CTIN 583
Week 3 Monday
Today
-
Hello Robert
-
Homework and builds
Why are we here
Fluency
- You don't need to be good at code or enjoy programming
- But you can!
- Still...
Humility
- There will always be things you don't know, about code or about Unity
- Learn to enjoy not knowing what you're doing
- This has a lot of benefits for the creative process
Fluency
Here is one idea of what skills you'd need to express a range of ideas (co-written with Richard and Peter):
Jeremy's book is great! Recommended once you have a little Unity knowledge.
Homework
Stretch
Vectors
all you need to know
(almost)
Vectors
A vector is a mathematical object that is the basis of how games represent space. In math, a vector is an arrow.
A math vector has two basic parts:
- a direction (which way it is pointing)
- a magnitude (length)
magnitude
Magnitude is just a number.
Direction is represented with several numbers:
- x and y for 2D vectors
- x, y and z for 3D vectors
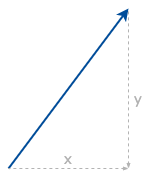
So you can use a Unity Vector to represent a math vector: an arrow pointing off into space.
But Unity also uses Vectors for other things, like position and scale. It's better to think of a Unity Vector as just a package of three numbers.
Some visuals will make this clearer.
This dot represents a position.
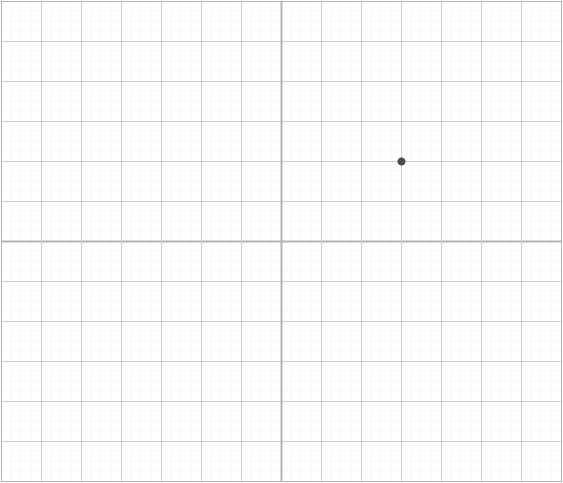
It can be described with two numbers, x and y.
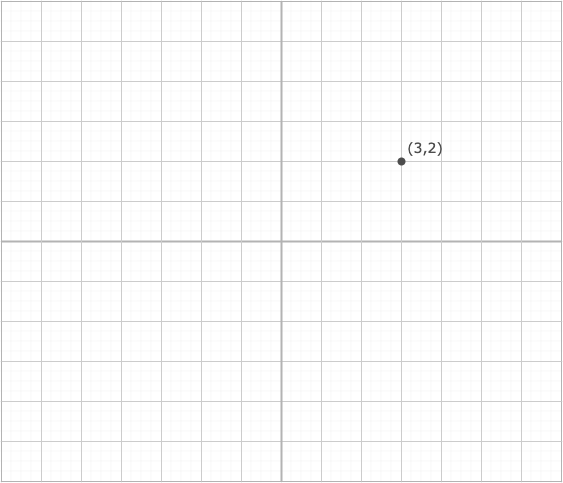
A different point.
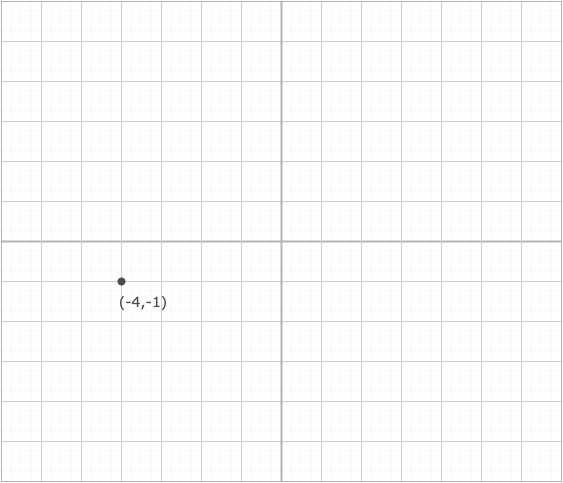
An arrow from (0,0) to a point is a vector.
