nano vazquez
@nanovazquez87

REACT

Typescript
Hi! I'm nano VAZQUEZ
Full-stack developer (10+ years)
International speaker
TechLead @ MuleSoft, a Salesforce company
Co-organizer of conferences & meetups
ReactBA, .NETConfAR & DevDayAr
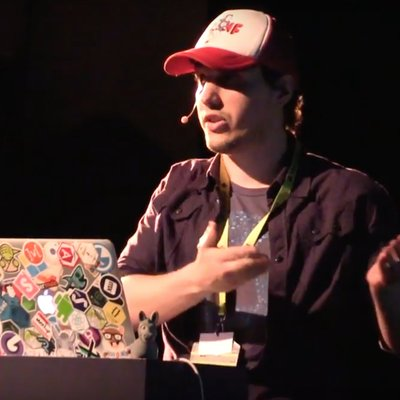
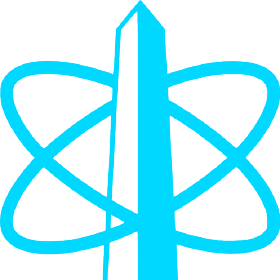
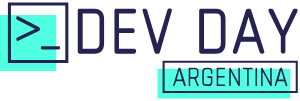
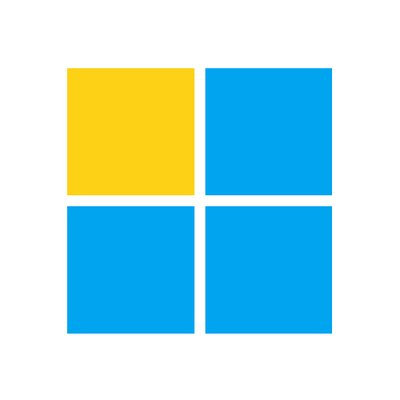
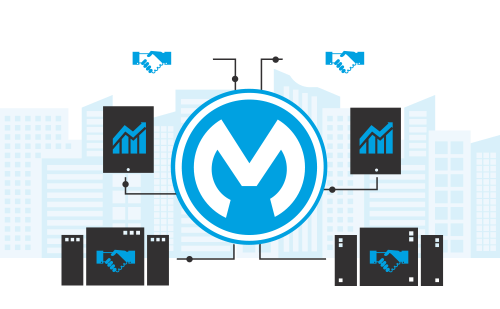
.NET CONF AR v2018
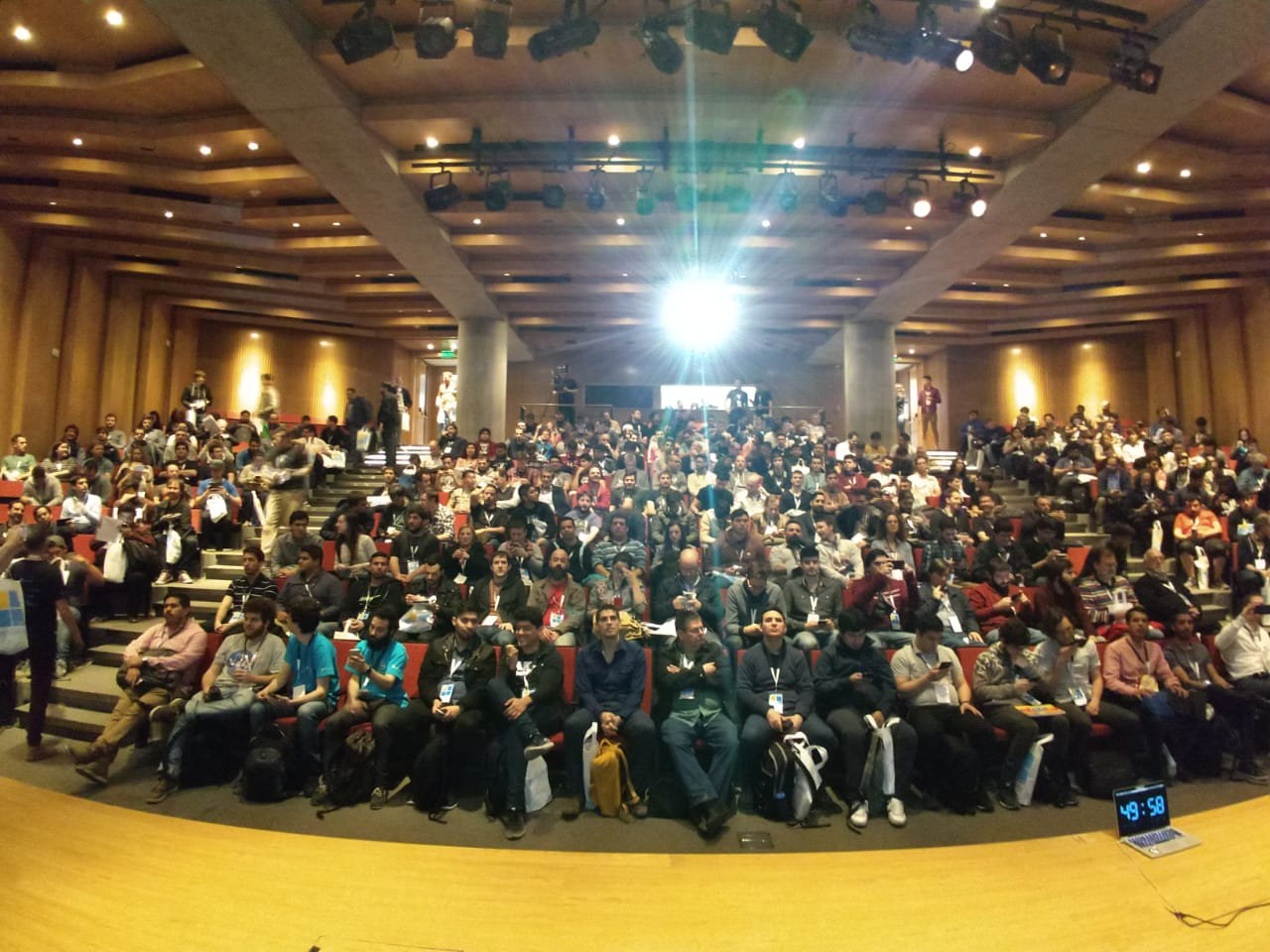
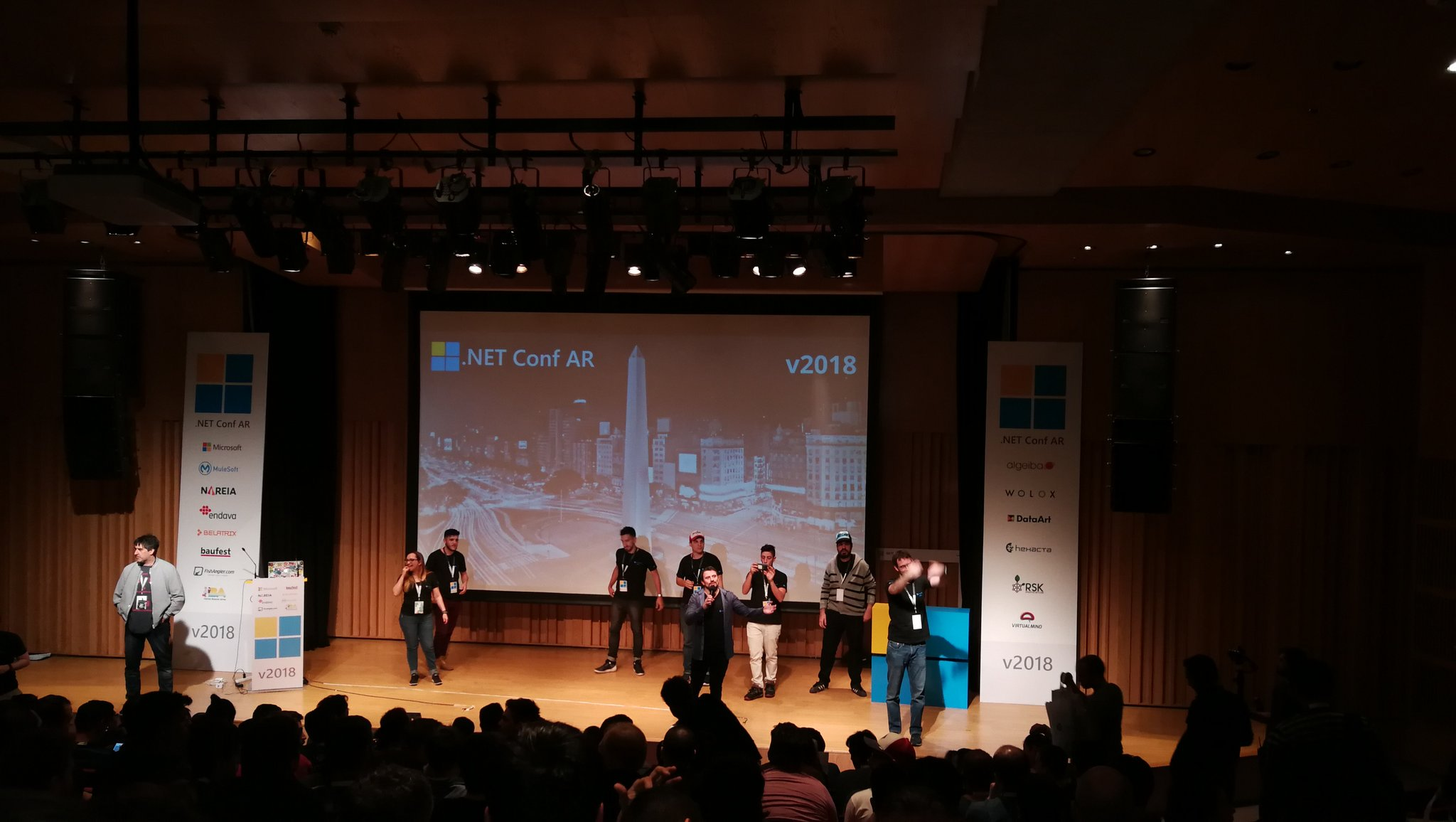
A QUICK SURVEY
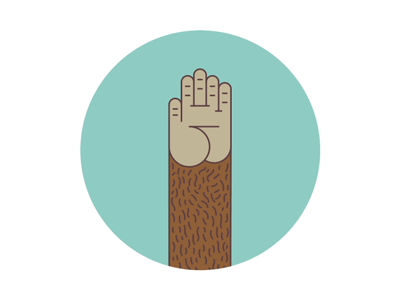
AGENDA
#1
DEFINE THE PROBLEM
#2
pick the best SOLUTION
#3
IMPLEMENT IT
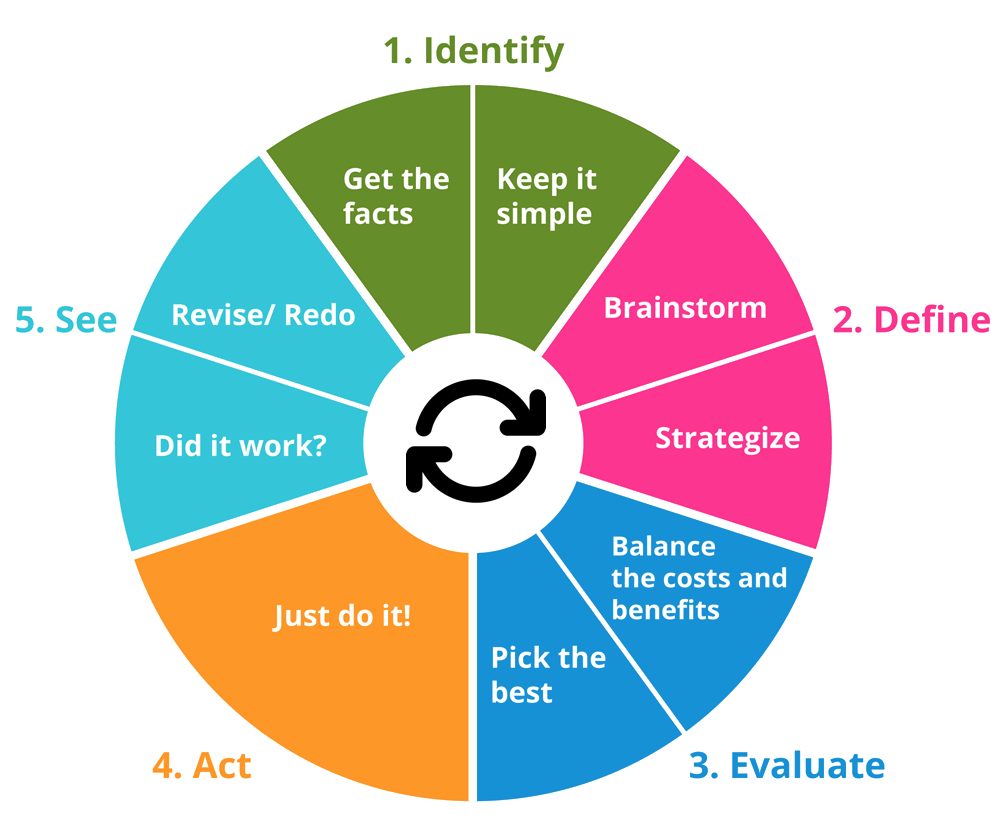
DEFINE THE PROBLEM
