Welcome again!
The Codeclub, SMVDU
- if-else conditions
- loops
- while
- for
- do-while
- Arrays
- Strings
2-D Array or Matrix
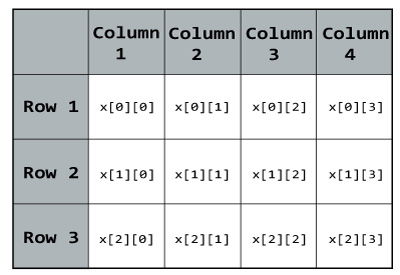
// taking 2-D array as input
for(i = 0; i < 5; i++)
{
for(j = 0; j < 5; j++)
{
scanf("%d",&p);
a[i][j] = p;
}
}
//same for output
for(i = 0; i < 5; i++)
{
for(j = 0; j < 5; j++)
{
p = a[i][j]
printf("%d",p);
}
}
Take a matrix of 3x3 as input and print the sum of every column individually.
Pointers
which stores the address for a value.
* = value at address
& = address of
How to declare a pointer?
int * x;
char * y;
Function
Function
or you can say a reusable code
Find half max/min/max/min/max/min in a list of 100 values.
A function can return something or nothing.
- a function which doesn't return anything is called a void function
- a function which returns something is called a non-void function.
void function( ){
}
void function( int a ){
}
int function( ){
int a;
return a; }
int function(int a){
int b;
return b; }
int sumIt(int a, int b)
{
int c;
c = a + b;
return c;
}
int main()
{
int ans;
ans = sumIt(45,2);
printf("%d",ans);
return 0;
}
Questions
1. Print the GCD of two given numbers using a function.
3. Take a number as an input from the user and check it is prime or not using a function.
4.Print all the prime numbers in an interval of two numbers take as input from the user, using a function.
2. Swap two numbers take input from the user, using a function.
deck
By sandeep chauhan
deck
- 761