Security Awareness
When theory meets practice for secure development
Presented By: Ivan Zlatanov
Prepared By: ICB's Internal Security Team
What is Secure Development Lifecycle?
# ICB
"The practice of designing, building, and maintaining software with a focus on minimizing vulnerabilities and ensuring the software is resilient against attacks"
Secure Development Lifecycle (SDL) is a multi-stage process:
- Secure Design - plan a secure software.
- Threat Modeling - analyse attack vectors.
- Secure Coding (our focus) - develop by following security practices.
- Secure Deployment - test and deploy in a secure manner.
- Security Testing - simulate attackers.
- Security Compliance - abide by industry recognised guidelines.
- Security Monitoring - monitor for patterns and behaviours of threat actors.
Why Security matters?
Growing Threats
New technology breeds new threat actors.
Threat actors are constantly advancing.
1.
2.
Misconceptions
"Security is somebody else's job."
"Nobody can find the IP."
"It is inside the local network, so it is safe."
3.
Customer trust
Breaches that lead to data exposure undermine customers' trust in the capabilities of the organisation.
# ICB
Developers are the first line of defence.
OWASP
# ICB
Open Worldwide Application Security Project
OWASP is:
- A nonprofit foundation that works to improve the security of software.
- Creator of multiple industry-recognised tools, guidelines, and standards.
- Provider of educational materials for beginners and advanced-level developers.
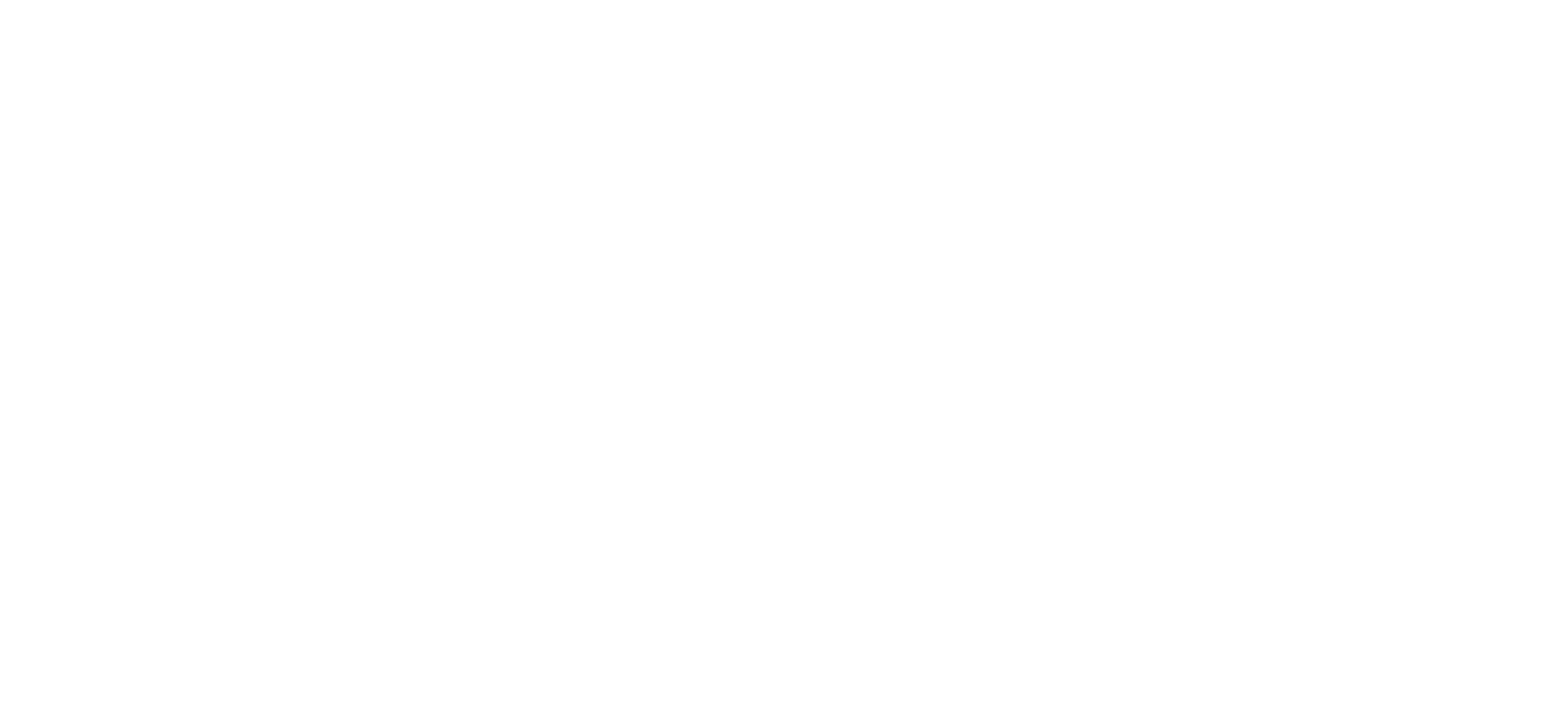
The OWASP Top 10
# ICB
Web Application Vulnerabilities
The Top 10 list is:
- A document containing the most critical security risks to web applications, compiled by OWASP.
