WEB
Performance TUNING
- Overview -
@vincent_lcy
Agenda
Basic Concepts of Web Performance
Intro to Chrome Profiling
Some Hands on JS Perf Best Practices
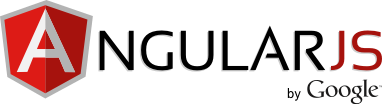
premature optimization is the root of all evil.
Longer Version:
Programmers waste enormous amounts of time thinking about, or worrying about, the speed of noncritical parts of their programs, and these attempts at efficiency are actually BAD CODE.
We should forget about small efficiencies, ~97% of the time.
Yet we should not pass up our opportunities in that critical 3%."
We should forget about small efficiencies, ~97% of the time.
Yet we should not pass up our opportunities in that critical 3%."
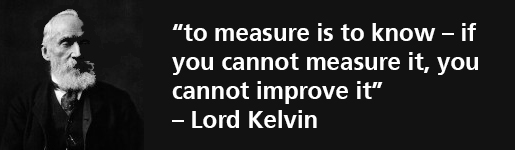
Tune The bottleneck
VERY UNLIKELY
SLOW FOR LOOP
SLOW ALGO
TOO MUCH OBJECTS
Web Performance
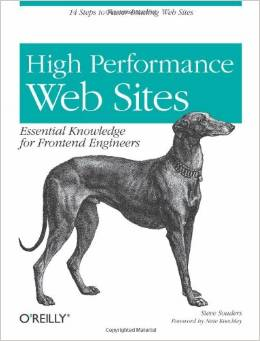
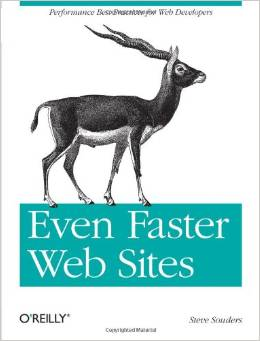
