Introduction to JavaScript & jQuery
Rule #1
If you have a question, answer it!
You can ask me, or you can ask the person next to you or you can look it up.
Rule #2
Help Each Other
Coding is a community! That’s one of the best things about it! Everyone helps everyone, even if you are brand new you may see something a different way…
What is JavaScript?
It gives your website a brain!
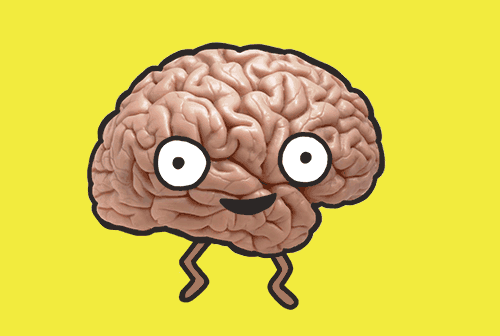
How we use JavaScript
Variables, Statements and Functions
JavaScript
It's a lot of :
This is this,
and if this does that,
then do this
or do something else
Let's Start
We're going to work with three files
index.html
style.css
myscript.js
The Console
Mac osx : Cmd + Alt + I
Windows : Ctrl + Alt + I
Variables
Variables are like a vocabulary that we make up, and that we use to speak to our program.
hey computer, what's my name?
what's a name ?
Statements
This is that.
The sky is blue.
We use statements to define variables and talk with our program.
My name is Teddy
Functions
are "mini programs"
they wrap up statements and logic and variables.
Function Example
i'm a robot.
i know four things.

"right foot up"
"right foot down"
"left foot up"
"left foot down"
Function Example
var stepLeft = function (){ console.log("left foot up"); console.log("left foot down");
}; var stepRight = function (){ console.log("right foot up");
console.log("right foot down");
};
Function Example
call a function
stepLeft();
Make it dance
var dance = function (){ stepLeft(); stepRight(); stepLeft();
stepRight(); };


jQuery
It's a JavaScript library that makes life easier.
JavaScript is like getting a puppy.

You can train it to do anything you want, but it takes time.
jQuery is like getting super well trained dog.
It already knows how to do a ton of stuff!!
Add jQuery
To add jQuery we just paste this line
in between <head> and </head>
<script src="https://code.jquery.com/jquery-2.1.1.min.js"></script>
Also add some content
inside the index.html
<div class="content">
<h1>Welcome to my awesome site!</h1>
</div>
Let's make it pretty
inside style.css
body {
width: 800px;
margin: 0 auto;
padding-top: 50px;
font-family: Helvetica;
background: whitesmoke;
}
.content {
padding: 20px;
background: white;
border: 1px solid grey;
border-radius: 5px;
}
Fun With Console
Open the console, and let's mess around with our title.
Try typing this into the console
$('h1').css('color','red');
Sweet, now give this a go
$('h1').css('font-size','50px');
What is going on?
We are using jQuery to change how our website looks!
Let's break it down
$("h1") is like saying "Hey jQuery, go find anything on the page that has an 'h1' tag, please."
.css("color","red") is saying "Now add some CSS style to all those things you can just got. I want that style to be the color, and make it red, please"
Let's make some interactive buttons!
index.html
<h1>Welcome to my awesome site!</h1>
<div id="redBtn" class="btn">Red</div>
<div id="greenBtn" class="btn">Green</div>
<div id="blueBtn" class="btn">Blue</div>
Let's make 'em pretty!
style.css
.btn {
padding: 10px;
background: lightgrey;
display: inline-block;
border-radius: 4px;
border: 1px solid grey;
}
.btn:hover {
background: pink;
cursor: pointer;
}
and lets power them!
jQuery "click" let's us listen for a click event.
When that event happens, we can have some code run!
$("TARGET ELEMENT").click(
function(){
//CODE TO RUN HERE
});
Code for our buttons
myscript.js
$("#redBtn").click(
function() {
$("h1").css("color","red");
console.log("test");
}
);
$("#blueBtn").click(
function() {
$("h1").css("color","blue");
}
);
$("#greenBtn").click(
function() {
$("h1").css("color","green");
}
);
if / then
let's make a toggle button
HTML
<div class="btn lightsOn switch">turn lights off</div>
if / then
We want to write some code that says
"when the button is clicked, check if it's 'on'.
If it's 'on', turn the lights off 'off'.
Otherwise, turn the lights 'on'.
"when the button is clicked, check if it's 'on'.
If it's 'on', turn the lights off 'off'.
Otherwise, turn the lights 'on'.
How do we do this?
What does off and on mean?
What does off and on mean?
jQuery click
$(“.switch”).click( function() {
//check if switch is on
//if on, turn off lights
//otherwise, turn on lights } );
Hint
$(element).hasClass("ClassName") returns true or false
$(element).removeClass("ClassName") removes a class
$(element).addClass("ClassName") adds a class
final code
myscript.js
var turnOff = function(){
$(".switch").removeClass("lightsOn");
$(".switch").addClass("lightsOff");
$(".switch").text("Turn Lights On");
$("body").css("background","black");
};
var turnOn = function(){
$(".switch").removeClass("lightsOff");
$(".switch").addClass("lightsOn");
$(".switch").text("Turn Lights Off");
$("body").css("background","whitesmoke");
};
$(".switch").click(
function() {
if ( $(".switch").hasClass("lightsOn") ) {
turnOff();
console.log("turned off");
} else {
turnOn();
console.log("turned on");
}
});
});
What else can jQuery do?
Fade In and Out Elements with .fadein() & .fadeOut()
Animate Elements with .animate()
Animate Elements with CSS Transitions
Slide Elements in and Out with .slideOut();
Create New Elements with .append()
Hide Elements .hide()
Remove Elements .remove()
Toggle Showing an Element with .toggle()
Trigger an event with the mouse moves, hovers, left click, right click...
If we have time, lets make something else... ideas?
Introduction to js & jquery
By Teddy K
Introduction to js & jquery
- 816