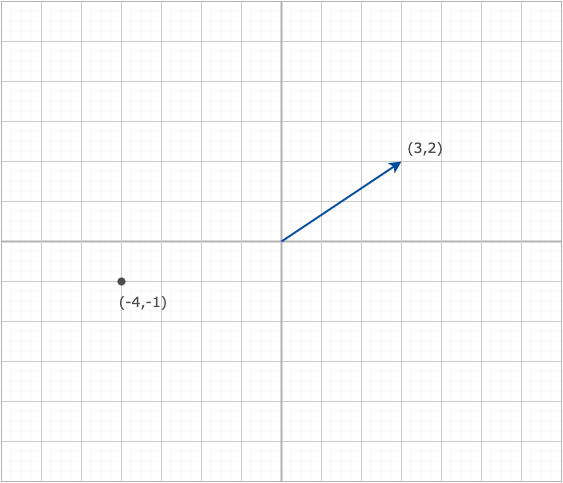
If you want to represent moving from one position to another, it might look like this.
What is the result?
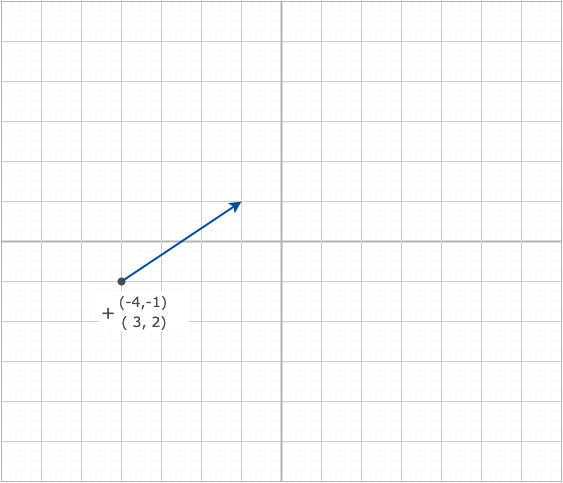
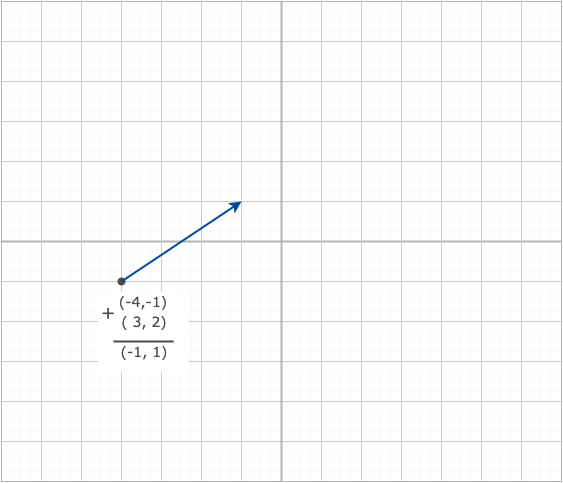
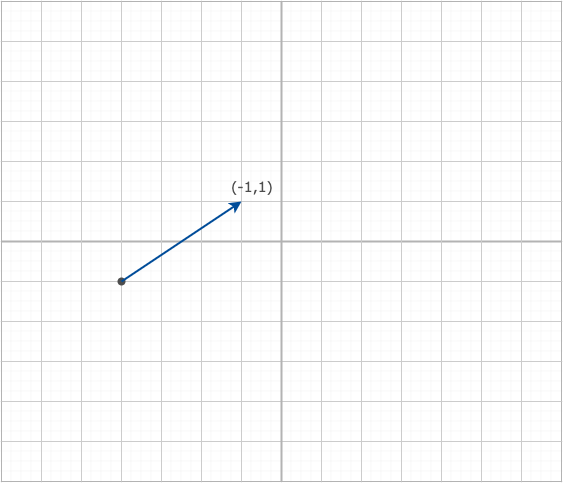
These are two vectors.