Let's break it down
UI
Load Time
UI Responsiveness
Avoid Memory Leak
Load TIME
E.g. Page Load, Up/Download IO
Network Stuff
GZip, Streaming
Bulk Request
concat, minified etc....
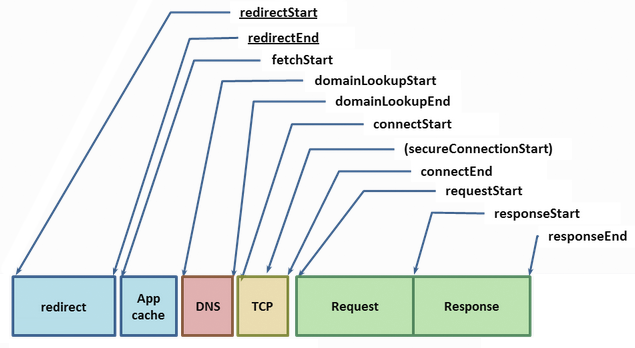
Fastest Request is a Request not made
UI Responsiveness
Basic concepts
How to measure
What to avoid
HOW FAST IS FAST
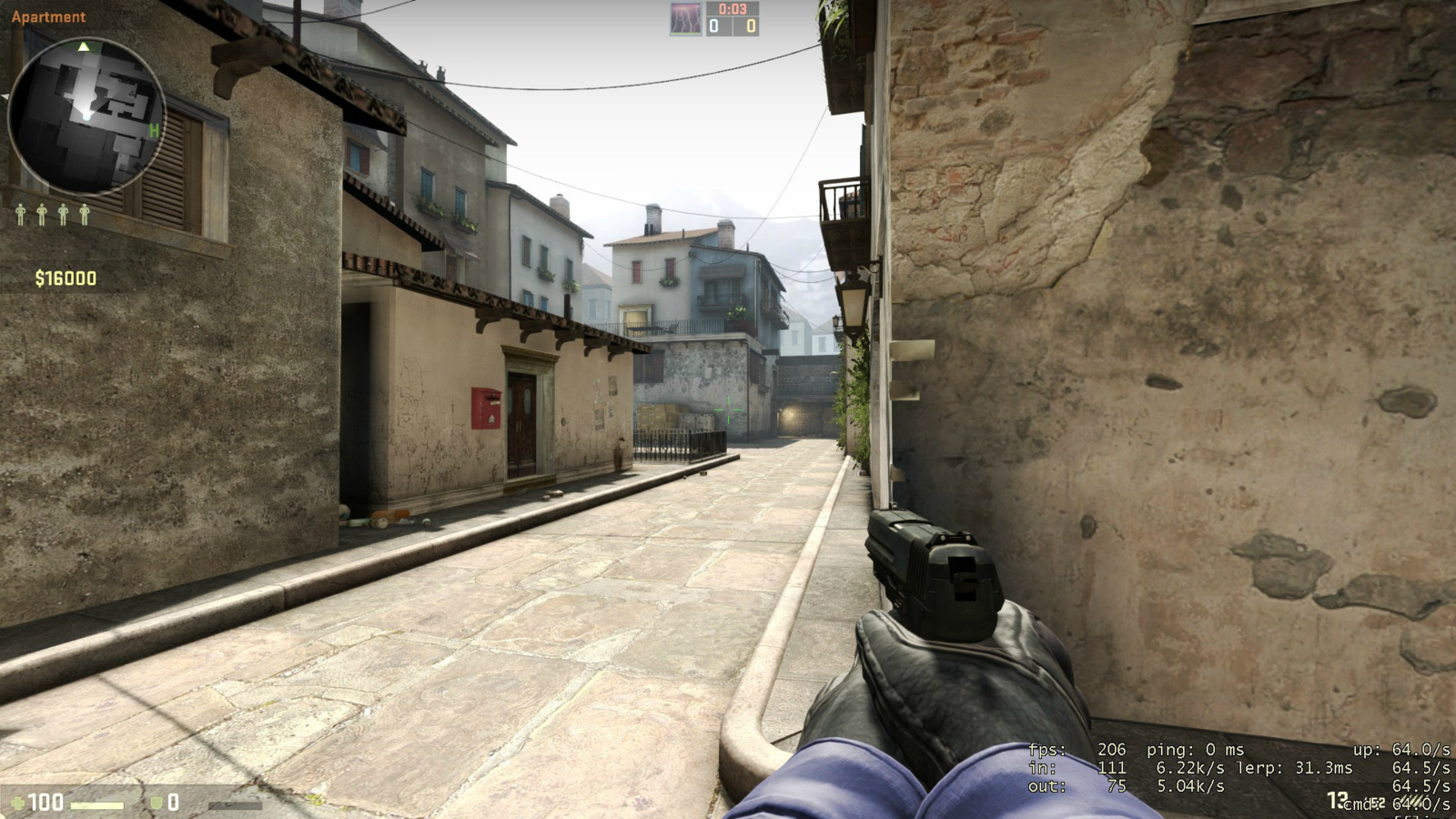
Magic Number: 30 & 60fps
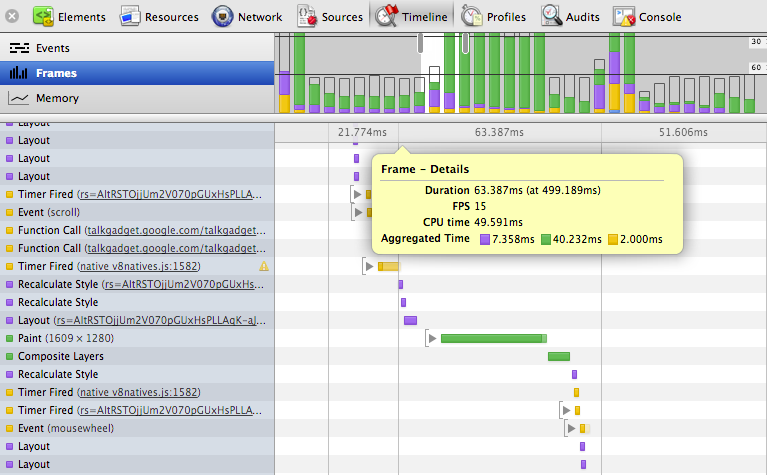
