
Welcome to JavaScript & jQuery
My name is Trevor,
and my TA is Michael, Mike, Bridget and Janelle
about me
I'm an entrepreneur.
I love to solve problems.
What are we doing today
JavaScript Refresher
Creating with jQuery and javaScript
How to Learn All the Things
Rule #1
Buzz words are bad!
Take scrap paper. Crumple it into a ball. If I use a word you don’t know, chuck it at me.
Rule #2
If you have a question, answer it!
You can ask me, or you can ask the person next to you or you can look it up.
Rule #3
Help Each Other
Coding is a community! That’s one of the best things about it! Everyone helps everyone, even if you are brand new you may see something a different way…
exercise
What are your going to do with this?
What's your project going to be?
JavaScript Refresher
JavaScript
What is JavaScript?
It gives your website a brain!
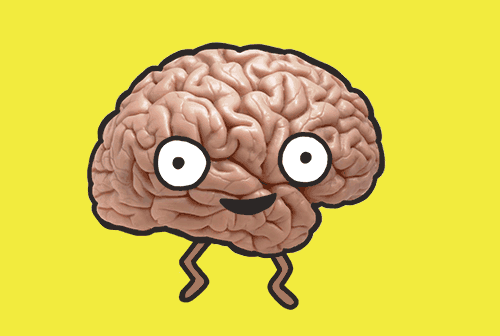
JavaScript
It's a lot of
"This is this, and if this does that, then do this other thing"
How we use JavaScript
Variables, Statements and Functions
Variables
Variables are like a vocabulary that we make up, and that we use to speak to our program.
hey computer, what's my name?
what's a name?
Statements
This is that.
The sky is blue.
We use statements to define variables and talk with our program.
My name is Trevor.
Functions
mini programs
wrap up statements and logic and variables
reusable
Function Example
i'm a robot.
i know four things.
"right foot up"
"right foot down."
"left foot up"
"left foot down"
Function Example
var stepLeft = function (){
left foot up;
left foot down;
};
var stepRight = function (){
right foot up;
right foot down;
};
Function Example
var step = function (){
stepLeft();
stepRight();
};
Part II
jQuery FUN!!
We're going to use
Google Chrome
Text Editor
jQuery
HTML
CSS
if statments
jQuery
It's a JavaScript library that makes life easier.
jQuery
JavaScript is like getting a puppy.

You can train it to do anything you want, but it takes time.
jQuery
jQuery is like getting super well trained dog.
It already knows how to do a ton of stuff!!
Let's Start
We're going to work with three files
index.html
style.css
myScript.js
You can download them here
Add jQuery
To add jQuery we just paste this line in between <head> and </head>
<script src="https://code.jquery.com/jquery-2.1.1.min.js"></script>
Also add some content
<div class="content">
<h1>Welcome to my awesome site!</h1>
</div>
Let's make it pretty
style.css
body {
width: 800px;
margin: 0 auto;
padding-top: 50px;
font-family: Helvetica, "Nimbus Sans L", "Liberation Sans", Arial, sans-serif;
background: whitesmoke;
}
.content {
padding: 20px;
background: white;
box-shadow: 0 1px 3px rgba(0,0,0,.5);
border-radius: 5px;
}
Fun With Console
Open the console, and let's mess around with our title.
Try typing this into the console
$('h1').css('color','red');
Sweet, now give this a go
$('h1').css('font-size','50px');
What is going on?
We are using jQuery to change how our website looks!
Let's break it down
$("h1") is like saying "Hey jQuery, go find anything on the page that has an 'h1' tag, please."
.css("color","red") is saying "Now add some CSS style to all those things you can just got. I want that style to be the color, and make it red, please"
Let's make some interactive buttons!
index.html
<h1>Welcome to my awesome site!</h1>
<div id="redBtn" class="btn">Red</div>
<div id="greenBtn" class="btn">Green</div>
<div id="blueBtn" class="btn">Blue</div>
Let's make 'em pretty!
style.css
.btn {
padding: 10px;
background: rgb(213, 207, 202);
display: inline-block;
border-radius: 4px;
border: 1px solid rgb(165, 165, 165);
box-shadow: 0px 1px 1px 0px rgba(0,0,0,.5);
-webkit-user-select: none;
}
.btn:hover {
background: rgb(200, 189, 133);
cursor: pointer;
}
and lets power them!
jQuery "click" let's us listen for a click event.
When that event happens, we can have some code run!
