DOM Manipulation
Manipulate your HTML/CSS using Javascript
The M
1
What's DOM
2
DOM Methods and Props
3
DOM Strucutre
5
Steps to creating an HTML element
+ Deleting
4
Steps to Modify HTML/CSS
6
Event Listeners
in DOM
What is DOM?
Dumb Old Monkey?
Don't Offer Me more work?
Dogs on Mars?
Daulphin Olympics Marathon?
Dangerous Overpriced Meals?
Document Object Model
When a web page is loaded, the browser creates a Document Object Model of the page.
DOM is constructed as a tree of Objects
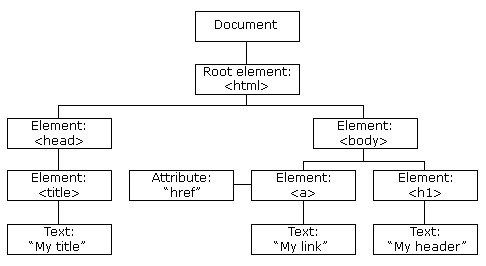
Document Object Model
Open a random webpage and inspect it!
Why are we learning about this?
Why are we learning about this?
Simply, because the DOM can be modified and played with using javascript!
DOM Methods
The methods are actions you perform on the HTML elements
DOM Properties
The values (of the HTML elements) that can be changed (using methods)
<html>
<body>
<p id="demo"></p>
<script>
document.getElementById("demo").innerHTML = "Hello World!";
</script>
</body>
</html>
Example:
DOM Method
Property
Note: DOM elements are manipulated as objects
Parent object: document
DOM Structure: Node, Child, Parent, Sibling
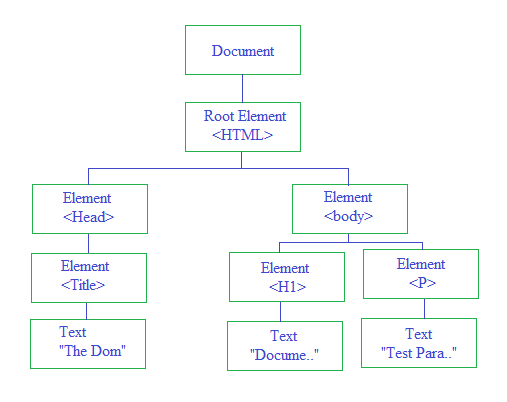
Steps to modify an HTML element:
- Find the element you'd like to edit
- Access the property you'd like to edit
- Write the new value you'd like to set it to
//Finding HTML elements by id
const element = document.getElementById("full_stack");
//Finding HTML elements by tag name
const element = document.getElementsByTagName("p");
//Finding HTML elements by class name
const x = document.getElementsByClassName("green");
//Finding HTML elements by CSS selectors
const x = document.querySelectorAll("p.intro");
Find the HTML element
//innerHTML
document.getElementById('index1').innerHTML = 'You can write whatever here, even <br> HTML tags';
//innerText
document.getElementById('index1').innerText = 'You can also write text here, but no html tags.';
//value
const input_value = document.getElementById('input').value;
//style
document.getElementTagName('div').style.color = 'blue';
//className
document.getElementTagName('div').className = 'class1 class2';
Accessing properties
// GENERALY WE USE:
paragraph.setAttribute('class', 'class1')
Steps to creating an HTML element:
- Find the element you'd like to add to
- Create the element you'd like to add
- Append the element created to the base one
// We're trying to add a paragraph inside a div with the id: index
const div = document.findElementById('index');
//or
const div = document.querySelector('div');
Select the element you'd like to add to
//Create the paragraph element
const paragraph = document.createElement('p');
paragraph.textContent = 'fullstack js training';
Create the new element
//Create the paragraph element
div.appendChild(paragraph);
Appending the new element
//Delete child
div.removeChild(paragraph);
////Delete an element specifically
const paragraph = document.findElementTagName('p');
paragraph.remove();
Deleting an Element
- Delete a child of an element
- Delete the element you're on
// element.addEventListener(event, function, useCapture);
document.getElementById("myBtn").addEventListener("click", displayDate);
document.getElementById("myBtn").addEventListener("mouseover", myFunction);
document.getElementById("myBtn").addEventListener("click", mySecondFunction);
document.getElementById("myBtn").addEventListener("mouseout", myThirdFunction);
Event Listeners
Event Listener: Yestenak to make the action, then executes a certain function
// To end the event listener
element.removeEventListener("mousemove", myFunction);
Done for today!
DM or @Tag if you need help!
DOM Manipulations
By Maria Oussadi
DOM Manipulations
- 64