What does it mean to add them?
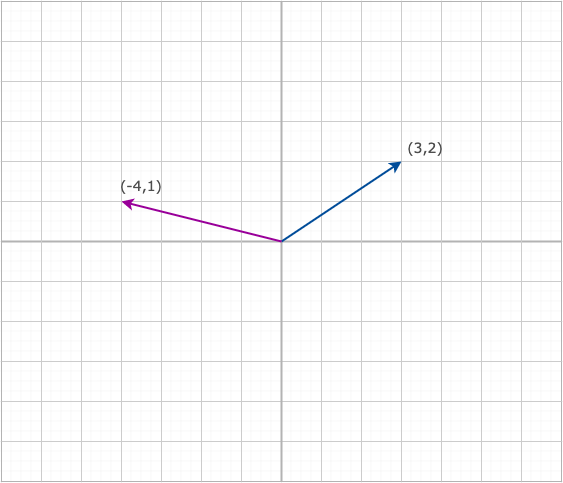
Visually, put the beginning of one at the end of the other. Mathematically, just add the numbers.
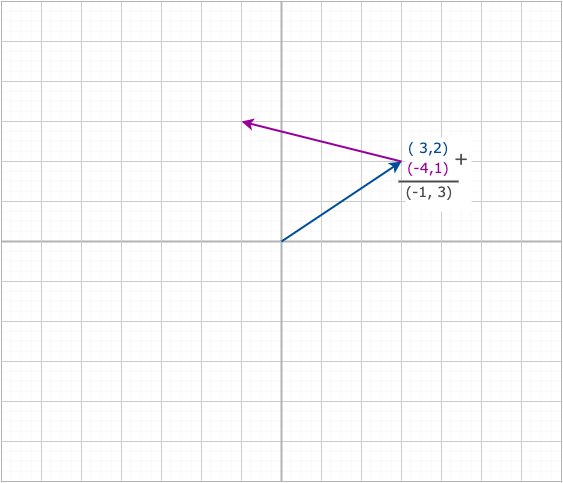
It works in the other order too.
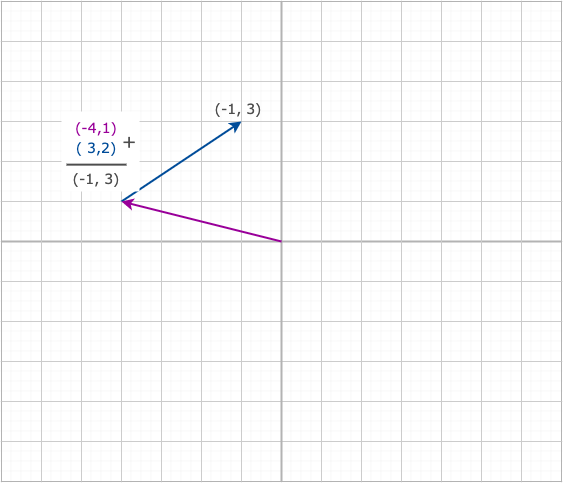
What does it mean to subtract two vectors?
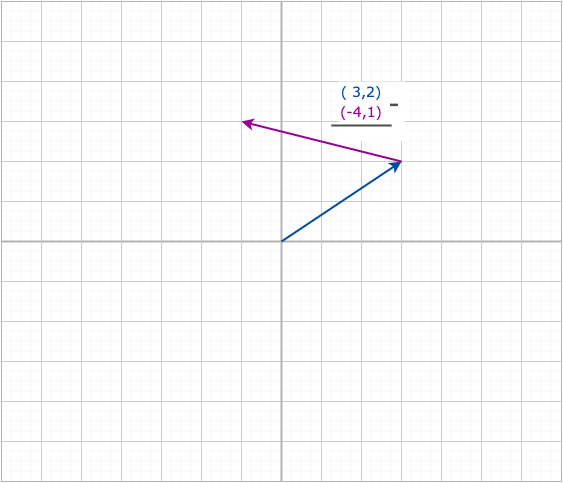
To subtract something is to add its opposite.
