{MongoDB}
Mongo Da Best
The M
2
1
Manipulating DB Data
2
Middlewares and instance methods
Manipulating the data
# FULLSTACK JS
What we mean here, is that we're gonna learn to use code to: find, update.. data in our DB
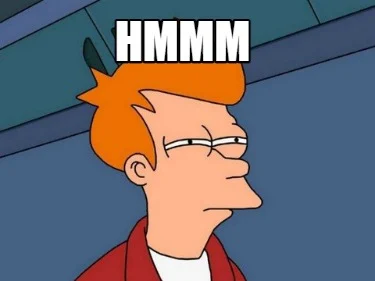
# FULLSTACK JS
async function findData(first_name){
try{
const users = await userModel.find({first_name});
return users;
}catch(e){
return [];
}
}
Find Data
Update Data
# FULLSTACK JS
async function updateUserByID(_id, newData){
try{
const users = await userModel.updateOne({_id}, newData);
console.log("user has been updated")
return users;
}catch(e){
console.error(e);
}
}
Deleting Data
# FULLSTACK JS
async function updateUserByID(_id){
try{
const users = await userModel.deleteOne({_id});
console.log("user has been deleted")
return users;
}catch(e){
console.error(e);
}
}
Middlewares and Methods
# FULLSTACK JS
# FULLSTACK JS
Middlewares
What are they?
Middleware (also called pre and post hooks) are functions which are passed control during execution of asynchronous functions.
# FULLSTACK JS
userSchema.pre('save', function(next) {
// do stuff
next();
});
Middlewares
userSchema.post('save', function(next) {
// do stuff
next();
});
Before saving
After saving
# FULLSTACK JS
Instance methods
What are they?
The instances of models have many methods that are already built in (create, update.. etc) but we can have our customized ones
# FULLSTACK JS
Instance methods
//Define a schema
//
userSchema.methods.findSimilarAge = function (cb){
return mongoose.model('user').find({age : this.age}, cb)
}
//now all the users instaces have a findSimilarAge method available in them
//
//to try it:
//
const User = mongoose.model('User', userSchema)
const person = new User({age: 20}) //we instanciAgeate User
person.findSimilarAge((err, persons)=>{
console.log(persons);
})
Thank you for attending
<3
MongoDB Training 2
By Maria Oussadi
MongoDB Training 2
- 31