RPC is not dead
RPC is not dead
It's just a niche now
What is a RPC?
Remote Procedure Call (RPC) is a protocol that one program can use to request a service from a program located in another computer on a network without having to understand the network's details. A procedure call is also sometimes known as a function call or a subroutine call.
When making a Remote Procedure Call:

Thrift
gRPC
[Apache] Thrift
language-agnostic
code generation
for P2P RPC
thrift --gen <language, like cpp> <filename>.thrift
filename.thrift
service Calculator extends shared.SharedService {
/**
* A method definition looks like C code. It has a return type, arguments,
* and optionally a list of exceptions that it may throw. Note that argument
* lists and exception lists are specified using the exact same syntax as
* field lists in struct or exception definitions.
*/
void ping(),
i32 add(1:i32 num1, 2:i32 num2)
}
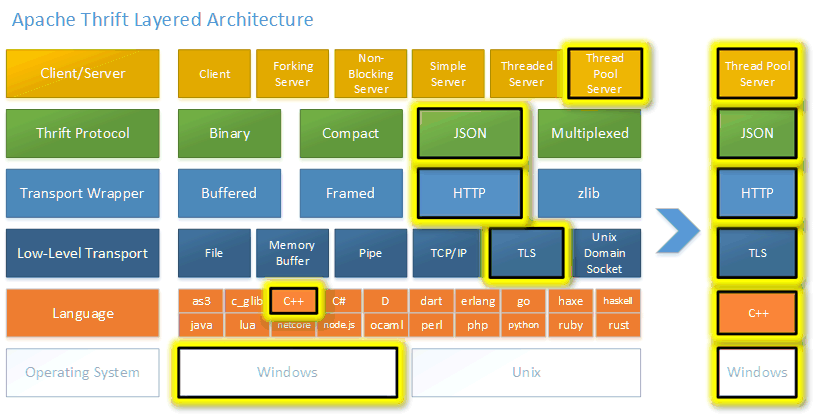
[not Apache] gRPC

Overview
In gRPC a client application can directly call methods on a server application on a different machine as if it was a local object, making it easier for you to create distributed applications and services.
Protocol Buffers
Protocol buffers are a flexible, efficient, automated mechanism for serializing structured data – think XML, but smaller, faster, and simpler.

In order to have everything up and running do:
- Define a service in a .proto file.
- Generate server and client code using the protocol buffer compiler.
- Use the C++ gRPC API to write a simple client and server for your service.
Define your service and its' rpc methods, specifying their request and response types
service RouteGuide {
...
}
Defining the service
* A simple rpc method
* A server-side streaming rpc
* A client-side streaming rpc
* A bidirectional streaming rpc
Create the server
class RouteGuideImpl final : public RouteGuide::Service {
...
}
Starting the server
void RunServer(const std::string& db_path) {
std::string server_address("0.0.0.0:50051");
RouteGuideImpl service(db_path);
ServerBuilder builder;
builder.AddListeningPort(server_address, grpc::InsecureServerCredentials());
builder.RegisterService(&service);
std::unique_ptr<Server> server(builder.BuildAndStart());
std::cout << "Server listening on " << server_address << std::endl;
server->Wait();
}
Create a client stub
grpc::CreateChannel("localhost:50051", grpc::InsecureChannelCredentials());
public:
RouteGuideClient(std::shared_ptr<ChannelInterface> channel,
const std::string& db)
: stub_(RouteGuide::NewStub(channel)) {
...
}
Thank you
Questions?
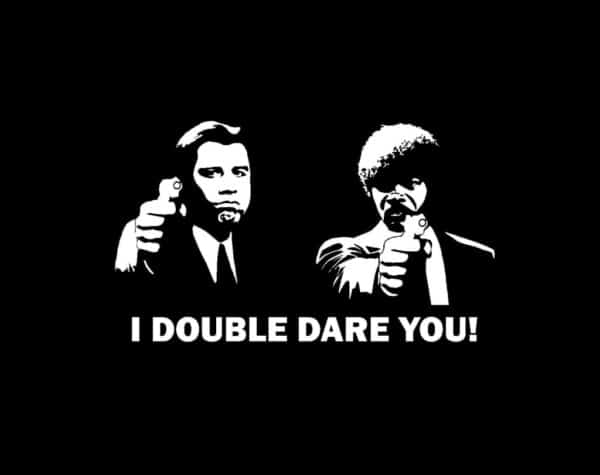
RPC is not dead
By Alexandru Burlacu
RPC is not dead
- 259