The opposite of a vector points the opposite direction.
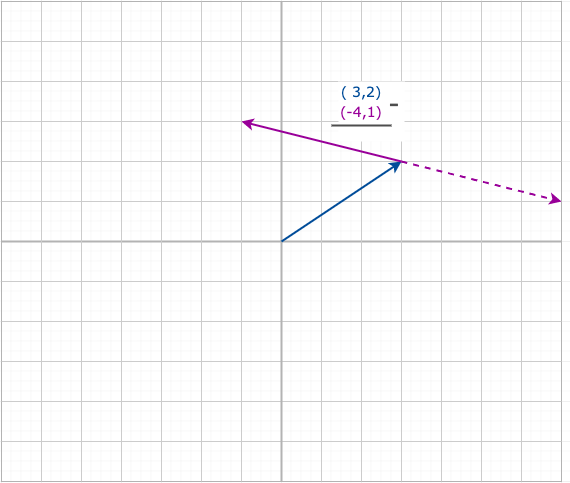
The math works the way you'd expect: subtract x from x and y from y.
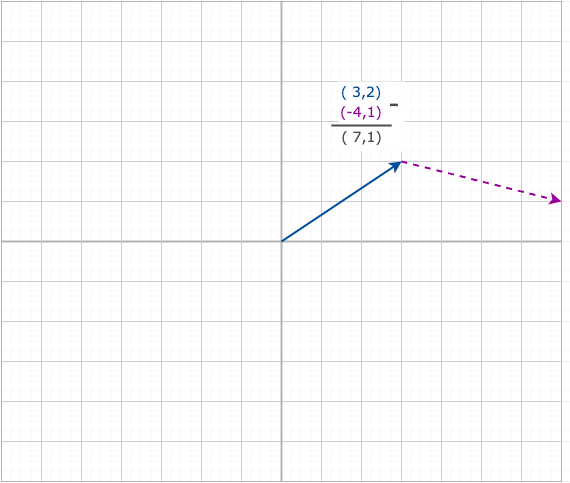
What about multiplying by one number?
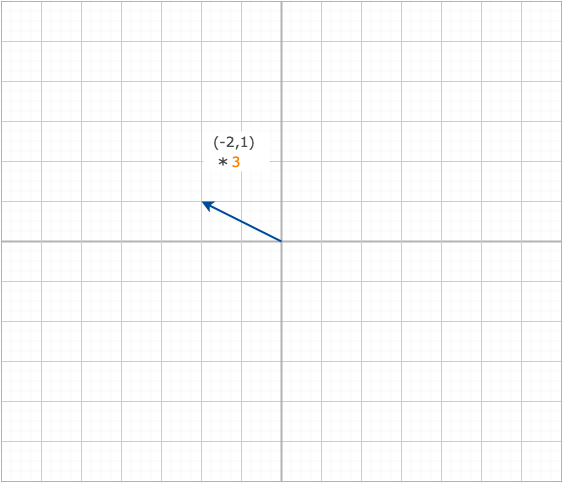
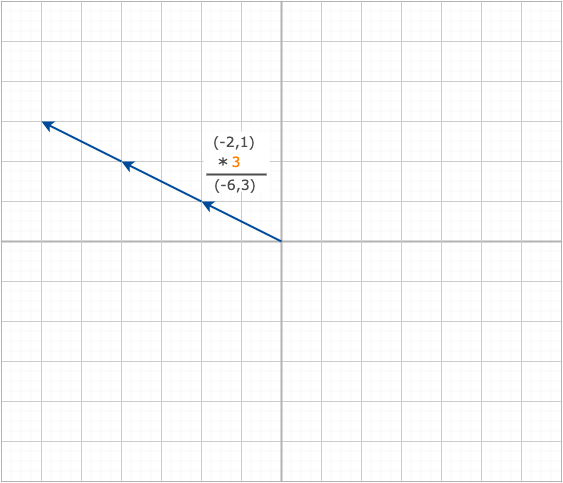
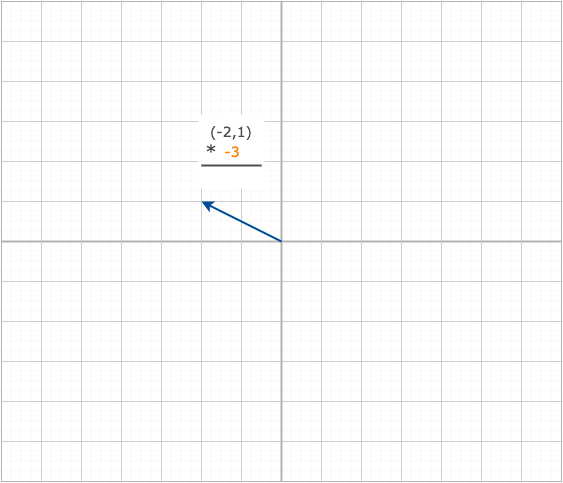
What about multiplying by a negative number?