HOw can we build APPS in a complex and dynamic environment?
What type of apps
A desktop app (ReactXP)
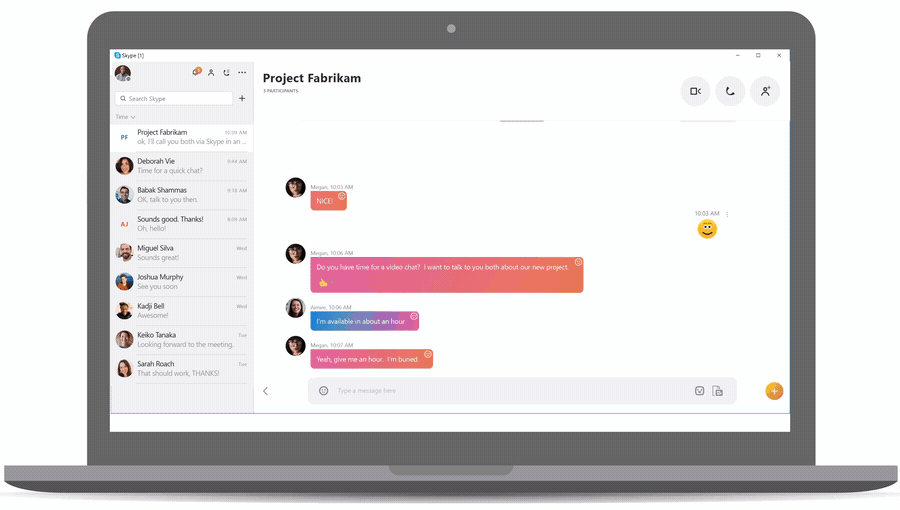
What type of apps
A web app (React)
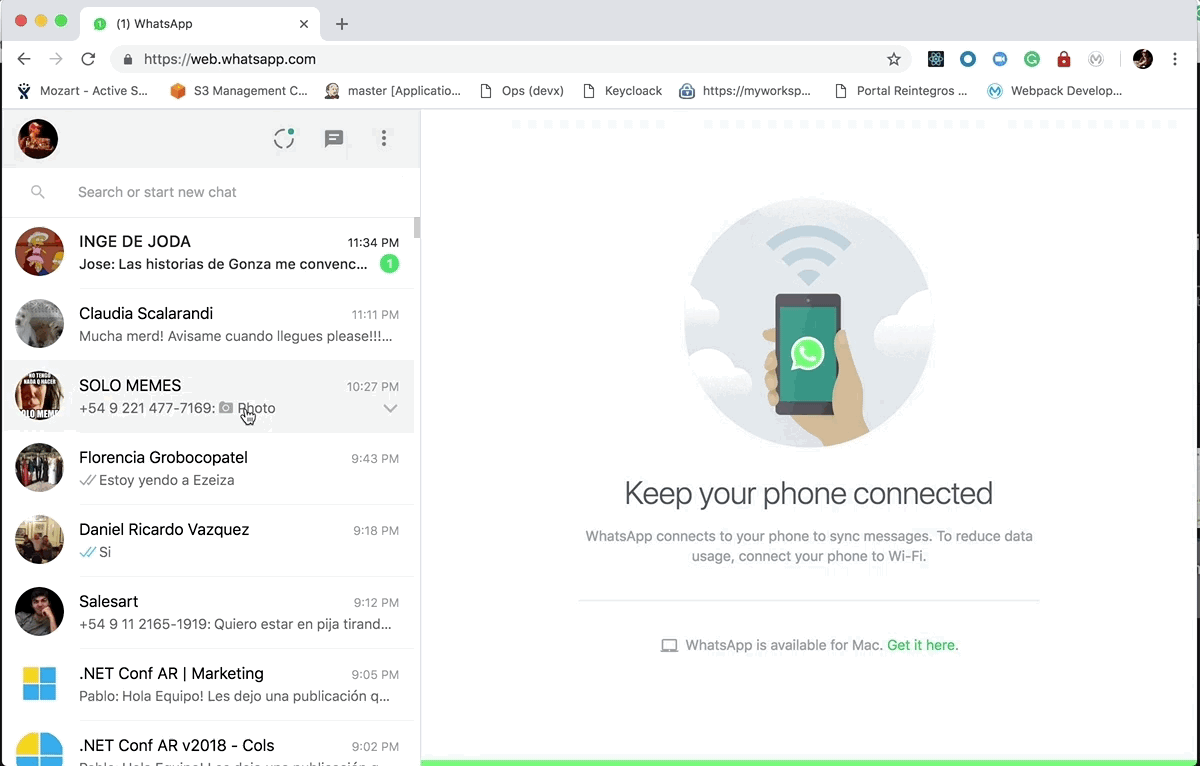
What type of apps
A mobile app (no idea)
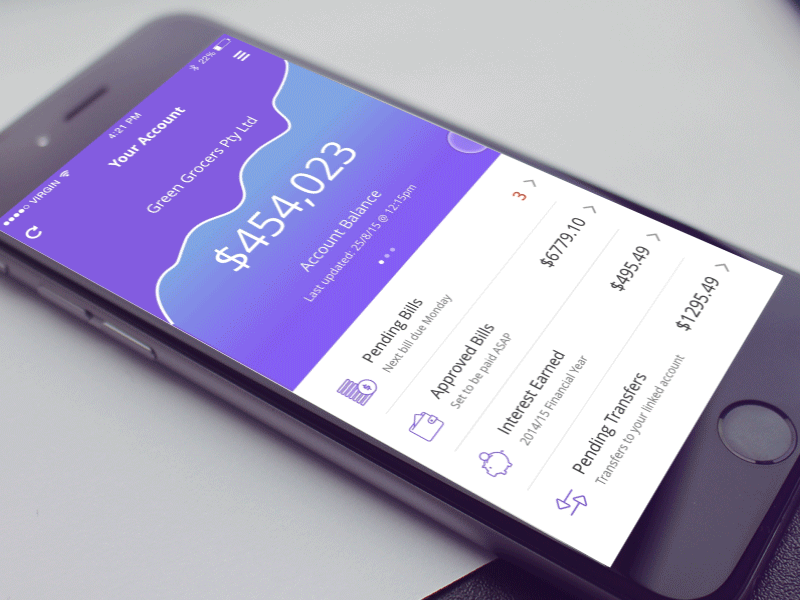
HOw complex
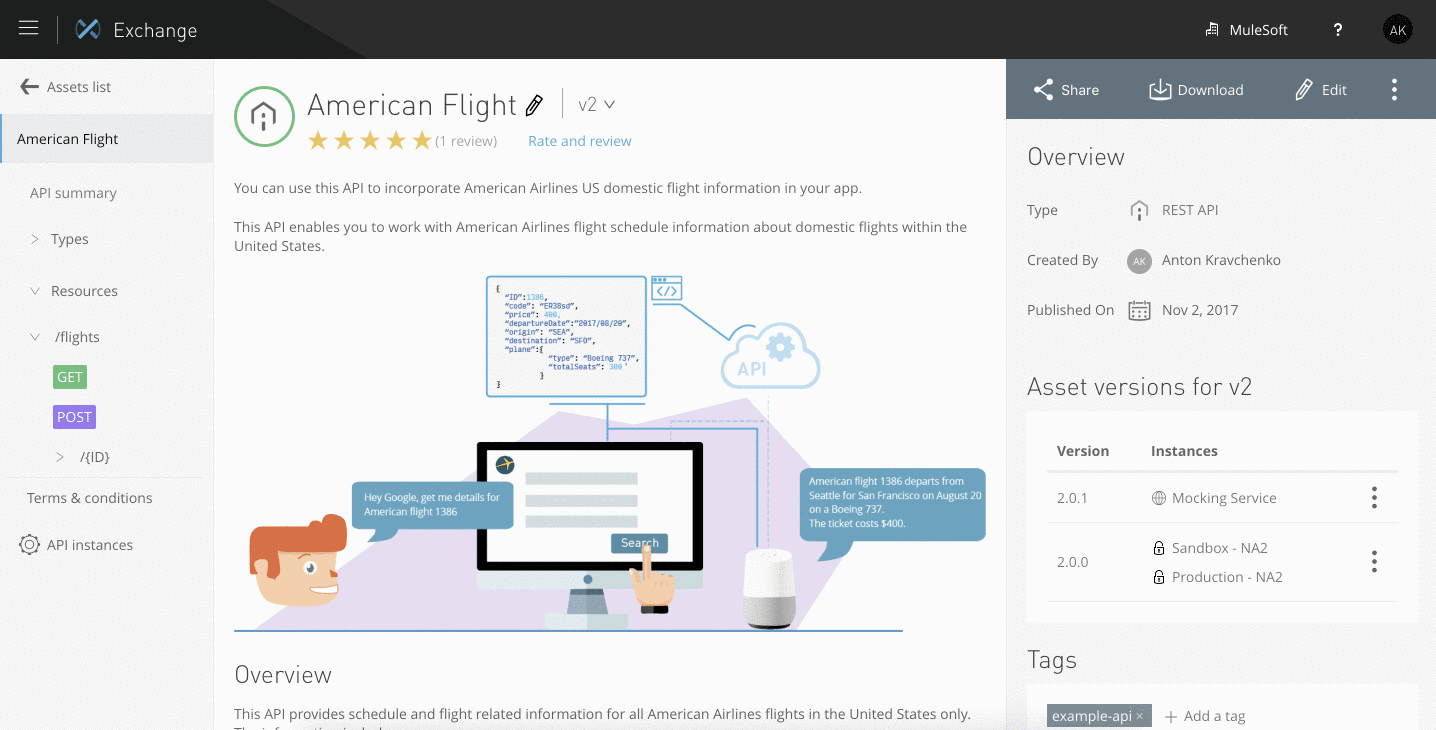
"CRUD" apps (SSR)
HOw complex
IDEs (React + TypeScript)
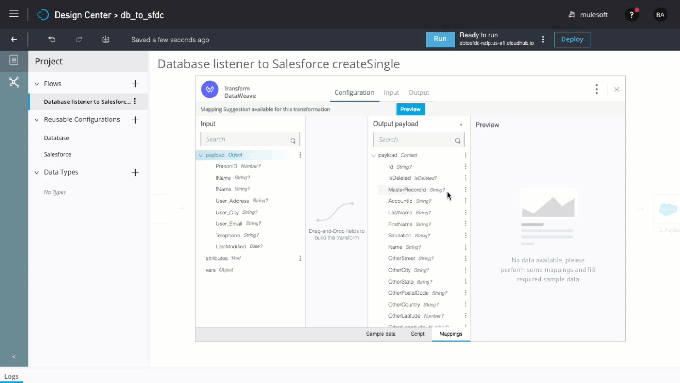
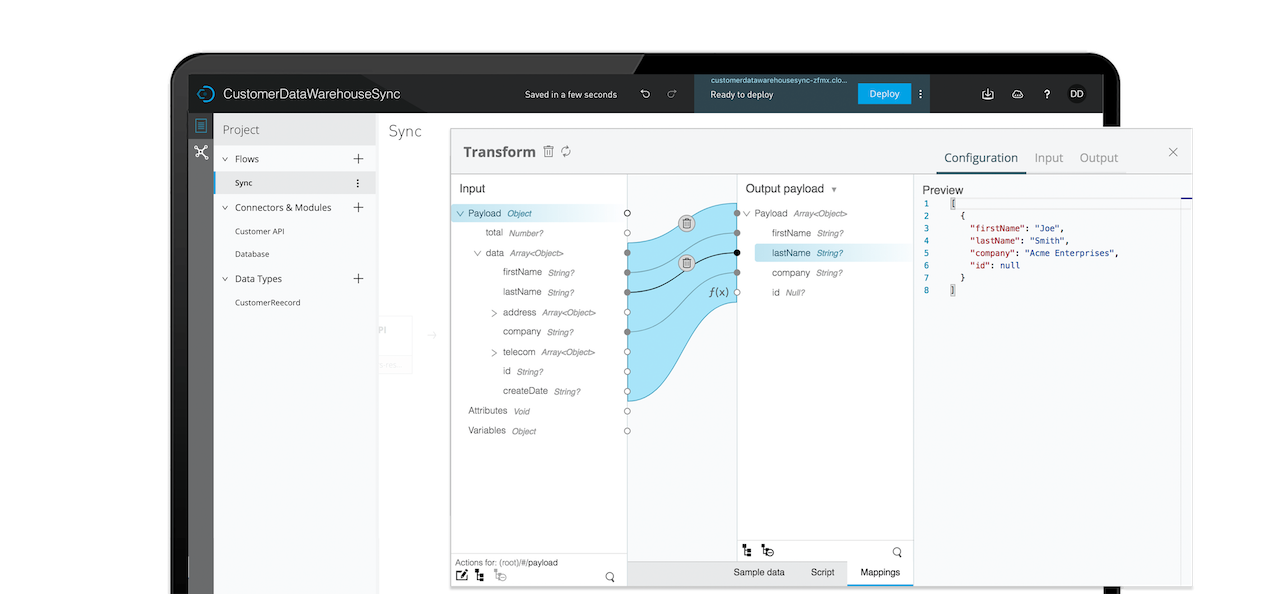
HOw DYNAMIC
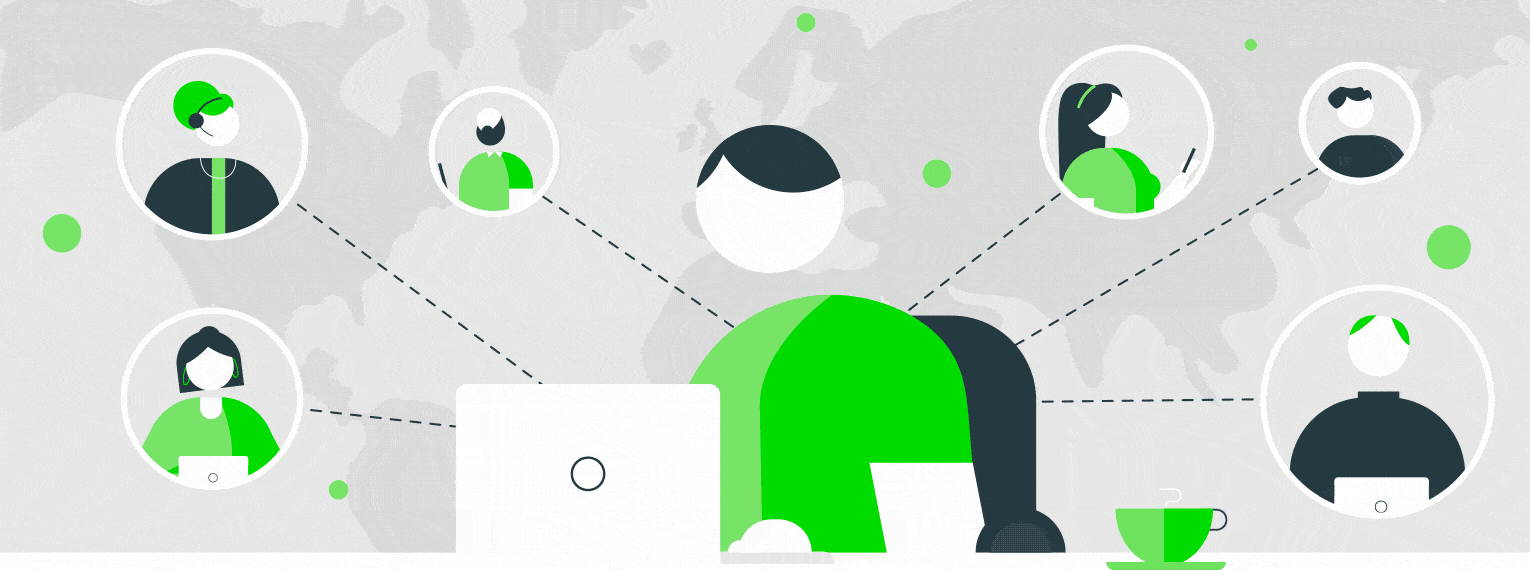
Remote teams everywhere
HOw DYNAMIC
Priorities changing very often
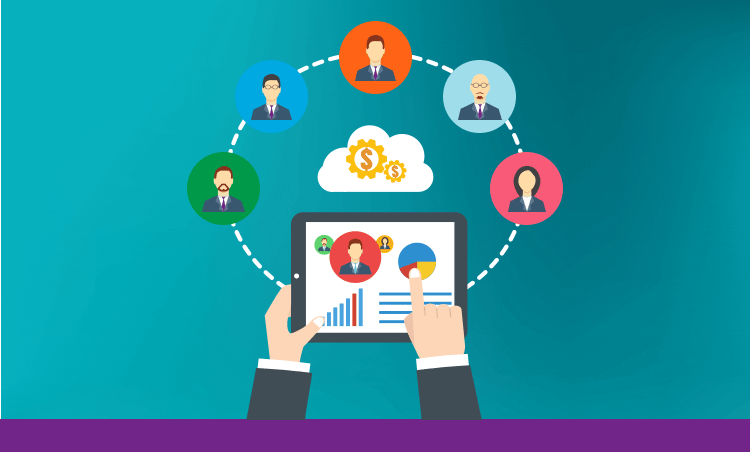
HOw DYNAMIC
Everything is connected
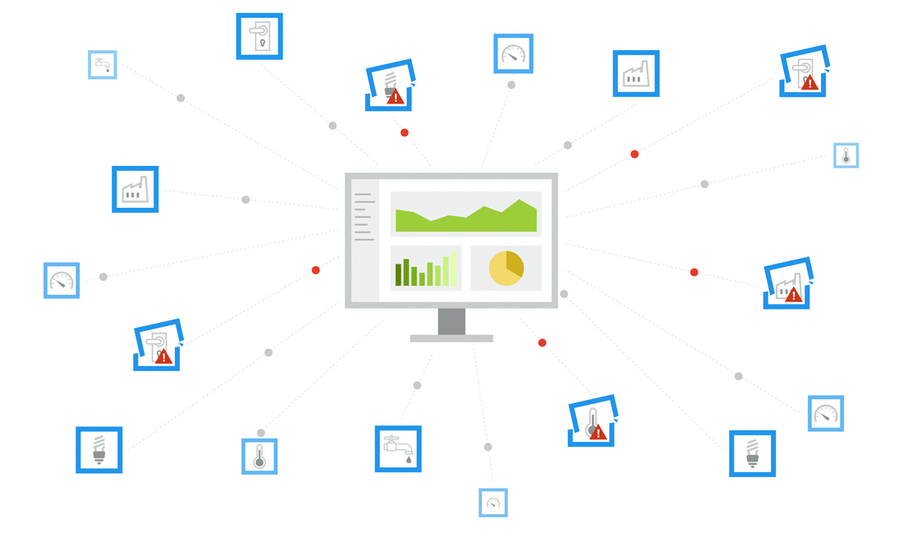
