Protractor
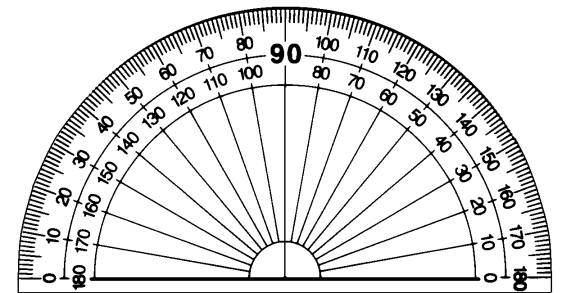
What Is Protractor
- An end-to-end test framework for AngularJS applications
- A wrapper on top of WebDriverJS to control browsers
- A Node.js program


Protractor
- Framework to simulate user interaction with the browser
- Written by Julie Ralph -Software Engineer in Test at Google, Lead developer for Protractor
- First version release in 2013
- Public Github project
- https://github.com/angular/protractor


@juliemr is very active
Protractor Is Using:
- WebDriverJS
- Selenium
- Jasmine



System Structure

WebDriverJS
- WebDriver refers to the language bindings and the implementation of the browser control code
- WebDriverJS has an important difference from other languages - it is asynchronous
Webdriver
VS
WebdriverJS
driver.get(page1);
driver.click(E1);
JAVA
driver.get(page1).then(function () {
driver.click(E1);
})
Javascript
So Why Protractor?
- No "Sleep" and "Wait" (control flow)
- Optimizes times
- Advantage for angular attributes
- Better to communicate with developers (This is for us)
Control Flow
- Managing entirely asynchronous API (WebdriverJS)
- Queue of pending promises
it('should find an element by text input model', function() {
browser.get('app/index.html#/form');
var username = element(by.model('username'));
username.clear();
username.sendKeys('Jane Doe');
var name = element(by.binding('username'));
expect(name.getText()).toEqual('Jane Doe');
// Point A
});
- At Point A, none of the tasks are executed yet. The browser.get method is in the front of the control flow queue, and the name.getText() call is at the back.
The value of name.getText() at Point A is an unresolved promise object.
driver.get("http://www.google.com").
then(function() {
return driver.findElement(By.name("q"));
}).
then(function(q) {
return q.sendKeys("webdriver");
}).
then(function() {
return driver.findElement(By.name("btnG"));
}).
then(function(btnG) {
return btnG.click();
}).
then(function() {
return driver.getTitle();
}).
then(function(title) {
assertEquals("webdriver - Google Search", title);
});
Avoid of doing this:
expect is adapted to Promises
expect(name.getText()).toEqual('Jane Doe');
Instead of this:
name.getText().then(function (name) {
expect(name).toEqual('jane Doe')
})
Easy To Catch Elements In the DOM
Protractor Global Variables
- element
- browser
- by
element, by
- element (by.css('#search'))
- element(by.model('name'))
by.model
In your test
In your application
element(by.model('myModel'));
<input ng-model="myModel" />
by.repeater
In your test
In your application
element( by.repeater('user in users'));
<ul>
<li ng-repeat="user in users" class="a">
<li ng-repeat="user in users" class="b">
</li>
</ul>
browser
- browser.get(url)
- browser.switchTo().iframe(indexOrName)
- browser.wait(1000)
element(by.css('#searchBtn')) === $('#searchBtn')
Use the '$' Symbole, It's equal
Protractor
- WebDriver
-
Selenium
-
Jasmine


Jasmine
- Jasmine is a testing framework for javascript
- Protractor uses Jasmine for its test syntax
- describe, it, expect, toBe...
- Does not rely on browser, DOMS
describe(‘A suite’, function() {
it(‘contains spec with an expectation’, function() {
expect(true).toBe(true);
});
});

Test Structure

Running Tests
- conf.js
- spec.js
conf.js
- Tells the files - specs - location
- How to run
- How to connect to selenium
- Browser options
exports.config = {
seleniumAddress: 'http://localhost:4444/wd/hub',
specs: ['angularPage-spec.js'],
capabilities: {
browserName: 'chrome',
'chromeOptions': {
'loggingPrefs': {
'browser': 'ALL'
}
}
};
spec.js
- The tests
How to run?
protractor someConfFile.js

In the terminal:
Command
Let See How It Really Works!
(*at this point presenting live demo)
Protractor + Wix
- Switching from ng-app pages ( Hotels, App Market, MA) to non ng-apps pages (Editor)
- Ignoring the protractor synchronisation (control flow)



Our Projects (Hotels, App-Market)
- NodeJS program
- Grunt
- Protractor (!)
- Jasmine
- Selenium


NodeJS
- Open source runtime environment for server-side and network app
- Uses the Google V8 Javascript engine to execute code
- npm

npm
- Node Package Manager
- Manage dependencies for an application


npm
Example - install Protractor

In Terminal

Example - Install Protractor

In our project:
- Creates 'Protractor' package in node-modules
Example - Install Protractor
Testing with non-ng pages

How the ???? do we deal with it?

Ignoring The Angular Synchronisation!

How?
browser.ignoreSynchronization = true;
Project Structure
- Page objects
- Tests specs


settings.js
Hotel BO Settings
Page object file
Page test file


Dealing with asynchronisation in our projects
Using the
protractor-infrastructure project





Example - waiting for the element to exist in the DOM

ElementProxy.js (protractor-infrastructure)
Test file

wrapper for selenium method
Thank You!
Ask me anything :)
Copy of Protractor
By danielagreen
Copy of Protractor
- 1,213