Angular 2 Forms
@jurisicmarko
marko.jurisic@infonova.com

AngularConnect takeaways
- Angular 2 features
- Release cycles
- Marketing & Ecosystem
Angular 2 overview
Angular release cyles

Text
*Angular 2.1.0 released on October 13
Angular Ecosystem
- Augury
- Electron
- Angular Universal
- ag-grid
- mobile/progressive
AngularJS
as new official name
Angular 2 Forms
Template Driven
Implicit creation of FormControls via template
easier to setup for simple cases
Reactive
Explicit creation of all form elements
easier to test, provides more control
Template Driven
FormsModule
ngForm
ngModel
ngModelGroup
Reactive
ReactiveFormsModule
formControlName
formGroupName
formControl
formGroup
formArray
Form Building Blocks
- Form Controls
- Validators
- Observers
Form Controls
- FormControl
- FormGroup
- FormArray
- FormBuilder
Form Controls
- Value
- Validation status
- User interaction
- Events
FormControl
// create a new FormControl with the value "Willy"
let nameControl = new FormControl("Willy");
let name = nameControl.value; // -> Willy
// now we can query this control for certain values:
nameControl.errors // -> StringMap<string, any> of errors
nameControl.dirty // -> false
nameControl.valid // -> true
<input type="text" [formControl]="name" />
FormGroup
let personInfo = new FormGroup({
firstName: new FormControl("Willy"),
lastName: new FormControl("Wonka"),
zip: new FormControl("1020")
})
<form [formGroup]="form" (ngSubmit)="onSubmit(form.value)">
<input formControlName="firstName" placeholder="First name">
<input formControlName="lastName" placeholder="Last name">
<input formControlName="zip" placeholder="zip">
<button type="submit" class="btn default">Submit</button>
</form>
FormArray
let formArray: FormArray;
addHolidayControl(holiday?:Holiday):void {
let holidayControl: FormGroup = this.fb.group(
{
description: this.fb.control(undefined, Validators.required),
date: this.fb.control(undefined, Validators.required)
});
this.formArray.push(holidayControl);
}
Validators
// simplest use-case
this.fb.control(undefined, Validators.required)
this.fg = this.fb.group(
{
common: this.fgCommon,
holidays: this.fgHolidays
},
{
validator: ((fg: FormGroup) => {
let fgCommon: FormGroup = <FormGroup>fg.get('common');
let fgHolidays: FormGroup = <FormGroup>fg.get('holidays');
let dateFromControl: AbstractControl = fgCommon.get('date_domain_from');
let dateToControl: AbstractControl = fgCommon.get('date_domain_to');
let fromToResult = this.validateFromTo(dateFromControl, dateToControl);
let validateHolidaysResult = this.validateHolidays(dateFromControl, dateToControl,
fgHolidays);
return _.assign({}, fromToResult, validateHolidaysResult);
})
}
);
Demo
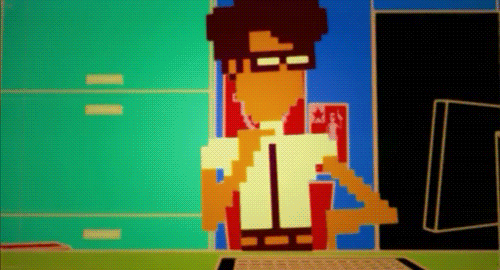
References
-
Day 2 Keynote | Igor Minar and Rob Wormald
https://www.youtube.com/watch?v=LWZO_Bu6c2I - Igor Minar, Versioning and Releasing Angular, Oct 08, 2016 http://angularjs.blogspot.co.at/2016/10/versioning-and-releasing-angular.html
- Kara Erickson, Angular Forms,
https://goo.gl/muQVAd
-
Lerner, Coury, Murray, Taborada, ng-book 2
https://www.ng-book.com/2/
QUESTIONS?
@jurisicmarko
marko.jurisic@infonova.com
Angular 2 Forms
By Marko Jurišić
Angular 2 Forms
- 1,388