Bad
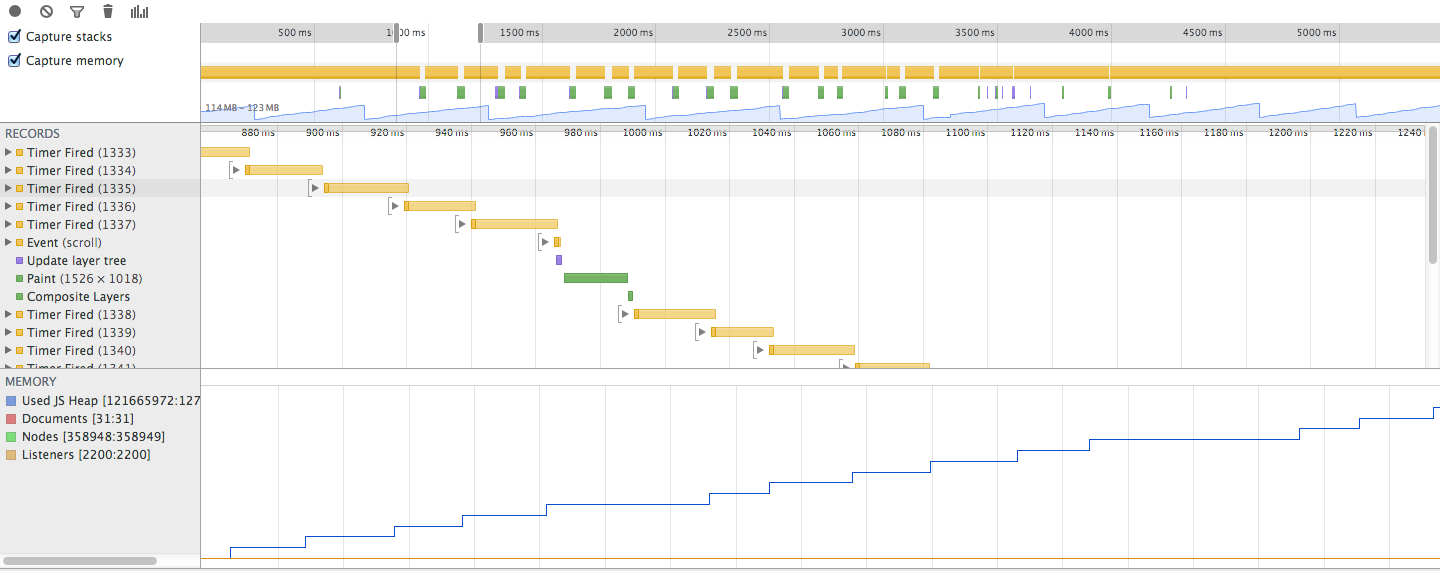
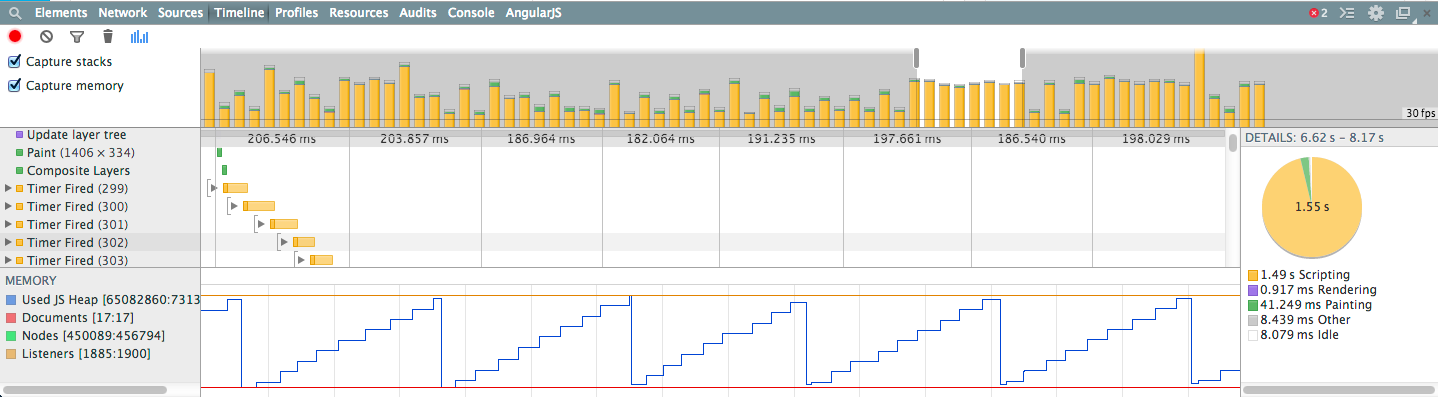
GOOD
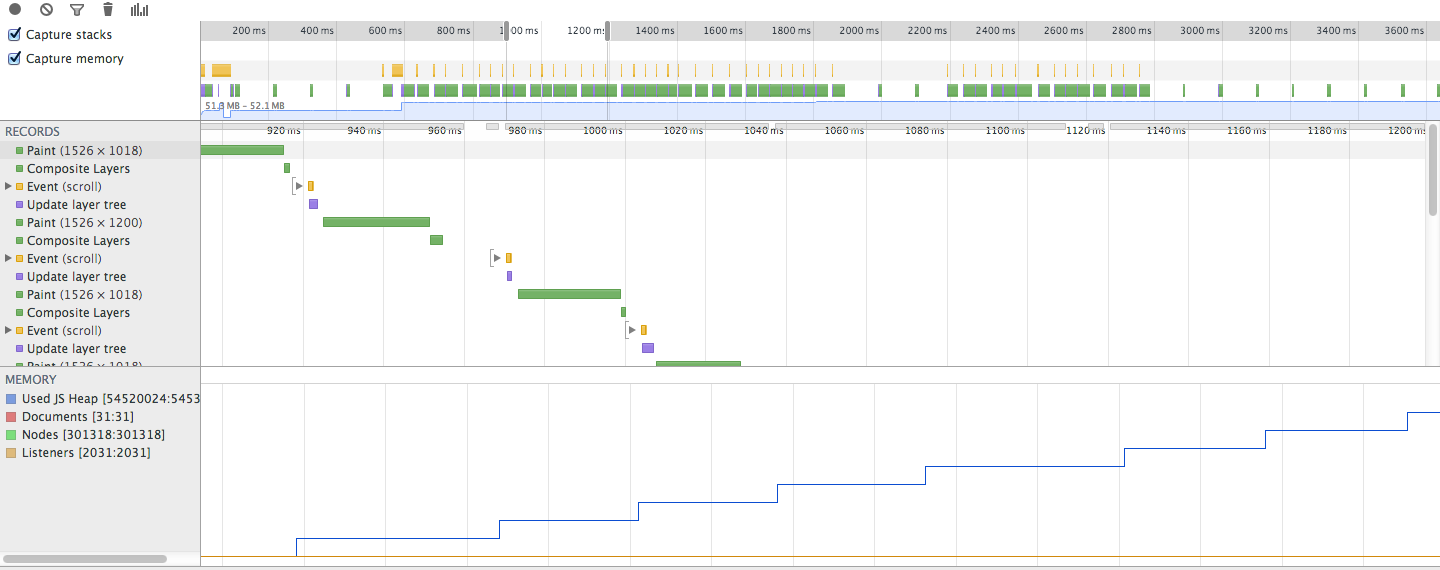
Your FRIENDs
CHROME DEV TOOLS (Canary preferred)
Dev TOOLS DEMO
- View Expressions
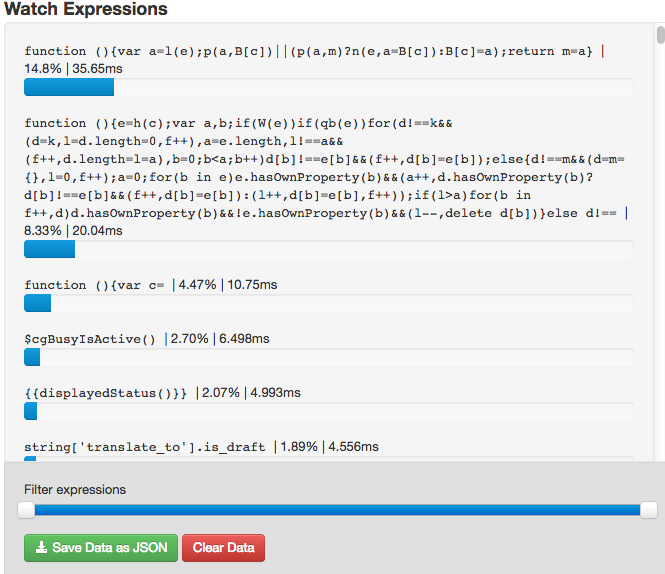
Basic idea
of angular watchers
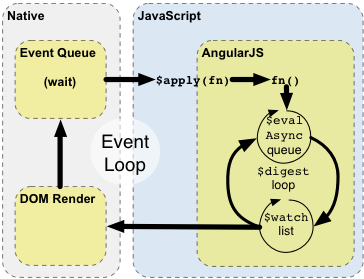
Experience 1
Unresponsive UI
1. Too many Watchers / Slow Expression
HOW MUCH WATCHERS ARE TOO MUCH?
