Typescript Intro

Accidents
由於Javascript的動態語言特性,諸如:資料格式/型別/結構,等潛在的bug會隨著專案規模持續擴大時增加找到的難度
Dynamically Language

Edge Cases

Type System
漸進式型別系統(Gradual Typing)
型別註記 annotation
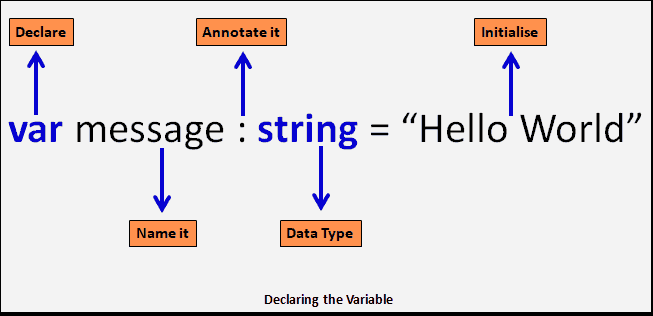
型別斷言 assertion

註記 v.s. 斷言
任何被註記到的變數、函式參數等,都必須遵照被註記過後的變數型別
被斷言過後的表達式之運算結果就是XX型別-覆蓋
型別推論 inference
Typescript也會透過這個方式提示User遺漏的edge cases
Other Advantages
Compiler
OOP Supportion

Definition File

Types
原始型別 Primitive Types
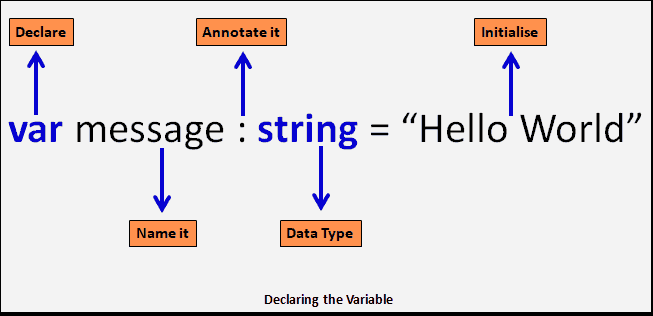
- number
- string
- boolean
- null
- undefined
- void
- symbol
物件型別 Object Types
- JSON
- Array
- Function
- Instance of Class
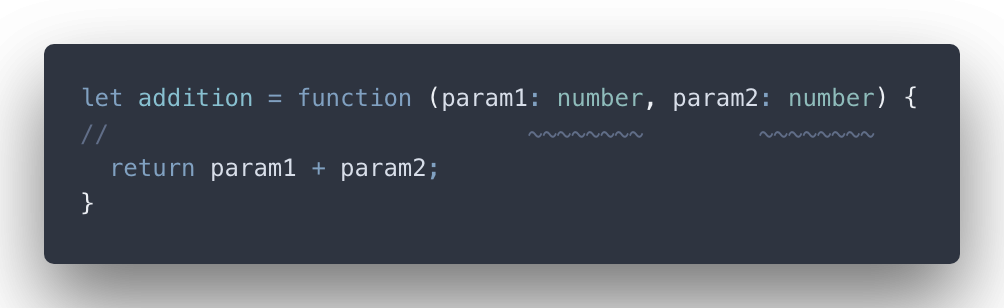

明文型別 Literal Types
// Number Literal
123;
// String Literal
'Hello World';
// Boolean Literal
true;
// Object Literal
{ name: 'Parker Chang', age: 3 }; // JSON
[1, 2, 3]; // Array

// Array Literal example
var arr: [1, 2, 3] = [1, 2, 3];
// Array Type annotation example
var arr: number[] = [1, 2, 3];
// Function Type annotation example
var greet: (someone: string) => void = [function declaration]
Typescript Provided Types
- Tuple(元祖)
- Enum(列舉)
// Tuple Example
const foo: [number, string, boolean] = [123, 'Number', 'false'];
// 陣列foo被限制只能存放三個元素且有順序限制,分別為:數字、字串、布林
// Enum
// 將相似性質的資料,用文字描述並且匯集而成的一種型別
enum Color {
Red,
Blue,
Green,
Yellow,
White
}
const baz: Color = Color.Yellow;
特殊型別 Special Types
- any
- never
- unknown
進階型別 Advanced Types
- Generic Type
- Indexable Type
- Index Type
- Composite Type (Union | Intersection)
Generics
// Generics-將型別本身「參數化」
function identity<T>(arg: T): T {
return arg;
}
let output = identity<string>("myString");
Indexable
通常用於鎖定object的key-value pair的對應型別
const dictionary: {
[key: string]: string
} = {
name: 'Parker',
description: 'The Spider man',
};
Index
Index Type Query Operator
// Indexable Type Example
const dictionary = {
name: 'Parker',
description: 'The Spider man',
};
// info的型別為:'name' | 'description'
const info: keyof dictionary;
Composite
// 聯集 Union
let numOrString: number | string;
// 交集 Intersection
let numAndString: number & string;
// 上述的case會被噴錯,因為出現了型別互斥的狀況
deck
By ian Lai
deck
- 394