FUNCTIONAL JAVASCRIPT
WITH RAMDA.JS
const whoami = ctx => name => ({
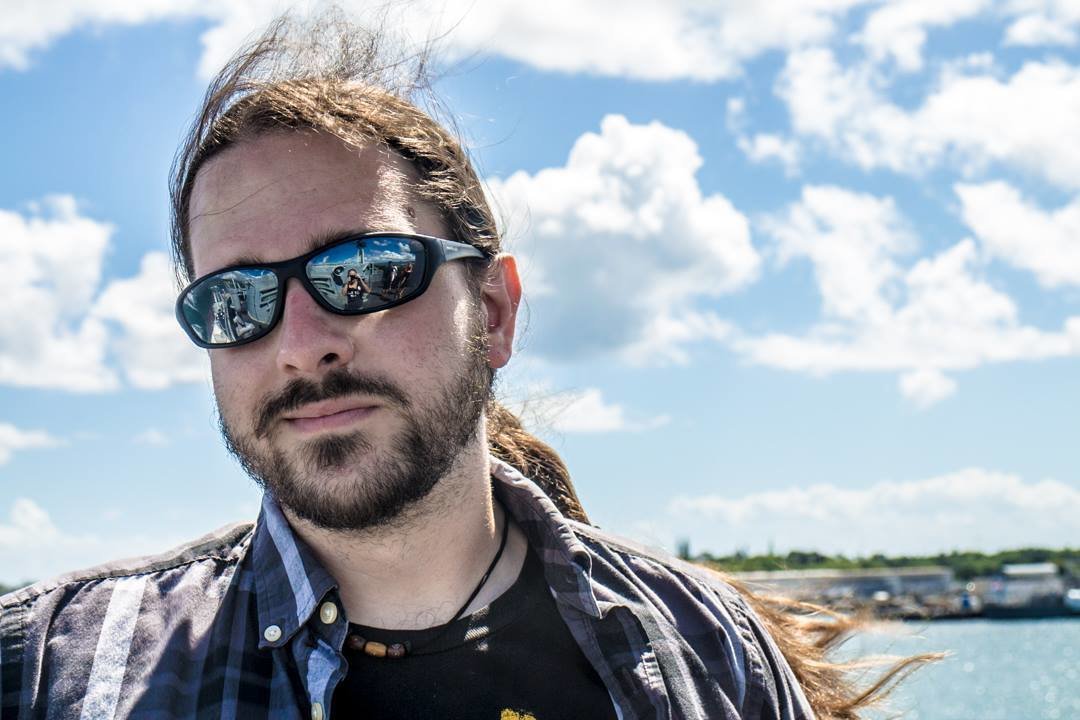
Jett Durham
"Mobile Web Developer" at High Touch Technologies in Wichita, KS
Cross-platform Mobile Apps with React Native
});
WHAT IS FUNCTIONAL PROGRAMMING?
A Programming paradigm
- Functions as values
- Declarative
- Pure Functions
- No Shared State
- No Mutating Data
- No Side-Effects
WHY FUNCTIONAL PROGRAMMING?
functional code becomes
- More predictable
- Safer
- More Modular
- More Testable
- Less Verbose
WHY FUNCTIONAL PROGRAMMING IN JAVASCRIPT?
BECAUSE WE CAN!
QUICK ES6 FEATURE REVIEW
CONST, LET
arrow functions
FUNCTIONAL SOFTWARE CONSTRUCTION
THE MOST IMPORTANT concept
ABSTRACTION
How do we use abstractions?
COMPOSITION
HOW DO WE COMPOSE?
OOP
classes
FP
functions
class-based composition has limits
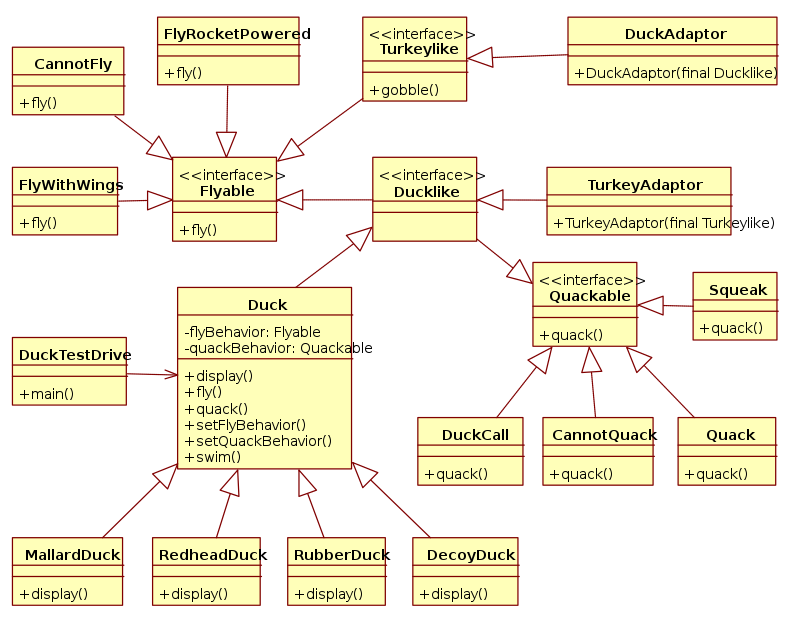
function-based composition IS more expressive
HIGher-level abstractions
Modularity
Verbosity
Functional programming is not strictly superior to object oriented programming
HELLO RAMDA.JS
Familiar...
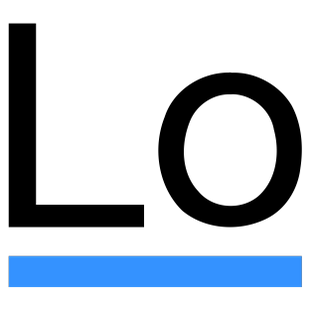

DATA-LAST
flexible calls
functional programming principles
functions are values
Declarative vs imperative
what is done
How it is done
Pure Functions & referential transparency
Immutable operations
Map, reduce, filter
Higher-order functions
Currying functions
Pointfree Style
Compose, Pipe
DRAWBACKS TO FP IN JS
hard to read
- Good Variable Naming
- Use a Type System (Typescript or Flow)
- Break up large anonymous compositions
harder to debug
- Insert transparent helpers for debugging
Less Efficient
- Don't prematurely optimize
- If performance matters, refactor
NEXT STEPS
Ramda Adjunct
Ramda extension library
Fantasy Land
Specification for "algebraic Data Structures" in JS
Type Systems
improve safety of functional programs
Functional Compile-to-JS Languages
-
ReasonML
-
Purescript
-
Elm
Other Pure Functional Languages
-
Haskell
-
OCAML
-
F# (.NET)
-
Scala, Clojure (JVM)
-
Erlang
-
Scheme, LISP
Resources
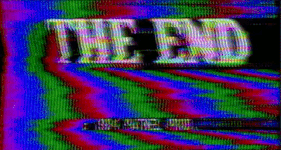
FUNCTIONAL PROGRAMMING & LAMBDA CALCULUS
"Lambda" Function
Anonymous function
monads
monoids
functors
chain
applicatives
bind
apply
setoids
CASE STUDY: DATA TRANSFORMATION PIPELINES
FUNCTIONAL JAVASCRIPT WITH RAMDA.JS
Functional Javascript with Ramda.JS
By Jett Durham
Functional Javascript with Ramda.JS
A gentle introduction to the functional approach of building software in Javascript with Ramda.JS
- 3,071