Rule of Thumb: >2000 *basic* watchers
More Often: Some Watchers are really slow
a "bug" $root.$braodcast a resize event
which all elements receive & try to resize
Really need to watch?
Bindonce
Experience 2
Unresponsive UI
2. Logical Error Causing Extra Stuff
Extra Request
Extra DOM Rendered
Extra Memory Used
Experience 3
Turn out a hot-key library
which create watchers inside each DOM in ng-repeat
but actually it bind to global configuration
WORST of both worlds
BASICS: Practical TIPS
-
Prevent inline method() expression [JS Fiddle]
- Avoid filters expression
- Push Logic to controller, outside $scope
- Check Network Log
- Check Controller initialization
BASICS:
VIEW MODEL vs Controller
VIEW MODEL Should only handle STUFF going to DISPLAY
Rendering Long List
Best way to speed up render long list
is render less
filter results before render is better than render & hide
- Js process less
- less nodes in DOM
- less implicit watchers
be reminded...
FILTER runs EVERYTIME
separate Data and displayedData
$watch vs $watchCollection
Even no change it hurts
http://jsperf.com/angularjs-watchcollection-on-large-dataset
tested on angular 1.2.15
AnGULAR:
Things to avoid
ngMouseEnter / ngMouseOver / ngMouseLeave
http://jsfiddle.net/czerwin/5qFzg/4/
MEMORY LEAK
Advanced Topic
Need to know how Engine internals works
But some simple tips to follow
Javascript:
Memory 'automatically' free'd when not 'need anymore'
Reference Counting GC? Not quite
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Memory_Management
"an object is unreachable"
1. has a full list of objects
2. Start at root (*the global object*)
3. Objects not reach are unreachable
=>Objects w/ 0 Reference must be unreachable
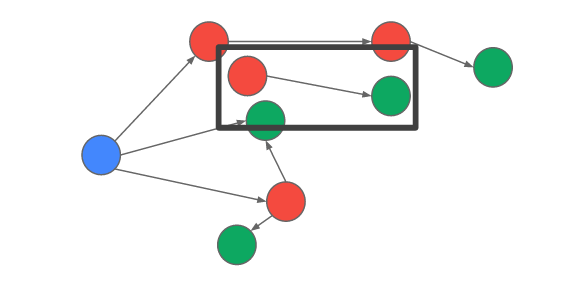
Single PAGE APP
HARDER
Signs of MEMORY LEAK
- FLASHING SCREEN
- Slower & Slower With Use (Not just slow)
- Browser freeze in some particular use case
MEMORY LEAK PATTERNS
jQuery LEAK EXAMPLES
var domObjects = $(".myClass");
domObjects.click(function(){
domObjects.addClass(".myOtherClass");
});
}
http://stackoverflow.com/questions/5046016/jquery-memory-leak-patterns-and-causesfunction run(){
1. Use .off() to remove listeners
2. Don't add reference from the beginning
function run(){
$(".myClass").click(function(){
$(this).addClass(".myOtherClass");
});
}
AngularJS Example
LIVE DEMO ON MEMORY LEAK
Tips to Identify Mem LEAK
- Use Incognito mode (=>no extensions)
- Don't use minified libraries during analysis
-
Use both breakpoint & console log
->stacktrace, print the closure - Check List of DOM Listerens
- Use Heap Snapshot to see any detached DOM tree
WEB Performance TUNING - Overview - @vincent_lcy
AngularJS Perf
By Chun Yin Vincent Lau
AngularJS Perf
- 2,427