What To Do When You Don’t Care
(About Finding Items in Arrays)
[What Do You Care About?]
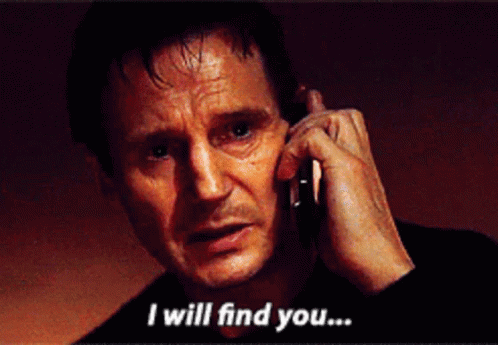
About Mike Frazier
Software Engineer @ Quizlet
Hack Reactor Remote
Former educator
Recent skateboarder
Music stuff too and junk
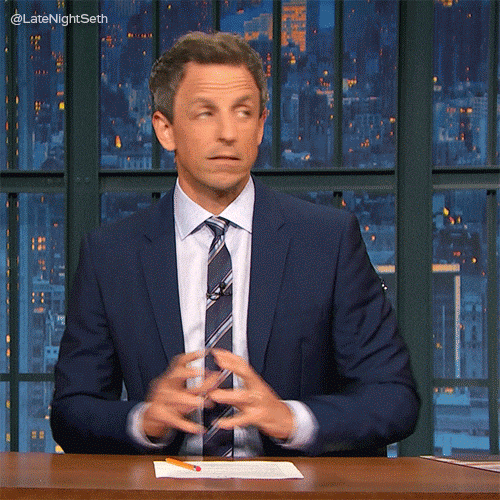
some
findIndex
lastIndexOf
includes
indexOf
find
ES5
ES6+
Note About Examples
Examples for object arrays are parsing for specific key-value pairs
To compare object to object, a workaround is to "stringify" the objects and compare strings
Repl available with some examples: https://bit.ly/2Lj2ZPV
I just care about getting back the index of the item!
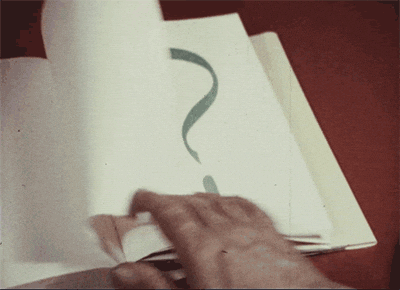
Array.prototype.indexOf
Array.prototype.lastIndexOf
Returns the index of the found item
Returns -1 if no item is found
Considerations
- Works really well for primitive values
- Uses a strict equality check (===)
- Won't find NaN
Array.prototype.indexOf
Array.prototype.lastIndexOf
let arr = [ 1, 2, 3, 4, 5, 3, 6 ];
let foundIdx = arr.indexOf(3); // 2
let lastFoundIdx = arr.lastIndexOf(3); // 5
let idxNotFound = arr.indexOf(7); // -1
let arr2 = [ { id: 1 }, { id: 2 }, { id: 3 } ];
let willNotWork = arr2.indexOf({ id: 2 }); // -1
Array.prototype.findIndex
Return the index of the found item that passes the comparator function
Returns -1 if no item is found
Considerations
- Returns the first item that passes the comparator
- Visits every index in the array -- even those without an assigned value
- Have to explicitly check for NaN
Array.prototype.findIndex
let arr = [
{ id: 1, color: 'red' },
{ id: 2, color: 'blue' },
{ id: 3, color: 'green' },
];
let foundIdx = arr.findIndex(item => item.color === 'blue'); // 1
let notFoundIdx = arr.findIndex(item => item.id === 4); // -1
let arr2 = [ 'hearts', 'stars', 'horseshoes', 'clovers' ];
let starsIdx = arr2.findIndex(item => item === 'stars'); // 1
I just care about getting back the item!

Array.prototype.find
Returns the found item that passes the comparator function
Returns undefined if no item is found
Considerations
- Returns the first item that passes the comparator
- Visits every index in the array -- even those without an assigned value
- Have to explicitly check for NaN
Array.prototype.find
let arr = [
{ id: 1, color: 'red' },
{ id: 2, color: 'blue' },
{ id: 3, color: 'green' },
];
let foundItem = arr.find(item => item.color === 'red');
// { id: 1, color: red }
let notFoundItem = arr.find(item => item.id === 4); // undefined
let arr2 = [ 'hearts', 'stars', 'horseshoes', 'clovers' ];
let starsItem = arr2.find(item => item === 'stars'); // 'stars'
I just care that the item might exist!
