CTIN 483
Week 3 Thursday
Drawing
Today
-
Scripts are Components
-
Public variables
-
2D Collisions
-
are just like 3D collisions
-
Drawing
Simple Game
- 2017, Asymmetric
- 1 bit color
- Stick figure style
- Cool 2D shadows
- Open-world RPG though
Review: Components
GetComponent
myLight = gameObject.GetComponent<Light> ();
GetComponent is how you can talk to other components from a script. We've seen this before:
GetComponent
myLight = gameObject.GetComponent<Light> ();
How do you know what to put in the <>s? It's almost always the same as the name of the component that you see in the Inspector.

Remember that gameObject refers to the GO that your script is attached to.
Get (someone else's) Component
theirLight =
someOtherGO.GetComponent<Light>();
Get (someone else's) Component
theirLight =
someOtherGO.GetComponent<Light>();
Once you have a reference, i.e. a variable pointing to some other GameObject, you just put [variable name] instead of gameObject.
Then you are talking to that other GameObject and you can ask it for its components.
someOtherGO
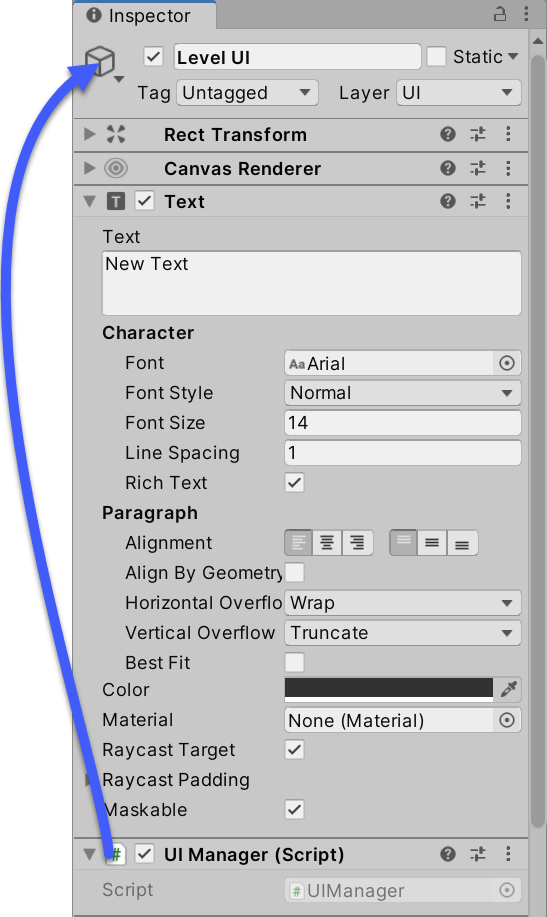
the gameObject you want to talk to
In this example, the script called UI Manager is attached to the gameObject called Level UI.
gameObject .GetComponent<Text>();
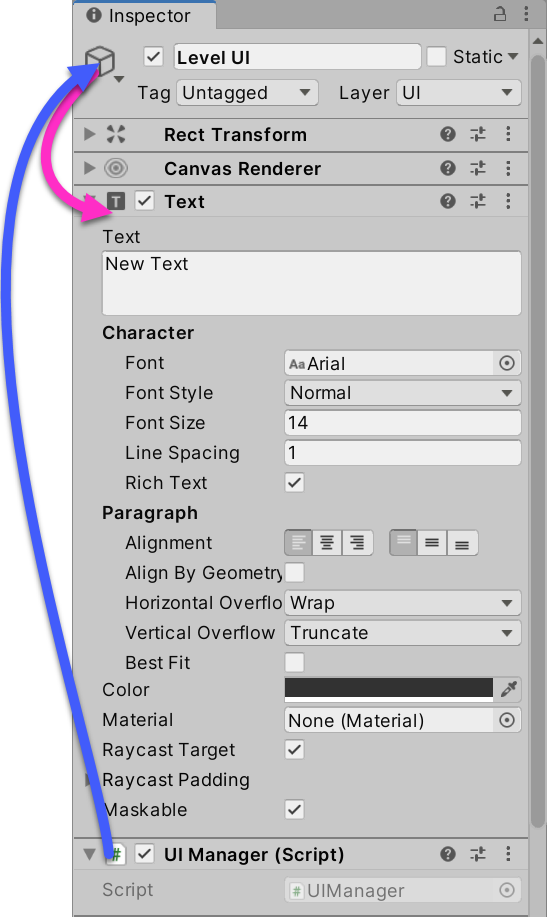
the function that asks the GO to look through its components and return a reference to the one with the type you want.