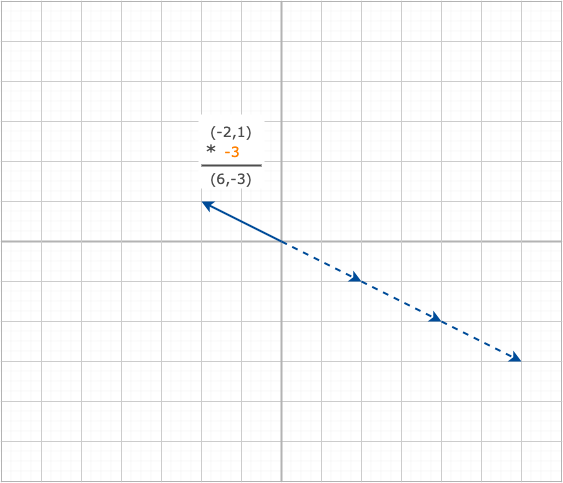
The opposite of a vector points the opposite direction.
These are useful shortcuts in code.
Each one is one unit long so you can multiply it by whatever you want.
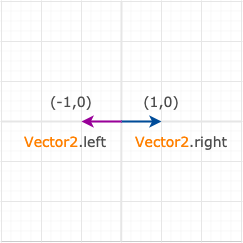
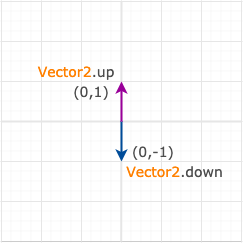
How about multiplying a vector by another vector?
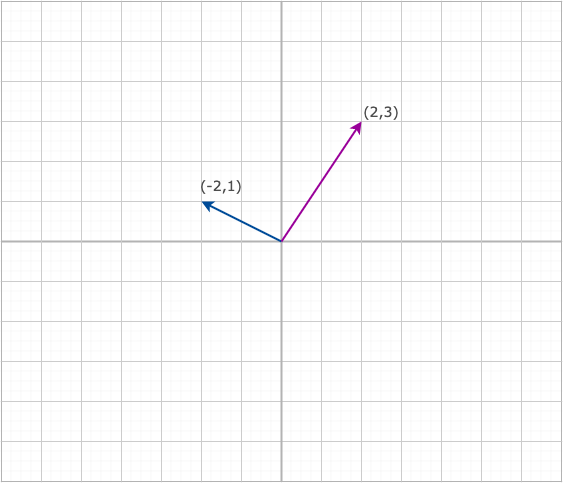
What would this mean, visually?
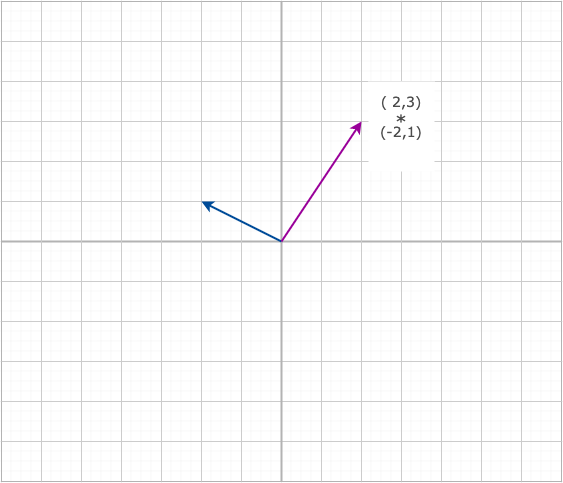
This doesn't seem to mean anything.