- A widely accepted framework for understanding and mitigating common vulnerabilities.
- A baseline cornerstone for improving web application security and reducing risk exposure.
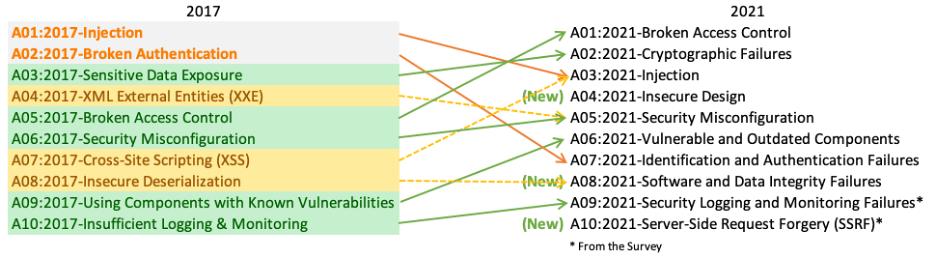
# ICB
Broken Access Control
Example: A user accessing admin-only features by modifying a URL.
Mitigation: Implement role-based access control and test for privilege escalation vulnerabilities.
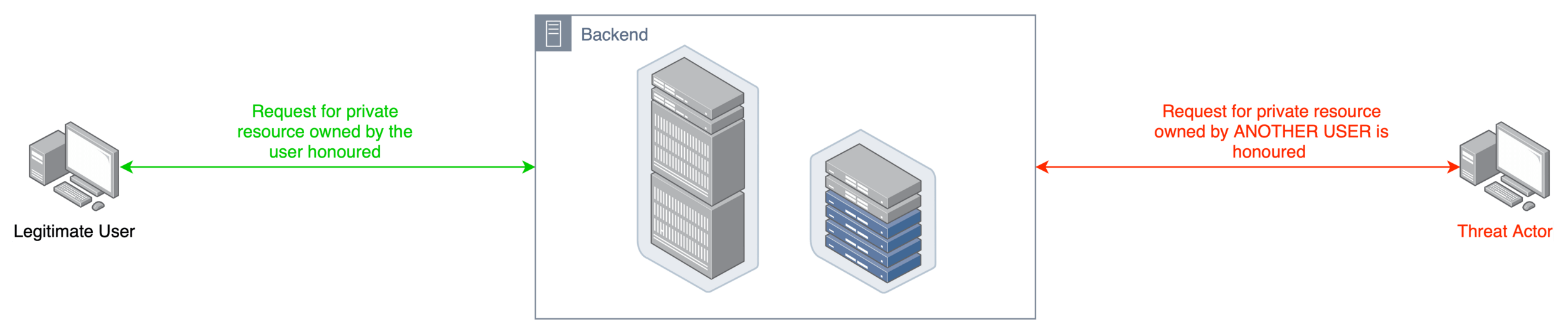
Occurs when users gain unauthorized access to resources or actions.
Commonly Observed Vulnerabilities
Commonly Observed Vulnerabilities
# ICB
Cross-Site Scripting (XSS)
Example: A comment box on a website accepts <script>alert('pwn!');</script>
as input and renders it directly to other users.
Mitigation: Always validate, sanitise, and escape user-supplied data. Implement appropriate Content Security Policy (CSP) to prevent execution.
Occurs when an attacker injects malicious scripts into web pages viewed by other users. These scripts execute in the victim’s browser.
