NodeJS
Crash course
02
PAY Namibia 2018
Async programming
Working with files
Synchronous
- Program waits until the previous call is finished
- Too slow when you wait for 3rd party responses, reading from fs
and doing some operations that require high CPU usage
Synchronous
const fs = require('fs')
let content
try {
content = fs.readFileSync('content.txt', 'utf-8')
} catch (ex) {
console.log(ex)
}
console.log(content)
You don't want this context.txt to be too big
Asynchronious
- Do time-consuming task without waiting for it.
- Register appropriate jobs when task is completed
Asynchronious
Santa's little helper - Callbacks
const fs = require('fs');
function afterReadFileDoThis(err, content) {
if (err) {
return console.log(err);
}
console.log(content);
}
fs.readFile('file.md', 'utf-8', afterReadFileDoThis);
NodeJS fs lib provides methods for reading files in both manners
Asynchronious
Santa's little helper - Promises
function slowFunction(){
return new Promise((resolve,reject) =>{
setTimeout(()=>{
resolve("slow function done");
},10000);
});
}
slowFunction().then((response)=>{
console.log(response);
});
Promises are another way of handling async functions/operations
Asynchronious
Santa's little helper - async/await
function slowFunction(){
return new Promise((resolve,reject) =>{
setTimeout(()=>{
resolve("slow function done");
},10000);
});
}
async function main() {
let x = await slowFunction();
console.log(x);
}
main();
Since NodeJS 7.10.0 async/await is added
Checkpoint #1
- Create a async function and try to call it using all three methods
- Create async function that calls async function (nested)
Working with files
NodeJS can as well be used for creating some useful scripts to automate your daily work
NodeJS provides fs module that enables easy file manipulation
Read files
//async
fs.readFile('file.md', 'utf-8', (err, content) =>{
if (err) {
return console.log(err);
}
console.log(content);
});;
let content;
try {
content = fs.readFileSync('content.txt', 'utf-8');
} catch (ex) {
console.log(ex);
}
console.log(content);
Write files
//async
fs.writeFile('message.txt', 'Hello Node.js', (err) => {
if (err) throw err;
console.log('The file has been saved!');
});
//stream
const wstream = fs.createWriteStream('where_am_i.txt');
wstream.write('We are in PAY\n');
wstream.write('Windhoek, Namibia\n');
wstream.end();
//creating dir
const dir = './pay_files';
if (!fs.existsSync(dir)){
fs.mkdirSync(dir);
}
Checkpoint #2
Pass three files to your program and print the shortest one
Read the config file and create appropriate structure on FS.
//example of config file
rootDir(dir1(file1.txt,file2.txt),dir2(subdir(subdir_file.txt), file.txt),emptyDir())
() - if there are parentheses after the word, that represents a folder.
All content inside parentheses represents content within that folder.
It can be another folder or file.
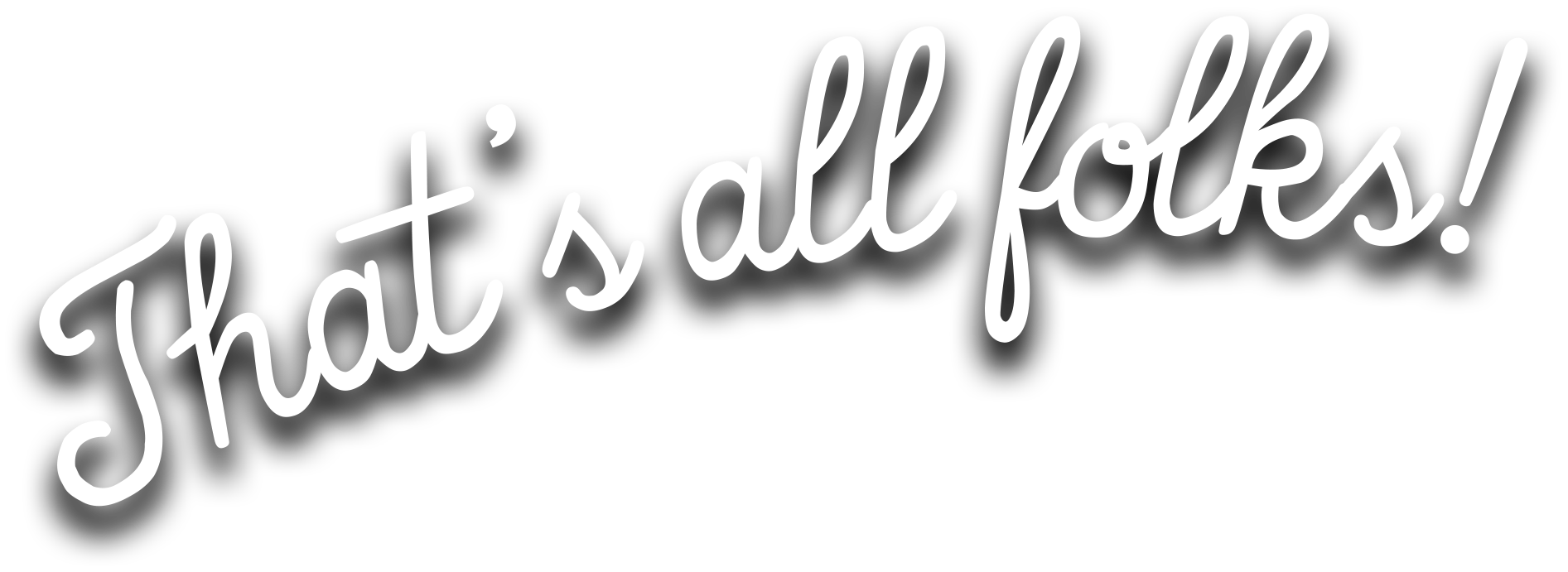
NodeJS crash course - 02
By Dušan Stanković
NodeJS crash course - 02
Async and fs module
- 1,016