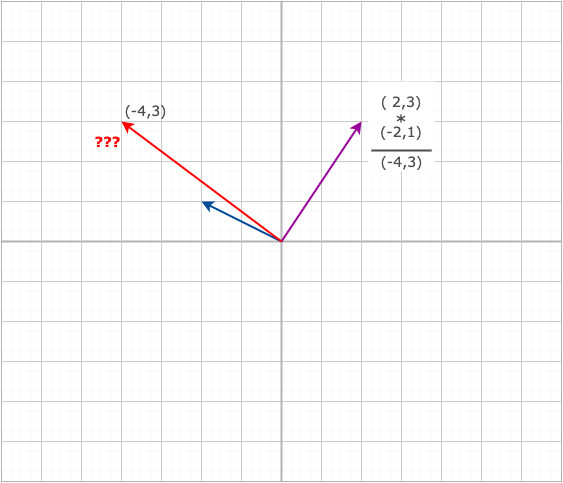
There are two ways to multiply vectors. But you don't need to know how to use Unity.
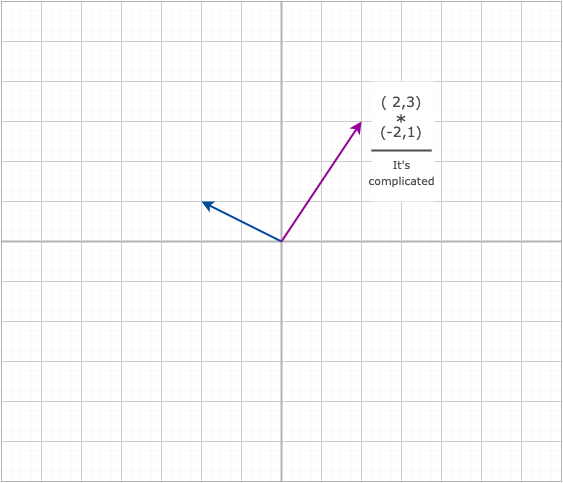
Stretch
Review: Components
GetComponent
GetComponent is an important and powerful bit of code, because it lets you talk to any of the components you can see in the Inspector (including other scripts).
So it is worth all the punctuation!
myLight = gameObject.GetComponent<Light> ();
GetComponent is how you can talk to other components from a script. For example, here's how you can get a reference to a Light component:
GetComponent
myLight = gameObject.GetComponent<Light> ();
How do you know what to put in the <>s? It's almost always the same as the name of the component that you see in the Inspector.

Remember that gameObject refers to the GO that your script is attached to. Later we will see how to talk to other GOs.
References
In the Unity editor you can make all the GameObjects, components, materials, scripts, etc. that you want.
You can see them. The engine can "see" them. But your scripts can't.
Each script is an island unto itself. To talk to or change anything else, you need a reference.
A reference is (conceptually) an arrow that points to the GameObject, component, or file you want.
In a MonoBehaviour script, Unity gives you a few built-in references. The most important one is
gameObject
which means the GO that this script is attached to.
When you use
GetComponent<ComponentName>()
what is really happening is
gameObject.GetComponent<ComponentName>()
So Unity looks on the same GameObject as your script.
This is the pattern to get a reference to a component. Declare a variable with the type of component we want, and then go find it in the Start function.
Rigidbody rb; Collider myCollider; void Start() { rb = gameObject.GetComponent<Rigidbody>(); myCollider = gameObject.GetComponent<Collider>(); }
We'll see this pattern over and over in the scripts we write in class.
But notice you don't have to GetComponent to talk to a transform.