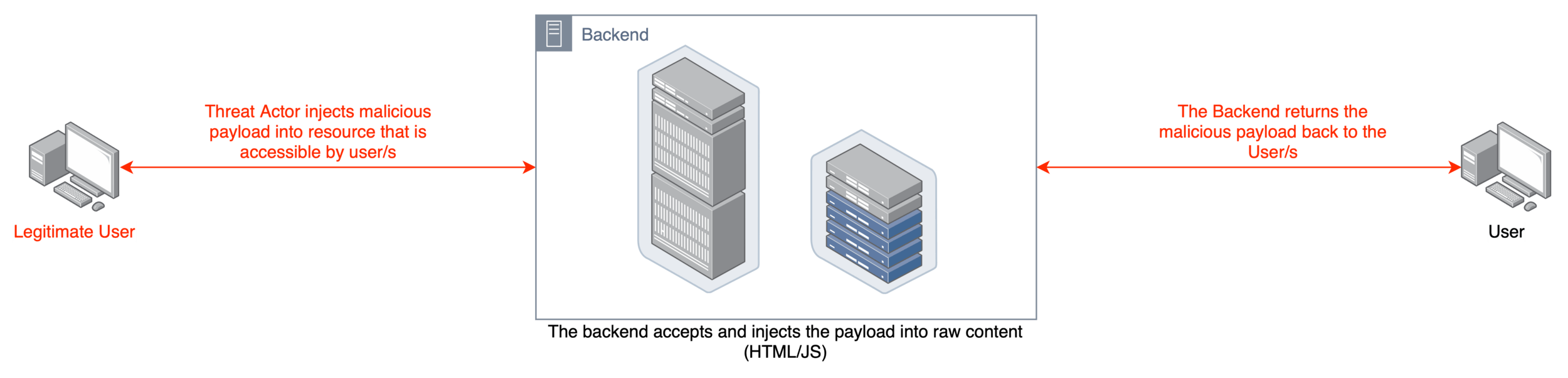
Threat Actor
Commonly Observed Vulnerabilities
# ICB
Cross-Site Request Forgery (CSRF)
Example: A victim clicks a malicious link or loads a crafted image that submits a hidden form to transfer funds using their banking session.
Mitigation: Implement anti-CSRF tokens, require re-authentication for sensitive actions. Utilise SameSite
cookies.
CSRF tricks a victim into performing actions they did not intend by exploiting their authenticated session with a website.
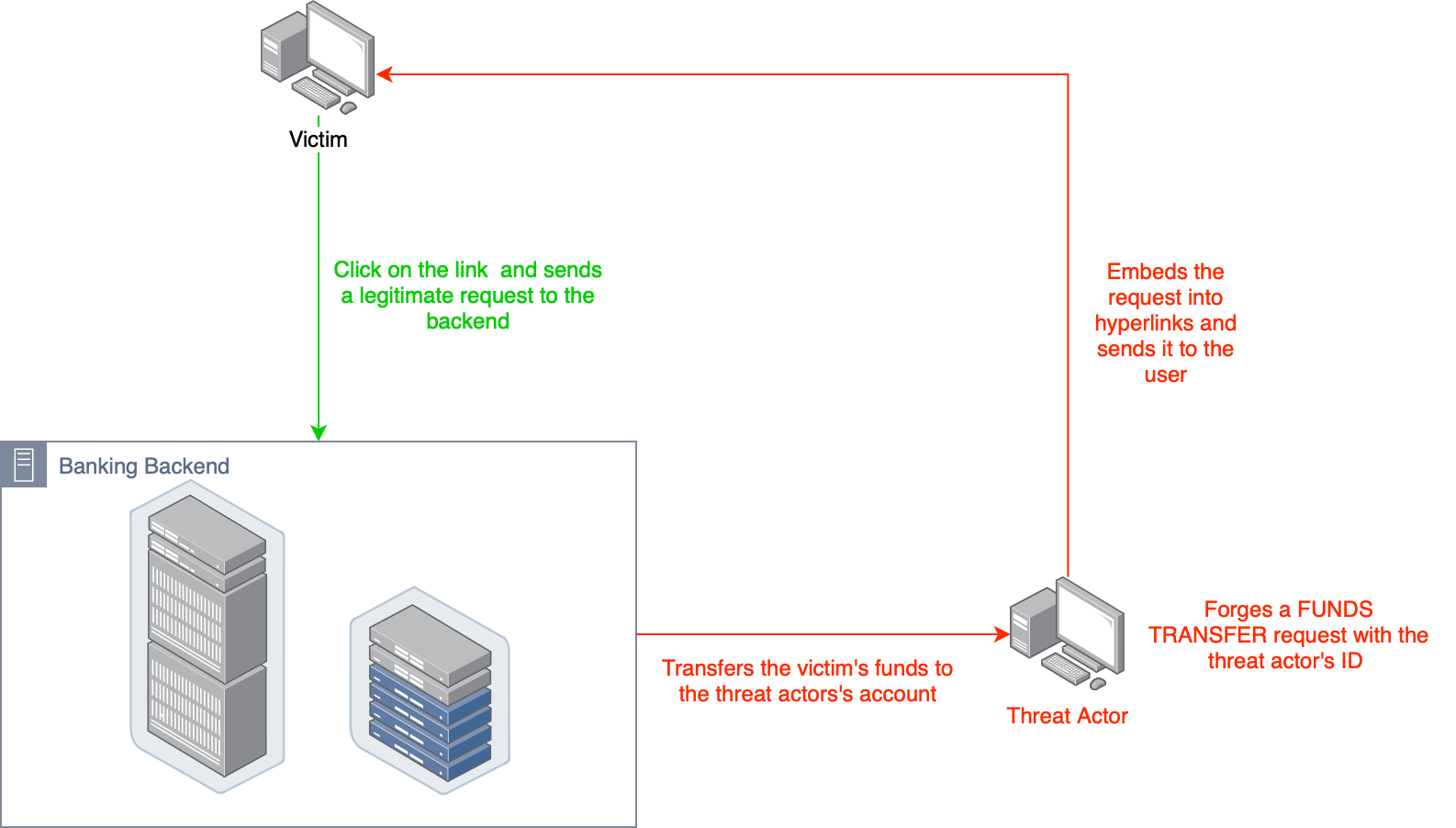
Commonly Observed Vulnerabilities
# ICB
SQL Injection (SQLi)
Example: Entering 1' OR '1'='1 in a vulnerable search field manipulates the SQL query and returns all data.
