Javascript Fundamentals 2
Script your way through the web.
Maria Oussadi (The M)
Who's The M?
Ex Comm dept leader
Community manager by day, developer by night
Maria Oussadi
1
Quick revision
2
Get to Loop your code
3
Functions
5
Intro to Arrays
4
Arrow Functions
6
Callback functions
Revision
Let's go through some basics again
Loops
Loop through your code
// Print on console numbers from 1 to 10
console.log(1)
console.log(2)
console.log(3)
console.log(4)
console.log(5)
console.log(6)
console.log(7)
console.log(8)
console.log(9)
console.log(10)
What's wrong with this code?
// Print on console numbers from 1 to 10
for(let i=1;i<=10; i++ ){
console.log(i);
}
For Loops
// Example 2
int i=5;
for(let i=1 ; i<=10 ; i++ ){
console.log(i);
}
console.log(i);
For Loops
For Loops
//Example 3
for(let i=1; i<=5; i++){
console.log("The value of k is:"+ i + "\n");
let k = 6;
console.log("The value of i is:" + k);
k++;
}
var i = 5;
i=5;
//declared globally in the program even inside a block
Global Variables
const i = 5;
let i=5;
i=5;
//declared inside a loop/condition/function -> blocks
Local Variables
"While" Loops
//Example
let i = 1
while(i<=3){
console.log(i);
i++;
}
"Do .. While" Loops
//Example
let result = '';
let i = 0;
do {
i = i + 1;
result = result + i;
} while (i < 5);
console.log(result);
Functions
Code shortcuts
// Function to compute the sum of a and b
function myFunction(a, b) {
let sum = a + b;
console.log(sum);
}
Function Syntax
Function declaration syntax
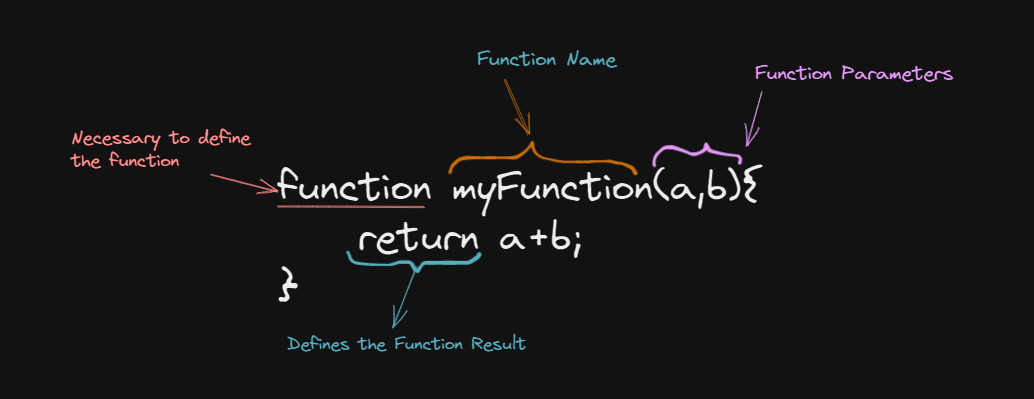
// After declaring the function you gotta call it
// Example:
myFunction(1,3); //output will be: 4
Function calling
// Function that does?
//
function fact(n){
let sum = 0;
for (let i = 1; i<=n ; i++){
sum = sum * i;
}
return sum;
}
Function examples
// Function that does?