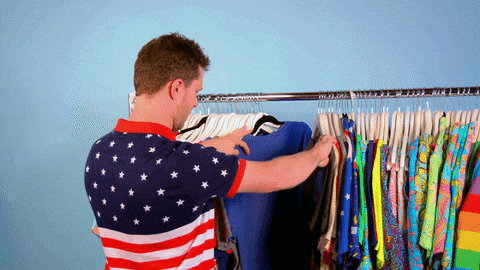
Array.prototype.some
Returns true/false if any item in the array passes the comparator function
As soon as function returns truthy, loop is exited
Skips over indices without assigned values
Considerations
- Changing values or adding indices are not tested/visited once some is invoked
Array.prototype.some
let arr = [
{ id: 1, color: 'red' },
{ id: 2, color: 'blue' },
{ id: 3, color: 'green' },
];
let foundItem = arr.some(item => item.color === 'red'); // true
let notFoundItem = arr.some(item => item.id === 4); // false
let arr2 = [ 'hearts', 'stars', 'horseshoes', 'clovers' ];
let starsItem = arr2.some(item => item === 'stars'); // true
Array.prototype.includes
Return true/false if the item is in the array
Uses SameValueZero algorithm for comparison
Considerations
- Works really well for primitive values
- Kind of like strict (===) equality
- -0 / +0 are the same
- NaN is NaN
Array.prototype.includes
let arr = [ 1, 2, 3, 4, 5, 3, 6, NaN ];
let found = arr.includes(3); // true
let notFound = arr.includes(7); // false
let nanFound = arr.includes(NaN); // true
let arr2 = [ { id: 1 }, { id: 2 }, { id: 3 } ];
let willNotWork = arr2.includes({ id: 2 }); // false
But, like, what about filter or reduce? Actually, I just care about using reduce.
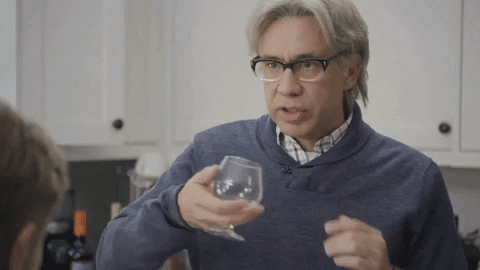
Array.prototype.filter
Return an array of items that pass the comparator function
Returns empty array if no items pass
Considerations
- Congrats! You have another array of items!
- Have to explicitly check for NaN
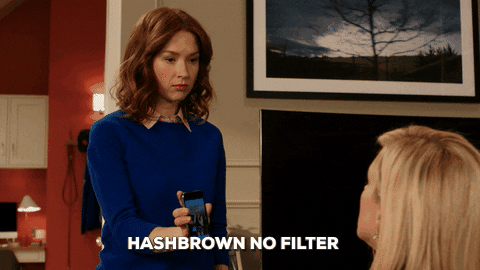
Array.prototype.filter
let arr = [
{ id: 1, color: 'red' },
{ id: 2, color: 'blue' },
{ id: 3, color: 'green' },
];
let foundItem = arr.filter(item => item.color === 'red');
// [ { id: 1, color: 'red' }]
let notFoundItem = arr.some(item => item.id === 4); // [ ]
let arr2 = [ 'hearts', 'stars', 'horseshoes', 'clovers' ];
let starsItem = arr2.some(item => item === 'stars');
// [ 'stars' ]
Array.prototype.reduce
Returns anything you want
Considerations
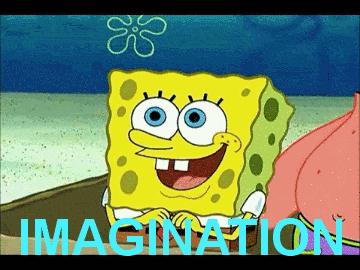
- Your imagination
- Have to explicitly check for NaN
Array.prototype.reduce
let arr = [
{ id: 1, color: 'red' },
{ id: 2, color: 'blue' },
{ id: 3, color: 'green' },
];
let foundItem = arr.reduce((acc, curr) => {
if (curr.color === 'red') return curr;
return acc;
}, null);
// { id: 1, color: 'red' }
I just care about performance. Talk about that.
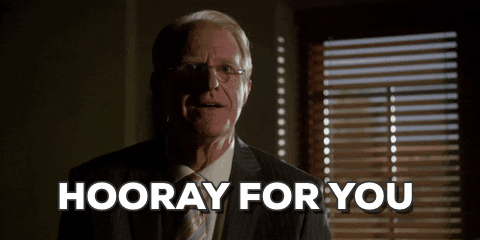
Performance
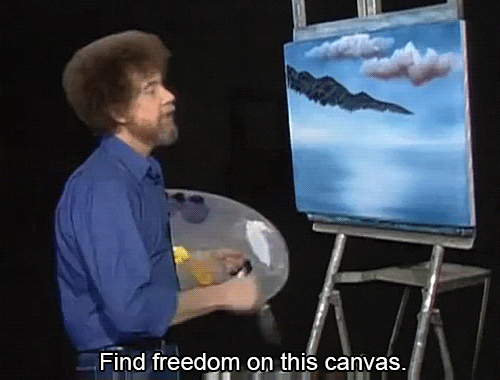
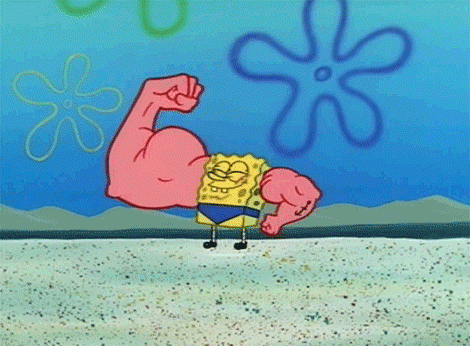
Resources
Selection of Examples (repl.it): https://bit.ly/2Lj2ZPV
MDN for Arrays: https://mzl.la/1kML43T
SameValueZero Algorithm: https://mzl.la/2wihz4x
Copy of What To Do When You Don't Care (About Finding Items In Arrays) [What Do You Care About?]
By Mike Frazier
Copy of What To Do When You Don't Care (About Finding Items In Arrays) [What Do You Care About?]
Presented at DenverScript 08/28/2018
- 91