SUMMARY
#1
WHAT TYPE OF APPS?
#2
HOW COMPLEX?
#3
HOW DYNAMIC?
Any kind of app
All levels of complexity
You
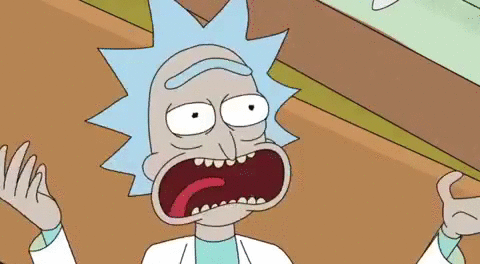
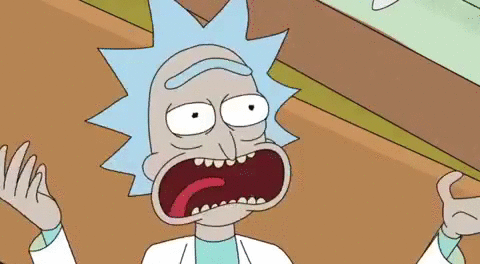
are
doomed
PICK THE BEST SOLUTION
SCENARIO
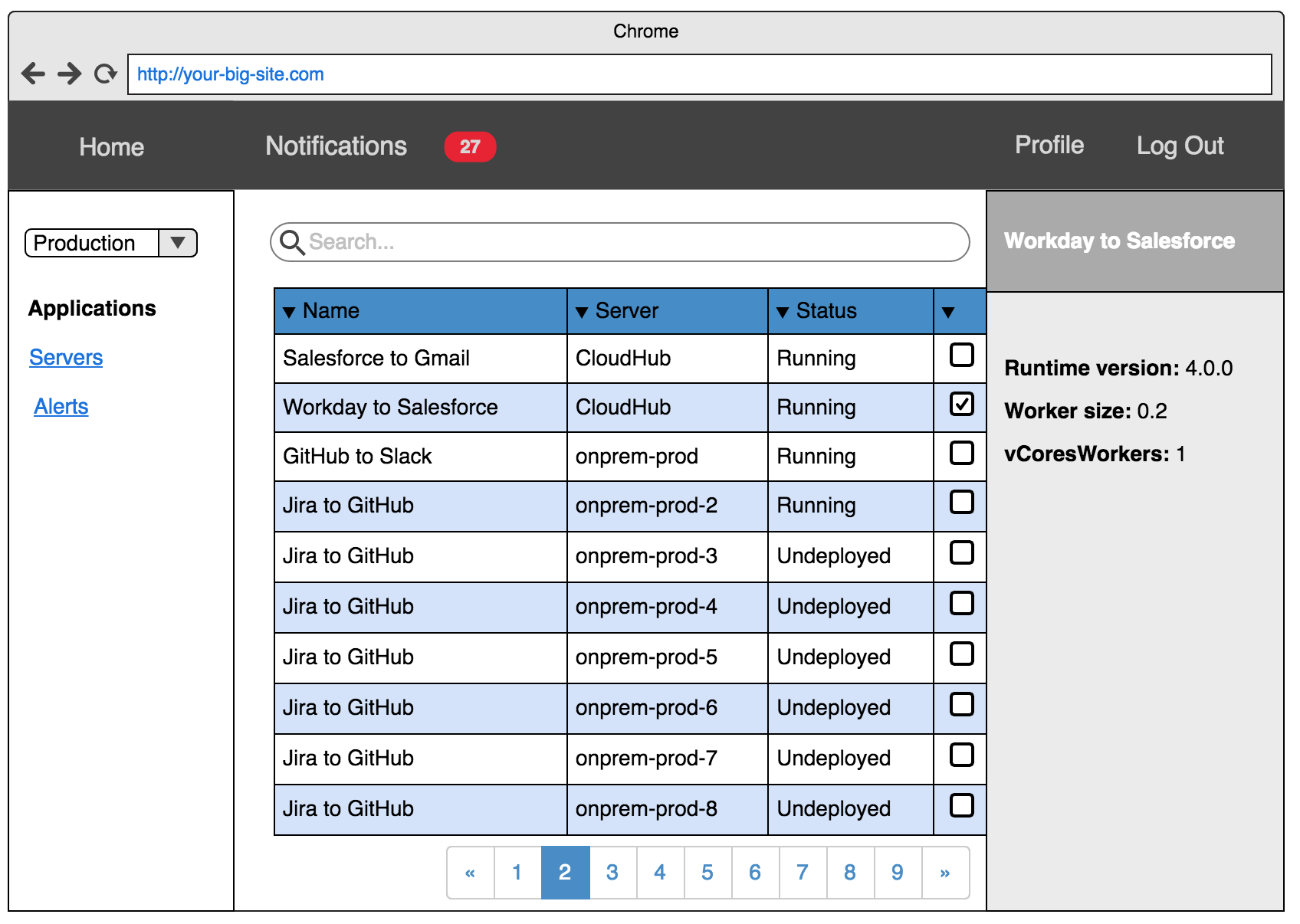
COMPONENTS
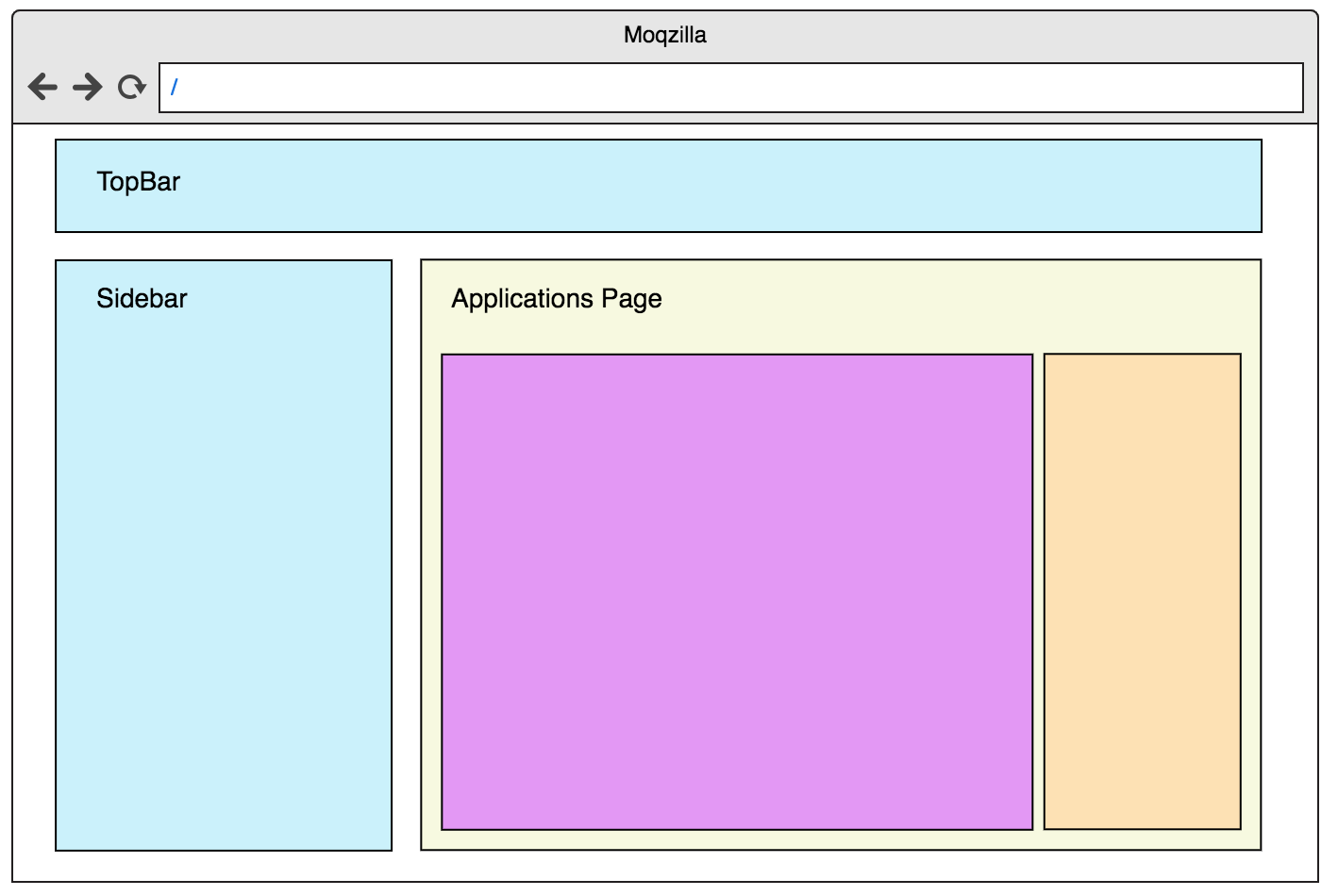
SPLIT THE PROBLEM INTO PIECES
COMPONENTS
Components let you split the UI into independent, reusable pieces, and think about each piece in isolation.
import { ApplicationsTable, Sidebar } from 'src/components';
class Application extends React.PureComponent {
render() {
const { items, selectedItem } = this.props;
return (
<div className="applications-page">
<ApplicationsTable items={items} />