$("TARGET ELEMENT").click(
function(){
//CODE TO RUN HERE
});
function(){
//CODE TO RUN HERE
});
Code for our buttons
myscript.js
$("#redBtn").click(
function() {
$("h1").css("color","red");
console.log("test");
}
);
$("#blueBtn").click(
function() {
$("h1").css("color","blue");
}
);
$("#greenBtn").click(
function() {
$("h1").css("color","green");
}
);
if / then
let's make a toggle button
HTML
<div class="btn lightsOn switch">turn lights off</div>
if / then
We want to write some code that says
"when the button is clicked, check if it's 'on'.
If it's 'on', turn the lights off 'off'.
Otherwise, turn the lights 'on'.
"when the button is clicked, check if it's 'on'.
If it's 'on', turn the lights off 'off'.
Otherwise, turn the lights 'on'.
How do we do this?
What does off and on mean?
What does off and on mean?
jQuery click
$(“.switch”).click( function() {
//check if switch is on
//if on, turn off lights
//otherwise, turn on lights } );
Hint
$(element).hasClass("ClassName") returns true or false
$(element).removeClass("ClassName") removes a class
$(element).addClass("ClassName") adds a class
final code
myscript.js
var turnOff = function(){
$(".switch").removeClass("lightsOn");
$(".switch").addClass("lightsOff");
$(".switch").text("Turn Lights On");
$("body").css("background","black");
};
var turnOn = function(){
$(".switch").removeClass("lightsOff");
$(".switch").addClass("lightsOn");
$(".switch").text("Turn Lights Off");
$("body").css("background","whitesmoke");
};
$(".switch").click(
function() {
if ( $(".switch").hasClass("lightsOn") ) {
turnOff();
console.log("turned off");
} else {
turnOn();
console.log("turned on");
}
});
});
What else can jQuery do?
Fade In and Out Elements with .fadein() & .fadeOut()
Animate Elements with .animate()
Animate Elements with CSS Transitions
Slide Elements in and Out with .slideOut();
Create New Elements with .append()
Hide Elements .hide()
Remove Elements .remove()
Toggle Showing an Element with .toggle()
Trigger an event with the mouse moves, hovers, left click, right click...
PART III
LEARN ALL THE THINGS
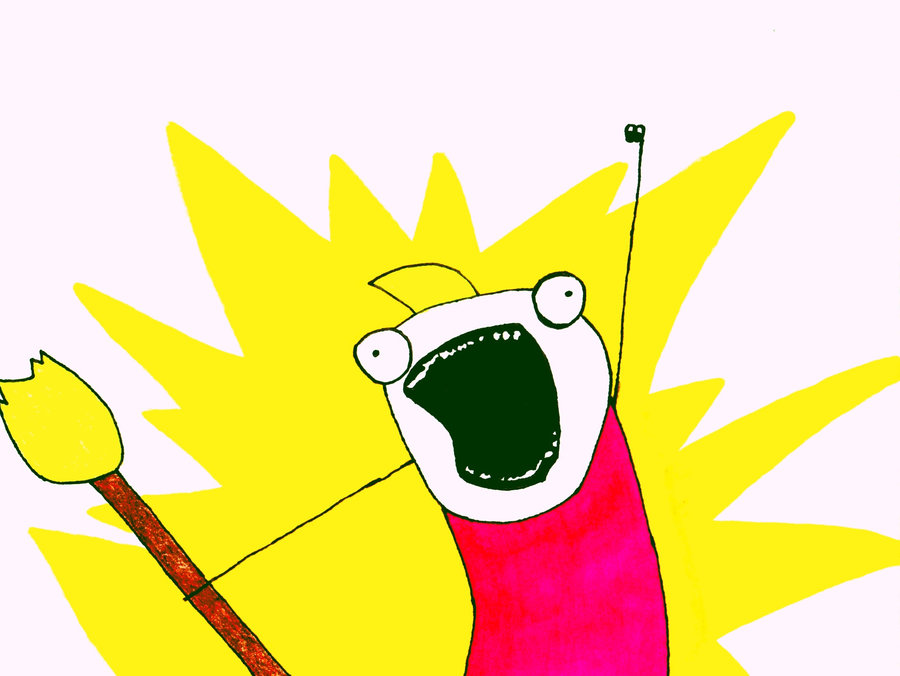
Resources
Codecademy.com
Stackoverflow
jQuery Docs | http://api.jquery.com/
Google | Learn to search for whatever you want to do.
jQuery plugins | Try googling “Awesome jquery plugins”
Me: @trevorgeise or trevorgeise@gmail.com
The future
Javascript is becoming more and more powerful. New technology is making it possible to create entire webapps in nothing but Javascript.
That will be the next class. Creating a Webapp Super Fast in Javascript!
If we have time, lets make something else... ideas?
techgirlz - js & jquery
By trevorgeise
techgirlz - js & jquery
- 839