Mitigation: Use parametrised queries, employ input validation, utilise a "Least Privileged" approach for your database.
SQLi exploits vulnerabilities in a web application’s database query mechanism, allowing attackers to execute arbitrary SQL commands
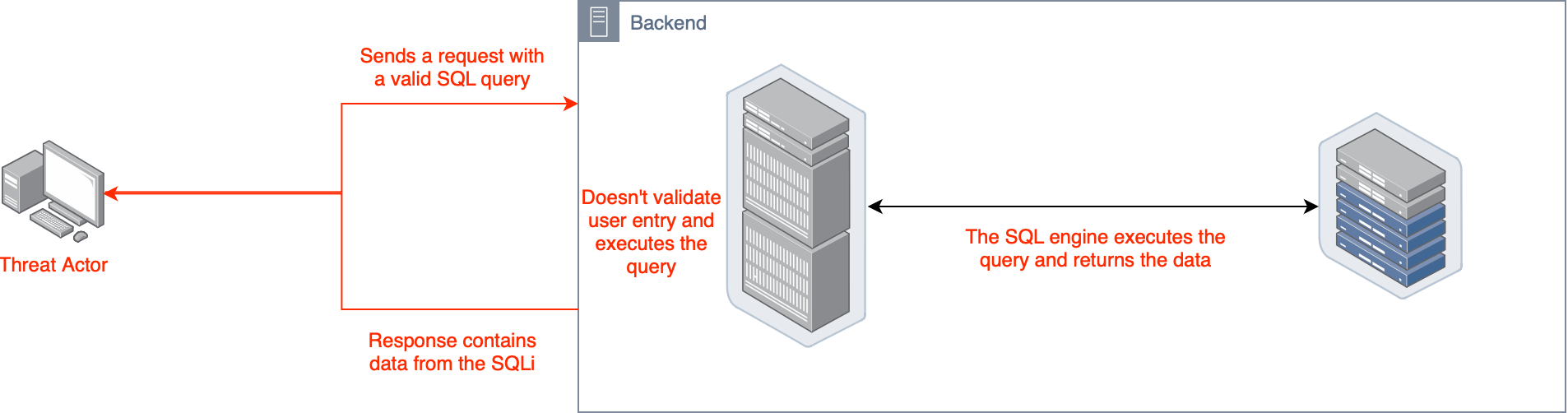
Commonly Observed Vulnerabilities
# ICB
Insecure Deserialisation
Example: An attacker sends a malicious serialised payload containing code to the server. When deserialized, the payload executes system commands, granting the attacker remote code execution (RCE) on the server.
Mitigation: Validate serialised data into predetermined objects. Validate data.
It happens when untrusted or manipulated serialized data is processed by an application, leading to code execution or other malicious actions.
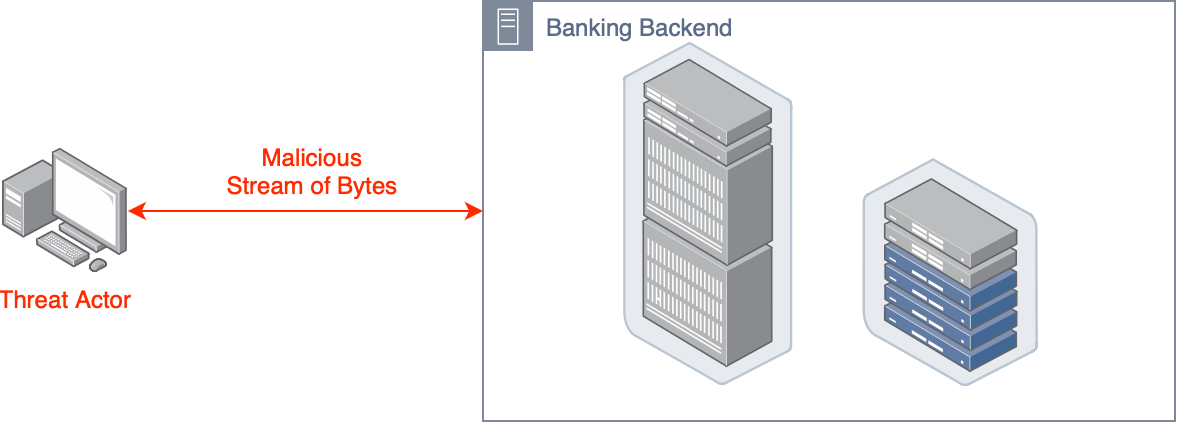
Commonly Observed Vulnerabilities
# ICB
Improper Session Management
Example: An application does not invalidate a user’s session after logout, allowing an attacker with the session token to access user data.
