ESP32 Server
Introduction to MicroPython Socket Module
- Purpose: Network communication in MicroPython.
- Comparison with CPython socket module.
- Basic socket operations: create, bind, listen, accept connections.
Socket Creation and Address Binding
-
socket.socket()
: Creating a new socket.
-
socket.bind(address)
: Binding the socket to an address.
- Address formats: IPv4, IPv6, raw addresses.
Listening and Accepting Connections
-
socket.listen([backlog])
: Prepares socket to accept connections.
-
socket.accept()
: Accepts a new connection.
- Importance of managing backlog in connections.
Sending and Receiving Data
-
socket.send(bytes)
: Sends data over the socket.
-
socket.recv(bufsize)
: Receives data from the socket.
- Data handling and buffer management.
Closing Sockets and Resource Management
-
socket.close()
: Closes the socket.
- Importance of releasing resources to prevent leaks.
- Exception handling for socket operations.
Example - Creating and Binding a Socket
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('localhost', 80))
Example - Listening and Accepting Connections
s.listen(5)
conn, addr = s.accept()
Example - Sending and Receiving Data
data = conn.recv(1024)
conn.send(data)
Socket Options and Advanced Features
- Setting and getting socket options.
- Non-blocking sockets and timeouts.
- Advanced socket functionalities.
Conclusion and Further Reading
- Recap of MicroPython socket capabilities.
- Encouragement to experiment with socket programming.
- Reference: MicroPython socket documentation.
import network, socket, time
from machine import Pin
wlan = network.WLAN(network.AP_IF) # Initializes the access point interface
wlan.active(False) # Deactivates the first AP interface
time.sleep(0.1) # Waits for 0.1 seconds to ensure the interface is properly deactivated
wlan.active(True) # Reactivates the first AP interface
wlan.config(ssid='TedESP32AP') # YourAP Name
wlan_ip = wlan.ifconfig()[0]
print('AP IP:', wlan_ip) # Displays the IP address of the ESP32 in AP mode
led=Pin(16,Pin.OUT)
led.value(0)
TCP Server Example - Control LED
def setup_server(wlan_ip):
# Create STREAM TCP socket
server=socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server.bind((wlan_ip, 80))
server.listen(1)
print("tcp server is listening")
tcp_conn, client_addr = server.accept()
print(client_addr, "Client connects sucessfully")
return tcp_conn
TCP Server Example - Control LED
def server_task(tcp_conn):
count = 0
while True:
msg = tcp_conn.recv(128).decode("utf-8").lower()
if "on" in msg:
led.value(1)
elif "off" in msg:
led.value(0)
elif "exit" in msg:
break
print(f"receive {count} message {msg}")
if count > 5:
break
count += 1
tcp_conn.close()
server.close()
if wlan_ip is not None:
tcp_conn = setup_server(wlan_ip)
server_task(tcp_conn)
TCP Server Example - Control LED
Test App
IOS

UDP/TCP/REST Network Utility
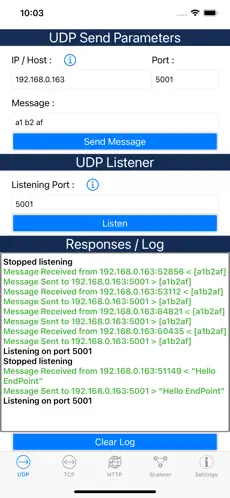
UDP/TCP/REST Network Utility
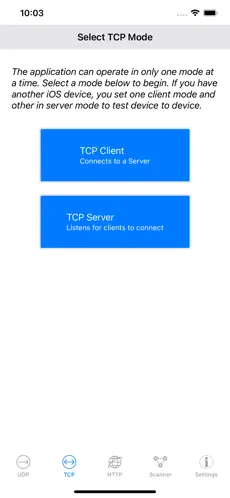
UDP/TCP/REST Network Utility
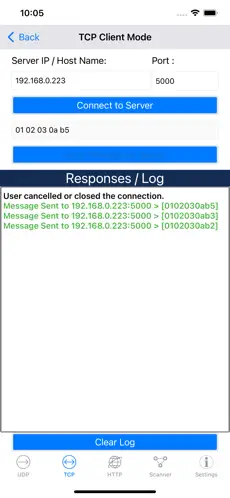
Adroid

Adroid TCP Client
Adroid TCP Client
ESP32 HTTP Server
reference
Online HTML Editor
Web Server code

ESP32 HTTP Server
import network, socket, time
from machine import Pin
wlan = network.WLAN(network.AP_IF) # Initializes the access point interface
wlan.active(False) # Deactivates the first AP interface
time.sleep(0.1) # Waits for 0.1 seconds to ensure the interface is properly deactivated
wlan.active(True) # Reactivates the first AP interface
wlan.config(ssid='TedESP32AP') # YourAP Name
wlan_ip = wlan.ifconfig()[0]
print('AP IP:', wlan_ip) # Displays the IP address of the ESP32 in AP mode
led=Pin(16,Pin.OUT)
led.value(0)
ESP32 HTTP Server
def setup_server(wlan_ip):
# Create STREAM TCP socket
server=socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
server.bind((wlan_ip, 80))
server.listen(1)
print("HTTP Server is listening")
return server
ESP32 HTTP Server
def server_task(server):
while True:
conn, client_addr = server.accept()
print(client_addr, "Client connects sucessfully")
msg = conn.recv(1024).decode("utf-8").lower()
if "get /?led=on" in msg:
led.value(1)
elif "get /?led=off" in msg:
led.value(0)
else:
pass
print(f"receive message {msg}")
response = web_page()
conn.send('HTTP/1.1 200 OK\n')
conn.send('Content-Type: text/html\n')
conn.send('Connection: close\n\n')
conn.sendall(response)
conn.close()
server.close()
ESP32 HTTP Server
def web_page():
if led.value() == 1:
gpio_state="ON"
else:
gpio_state="OFF"
html = """<html>
<head>
<title>ESP Web Server</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" href="data:,">
<style>
html{font-family: Helvetica; display:inline-block; margin: 0px auto; text-align: center;}
h1{color: #0F3376; padding: 2vh;}
p{font-size: 1.5rem;}
.button{display: inline-block; background-color: #e7bd3b; border: none;
border-radius: 4px; color: white; padding: 16px 40px;
text-decoration: none; font-size: 30px; margin: 2px; cursor: pointer;}
.button2{background-color: #4286f4;}
</style>
</head>
<body>
<h1>ESP Web Server</h1>
<p>GPIO state: <strong>""" + gpio_state + """</strong></p>
<p><a href="/?led=on"><button class="button">ON</button></a></p>
<p><a href="/?led=off"><button class="button button2">OFF</button></a></p>
</body>
</html>"""
return html
ESP32 HTTP Server
if wlan_ip is not None:
server = setup_server(wlan_ip)
server_task(server)
ESP32 Server
By wschen
ESP32 Server
This presentation introduces the ESP32 Server and its features, such as Socket Creation, Sending and Receiving Data, and Socket Options. It also includes examples and further reading materials. Explore how to control LED using TCP Server Example and learn about the ESP32 HTTP Server.
- 179