Java Multithreading
Parsa Hejabi
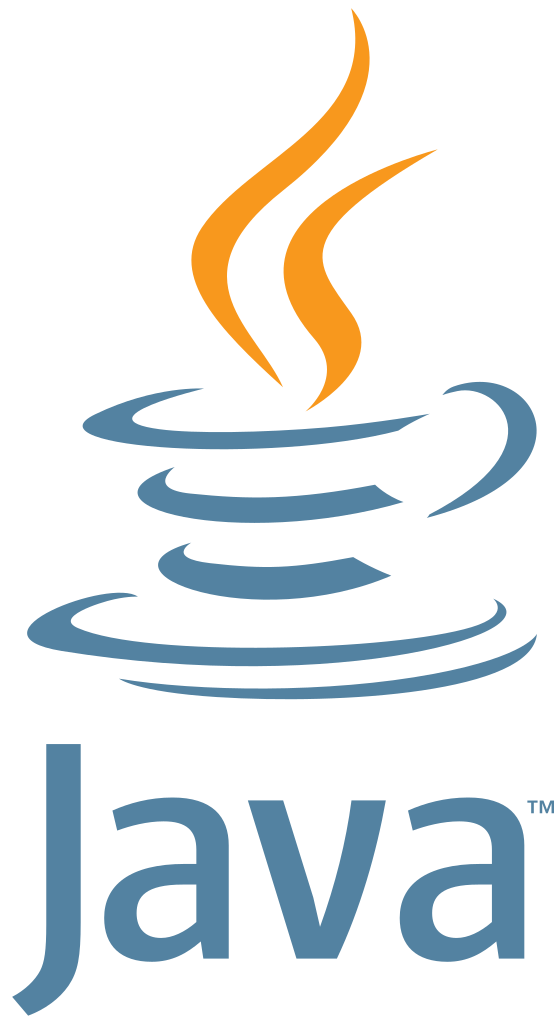
Table of Contents
- Concept of Concurrency.
- What is Thread and what is Multi-Thread programming?
- What is synchronization?
- What states a Thread has?
"everything Sequential
...!"
What is a String?
- A sequence of characters.
- In Java, Strings are treated as objects.
- String is a predefined Class in Java.
- Like any class that you create...
String Class
- You can create a string by just typing OR create with "new" keyword...
- 11 constructors for String class.
String greeting = "Hello world!";
public class StringDemo { public static void main(String args[]) { char[] helloArray = { 'h', 'e', 'l', 'l', 'o', '.' }; String helloString = new String(helloArray); System.out.println(helloString); } } //hello.
!
The String class is immutable so that once it is created a String object cannot be changed.
What is Immutable?
- Immutable means unmodifiable or unchangeable.
- Once string object is created, its data or state can't be changed but a new string object is created.
public class testImmutableString { public static void main(String args[]) { String s = "salam "; s.concat("Java"); //concat() method appends the string at the end System.out.println(s); } } //will print Sachin because strings are immutable objects.
Immutable
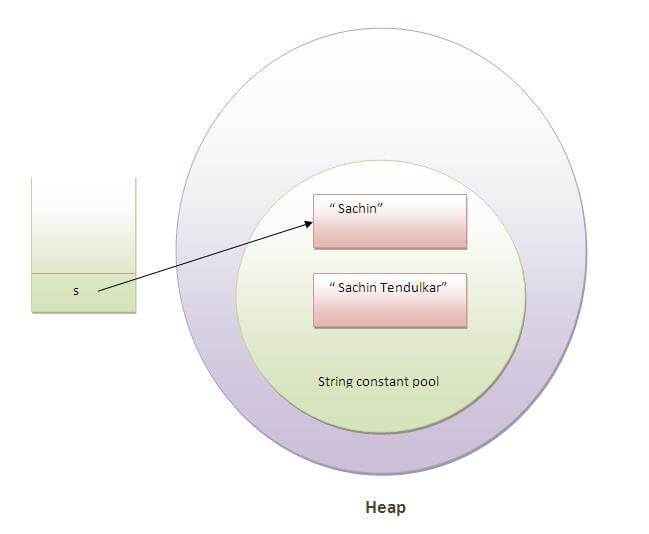
Format Strings
- Methods: .printf(), .format()
System.out.printf("The value of the float variable is " + "%f, while the value of the integer " + "variable is %d, and the string " + "is %s", floatVar, intVar, stringVar);
String fs; fs = String.format("The value of the float variable is " + "%f, while the value of the integer " + "variable is %d, and the string " + "is %s", floatVar, intVar, stringVar); System.out.println(fs);
Characters in Java
- Unicode
- Problems in ASCII.
- The Unicode standard is a character set.
- Characters are saved as numbers.
- What is encoding?
- UTF-8, UTF-16, UTF-32
- The default for Java is UTF-16.
Escape characters
- New line: "\n"
- Tab: "\t"
- ": "\""
- ': "\'"
- \: "\\"
String S = "Salam\\ Ok\' Bye\""; System.out.println(s); //Salam\ Ok ' Bye"
Let's see the METHODS.
Visit:
https://docs.oracle.com/en
for EVERYTHING...!
Java Multithreading
By Parsa Hejabi
Java Multithreading
This slide was used to teach Multithreading in Java for AP students of SBU CE faculty in Spring 2019.
- 455