Mitigation: Use secure, random session tokens, enforce HTTPS, and implement proper session timeout and invalidation mechanisms.
It occurs when an application does not securely handle session data, such as session IDs or cookies, making it easier for attackers to hijack active sessions or impersonate users.
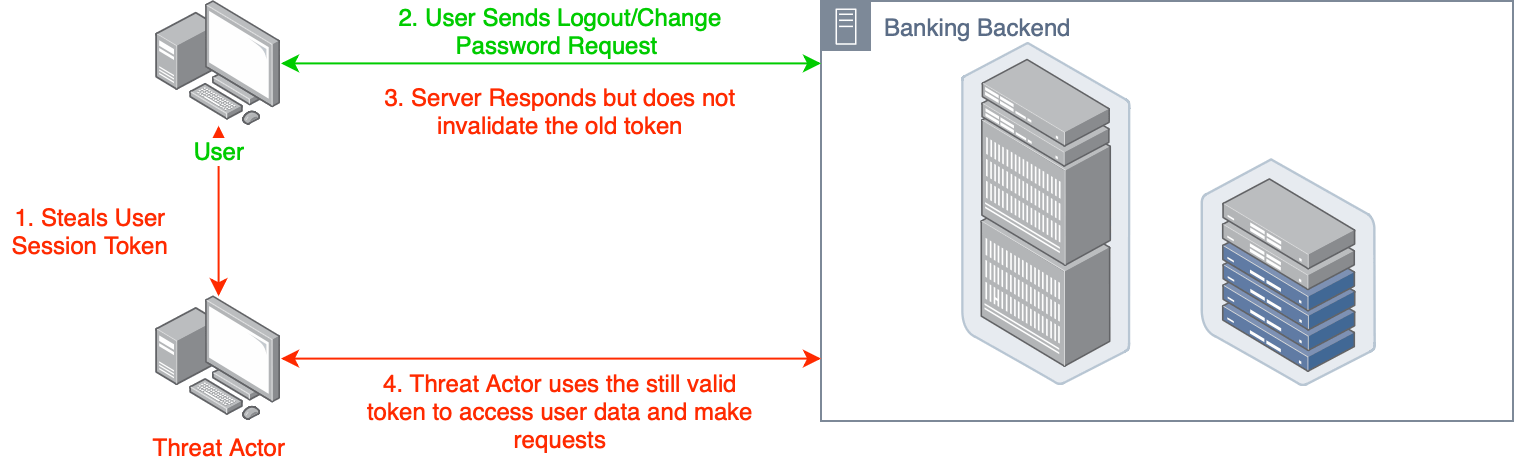
Some other commonly observed vulnerabilities
# ICB
- Remote Code Execution (RCE).
- Lack of Rate Limiting.
- Improper Key Management with Symmetric Cryptography.
- Local File Inclusion.
- Unrestricted/Unverified File Upload.
- Lack of Antivirus Scanning on Storage Blobs, Buckets, Containers, etc.
- Usage of Outdated and/or Vulnerable Dependencies.
- Server-Side Request Forgery (SSRF).
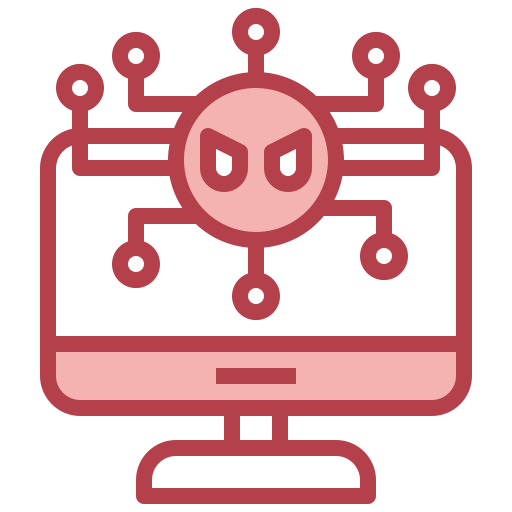
The OWASP Application Security Verification Standard (ASVS)
# ICB
What is the OWASP ASVS:
- A framework to standardise security requirements for application development and testing.
- It provides a baseline for secure software design, independent verification, and secure coding practices.
- It encourages systematic integration of security into development processes.
