...ES6

Constants
const PI = 3.141593
const MAX_WIDTH = 300

PI = 666
PI = 666 //nope
let Vs. var
var i = 123
var x = 456
for (let i = 0; i < a.length; i++) {
let x = a[i]
// ...
}
console.log(i) //123
console.log(x) //456

Property shorthands
var name = 'peter'
var age = 25
var person = {
name,
age,
size: 170
};
var name = 'peter',
age = 25;
var person = {
name: name,
age: age,
size: 170
};
Arrow functions
var sum = (a, b) => a + b
var sum = function sum(a, b) {
return a + b;
};
var numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
var odds = numbers.filter(number => number % 2);
var odds = numbers.filter(function (number) {
return number % 2;
});
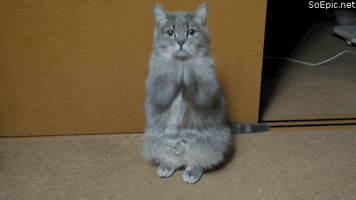
Defaults
http://es6-features.org/#DefaultParameterValues
https://github.com/lukehoban/es6features#default--rest--spread
function sum (x, y = 10, z = 20) {
return x + y + z
}
sum(3)
sum(3) // 33
sum(null, null, 50)
sum(null, null, 50) // 50
Templating
// Multiline strings
var moreLines = `In JavaScript this is
not legal.
But in ES6
it is`
// String interpolation
var name = 'Bob',
time = 'today';
var printIt = `Hello ${name}, how are you ${time}?`
Classes...
class Animal {
constructor (name, fly=false, sound) {
this.name = name
this.fly = fly
this.sound = sound
}
canFly (fly) {
if(this.fly) {
return 'goes up up in the sky'
}
return 'stays on the ground'
}
talk (sound) {
return this.sound;
}
describe () {
console.log(`${this.name}
${this.canFly()}
and shouts ${this.talk()}`)
}
}
var bird = new Animal('birdy', true, 'tchirp')
var cat = new Animal('kitty', false, 'miao')
var strangething = new Animal()
bird.describe()
cat.describe()
strangething.describe()
// "birdy
// goes up up in the sky
// and shouts
// tchirp"
// "kitty
// stays on the ground
// and shouts
// miao"
// "undefined
// stays on the ground
// and shouts
// undefined"
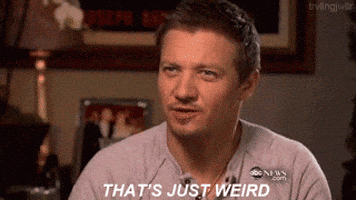
...Extend
class Cat extends Animal {
constructor() {
super()
this.name = 'cat'
this.fly = false
this.sound = 'miao'
}
}
class Bird extends Animal {
constructor() {
super()
this.name = 'bird'
this.fly = true
this.sound = 'tchirp'
}
}
var bird = new Bird()
var cat = new Cat()
bird.describe()
cat.describe()
// "birdy
// goes up up in the sky
// and shouts
// tchirp"
// "kitty
// stays on the ground
// and shouts
// miao"
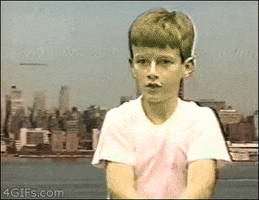
Modules
//animal.js
class Animal {
...
}
export { Animal }
//catbird.js
import { Animal } from './animal.js';
class Cat extends Animal {
...
}
// catbird.js
import { Animal as foo} from './animal.js';
class Cat extends foo {
...
}
// calc.js
import {add, subtract} from "math.js";
sum(5, 3); // 8
// math.js
function sum(a, b) { ... }
function subtract(a, b) { ... }
export { sum, subtract }
links and more
Questions?
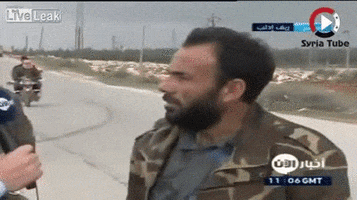
YES, we're hiring!
Babel / ES6
By hannes
Babel / ES6
Short intro to babeljs and some ES6 features.
- 2,450