Angular 2 preview
Wien, 06.08.2015
Łukasz Rzeszotarski @lrzeszotarski
Marko Jurisic @jurisicmarko
Angular 2 Motivation
- Angular 1 is aging - codebase from 2009
- Web standards
- shadow DOM
- ES6
- Performance: "Today, Angular2 is 5x faster than Angular 1"
- Simple cognitive model
https://angular2-intro.firebaseapp.com
Angular 1.x Concepts
-
Controller
-
Factory
-
Service
- Provider
- Directive
- Transclusion
- Module
- Scope
Angular 2
- Complete rewrite, no backwards compatibility

Angular 2
Our goal with Angular 2 is to make the best possible set of tools for building web apps not constrained by maintaining backwards compatibility with existing APIs.
Angular blog
Goodbye
- $scope
- $apply(), digest loop
- Controllers
- Directive Definition Object
- transclusion
- angular.module system
- jqLite (angular.element)
- 2-way data binding
- service vs. factory vs. provider vs. ...
Hello
- classes (think controllerAs in Angular 1.2+)
- zone.js
- classes
- classes + annotations
- shadow DOM
- ES6 modules
- plain DOM methods
- explicit data flow
- di.js
Angular 2
http://slides.com/michaelbromley/angular-2-a-first-look#/4
ANGULAR 1.X
- Create Module
- Declare ng-app
- Create controller
- Attach items to $scope
- Declare Controller
- Create Template
ANGULAR 2
- Create Component
- Create Template
- Bootstrap
- Transpilation
Bootstrapping
ANGULAR 1.X
ANGULAR 2
Bootstrapping
// create module
var app = angular.module('app', []);
// create controller
app.controller('MyCtrl', function($scope) {
// attach items to $scope
$scope.name = 'David';
});
import {Component, Template, bootstrap} from 'angular2/angular2';
import {TodoApp} from 'todoapp';
// create component
@Component({
selector: 'todo-app',
})
@Template({ url: 'todos.html' })
export class TodoApp {
todos;
constructor() {
this.todos = ['Item1', 'Item2'];
}
}
// bootstrap
bootstrap(TodoApp);
<!-- declare ng-app -->
<body ng-app="app">
<div ng-controller="MyCtrl">
My name is {{ name }}
</div>
</body>
Template syntax
<div> My name is {{ name }} </div>
<div>
<input #newname type="text">
<button (click)="changeName(newname.value)"
[disabled]="newname.value == 'David'"> Change Name
</button>
</div>
They are pretty much using the entire keyboard for all the symbols, letters and numbers you can think of. I haven't used the `@%^~ for many years, and i liked it that way
@Template({
url: 'name-change.html'
})
@Component({
selector: 'name-change',
})
class NameChange {
constructor() {
this.name = '';
}
changeName(newName) {
this.name = newName;
}
}
Uniformity
- #element marks plain DOM element
- (event) for events
- [property] for properties
- * for built-in directives (ng-for, ng-if)
<input #newname />
<button (click)="changeName(newname.value)"
[disabled]="newname.value == 'David'"> Change Name
</button>
<ul>
<li *ng-for="#error of errors; #i = index">
Error {{i}} of {{errors.length}}: {{error.message}}
</li>
</ul>
<some-component [prop]="someExp" (event)="someEvent()" [(two-way-prop)]="someExp"></show-title>
<--! same as: -->
<some-component bind-prop="someExp" on-event="someEvent()" bindon-two-way-prop="someExp">
ANGULAR 1.X
ANGULAR 2
Event handlers
<div>
<input ng-model="name" type="text">
<button ng-click="changeName()">
</div>
<div>
<input #newname type="text">
<button (click)="changeName($event, newname.value)">
</div>
Change detection
http://victorsavkin.com/post/110170125256/change-detection-in-angular-2
Every component gets a change detector responsible for checking the bindings defined in its template
Zone.js - Informs Angular when to run change detection
Local Variables
<div>
<input #newname type="text">
{{ newname.value }}
</div>
Migration path
https://www.airpair.com/angularjs/posts/preparing-for-the-future-of-angularjs
"It's really hard for us to build a bridge to a city that doesn't exist yet."
Brad Green, Engineering Director, Angular core team
Migration path
- Make Directives, not Controllers
-
Clean up your $scopes (controllerAs)
-
Avoid scope.$parent
-
Avoid scope.$on (if possible)
-
Avoid scope message passing
-
Prefer scope inheritance with namespaces
-
-
TypeScript
http://paislee.io/migrating-to-angularjs-2-0/
References
- https://angular.io/
- http://ng-learn.org/2014/03/AngularJS-2-Status-Preview/
- http://slides.com/michaelbromley/angular-2-a-first-look
- http://blog.mgechev.com/2015/04/06/angular2-first-impressions/
- https://angularjs.de/artikel/controller-as-syntax
- http://victorsavkin.com/post/110170125256/change-detection-in-angular-2
- http://victorsavkin.com/post/119943127151/angular-2-template-syntax
- https://github.com/mgechev/angular2-seed
- http://blog.thoughtram.io/angular/2015/05/09/writing-angular-2-code-in-es5.html
- http://paislee.io/migrating-to-angularjs-2-0/
- https://www.airpair.com/angularjs/posts/preparing-for-the-future-of-angularjs
Code
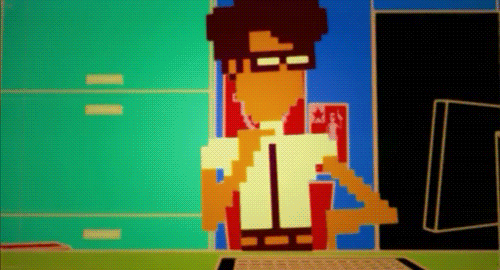
Angular 2 preview
By Marko Jurišić
Angular 2 preview
- 968