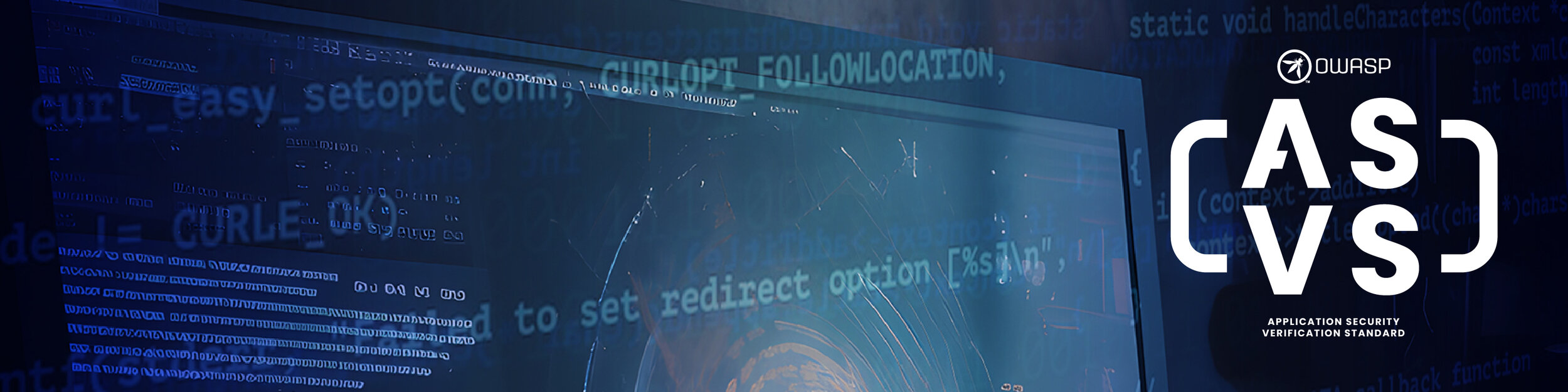
The OWASP Application Security Verification Standard (ASVS)
# ICB
Key features of the OWASP ASVS:
- 13 Security Categories: Covers areas like authentication, data protection, and configuration.
-
3 Levels of Security:
- Basic applications with no security risk..
- Applications handling sensitive data.
- Critical applications with high-security demands.
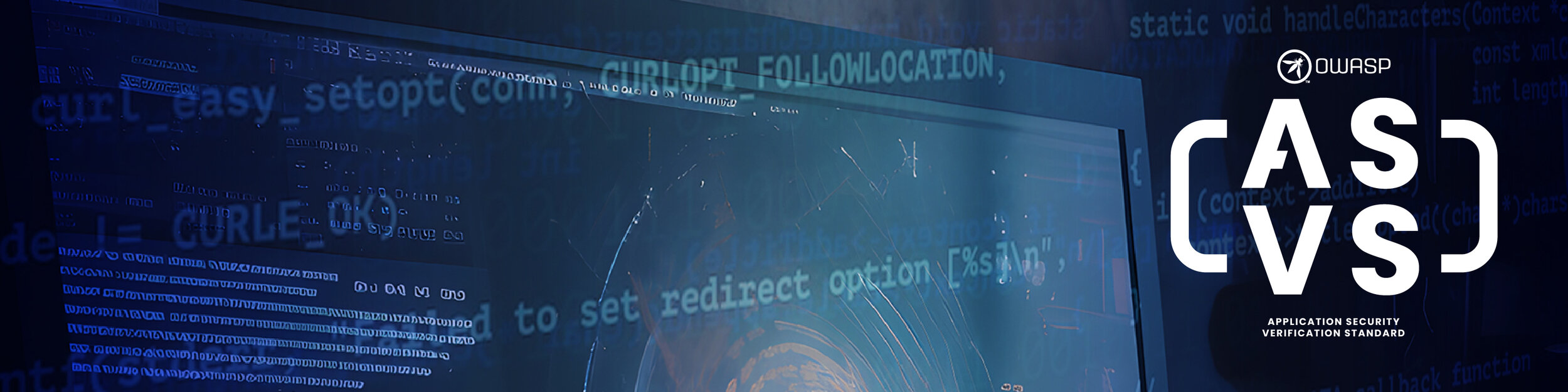
Fun Code Samples
of different vulnerabilities
Insecure Deserialisation Sample
# ICB
[HttpPost]
public IActionResult ProcessData([FromBody] byte[] serializedData)
{
var formatter = new BinaryFormatter();
using (var ms = new MemoryStream(serializedData))
{
// Deserializing user-provided data
var obj = formatter.Deserialize(ms);
ProcessObject(obj); // Perform some actions with the deserialized object
}
return Ok();
}
private void ProcessObject(object obj)
{
// Simulating processing
Console.WriteLine(obj.ToString());
}
Not So Insecure Deserialisation
# ICB
public class SafeBinder : SerializationBinder
{
public override Type BindToType(string assemblyName, string typeName)
{
var allowedTypes = new[] { typeof(MySafeObject) };
var type = Type.GetType($"{typeName}, {assemblyName}");
if (type != null && allowedTypes.Contains(type))
{
return type;
}
throw new SerializationException("Unauthorized type detected.");
}
}
[HttpPost]
public IActionResult ProcessData([FromBody] byte[] serializedData)
{
var formatter = new BinaryFormatter
{
Binder = new SafeBinder()
};
using (var ms = new MemoryStream(serializedData))
{
var obj = formatter.Deserialize(ms);
ProcessObject(obj);
}
return Ok();
}
Local File Inclusion (LFI) Code Sample
# ICB
[HttpGet]
public IActionResult GetFile(string filename)
{
string path = Path.Combine("C:\\Files", filename);
if (System.IO.File.Exists(path))
{
return PhysicalFile(path, "application/octet-stream");
}
return NotFound("File not found");
}
// Similar code can introduce vulnerability to blobs and other storage units.