Transform is special, because every GameObject has a transform, even empty ones. Every other component will be on some GOs and not others.
someGameObject.transform
If you want access to another GameObject, you have to set that up yourself. We'll see many different ways to do that this semester.
The simplest one is this:
[SerializeField] GameObject theOtherGameObject;
This makes a slot on the script in the editor where you can just drag in another GO.
Once you have a reference to that other GO you can do all the GameObject-y things with it:
theOtherGameObject.name theOtherGameObject.transform theOtherGameObject.GetComponent<Light>()
and so on.
Stretch
Game State
You saw a simple example of game state in the homework.
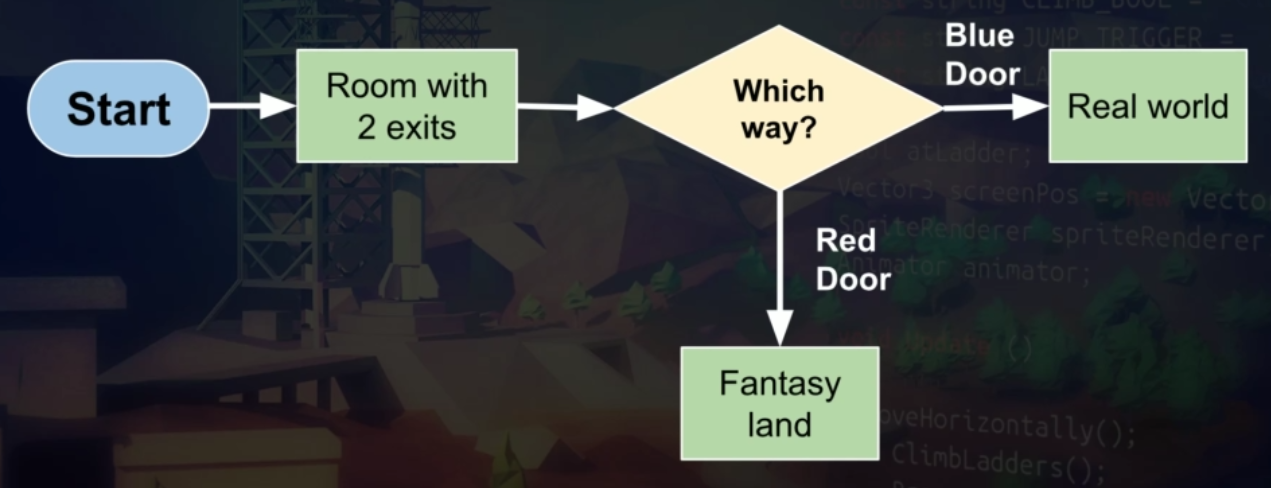
A state in Text 101 is two things:
- the text to show on the screen
- the options for going to other states
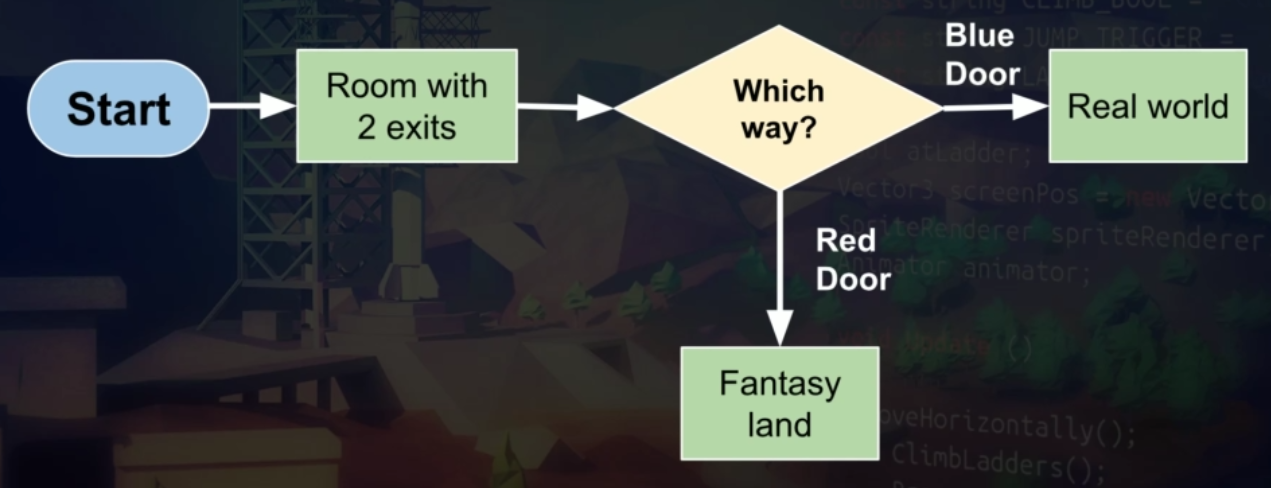
A state in Text 101 is two things:
- the text to show on the screen
- the options for going to other states
The states are discrete, meaning divided.