<Sidebar selectedItem={selectedItem} />
</div>
);
}
}
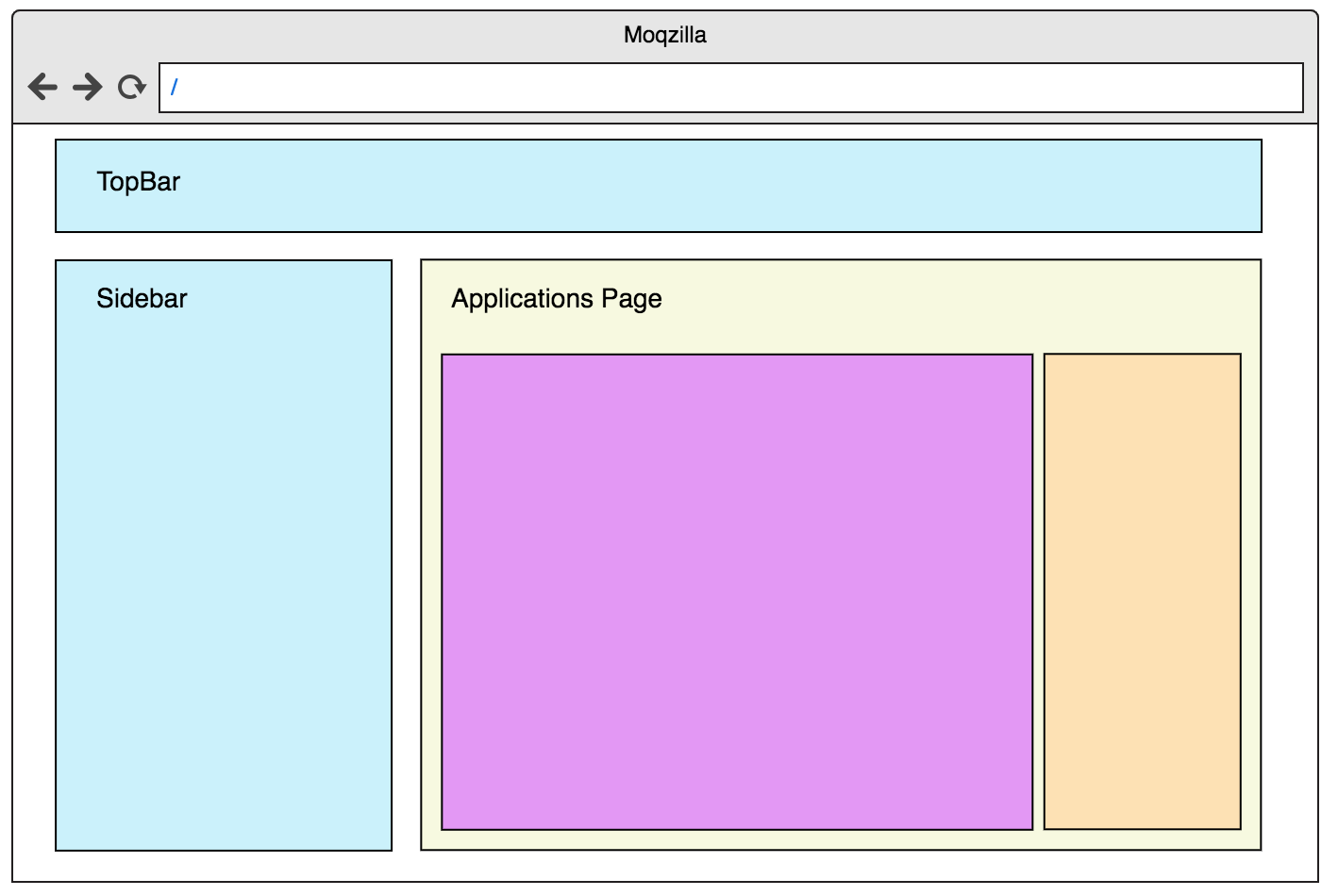
import { ApplicationsTable, Sidebar } from 'src/components';
class Application extends React.PureComponent {
render() {
const { items, selectedItem } = this.props;
return (
<div className="applications-page">
<ApplicationsTable items={items} />
<Sidebar selectedItem={selectedItem} />
</div>
);
}
}
COMPONENTS
Components = Modularity + encapsulation
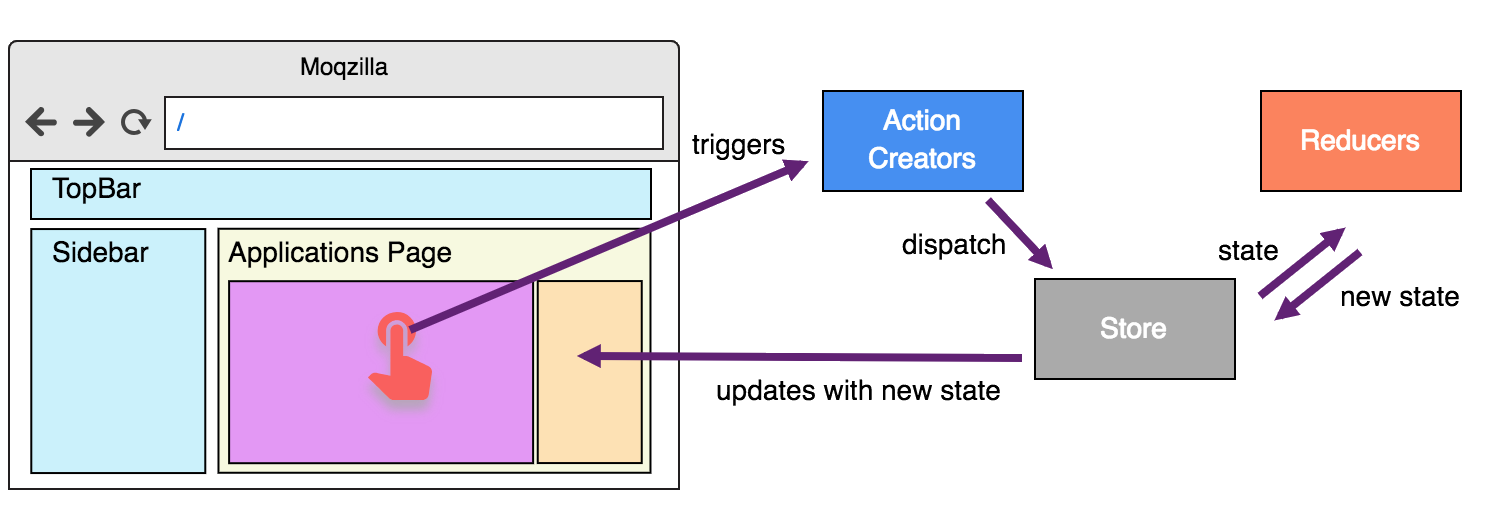
But we need CONTRACTS!
<Button
/** Component content */
children: any,
/** Determines the style of the button */
kind?: KindT,
/** Theme object for the component */
theme?: ThemeT,
/** ID applied to Button component */
testId?: string,
