declarative and imperative Programming
An exclamatory introduction with interrogative explanations
About me
- Name: Mike Frazier
- Occupation: Software Engineer at Quizlet
- Past life: High school science teacher
- Hobbies: (Skate/Snow)boarding, reading, music stuff
- Zodiac: Aries
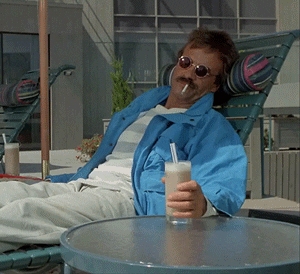
- Twitter: @AFrazGuy
- DenverDevs: @frazier
- Outdated blog: www.mikedoescoding.com
Question
How would you describe the process of making a peanut butter and jelly sandwich to someone who has never seen a sandwich before?
How would you describe to get to your house?
- If the person has an internet enabled phone
- If the person has an atlas in her car
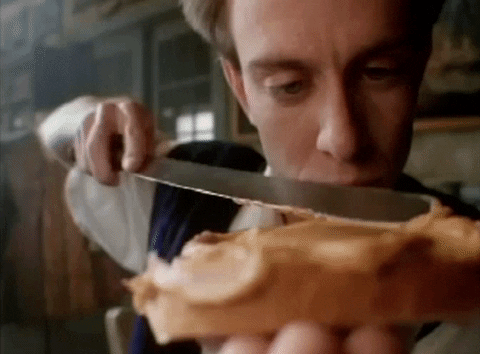
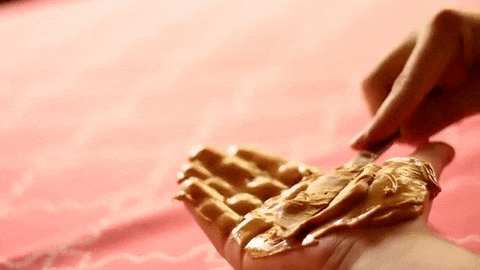
Imperative
Imperative programming is writing steps that explain how to complete a task. For example, this means describing in excruciating detail how to open a bag of bread.
Declarative
Declarative programming is writing a program that explains more about what to do. For example, this means expressing what should happen to each piece of bread.
Let's log everything in this array
const myArr = [ 1, 2, 3, 4, 5, 6 ];
const log = (value) => console.log(value);
// Imperative Code
for (let i = 0; i < myArr.length; i++) {
log(myArr[i]);
}
// Declarative Code
myArr.forEach(log);
Let's Double the values in this array
const numArr = [ 1, 2, 3, 4, 5, 6 ];
const double = (value) => value * 2;
// Imperative Code
let doubles = [];
for (let i = 0; i < numArr.length; i++) {
doubles.push(double(numArr[i]));
}
console.log(doubles); // [ 2, 4, 6, 8, 10, 12 ]
// Declarative Code
let doubles2 = numArr.map(double);
console.log(doubles2); // [ 2, 4, 6, 8, 10, 12 ]
Let's only keep key-value pairs of strings
const myObj = {
a: 'earth',
b: 'air',
c: 'fire',
d: 25,
e: 'water',
f: 'heart',
g: ['I AM CAPTAIN PLANET']
};
const isString = (value) =>
typeof value === 'string';
// Imperative Code
let onlyStrings = {};
for (let key in myObj) {
if (isString(myObj[key])) {
onlyStrings[key] = myObj[key];
}
}
// Declarative Code
let onlyStrings2 = Object.entries(myObj)
.reduce((memo, kVTuple) => {
if (isString(kVTuple[1])) {
memo[kVTuple[0]] = kVTuple[1];
}
return memo;
}, {});
Okay but what's a real example?
// Imperative DOM Manipulation
document.querySelector('#ok-button')
.addEventListener('on-click', handleClickFn);
document.querySelector('#ok-button')
.classList.toggle('is-valid', state.isValid);
// Declarative DOM Manipulation
import React from 'react';
export function OkButton({
handleClickFn,
isValid
}) {
return (
<div id="ok-button">
<button
onClick={handleClickFn}
className={isValid ? 'is-valid' : null}>
OK!
</button>
</div>
);
}
What's the BFD? Why should i care?
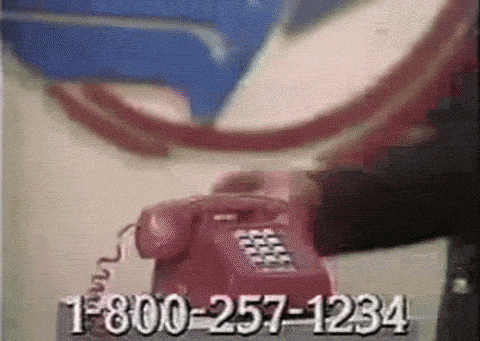
Trade offs and considerations
- Readability
- Cognitive overhead
- Personal (professional) choice
- Run-time speed
Thanks
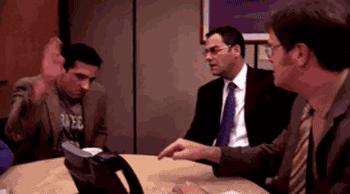
deck
By Mike Frazier
deck
- 63