Each is its own world, and they do not overlap. The 1 key means different things in different states.
More broadly, at any given moment, your game (and all your GameObjects) will be in some condition.
- the ball is moving in this direction or that direction
- the player has a taco or doesn't
- an NPC knows the player's terrible secret or doesn't
- the cat is showing its bathing animation, or its angry-tail animation
- the ball is moving in this direction or that direction, but not both
- the player has a taco or doesn't, but not both
- an NPC knows the player's terrible secret or doesn't, but not both
- the cat is showing its bathing animation, or its angry-tail animation, but not both
The word state can mean the condition of a particular GameObject.
- its position, rotation and scale
- its velocity and any forces being applied to it
- which animation it is showing
- whether its components are enabled or disabled
- the health variable of the PlayerHealth script
But state can also describe the condition of your whole game.
- the positions of all the GOs, and
- whether each door has been unlocked, and
- the collectibles that are still in the game, and
- whether the player just pressed Space, and
- whether the player is standing on something.
idea of state is important because it lets you, the developer, control what is going on.
Unity keeps track of some things for you, such as position, rotation and scale.
Unity has some rules for responding when those things change.
For example, if an object collides with another object, the physics system knows they will change direction or velocity.
You control other things, such as player health. And you control what happens when they change.
Sometimes a particular value changes the state of the whole game.
For example, when player health reaches zero, you might want to change a number of other things.
Demo: Simple Race
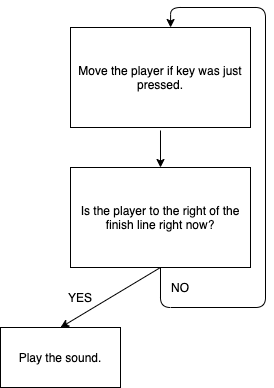
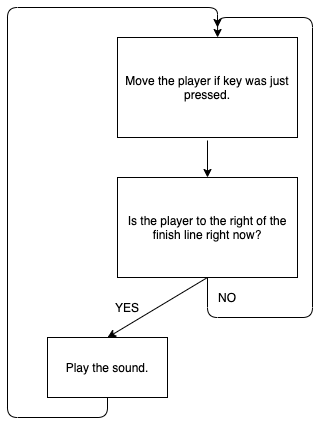
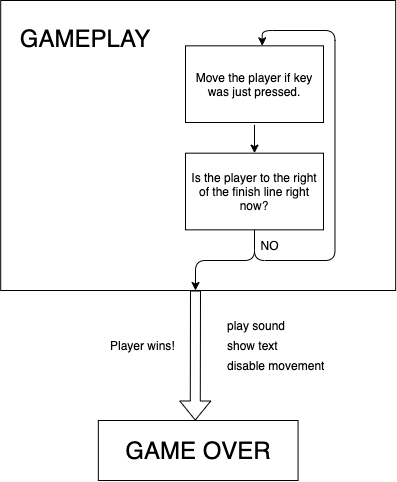
Here is the pattern for responding to something only once:
CTIN 583 - W3 Mo Jan 24 - 2022 Spring
By Margaret Moser
CTIN 583 - W3 Mo Jan 24 - 2022 Spring
Vectors. References. Game state.
- 223