React.js
Mar 2017 @vekzdran

A VERY SIMPLE 101 GUIDE
...that will persuade your dev team to start using it!
PRESENTATION Agenda
15 min
- how it looks
- what it is? + philosophy
- the data
- the lifecycle
- the virtual DOM
- recap with pros/cons
15 min
- demo & questions
included
- trolling (js), sarcasm and 😀
- one intentional bug!
+
IT MUST BE .JS MAGIC ...
var React = require("react");
var Link = require("react-router").Link;
var SearchBox = require("../components/SearchBox.js");
var Header = React.createClass({
render: function() {
return (
<div className="header">
<Link to="/">
<h1>Weather App</h1>
</Link>
<SearchBox className="searchBoxSmall" />
</div>
);
}
});
// CommonJS module approach
module.exports = Header;
... possibly even worse witchcraft than Angular 2!

Well... IT's just js...
render: function() {
// the <div>
return React.createElement("div", {className: "header"},
[
// the <Link>
React.createElement(Link, {to: "/"},
// the <h1>
React.createElement("h1", null, "Weather App")),
// the <SearchBox>
React.createElement(SearchBox, {className: "searchBoxSmall"}, null)
]
);
}
... syntactic sugar known as JSX
... that transforms to JavaScript!
ECMASCRIPT 6
import React from "React";
import { Link } from "react-router";
import SearchBox from "../components/SearchBox.js";
class Header extends React.Component {
render() {
return (
<div className="header">
<Link to="/">
<h1>Weather App</h1>
</Link>
<SearchBox className="searchBoxSmall" />
</div>
);
}
}
export default Header;
... basically the standard for writing React apps
A jsx TL;DR
JSX is like a healthy vegetable 🥕 that tastes like decadent chocolate 🍰 cake. You feel guilty, but it’s good for you.
Eric Elliot
oh Modern JavaScript ...
... (╯°□°)╯︵ ┻━┻
...HTMLish stuff in .JS(X)?! 😨
return (
<BattleAlert gameId={this.props.gameId}>
<h1>Hello {username}, welcome to: {this.props.gameName}</h1>
<ConfirmBattle
isLoading={this.state.isLoading}
onInitiateBattle={this.handleInitiateBattle} />
</BattleAlert>
);
...AHH, A(nother) {TEMPLATING} ENGINE!
...TON OF STUFF* DECLARED and bound IN 1 FILE!?
please again... W'at is this!? :)
SEPARATION OF CONCERS
DO YOU EVEN?
¯\_(ツ)_/¯
SEPARATION OF...
SELF CONTAINED COMPONENTS
...concerNs are INDEED separated

- It's "just" (a) JavaScript (view library by Facebook)
- It's the V in MVC, but no models & no controllers
- Components are the building blocks
- Plain JS + JSX syntax + Virtual DOM
SO WHAT IS REACT ... ?
... it's like Lego, but with components!
All it does is render stuff efficiently and delegate events.
It knows the data it needs to render.
It knows when to render it (when the data changes).
It knows how to render it efficiently.
E. Elliot
It's
Just
efficient
rendering
:)
And WHY COMPONENTS ?
...because they are:
- isolated
- composable
- testable
- reusable
- maintainable

UI components example again :)
hmm... NO MODELS, BUT WHERE's DATA?
Traditional 2-way bindings, nope- Data flows in 1-way
- Parent sends to Child(ren)
- Props and State...

-bind="text: starDate"
Props PASSES DATA* down...
...to child components
import PrettyPrint from "./PrettyPrint";
var Writer = React.createClass({
render: function () {
return (
<PrettyPrint text="Foo... Bar!" id={this.props.id} />
);
}
});
var PrettyPrint = React.createClass({
render: function() {
return (
<p id={this.props.id} className="aPrettyParagraph">
{this.props.text}
</p>
);
}
});
this.props is immutable
*also means functions, i.e. events
#REMINDER #neverforget
...JSX is just a bunch of .js nested React.createElement() calls
STATE IS MUTABLE
It's optional
var PrintRandomNumber = React.createClass({
setRandomNumber: function() {
// changing state will fire render()
this.setState({ number: Math.random() });
}
render: function () {
return <PrettyPrint text="{this.state.number}" />;
}
});
// This a Stateless Functional Component
// They are pure functional transforms of their input, with zero boilerplate.
var ICanOnlyRenderWithProps = function(props) {
return (
<p id={props.id} className="statlessPrettyParagraph">
{this.props.text}
</p>
);
};
mutating state will fire render() and all child render() methods
DID you notice the bug? 🐛
TAKE CARE HOW YOU PASS (which) value to props...
var PrintRandomNumber = React.createClass({
setRandomNumber: function() {
// changing state will fire render()
this.setState({ number: Math.random() });
}
render: function () {
return <PrettyPrint text="{this.state.number}" />;
}
});
component life
MOUNT -> update -> UNMOUNT
Mounting (component mounts DOM):
Updating (state mutates or new props come):
Unmounting:
getDefaultProps() -> getInitialState() -> componentWillMount()
render() -> children component(s) lifecycle
componentDidMount()
componentWillReceiveProps() -> shouldComponentUpdate() - >componentWillUpdate()
render() -> children component(s) lifecycle
componentDidUpdate()
componentWillUnmount() -> children component(s) lifecycle
Instances destroyed for GC


virtual dom
to solve the problems of the original dom
- a HTML DOM abstraction
- light and deattached from browser specif.
- does not deal with drawing stuff and UI
- React updates vDOM
- vDOM updates DOM only for diffs!
- efficient thanks to good diff algos
- a single top level event listener
Don't you know HTML dom is ALSO an abstraction ? 💥
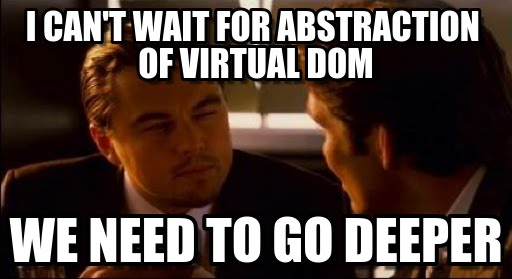
It's so complex and cool - South Park s14e10
But what's the matter with THE normal dom?
- When it grows... 🔥 traversing it becomes very slow
- SPAs these days, a ton of divs...
- abusing $(".getMeThisNode"), selects all the nodes
- a ton of event listeners, try finding & editing one
- data-mySuperDataInDom* !?
HTML DOm bad keypoints
- hard to manage
- inefficient
that React + vDOM solve
LEt's RECAP
PRO
CONTRA
- declarative (JSX)
- it is plain js
- no framework specific magic
- just the V of MVC
- Separation of C/SCC
- efficient vDOM
- functional style is a +
- easy to learn
-
React + Redux: 150kB
-
server rendering
-
pluggable on any page
- will not play nice with other existing libs like DOM and $
- optimize rerendering
- store state management...
- a dev philosophical shift

Apples vs Orangutans? :)
THX & DEMO TIME!
react-dead-simple-guide
By Vedran Mandić
react-dead-simple-guide
This is a short and fun presentation about React.js from Facebook that is used for building powerful web UIs.
- 1,935