"Patched" LFI Code Sample
# ICB
[HttpGet]
public IActionResult GetFile(string filename)
{
// Ensure filename contains no directory traversal
string sanitizedFilename = Path.GetFileName(filename);
string path = Path.Combine("C:\\Files", sanitizedFilename);
if (System.IO.File.Exists(path))
{
return PhysicalFile(path, "application/octet-stream");
}
return NotFound("File not found");
}
Remote Code Execution Code Sample
# ICB
[HttpPost]
public IActionResult Execute(string command)
{
var process = new Process
{
StartInfo = new ProcessStartInfo
{
FileName = "cmd.exe",
Arguments = "/C " + command,
RedirectStandardOutput = true,
UseShellExecute = false,
CreateNoWindow = true
}
};
process.Start();
string result = process.StandardOutput.ReadToEnd();
return Ok(result);
}
"Patched" RCE Code Sample
# ICB
[HttpPost]
public IActionResult ExecuteSafe(string operation)
{
if (operation == "list")
{
string[] files = Directory.GetFiles("C:\\Files");
return Ok(files);
}
return BadRequest("Invalid operation");
}
SQL Injection Code Sample
# ICB
[HttpGet]
public IActionResult GetUser(string username)
{
string query = $"SELECT * FROM Users WHERE Username = '{username}'";
using (var connection = new SqlConnection("your_connection_string"))
{
var command = new SqlCommand(query, connection);
connection.Open();
var reader = command.ExecuteReader();
// Process the results
}
return Ok();
}
"Patched" SQL Injection Code Sample
# ICB
[HttpGet]
public IActionResult GetUser(string username)
{
using (var connection = new SqlConnection("your_connection_string"))
{
var query = "SELECT * FROM Users WHERE Username = @Username";
var command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@Username", username);
connection.Open();
var reader = command.ExecuteReader();
// Process the results
}
return Ok();
}
Cross-Site Scripting Code Sample
# ICB
[HttpGet]
public IActionResult Greet(string name)
{
return Content($"<h1>Welcome, {name}</h1>", "text/html");
}
"Patched" XSS Code Sample
# ICB
[HttpGet]
public IActionResult Greet(string name)
{
string sanitized = System.Net.WebUtility.HtmlEncode(name);
return Content($"<h1>Welcome, {sanitized}</h1>", "text/html");
}
Weak JSON Web Token Code Sample
# ICB
var token = new JwtSecurityToken(
issuer: "myapp",
audience: "myaudience",
claims: claims,
expires: DateTime.UtcNow.AddDays(1),
signingCredentials: new SigningCredentials(
new SymmetricSecurityKey(Encoding.UTF8.GetBytes("random-weak-key")),
SecurityAlgorithms.HmacSha256
)
);
Not so weak JWT Code Sample
# ICB
var token = new JwtSecurityToken(
issuer: "myapp",
audience: "myaudience",
claims: claims,
expires: DateTime.UtcNow.AddDays(1),
signingCredentials: new SigningCredentials(new SymmetricSecurityKey(GenerateSecureKey()), SecurityAlgorithms.HmacSha256)
);
private byte[] GenerateSecureKey()
{
using (var rng = new RNGCryptoServiceProvider())
{
var key = new byte[32];
rng.GetBytes(key);
return key;
}
}
Important Takeaway: Aways use secure random crypto providers.
Improper WebSocket Message Handling
# ICB
webSocket.OnMessage += async (sender, e) =>
{
var message = Encoding.UTF8.GetString(e.RawData); // No validation
await ProcessMessage(message); // Allows potentially harmful input
};
Good WebSocket Message Handling
# ICB
webSocket.OnMessage += async (sender, e) =>
{
var message = Encoding.UTF8.GetString(e.RawData);
if (!IsValidJson(message))
{
await webSocket.CloseAsync();
return;
}
await ProcessMessage(message);
};
private bool IsValidJson(string input)
{
try
{
JToken.Parse(input); // Validate input as JSON
return true;
}
catch
{
return false;
}
}
Enough Examples
For Now...