gameObject .GetComponent<Text>();
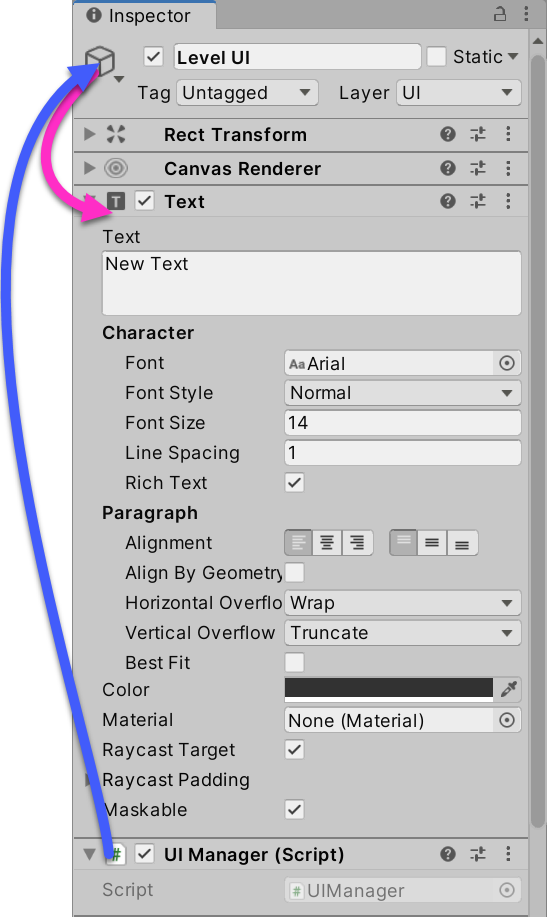
In this example, the gameObject called Level UI has a Text component.
So this piece of code in UIManager.cs will give you a reference to the Text component. Then your script can change the text it shows, or the color, or whatever you need.
The second line talks to the Text component and sets its "text" property to a new value.

levelNameText.text =
"LEVEL "+currentLevel.ToString();
Just like the Light component, the Text component has lots of different properties; its text is just one of them.
You can also change the text size, font, color, etc. by setting those properties.
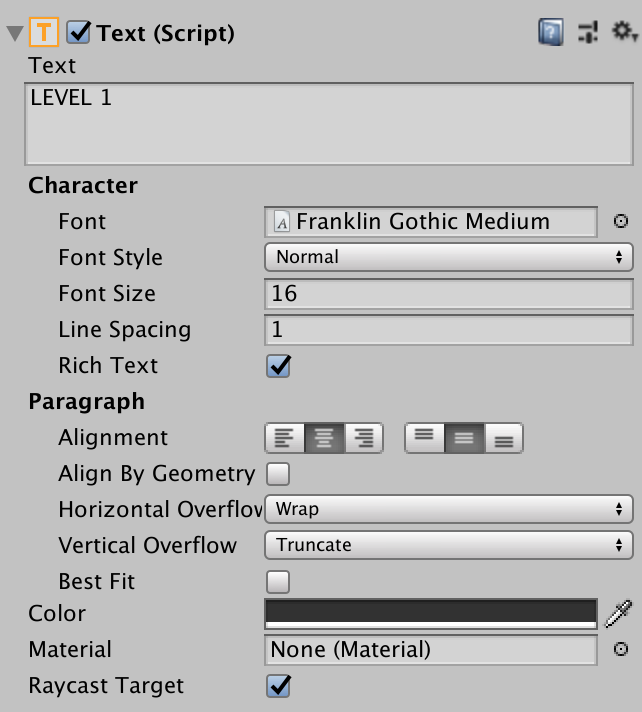
Stretch
References
In the Unity editor you can make all the GameObjects, components, materials, scripts, etc. that you want.
You can see them. The engine can "see" them. But your scripts can't.
Each script is an island unto itself. To talk to or change anything else, you need a reference.
A reference is (conceptually) an arrow that points to the GameObject, component, or file you want.
In a MonoBehaviour script, Unity gives you a few built-in references. The most important one is
gameObject
which means the GO that this script is attached to.
When you use
GetComponent<ComponentName>()
what is really happening is
gameObject.GetComponent<ComponentName>()
So Unity looks on the same GameObject as your script.
This is the pattern to get a reference to a component. Declare a variable with the type of component we want, and then go find it in the Start function.
Rigidbody rb; Collider myCollider; void Start() { rb = gameObject.GetComponent<Rigidbody>(); myCollider = gameObject.GetComponent<Collider>(); }
We'll see this pattern over and over in the scripts we write in class.
But notice you don't have to GetComponent to talk to a transform.
Transform is special, because every GameObject has a transform, even empty ones. Every other component will be on some GOs and not others.
someGameObject.transform
If you want access to another GameObject, you have to set that up yourself. We'll see many different ways to do that this semester.
The simplest one is this:
[SerializeField] GameObject theOtherGameObject;
This makes a slot on the script in the editor where you can just drag in another GO.
Once you have a reference to that other GO you can do all the GameObject-y things with it:
theOtherGameObject.name theOtherGameObject.transform theOtherGameObject.GetComponent<Light>()
and so on.
Stretch
Demo: Arkanoid
Credits
Paddle code and tutorial concept: NoobTuts
Draw Diagrams
CTIN 483 - W3 Th Sep 9 - 2021 Fall
By Margaret Moser
CTIN 483 - W3 Th Sep 9 - 2021 Fall
Talking to other scripts. 2D collisions. A simple game manager.
- 266