/** Provides an alternative style with transparent background */
noFill?: boolean,
/** Handler called when the user clicks the component */
onClick?: () => void,
/** className applied to the component */
className?: string,
/** If true, the component is disabled */
disabled?: boolean,
/** If true, displays a loading indicator and disables the component */
isLoading?: boolean,
/** If true, sets rounded borders in the component */
isCircular?: boolean
>
Click me
</Button>

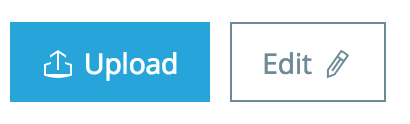

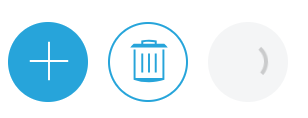
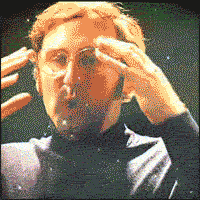
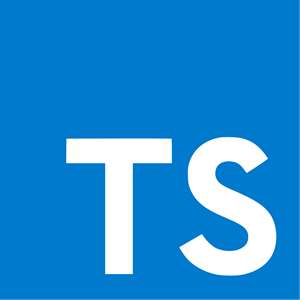
IMPLEMENT
IT
A QUICK SURVEY
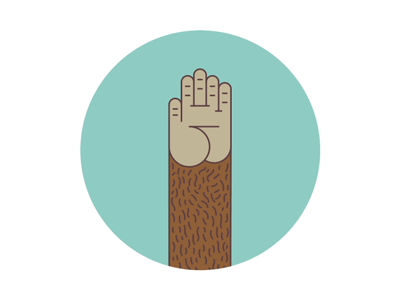
Choose your path