How Do We Write Secure Code?
Or Code That Can Be Considered Secure?
Secure Coding Practices
# ICB
- Design the architecture with a security-focused mindset.
- Write code with security in mind from the start.
- Never hardcode sensitive information:
- Passwords, Keys, and etc. must be kept as ENV variables.
- Don't keep sensitive data in configuration files.
- Never trust the client:
- Aways sanitise, escape and validate data supplied to your backend.
- Implement proper error and exception handling.
- Always use parameterised database queries and proper ORMs.
- Never implement your own cryptography (unless you have PhD in the field).
- Always use tried and tested libraries and algorithms.
- Always use a cryptographically secure random generators for sensitive data.
- Generate cryptographically secure salts, keys, and etc.
- When in doubt, check the OWASP Secure Coding Practices-Quick Reference Guide.
Managing 3rd Party Risks
# ICB
- Evaluate the security posture of third-party libraries.
- Track versions and update when a security patch is available.
- Scan 3rd party dependencies for vulnerabilities.
- Use tools like Software Composition Analysis (SCA) for monitoring.
- Employ a secure supply chain framework (e.g., SBOM - Software Bill of Materials).
- Keep an inventory of 3rd party dependencies that your software uses.
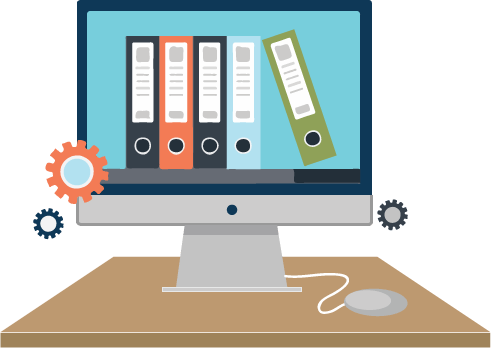
Perform Application Security Testing
# ICB
- The process of statically or dynamically testing a software application with the purpose to uncover security weaknesses.
- It must be performed on a regular basis.
- Can be separated in three major types:
- Static Application Security Testing (SAST).
- Dynamic Application Security Testing (DAST).
- Interactive Application Security Testing (IAST).
- Works best when combined with security controls in the CI/CD pipelines.
- Yields high success rates when combined with Penetration Testing or Security Assessments.
A Basic Security Pipeline
# ICB

Sensitive Data: Protection and Storage
# ICB
- Encrypt all data in transit (eg. TLS or E2EE) and at rest (eg. AES).
- Implement strong authentication mechanisms (e.g., MFA).
- Always validate that OTPs that are used expire as per specification.
- Use secure APIs to access sensitive information.
- Regularly audit and test access controls.
- Utilise encrypted backups.
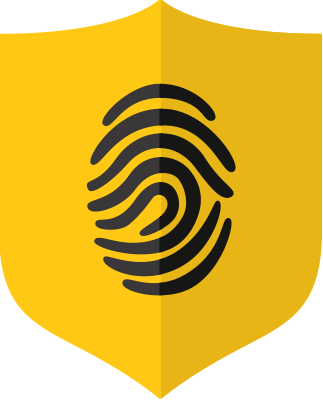
Exploring Useful Tools
# ICB
- Static Analysis Tools:
- SonarQube: Analyzes code quality and identifies vulnerabilities.
- Checkmarx: SAST tool for identifying security flaws in code.
- Dynamic Analysis Tools:
- Burp Suite: Comprehensive platform for web vulnerability testing.
- OWASP ZAP: Open-source tool for finding web application vulnerabilities.
- Acunetix: Automated web application security scanner for detecting vulnerabilities.
- Dependency Scanning Tools:
- Snyk: Helps identify vulnerabilities in third-party libraries.
- Dependabot: Automates dependency updates.
- Sonatype Nexus Lifecycle: Tracks and secures dependencies in the software lifecycle.
Some Practice
Let's see how attacker exploit vulnerabilities
Enter:
root-me.org
Some Useful Resources
# ICB
"Security is not a product, but a process." — Bruce Schneier
- https://owasp.org/ - documents, guidelines, and standards on secure development.
- https://www.cybrary.it/ - courses with focus on cybersecurity.
- "The Web Application Hacker's Handbook" by Dafydd Stuttard and Marcus Pinto.
- "Building Secure Software" by John Viega and Gary McGraw.
- "Threat Modeling: Designing for Security" by Adam Shostack.
And some interesting reads:
Useful links:
"Building secure software is not about eliminating risks but managing them effectively." — Gary McGraw
Questions?
Thank you
Code
By Ivan Zlatanov
Code
- 16