Groovy for Java Developers
Agenda
(1) What is Groovy
(2) Arrays in Groovy
(3) Default imports for groovy
(4) Groovy Truth
(5) Equals in Groovy
(6) Optionals
(7) Everything is Object
(8) Strings
(9) Groovy bean
(10) Additional Operator
(11) Switch Statement
(12) Dynamic Method Dispatch
(13) Collections
(14) lambdas vs closures
What is Groovy ?
Groovy is feature-rich Java-friendly dynamic language for the Java Platform
What is a dynamic language ?
A language that allows the types of variables to be changed at runtime
def a=1
println a
a="str"
println a
Groovy is:
- A super version of java
- Designed as a companion language of java
- Seamless integration with java
- Syntatically aligned with java
- Compiles into JVM bytecode
Awesome Groovy based tools and frameworks
- Grails: Web development framework
- Gradle: Build Automation tool
- Spock: Testing franework
- CodeNarc: Static Analysis tool
- Easyb: Behavior driven development framework
- Geb: browser automation tool
- Griffon: desktop app framework
- Gpars: Concurrency framework
Different ways of running groovy code
- groovyc : Compiles groovy source to jvm class files
- groovy : executes groovy script
- groovyConsole: GUI for interactive code execution
- groovy interpreter : very handy to run groovy code from command linee.g groovy -e "println 'hello'"
Groovy Scripts
- No Mandatory class definitions, no main method, no boilerplate code
- Groovy creates them for you automatically
- args passed at runtime for a groovy file are accessible in args variable
Arrays in groovy
def testArray = new String[3]
testArray[0] = "A"
testArray[1] = "B"
testArray[2] = "C"
String[] testArray = ["A", "B", "C"]
def testArray = ["A", "B", "C"] as String[]
def testArray = ["A", "B", "C"].toArray()
def testArray = ["A", "B", "C"].toArray(new String[0])
Default imports for groovy
- java.lang.*
- java.util.*
- java.net.*
- groovy.lang.*
- groovy.util.*
- java.math.BigInteger
- java.math.BigDecimal
Groovy Truth
- Null value in false
- Empty Collection or map is false
- Empty String is false
- Zero value is false
Equals in groovy
- == operator checks for value equality (like equals in java)
- Use method is for identity
String str = new String("str1")
String str2 = new String("str1")
println str.is(str2)
// false
String str = new String("str1")
String str2 = new String("str1")
println str==str2
//true
Optionals
- Semicolons
- Variable types
- Return Statements
- Parentheses
println fun(2)
Integer fun(var){
var *2
}
Everything is Object in groovy
- All Objects are derived from groovy.lang.GroovyObject which extends java.lang.Object.
- All integral numbers are java.lang.Integers.
- All real numbers are java.lang.BigDecimals.
- All booleans are java.lang.Booleans.
println (true.getClass())
println (1.getClass())
println (1.0.getClass())
/*
class java.lang.Boolean
class java.lang.Integer
class java.math.BigDecimal
*/
Strings
- Single Quotes : Ordinary String
- Double Quotes : Ordinary String with variable expansion
- Triple Quotes : Multiline String with variable expansion
println ('hello world')
def a=1
println ("hello world ${a}")
println("""Hello world
${a}""")
Groovy classes/beans
- Methods and classes are public by default.
- Members are private by default.
- Dynamic constructor arguments
- Getters and Setters are generated automatically.
- You can use this keyword inside static method.
Additional Operators in groovy
- Safe Navigation : x?.method()
- Elvis : x= y ?:false
- ["groovy","java"]*.size()
Integer i=null
println(i?.intValue())
def y=0
println y?:"false"
println (["java","groovy"]*.size())
Switch Statement
def a="abc"
switch (a){
case "abc":
println "String"
break
case 1:
println "Integer"
break
case 1..2:
println "Range"
break
case [3,4]:
println "List"
break
}
Dynamic method dispatch
- Java dispatches method according to the type of argument at compile time.
- Groovy dispatch method according to the type of the argument at runtime.
public void foo(String str){ println "String"}
public void foo(Object obj){ println "obj"}
java.lang.Object object="Hello"
foo(object)
// Java : Object
// Groovy : String
Closure
Closure are anonymous functions that:
- May accept parameter or return value
- Can be assigned to variables
- Can be passed as arguments
- capture the variable of their surrounding.
void myOperation(int a,int b,Closure closure){
closure(a,b);
}
Closure sum ={a,b-> println a+b}
Closure sub ={a,b-> println a-b}
myOperation(1,2,sub)
Lambda Expressions
- Lambda expressions are implemented using functional interfaces.
- Variables used within lambda expressions must be final or effectively final.
List
// Creating List
List list = [1,2,3,4,5]
// Sum of elements
println list.sum()
// Max among elements
println list.max()
// Min among elements
println list.min()
// Second last element in List
println list[-2]
list = list + [23]
println list
list = list - [23]
println list
list = list << 11
println list
println ([1,2,3,[44,55]].flatten())
println ([1,1,2,3,3,2].unique())
Few more operations with list
Groovy and Java list operations
Iterating a list
// Java
list.forEach(e-> System.out.println(e));
// Groovy
list.each({e-> println (e)})
Modifying a list
// Java
System.out.println(
list.stream().map(e->e*2).collect(Collectors.toList())
);
// Groovy
println list.collect({e->e*2})
Filtering elements
// Java
System.out.println(
list.stream().filter(e->e>2).collect(Collectors.toList())
);
// Groovy
println list.findAll({e->e>2})
Creating a Map
// Creating Map
def map = [1:"One", "2":"Two", 3:"Three"]
// Iterate Map
map.each{ k,v->
println k
println v
}
// Finding size of Map
println map.size()
// Accessing Map values
println map.get(1)
println map."2"
// Check the key
println map.containsKey(1)
// Check the value
println map.containsValue("Two")
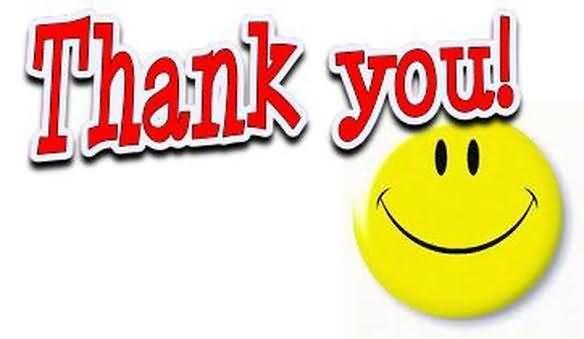
Groovy for Java Developers
By Pulkit Pushkarna
Groovy for Java Developers
- 1,137