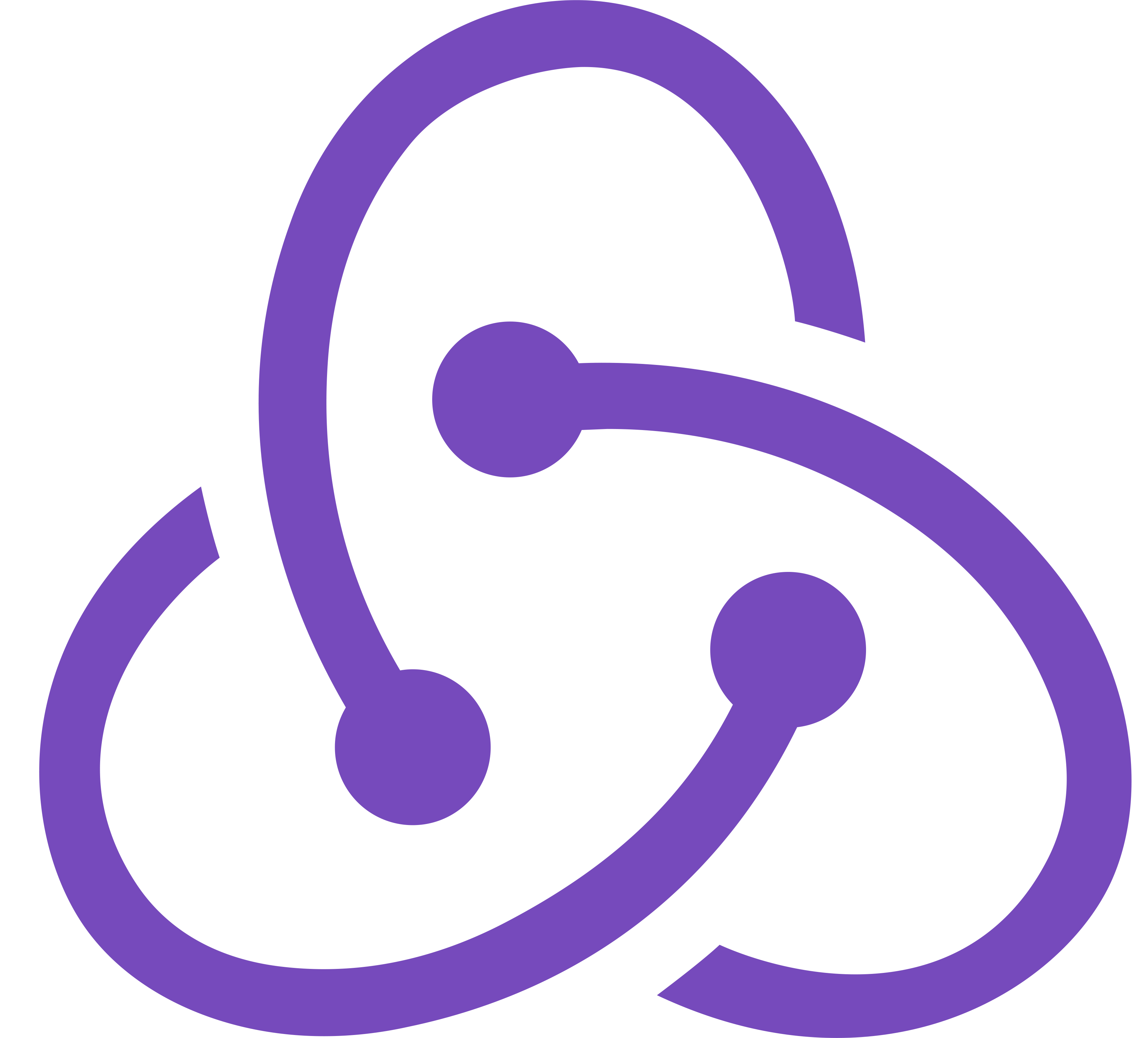
Predictable State Container for JavaScript Apps
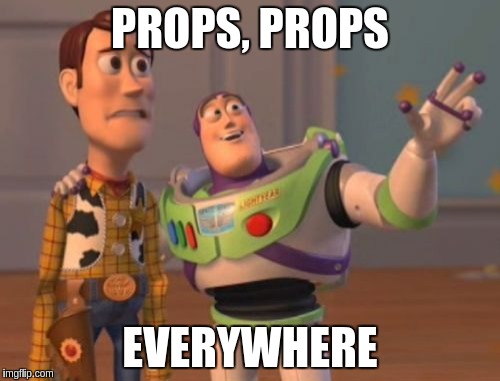
Motivation
-
As applications become complex, managing state becomes pain
-
Libraries like react remove asynchronicity and direct DOM manipulation but you still need to maintain state
-
State bloats with every increasing demands of a UI platform
Three Principles
- Single source of truth
- State is read only, it can be only changed through actions
- Changes are made through pure functions called reducers
Single Source of Truth
The state of your whole application is stored in an object tree within a single store.
State is read-only
The only way to change the state is to emit an action, an object describing what happened.
Changes are made with pure functions
To specify how the state tree is transformed by actions, you write pure reducers.
These reducers take in old state and an action and return back new state
Basic Principle
(previousState, action) => newState
Data Flow Diagram
Example
Chrome DevTools
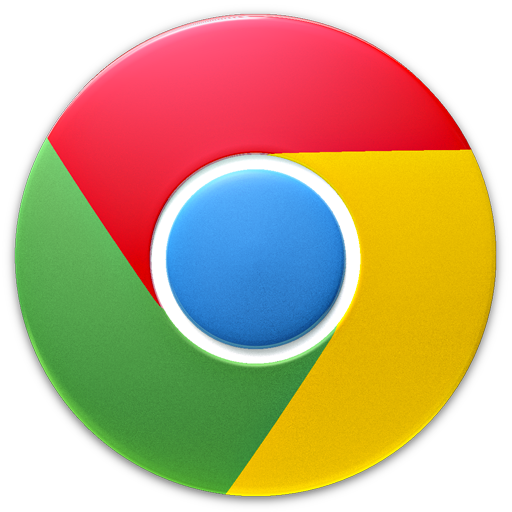
Thanks for your attention!
Questions?
React, Redux and Chrome DevTools
By Amanpreet Singh
React, Redux and Chrome DevTools
- 951