Functional Reactive Programming
Johnny Austin
@recursivefunk
CodePen DC
1/15/15
About Me

Director of Technoloy

Contributor

Reluctant Proponent

Fanboy! (oh-em-gee)

Agenda
- Functional Programming
- Reactive Programming
- Functional Reactive
- Demo!
Functional Programming

Functional Programming
- No clear definition
- Contrast with OOP
- OOP composes objects / methods
- OOP owns it's data and operations
- FP just uses functions to perform operations
- Central data structure are functions
- Function composition
- Loosely coupled from data
- Generic operations
- Data in, data out
- No side effects
- Generic operations
Functional Programming cont.
var bar = [ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 ];
var foo = bar
.filter(function(item) {
return item > 5;
})
.map(function(item) {
return item * 2;
});
log( foo ); // [ 12, 14, 16, 18, 20 ]
log( bar ); // [ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 ]
Reactive Programming
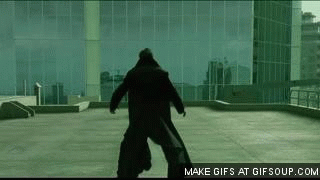
Reactive Programming
- Programming around data streams
- Streams
- Data over time
- Old Concept
- Events
- document.addEventListener
- $('#foo').on('click')
- Node.js (io.js? hehe) Streams
- Pub/Sub
- Events
- Signals
- More efficient than polling
- Easier to understand
Reactive Programming cont.
// the dom ---------------------------------
$('#text-input').on('click', function(e){
var entered = e.target.value;
$('#output').html( entered );
});
// the server ------------------------------
fs
.createReadStream( './some/file.txt' )
.on('data', function(){
// do something with the data
})
.on('error', function(){
// :(
})
.pipe( anotherStream );
// socket.io --------------------------------
io.sockets.on('connection', function (socket) {
var payload = {
msg: 'all your events are belong to us'
};
socket.broadcast.emit( 'readMe', payload );
});
Reactive Programming cont.
- Bigger than code
- System architecture
- Non-monolithic
- Distributed
- System architecture
Functional
- Non OO
- Single concern
- No side effects
- Immutability
Reactive
- Streams of data over time
- Events/PubSub etc
- Signals (error, complete)
Review (so far)
Functional Reactive Programming
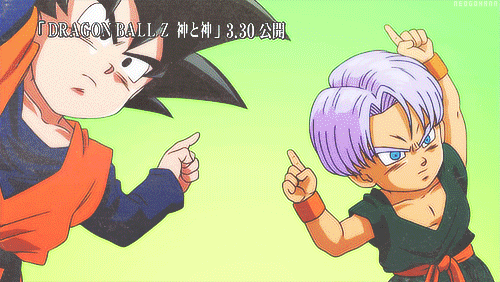
DEMO
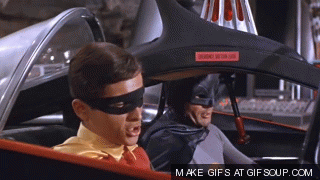
github.com/jray/tiny-frp
Your Turn
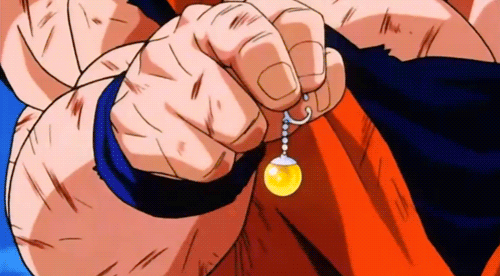
CodePen - FRP
By Johnny Ray Austin
CodePen - FRP
FRP talk for CodePen DC meetup
- 3,351