Pipeline Operator and
Partial Application Syntax
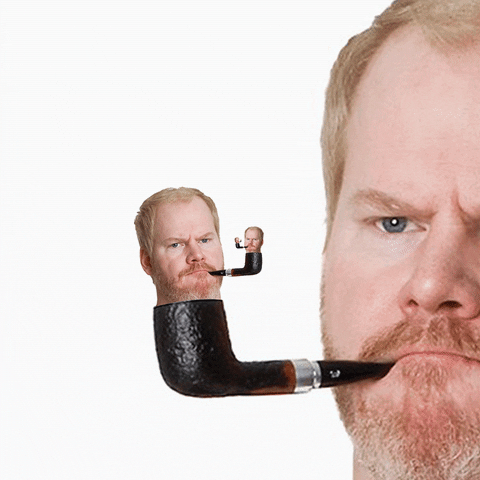
About Mike Frazier
Software Engineer @ ClickFox
Hack Reactor Remote
Educator
Skateboarder
Music stuff
Please Note...
Everything that is presented may change tomorrow. Or next week. Or never.
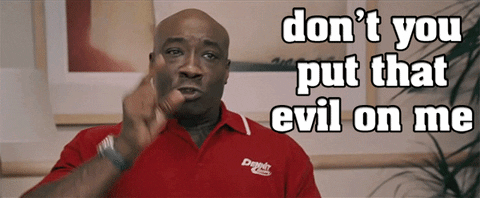
Pipeline Operator
-
|>
- Stage 1 proposal (of 4)
- Expect major changes after being accepted to Stage 2
- Can be used in FireFox 58+
-
--enable-pipeline-operator
-
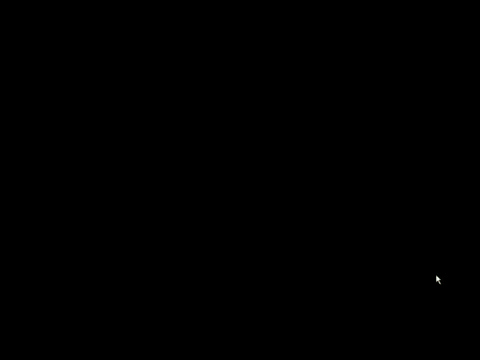
Pipeline Operator
Minimal Proposal Syntax
-
|>
-
expression |> function
- Allows for easier chaining of function calls
-
input |> f1 |> f2 |> f... |> fn
-
fn(f...(f2(f1(input)))
-
- Using with functions with multiple parameters
-
const add = (x, y) => x + y
-
10 |> ( _ => add(5, _) )
-
Pipeline Operator
Minimal Proposal Syntax Example
const doubleSay = (str) => str + ", " + str;
const capitalize = (str) => str[0].toUpperCase() + str.substring(1);
const exclaim = (str) => str + '!';
let res = "hello" |> doubleSay |> capitalize |> exclaim
let res = exclaim(capitalize(doubleSay("hello")))
console.log(res) // "Hello, hello!"
