JavaScript Next
Web Programming Course
SUT • Fall 2018
TOC
-
Arrow functions
-
let
,const
vsvar
-
Default parameters
-
Spread, rest operator
-
Template literals
-
...
ECMAScript 2015+
-
The Idea
-
Backbone
-
Knockout
-
Ember
-
AngularJS
-
React
-
Angular
-
Vue
-
Progressive Web Apps
Web Applications
-
Package Managers
-
Polyfills
-
Transpilers
Tooling
-
Task Runners
-
Bundlers
ES 2015+
-
let
,const
vsvar
-
Arrow Functions
-
for…of
-
Default parameters
-
Spread, rest operator
-
Template literals
-
Enhanced object literal
-
Deconstructing
What's New
- Modules
- Class
- Iterators & Generators
- Symbols
- Map & WeakMap, Set & WeakSet
- ...
ES 2015+
let
, const
let a = 50;
const b = 100;
if (true) {
let a = 60;
var c = 10;
console.log(a/c); // 6
console.log(b/c); // 10
}
console.log(c); // 10
console.log(a); // 50
b = 50 // TypeError: Assignment to constant variable.
Arrow Functions & Template Literals
const greetings = name => `hello ${name}`;
Examples
ES 2015+
import http from './http';
export default class User {
constructor({ name, id }) {
this.data = {
name,
id,
info: {}
}
this.getUserInfo(id)
}
getUserInfo(id = 0) {
return http.get(`/api/users/${id}`)
.then(data => data.json())
.then(data => {
this.data.info = data;
})
.catch(() => {})
}
}
Examples
ES 2015+
References
Tooling
Polyfills
Transpilers
Task Runners
Bundlers
Package Managers
Tooling

Task Runners
Tooling
Bundlers

Web Apps
Frameworks/Libraries
The Idea?




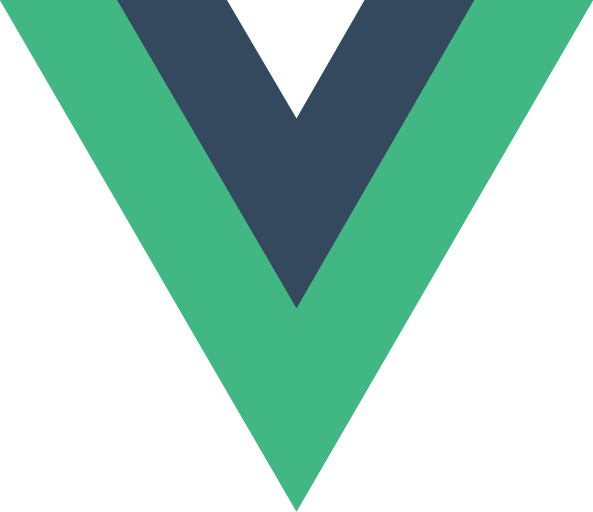
Web Apps
Examples - React
Web Apps
Examples - Vue
Web Apps
Examples
Progressive Web Apps
- Progressive - Work for every user/browser.
- Responsive - Desktop, mobile, tablet.
- Network independent - work offline with Service workers.
- App-like - Feel like an app. App-style interactions & navigation.
- Fresh - Up-to-date with service worker update process.
- Safe - HTTPS to prevent snooping.
- Discoverable - allowing search engines to find them.
- Re-engageable - push notifications.
- Installable - Add to home screen without using the app store.
- Linkable - Easily shared via a URL. No installation.
ES 2015+
Learn More
JavaScript Next
By Hashem Qolami
JavaScript Next
JavaScript Next / Web Programming Course @ SUT, Fall 2018
- 1,389