Détection de collision

Distance entre deux points


Code distance
function distanceBetween(x1, y1, x2, y2) {
var dx = x1 - x2,
dy = y1 - y2;
return Math.sqrt(dx * dx + dy * dy);
}
Un point dans un rectangle


Code
function isPointInRect(point, rect) {
if( point.x > rect.x && point.x < rect.width &&
point.y > rect.y && point.y < rect.height){
return true;
}
return false;
}
Bounding box



Code
function overlapSquare(x1, y1, w1, h1, x2, y2, w2, h2) {
if (x1 + w1 > x2 && x1 < x2 + w2 && y1 + h1 > y2 && y1 < y2 + h2) {
return true;
}
return false;
}
Point dans un cercle

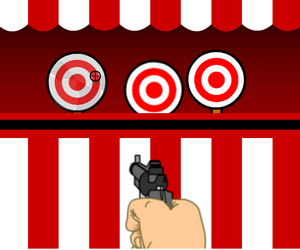
Code
function isPointInCircle(cx, cy, r, x, y){
var dx = (cx - x)^2,
dy = (cy - y)^2,
d = Math.sqrt( dx + dy);
return d <= r;
}
Collision entre 2 cercles



Code
function overlapCircle(cx1, cy1, cr1, cx2, cy2, cr2) {
var dx = cx1 - cx2, dy = cy1 - cy1, distance = (dx * dx + dy * dy);
if (distance <= (cr1 + cr2) * (cr1 + cr2)) {
return true;
}
return false;
}
Intersection de ligne


Code
function checkLineIntersection(line1StartX, line1StartY, line1EndX, line1EndY, line2StartX, line2StartY, line2EndX, line2EndY) {
var denominator, a, b, numerator1, numerator2, result = { x: null, y: null, onLine1: false, onLine2: false };
denominator = ((line2EndY - line2StartY) * (line1EndX - line1StartX)) - ((line2EndX - line2StartX) * (line1EndY - line1StartY));
if (denominator === 0) { return result; }
a = line1StartY - line2StartY;
b = line1StartX - line2StartX;
numerator1 = ((line2EndX - line2StartX) * a) - ((line2EndY - line2StartY) * b);
numerator2 = ((line1EndX - line1StartX) * a) - ((line1EndY - line1StartY) * b);
a = numerator1 / denominator;
b = numerator2 / denominator;
// if we cast these lines infinitely in both directions, they intersect here:
result.x = line1StartX + (a * (line1EndX - line1StartX));
result.y = line1StartY + (a * (line1EndY - line1StartY));
// if line1 is a segment and line2 is infinite, they intersect if:
if (a > 0 && a < 1) { result.onLine1 = true; }
// if line2 is a segment and line1 is infinite, they intersect if:
if (b > 0 && b < 1) { result.onLine2 = true; }
// if line1 and line2 are segments, they intersect if both of the above are true
return result;
}
Intersection de ligne et d'un cercle

Code
function interceptOnCircle(p1, p2, c, r) {
var p3 = {x:p1.x - c.x, y:p1.y - c.y}; //shifted line points
var p4 = {x:p2.x - c.x, y:p2.y - c.y};
var m = (p4.y - p3.y) / (p4.x - p3.x); //slope of the line
var b = p3.y - m * p3.x; //y-intercept of line
var underRadical = Math.pow(r,2)*Math.pow(m,2) + Math.pow(r,2) - Math.pow(b,2);
if (underRadical < 0) {
//line completely missed
return false;
} else {
var t1 = (-m*b + Math.sqrt(underRadical))/(Math.pow(m,2) + 1);
var t2 = (-m*b - Math.sqrt(underRadical))/(Math.pow(m,2) + 1);
var i1 = {x:t1+c.x, y:m*t1+b+c.y};
var i2 = {x:t2+c.x, y:m*t2+b+c.y};
return [i1, i2];
}
}
Formes complexes

svg path simplification

Code
function checkPathIntersection(path1, path2, accuracy, debug){
var collision, line1StartX, line1StartY, line1EndX, line1EndY,l,m,
line1 = pathToPolyline(path1, accuracy, debug),
line2 = pathToPolyline(path2, accuracy, debug),
colisions = [];
if(debug){
svg.appendChild(line1.polyline);
svg.appendChild(line2.polyline);
}
for(l = 1; l < line1.points.length; l++){
line1StartX = line1.points[l-1][0];
line1StartY = line1.points[l-1][1];
line1EndX = line1.points[l][0];
line1EndY = line1.points[l][1];
for(m = 1; m < line2.points.length; m++){
line2StartX = line2.points[m-1][0];
line2StartY = line2.points[m-1][1];
line2EndX = line2.points[m][0];
line2EndY = line2.points[m][1];
collision = checkLineIntersection(line1StartX, line1StartY, line1EndX, line1EndY, line2StartX, line2StartY, line2EndX, line2EndY);
if(collision.onLine1 && collision.onLine2){
colisions.push(collision);
}
}
}
if(colisions.length){
return colisions;
}
return false;
}
Outils


Quelques liens
Tutoriel : collision-detection-with-svg
Théorie : basic-collision-detection-in-2d
Livre sur le SVG : svgpocketguide
Cheat sheet : SVG
Merci

Detection de collision
By Teddy K
Detection de collision
- 588