#1
Create UI APPS (SPA)
#2
Create UI LIBRARIES
#3
Create micro frontends
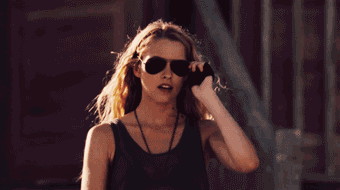
DEMO
UI APPS (SPA) + UI COMPONENTS
DEMO
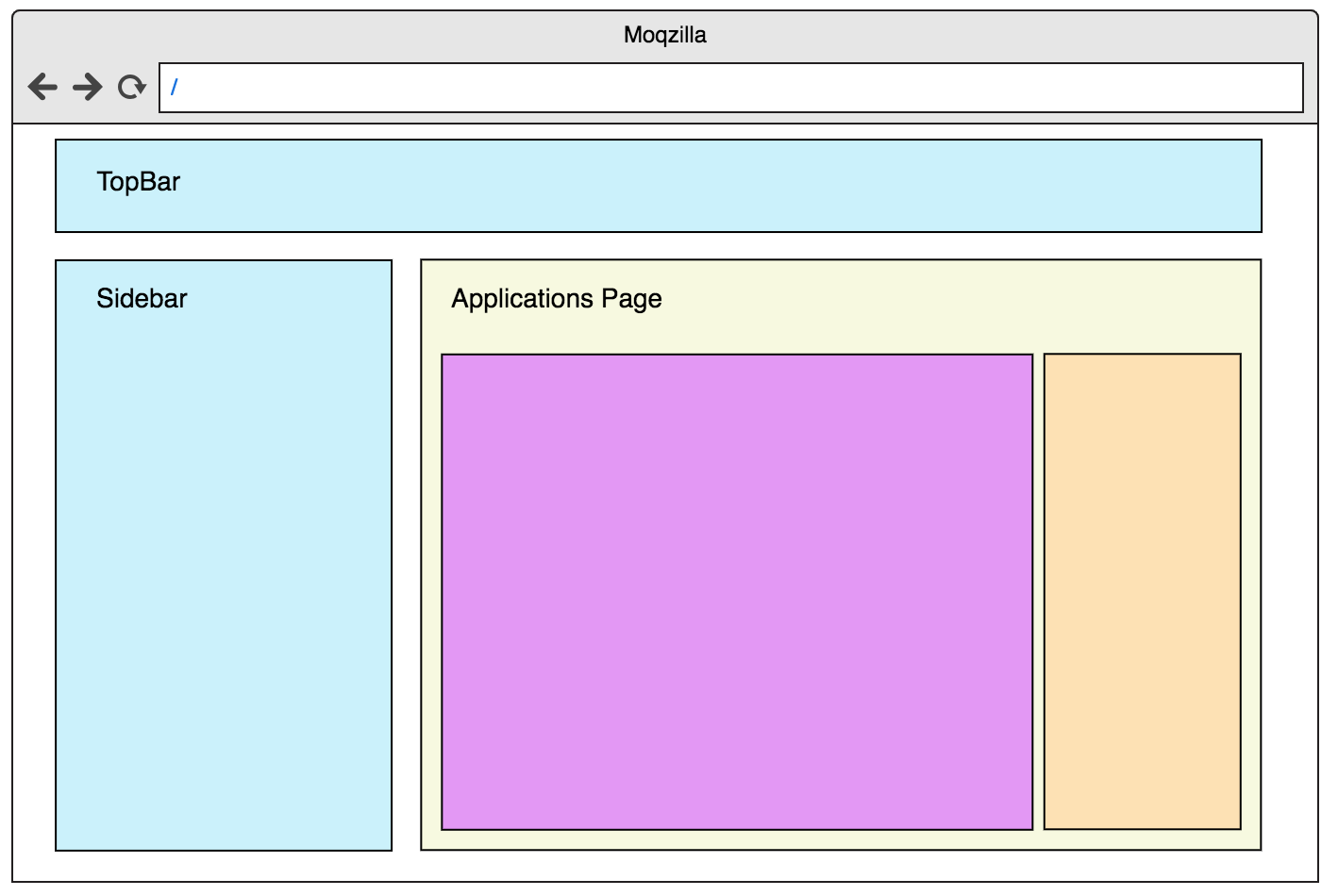
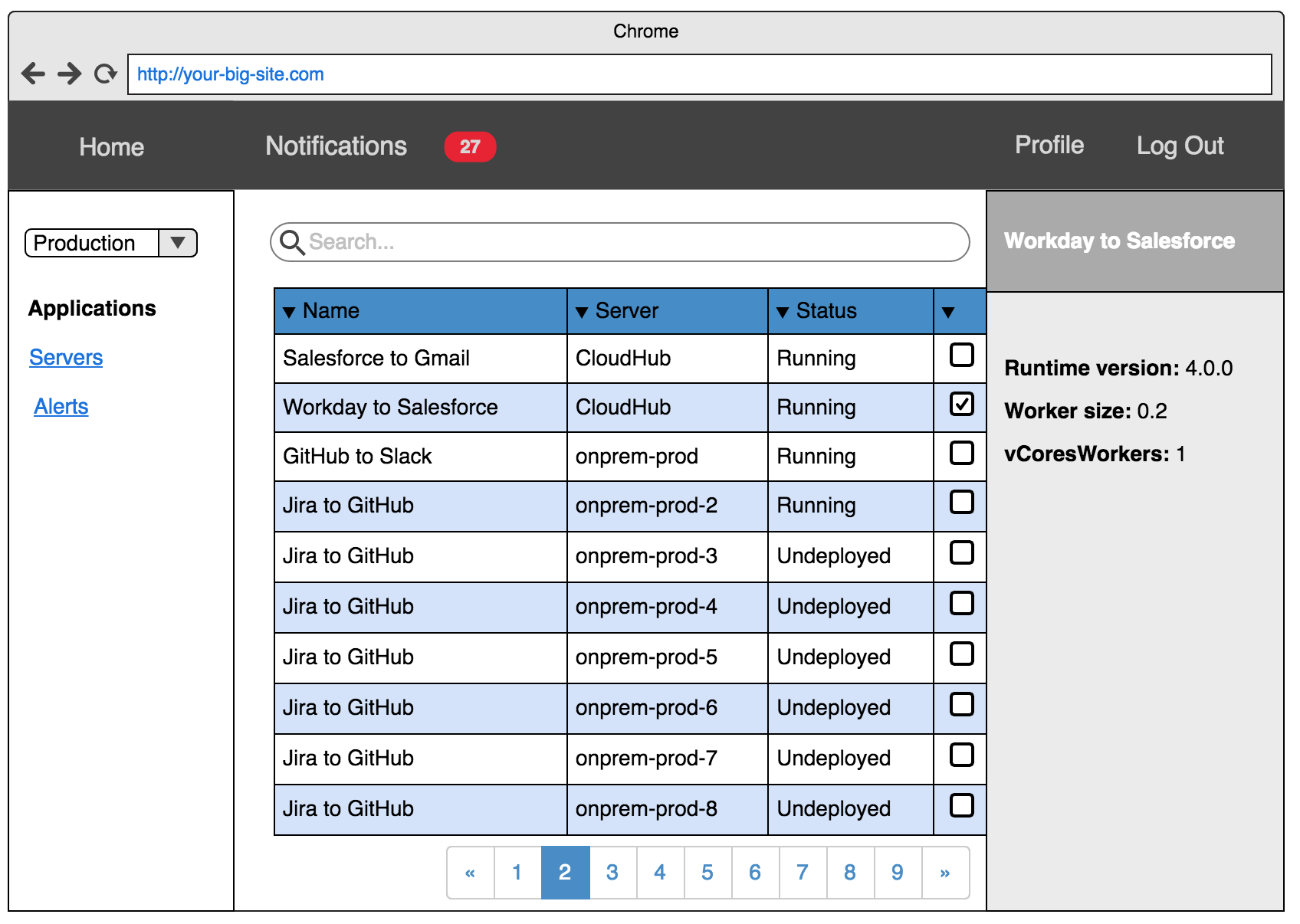
TOPBAR
import React from "react";
import styles from "./styles.module.css";
import { IProps } from "./types";
export default class TopBar extends React.PureComponent<IProps> {
static defaultProps = {
productName: "Anypoint Platform"
};
render() {
const { productName, user } = this.props;
return (
<div className={styles.topBar}>
<div className={styles.hamburger} />
<div className={styles.product}>{productName}</div>
<div className={styles.settings}>
<span className={styles.help}>?</span>