//
function myFunction(n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * myFunction(n - 1);
}
}
Function examples
// Arrow function syntax makes the function shorter
let myFunction = (a, b) => a * b;
Arrow (lambda) functions
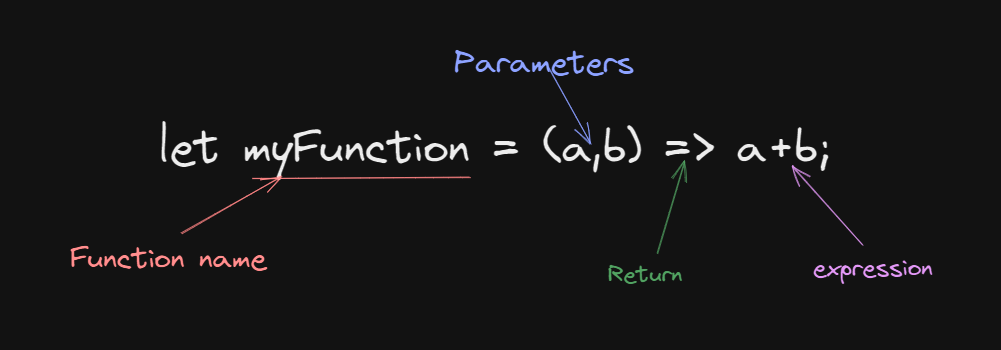
Write a function where you introduce yourself and give your hobbies:
function must include as parameters:
- full_name
- age
- hobby1
- hobby2
Don't forget to respect the camelCase for the function naming
Callback Functions
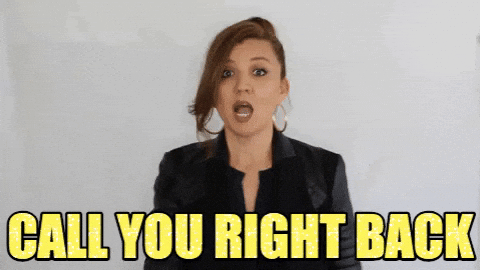
function Display(arr, callback){
for (let i = 0; i < arr.length; i++){
var elm = callback(arr[i]);
console.log(elm);
}
}
//Defining the double function
function double(n){
return n*2;
}
//calling the display function
var result = Display([1, 4, 0, 3], double);
Callback functions
//A usecase:
//For example, the data coming from a server
//because it takes time for data to arrive.
//setTimeout(callback, duration)
setTimeout(() => {
console.log("Delayed for 1 second.");
}, "1000");
Callback functions
Callbacks are not the same as nested functions!
function Display(arr, callback){
for (let i = 0; i < arr.length; i++){
var elm = callback(arr[i]);
console.log(elm);
}
}
//Defining the double function
function double(n){
return n*2;
}
//calling the display function
var result = Display([1, 4, 0, 3], double);
function greeting() {
// [1] Some code here
console.log("Greeting start");
sayHi();
// [2] Some code here
console.log("Greeting end");
}
//declare sayHi function:
function sayHi() {
return "Hi!";
}
// Invoke the `greeting` function:
greeting();
Callback:
Nested function:
Arrays
[m, i, c, r, o..]
//Arrays declaration
let arr = []; //Empty array
let arr1 = [1,2,3]; //array that contains values of: 1, 2, 3
Arrays
//length method:
const clothing = ['shoes', 'shirts', 'socks', 'sweaters'];
console.log(clothing.length);
Methods on arrays
//IndexOf method:
const clothing = ['shoes', 'shirts', 'socks', 'sweaters'];
console.log(clothing.indexOf('shoes'));
If you need help OR would like to understand something feel free to
DM her!
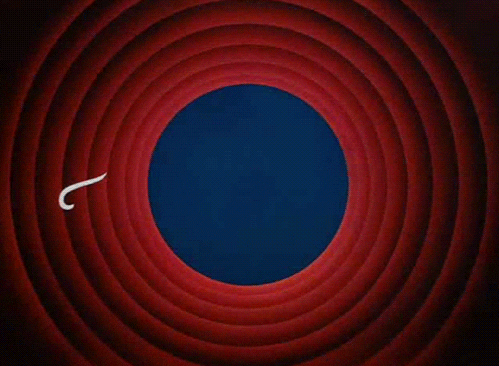
Javascript Fundamentals 2
By Maria Oussadi
Javascript Fundamentals 2
- 69