JavaScript
Coding w/ CORE + ADI
Workshop Topics
-
Introduction to JavaScript
- Introduction to jQuery
- Overview of some of the incredible Javascript libraries available
What is JavaScript?
- The programming language of the web and present in every web browser
- Can change the HTML and CSS of any website
- Is used for communicating with your server
- Is used for accessing APIs
It's all about the behavior of a website
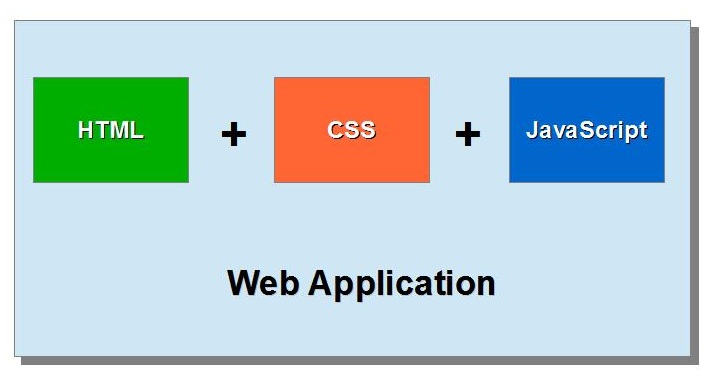
Tools for Tonight
-
http://jsfiddle.net/ - great way to create and share JavaScript code online
- Web browser console
- Chrome: Go to View -> Developer -> JavaScript Console
- Codecademy JavaScript Console: web console
- Sublime Text - Great text editor for JavaScript and web programming (download here)
Variables and Values
- JavaScript will automatically recognize numbers and mathematical expressions
-
>24
24
>6 + 4
10
>7 * 2 + 1
15
- You can assign these numbers to variables for later use
> var x = 13
> x
13
> var y = 7
> y + x
20
Strings & Concatenation
- We can also store text in JavaScript
-
> "Hello World"
Hello World
> var websiteTitle = "Facebook";
- We can log strings and other objects (aka print to the console)
> console.log(websiteTitle);
- We can also concatenate (aka add) strings together
> "Hello " + "Jane"
Hello Jane
> "How could you eat " + 8 + " hot dogs??"
How could you eat 8 hot dogs??
Conditionals
- Any expression that evaluates to true or false
-
> 3 > 5
false
> var s = true;
> s
true
- Can combine expressions with && (is true if both expressions are true) or || (is true if either is true)
-
> (1 > 3) || (3 > 1)
true
> var cookies = true
> var code = true
> cookies && code
true
While Loops
while (<insert expression that is true/false>) {
<do stuff>
}
For Loops
for ( <do before loop> ; <if this is true, run block> ; <do this after>) {
<do stuff here>
}
If/Else Statements
if (<something is true>) {
<do this>
} else {
<do this>
}
Functions
- Functions run a block of code whenever you call them
-
> function sayHi(name) {
}console.log("Hi " + name);
> sayHi("Don");
Hi Don
- Some functions come with JavaScript
-
alert("Hello");
- Functions can be stored as variables and passed to other functions
-
> var t = sayHi;
Objects
- In simplest sense, is just a dictionary of key-value pairs
> var batman = {
name: "Bruce Wayne",
enemy: "Joker",
playThemeSong: function() {
console.log("nananana Batman");
}
}
> batman.playThemeSong();
nananana Batman
> batman.name
Bruce Wayne
How to include JavaScript in your website
- Remember how we have different HTML elements that tell our web browser what to include in the site?
- Well there is also a <script></script> element that tells the web browser what javascript files to load with your website
<html>
<header>
</header><script src="flashingColors.js"></script>
<body><h1>Colors are flashing!!</h1>
</body>
</html>
Additional Resources
(Beginner)
Codecademy - link
Codeschool - link
(Intermediate)
All You Ever Want to Read About - book
(Advanced)
Design Patterns - book
jQuery
- A JavaScript library that let's you easily do the stuff that JavaScript was meant to do
- Find HTML elements and do stuff with them
- Execute code on an event like a user click or hover
- Communicate with remote servers

DOM Selection
- Select with $('<insert what you want to select>');
- Select by CSS property i.e.
- $('.onePercent');
- $('#batman');
- Select by HTML element
- $('p');
- $('h1');
- Select by both
- $('h1 #batman');
-
Selecting HTML elements and changing their CSS/HTML
- remove()
- append()
- html()
- removeClass()/addClass()
- attr()
Events in jQuery
- Here we can check for if a user clicks some HTML element
- click()
- dblclick()
- hover()
$('#batman').click(function() {
alert("Na Na Na Batman");
});
$('#batman').bind('dblclick', function() {
alert("Na Na Na Double Batman");
});
Ajax
Communicating with remote servers
We will send a call to a server and wait for a response back
When we get a response, we can then do stuff with it
$.ajax({
data: { name: 'Don' },
url: 'https://graph.facebook.com/me/friends?',
type: 'GET',
success: function(response) {
alert("Don's friends are here");
}
});
F
Additional jQuery Resources
(Beginner)
Interactive Tutorial - http://www.codecademy.com/tracks/jquery
Interactive Tutorial - http://try.jquery.com/
(Intermediate)
Interactive Tutorial - http://jqfundamentals.com/
(Advanced)
Plugin List - http://www.unheap.com/
Plugin Repository - http://plugins.jquery.com/
JavaScript libraries
- Parallax Effects - http://wagerfield.github.io/parallax/
- JavaScript Slideshows - http://lab.hakim.se/reveal-js/#/
- Scrolling effects - http://johnpolacek.github.io/scrollorama/
- Bootstrap - http://getbootstrap.com/javascript/
- Angular.js - http://angularjs.org/
- Node.js - http://nodejs.org/
- JavaScript Game Engines - complete list
Future Workshops
10/2 - Frontend Development w/ Angular.js
10/9 - Vimeo Tech Talk on Web Design
10/16 - Backend Development w/ Node.js
Sign up for our listserv for info on future events & workshops
Give us feedback here
Javascript
By Don Yu
Javascript
- 2,121