{user && <span className={styles.userInitials}>{user.initials}</span>}
</div>
</div>
);
}
}
TOPBAR
import { IUser } from "../types";
export interface IProps {
/** The name of the active product */
productName?: string;
/** The user logged in */
user?: IUser;
}
export interface IUser {
id: string;
name: string;
initials: string;
}
TOPBAR
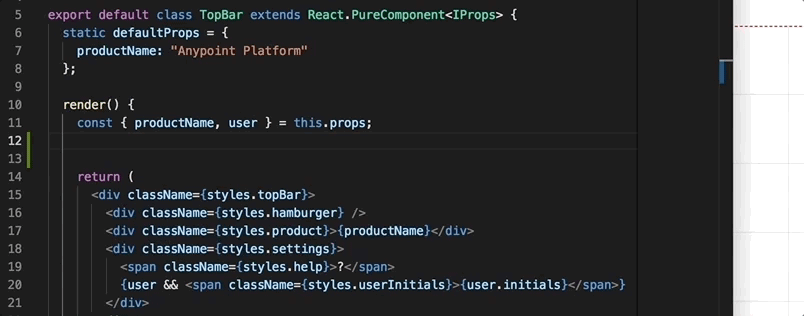
SIDEBAR
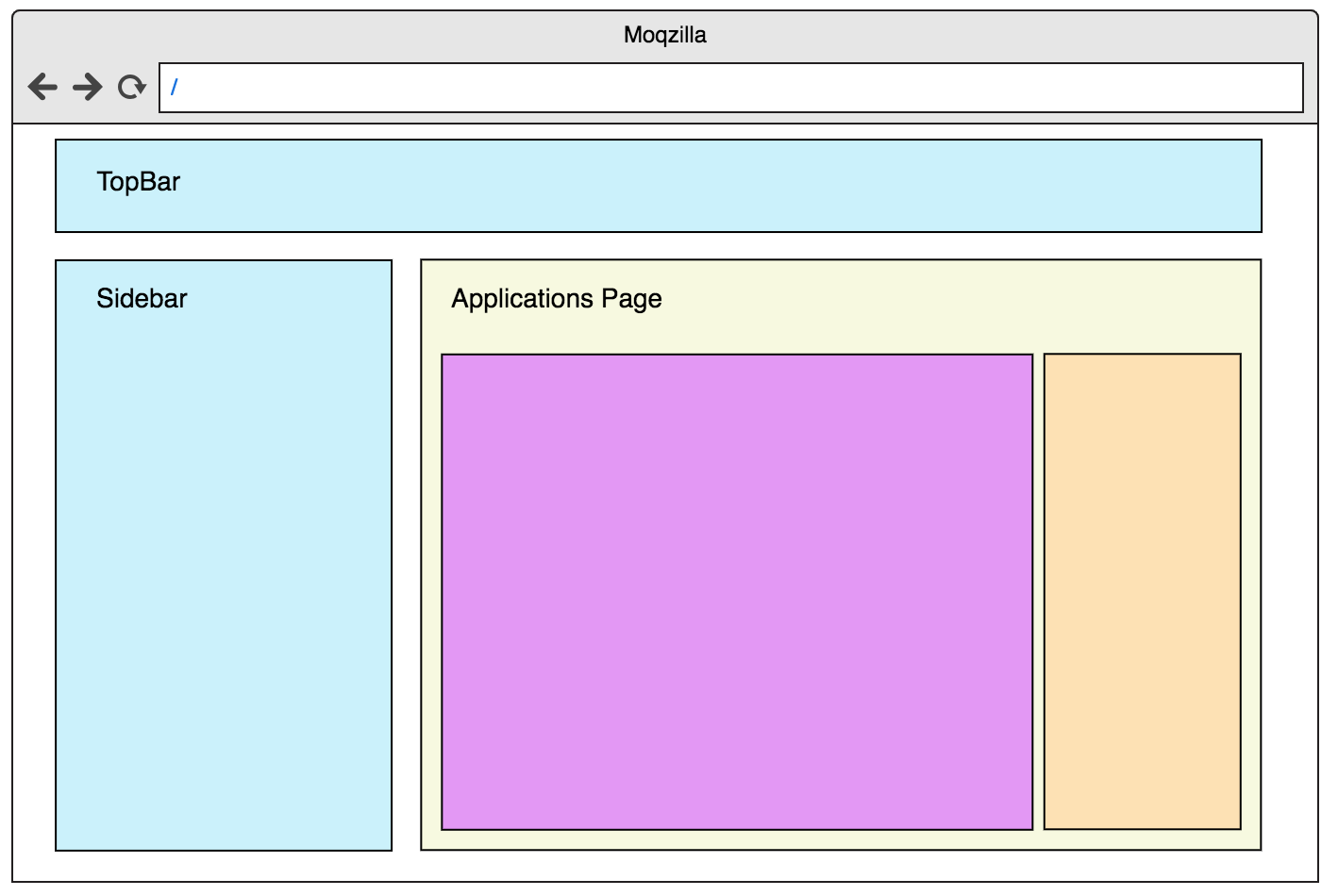
SIDEBAR
import React from "react";
import { IProps } from "./types";
import { IPage } from "../types";
import styles from "./styles.module.css";
export default class SidePanel extends React.PureComponent<IProps> {
static defaultProps = {
pages: []
};
getPage = (page: IPage) => {
...