Pipeline Operator
Minimal Proposal Limitations
-
No support for async-await functions
-
Will throw an error
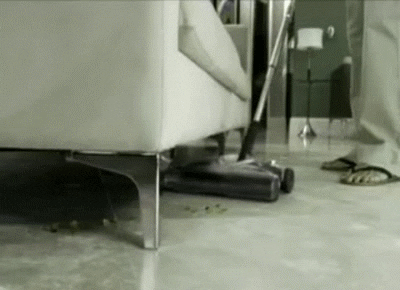
Pipeline Operator
Competing Proposals
-
- Invokes the right-hand side with the evaluated result of the left
- Implicit invocations
- Includes support for await
x |> f //--> f(x)
x |> f(y) //--> f(y)(x)
x |> (a => f(a,10)) //--> f(x,10)
// Async Solution
x |> f |> await //--> await f(x)
x |> f |> await |> g //--> g(await f(x))
Pipeline Operator
Competing Proposals
-
Hack Style Only
- Evaluates the left-hand side and assigns it to a temporary binding scoped to the right-hand side
- Fully explicit invocations
- Includes support for await
x |> f(#) //--> f(x) x |> f(y)(#) //--> f(y)(x) x |> f //--> Syntax Error x |> f(#,10) //--> f(x,10) // Async Solution x |> await f(#) //--> await f(x) x |> await f(#) |> g(#) //--> g(await f(x)) [Note: # is not the exact symbol for the temporary scoped binding]
Pipeline Operator
Competing Proposals
-
Split Mix Style
- One operator for implicit invocation (F# style),|>
- One operator for explicit placeholders (Hack style),|: or |>>
-
Smart Mix Style
- Only one operator required
- Method binding naturally occurs: … |> document.querySelector |> … is same as … |> document.querySelector(#) |> …
- Does not need additional partial application syntax
Partial Application Syntax
-
Stage 1 proposal
- Expect major changes after being accepted to Stage 2
- Currently no browser support
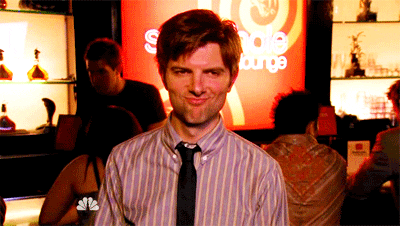
Partial Application Syntax
- Allows partial application of an argument list to a function invocation using ? as a placeholder for later
- Can be viewed as a replacement for bind method
-
add.bind(null, 1)
-
-
const addOne = add(1, ?); // apply from the left
-
addOne(2); // 3
-
-
const addTen = add(?, 10); // apply from the right
-
addTen(2); // 12
-
Putting It All Together
-
x |> add(1, ?)
-
where x is applied as second argument to add
-
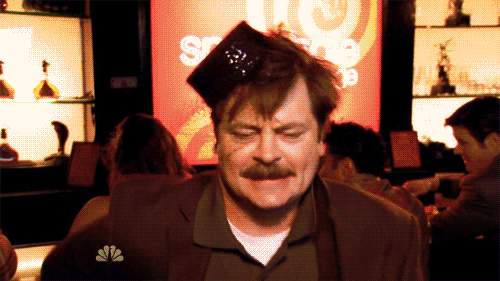
Putting It All Together
const player = { score: 44 }; let newScore = player.score |> add(8, ?) |> divide(?, 2) |> multiply(?, 3) |> subtract(?, 23) |> (x => Math.max(0, Math.min(?, 100))) console.log(newScore) // 55
Can I Use?
Pipeline Operator
FireFox v58+ Flag :: --enable-pipeline-operator
Babel Plugin :: @babel/plugin-proposal-pipeline-operator
James-H33 :: Pipeline Operator Repo
Partial Application Syntax
citycide :: param.macro Repo
Final (Deep) Thoughts
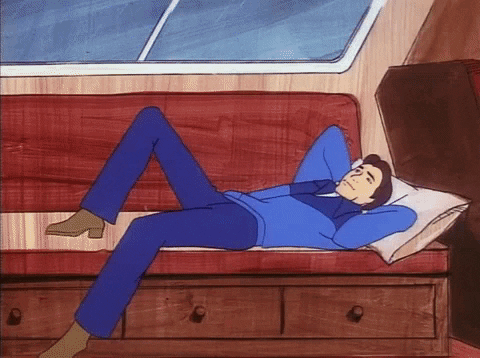
My opinion?
Resources
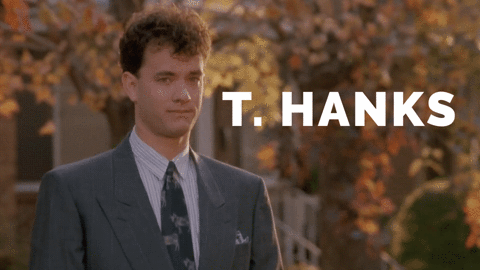
Pipeline Operator and Partial Application Syntax
By Mike Frazier
Pipeline Operator and Partial Application Syntax
Presented at DenverScript 11/27/2018
- 164