...or why we actually decided to have two type checkers instead of one...

From
To
ABOUT ME
- Ales Tsvil
- Frontend Architect in Elivar
- 8+ years of experience in web dev
- Functional Programming Enjoyer
- What are Static Types
- Flow
- TypeScript
- Flow vs TS
- So why Flow?
- And why TypeScript then?
- How to migrate
- Alternatives?
Static Types
- Require Types
- Compile Time Check
- Type Inference?
- Boilerplate?

DYNAMIC TYPES
- No Types
- Runtime Check
- Less Code?
- Error Prone?
class Cat {
voice = () => "nope.";
}
class Dog {
voice = () => "woof!";
}
class NuclearSafetyInspector {
voice = () => "D'oh!";
}
function say(speaker) {
console.log(speaker.voice());
}
say(new Dog());
say(new Cat());
say(new NuclearSafetyInspector());


class Cat {
voice = () => "nope.";
}
class Dog {
voice = () => "woof!";
}
class NuclearSafetyInspector {
voice = () => "D'oh!";
}
function say(speaker) {
console.log(speaker.voice());
}
say(new Dog());
say(new Cat());
say(new NuclearSafetyInspector());
Compiler Error!
interface CanSpeak {
voice(): string;
}
class Cat implements CanSpeak {
voice = () => "nope.";
}
class Dog implements CanSpeak {
voice = () => "woof!";
}
class NuclearSafetyInspector implements CanSpeak {
voice = () => "D'oh!";
}
function say(speaker: CanSpeak): void {
console.log(speaker.voice());
}
say(new Dog());
say(new Cat());
say(new NuclearSafetyInspector());


Static Type Checker
Created By Facebook
Has Type Inference
Plain JS is Valid Flow

// @flow
class Cat {
voice = () => "meow!";
}
class NuclearSafetyInspector {
voice = () => "D'oh!";
}
function say(speaker) {
console.log(speaker.voice());
}
say(new Cat());
say(new NuclearSafetyInspector());


// @flow
class Cat {
voice = () => "nope.";
}
class NuclearSafetyInspector {
voice = () => "D'oh!";
}
class Empty {}
function say(speaker) {
console.log(speaker.voice());
}
say(new Cat());
say(new NuclearSafetyInspector());
say(new Empty());


// @flow
class Cat {
voice = () => "nope.";
}
class NuclearSafetyInspector {
voice = () => "D'oh!";
}
class Empty {}
function say(speaker) {
console.log(speaker.voice());
}
say(new Cat());
say(new NuclearSafetyInspector());
say(new Empty());


Strongly Typed Programming Language
Created By Microsoft
Has Type Inference
Plain JS is Valid TS
type CanSpeak = {
voice(): string,
}
class Cat {
voice = () => "meow!";
}
class NuclearSafetyInspector {
voice = () => "D'oh!";
}
function say(speaker: CanSpeak): void {
console.log(speaker.voice());
}
say(new Cat());
say(new NuclearSafetyInspector());

VS


Any
Object, Enum
Union, Intersection
Mixin
Functions
Generics
Optional
Casting
Inference
✅
✅
✅
✅
✅
✅
✅
✅
✅
✅
✅
✅
✅
✅
✅
✅
✅
✅
and much more...
- Exact and Non-Exact Types
- Better Indexers
- Opaque Aliases
- Comments Syntax
- Type Spread Operator

- Conditional Types
- Declaration Merging
- Modules and Namespaces
- Function Overload
- Mapped Types
- Template Union Types
- Iterators and Generators
- Decorators and Metadata
- ...
THERE IS NO
SILVER BuLLET
const arr: number[] = [];
const a: number = arr[0];

// Command pattern imposibility
class A {
method1() {}
method2() {}
exec(command: "method1" | "method2") {
this[command](); //FlowError
// (this: any)[command]();
}
}



class Animal {}
class Dog extends Animal { bark() {} }
class Cat extends Animal { meow() {} }
const cats: Array<Cat> = [new Cat(), new Cat()];
const animals: Array<Animal> = cats;
animals[1] = new Dog();
cats[1].meow(); //TypeError!
class Animal {}
class Dog extends Animal { bark() {} }
class Cat extends Animal { meow() {} }
const cats: Array<Cat> = [new Cat(), new Cat()];
const animals: Array<Animal> = cats;
animals[1] = new Dog();
cats[1].meow(); //TypeError!
ALlmightY Any
(null as any).method(); //TS
(null: any).method(); //Flow

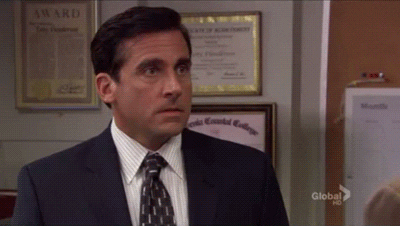
The reason to add more entropy
- Breaking Changes
- Exact Types
- Support (community, libs)
- Tooling
Breaking Changes

Exact Types
type NonExact = {
some: number;
};
const a: NonExact = { some: 1, a: "2" };
type Exact = {|
some: number;
|};
const b: Exact = { some: 1, a: "2" }; // Cause Error
Exact Types
type NonExact = {
some: number;
};
const a: NonExact = { some: 1, a: "2" };
type Exact = {|
some: number;
|};
const b: Exact = { some: 1, a: "2" }; // Cause Error
Support



TYPINGS


HOW TO MIGRATE

DEMO TIME!
MANY THANKS!
Flow vs TS
By diodredd
Flow vs TS
- 114