}
render() {
const { pages } = this.props;
return (
<div className={styles.sidePanel}>
{pages.map(this.getPage)}
</div>
);
}
}
Sidebar
import React from "react";
...
export default class SidePanel extends React.PureComponent<IProps> {
...
getPage = (page: IPage) => {
const { activePage } = this.props;
const cssClassNames = activePage.url === page.url
? styles.activeLink
: styles.link;
return (
<a key={page.url} href={page.url} className={cssClassNames}>
{page.title}
</a>
);
}
render() {
...
}
}
APPLICATIONS PAGE
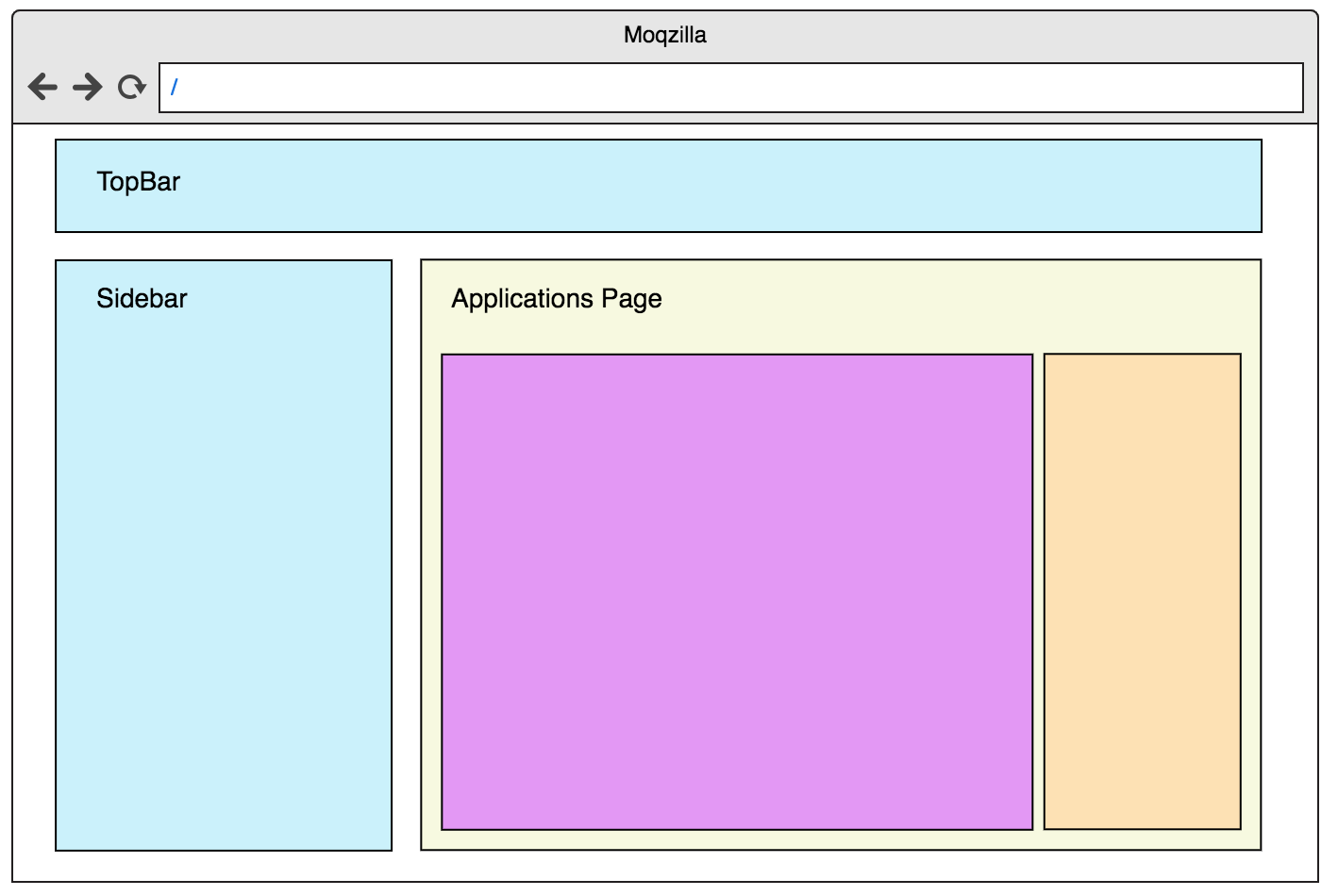
APPLICATIONS PAGE
import React from "react";
import ReactTable from "react-table";
import applicationsService from "../../services/applicationsService";
...
export default class ApplicationsPage extends React.PureComponent<IProps, IState> {
state = { applications: [] };
componentDidMount() {
applicationsService.getApplications().then(applications => {
this.setState({ applications });
});
}
render() {
const { applications } = this.state;
const columns = [ { Header: "Name", accessor: "name" }, ...];
return (
<div className={styles.applicationsPage}>
<ReactTable data={applications} columns={columns} className={styles.table} />
</div>
);
}
}
App
import React, { Component } from "react";
import { ApplicationsPage, SidePanel, TopBar } from "./components";
import styles from "./App.module.css";
class App extends Component {
render() {
return (
<div className={styles.app}>
<TopBar user={user} productName={productName} />
<SidePanel pages={pages} activePage={activePage} />
<ApplicationsPage />
</div>
);
}
}
export default App;
App
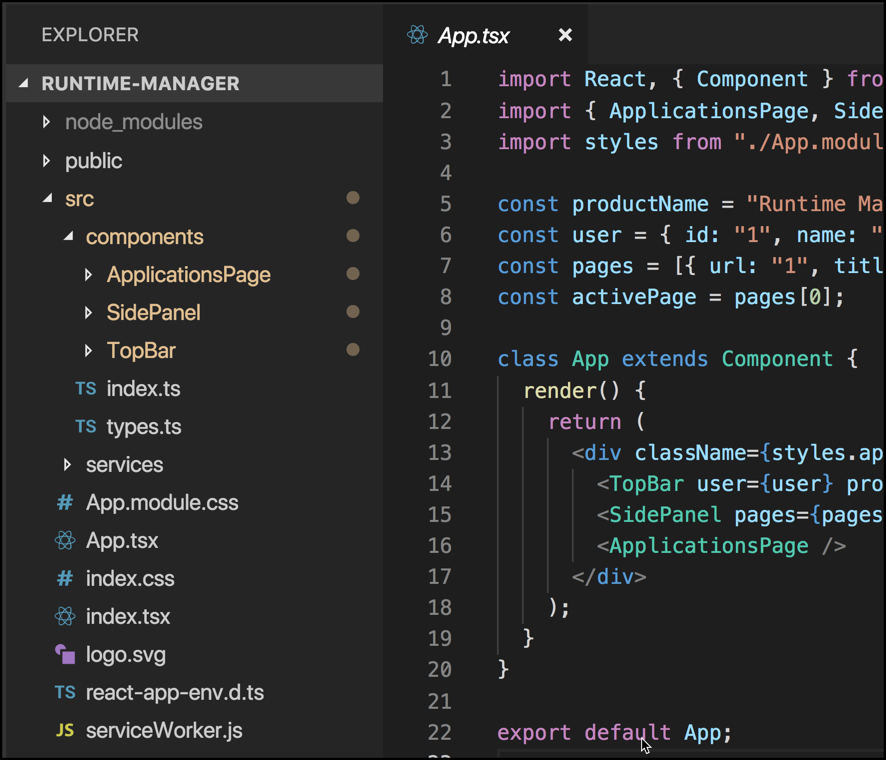
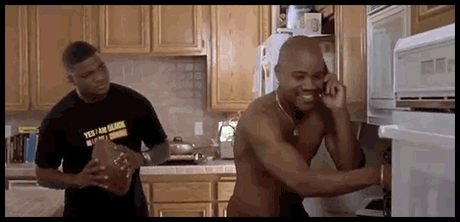
Questions
..?
More INFO
Thanks!
TypeScript ❤ React
By Mariano Vazquez
TypeScript ❤ React
TypeScript provides enormous help for creating large JavaScript applications, by assisting developers to communicate better and detect errors. ReactJS was created to simplify the development of web-applications, providing rendering speed and scalability. Can we use both together? Yes! This talk is focused on showing how easy is to build large applications with React and TypeScript and the benefits you, your app and the consumers of it will get by combining these two powerful tools.
- 1,790