CS50P 0
Functions, Variables
Sample Code
Datatype (資料型態)
Name | Type | Note |
---|---|---|
integer | int | -1,-2,0,1,2 (整數) |
floating point | float | -1.1, 0.3, 1.2 (浮點數) |
boolean | bool | True / False (布林值) |
-
Numeric type(數值型態): int, float, bool
-
String type(字串型態) : str
Datatype Example
iv = 10 # 將整數 10 指派給變數 iv
fv = 12.3 # 將浮點數 12.3 指派給變數 fv
sv = 'hello python' # 將字串 'hello python' 指派給變數 sv
bv = True # 將布林值 True 指派給變數 bv
print('iv :', iv, '; fv :', fv, '; sv :', sv, '; bv :', bv)
print(type(iv)) # type(iv)會得到 iv 的形態 ==> int
print(type(fv)) # type(fv)會得到 fv 的形態 ==> float
print(type(sv)) # type(sv)會得到 sv 的形態 ==> str
print(type(bv)) # type(bv)會得到 bv 的形態 ==> bool
iv : 10 ; fv : 12.3 ; sv : hello python ; bv : True
<class 'int'>
<class 'float'>
<class 'str'>
<class 'bool'>
Python Tutor 🔗
Datatype Example
Numeric Operation : Int 🆚 Float
不同型態間的運算,自動轉換成範圍比較大的型態
int < float
print(4 + 2) # 6
print(4.0 + 2) # 6.0
print(4.0 + 2.0) # 6.0
print(2 / 5) # 0.4
print(2.0 / 5) # 0.4
print(2 / 5.0) # 0.4
print(2.0 / 5.0) # 0.4
print(2 // 5) # 0
str - string type (字串型態)
Declaring Strings in Python (在 Python 中宣告字串)
-
Use single quotes: '...'
-
Or double quotes: "..."
What is a String? (什麼是字串?)
-
A sequence of characters (一串字)
String Example
str1 = '12345' # 用 '...' 宣告字串 str1
str2 = "hello world" # 用 "..." 宣告字串 str2
print(f"str1 is {str1} {type(str1)}") # 印出 str1 的內容與型態
print(f"str2 is {str2} {type(str2)}") # 印出 str2 的內容與型態
str1 is 12345 <class 'str'>
str2 is hello world <class 'str'>
Is it a String?
Example | String? |
---|---|
“2” | Yes ✅ |
‘3.6’ | Yes ✅ |
“True” | Yes ✅ |
7 | No ❌ |
HELLO | No ❌ |
True | No ❌ |
Declare a String containing ' and "
' I'm learning Python! '
" It is a word "test". "
Single-Quoted String with Apostrophe ❌
程式中的第一個引號 ' 視為字串的開始,並將第二個引號 ' 視為字串的結束
Python sees the string as starting with the first single quote and ending with the apostrophe in "I'm",
' I'm learning Python! ' # 用 '...' 宣告的字串有 '
File "<ipython-input-36-f8fc62258cda>", line 1
' I'm learning Python! ' # 用 '...'' 宣告的字串有 ''
^
SyntaxError: invalid syntax
👆
👆
Double-Quoted String with Internal " ❌
程式中的第一個引號 " 視為字串的開始,並將第二個引號 " 視為字串的結束
The string starts with the first double quote and Python will interpret the next double quote (before "test") as the end of the string
"It is a word "test"." # 用 "..." 宣告的字串有 "
File "<ipython-input-20-8d044cdc5263>", line 1
"It is a word "test"." # 用 "..." 宣告的字串有 "
^
SyntaxError: invalid syntax
👆
👆
How to Properly Declare Strings
Leveraging Escape Characters (利用跳脫字元)
Represent special characters within strings.
Choosing Between Quotation Marks (選擇不同的引號)
Opt for single ('...') or double ("...") quotes based on the content.
Escape Characters \
\\ : Represents the backslash character itself (\).
\n : Represents a newline
\t : Represents a tab
\" : Used to include a double quote character ("") inside a double-quoted string without ending the string.
\' : Used to include a single quote character (') inside a single-quoted string without ending the string.
Single-Quoted String with Apostrophe \ ' 🉑
' I\'m learning Python! ' # 用 '...' 宣告的字串有 '
" I'm learning Python! "
Double-Quoted String with Internal " \" 🉑
"It is a word \"test\"." # 用 "..." 宣告的字串有 "
'It is a word "test".'
Choosing Between Quotation Marks 🉑
str1 = "I'm learning Python! " # 用 "..." 宣告的字串有 '
str2 = 'It is a word "test".' # 用 '...' 宣告的字串有 "
print(f"str1: {str1}")
print(f"str2: {str2}")
I'm learning Python!
It is a word "test".
String Operations
-
+
-
*
-
in
String Operation +
+ (串接 ):
- 將兩個或多個字串組合成一個新的字串
- " Hello " + " Ted . " --> " Hello Ted. "
- 何時以及為什麼我們需要串接?
- 組合多個資訊生成一個完整的消息。
- 組合固定的文本和變數值。
- 從多個來源收集資訊並組合。
string + string
字串 + 字串 ==> 字串串接
name = "May"
print("Hello " + name + " :)")
gretting = "Hi, " + name
print(gretting + " :)")
Hello May :)
Hi, Amy! :)
String Operation *
* (重複) :
- 重複一個字串多次
- "abc" * 3 → "abcabcabc"
- 字串重複的實際應用是什麼?
- 生成重複的模式或邊界。
- 文本格式化時的填充。
- 重複特定的模式或句子以產生特效。
string * number
字串 * n ==> 字串重複 n 次
hi = "Hi. " # 這是字串 hi
hi3 = hi * 3 # 重覆3次 hi 的內容
print("hi :", hi)
print("hi3 :", hi3)
hi : Hi.
hi3 : Hi. Hi. Hi.
String Operation in
in (包含):
- 檢查一個字串是否包含在另一個字串中
- “is" in "It is a pen" --> True
- 檢查字串中的字串的實際應用是什麼?
- 搜索特定的關鍵字或文句。
- 驗證輸入的合法性。
- 文本分析中的模式識別。
string in string
"字串A" in "字串B": 字串 A 是否在字串 B 中
string = "Emma is there"
print("Emma" in string) # "Emma" 是否在 string 中
print("emma" in string) # "emma" 是否在 string 中
True
False
String Method Reference
Demo I of String Method
s = " hello WORLD. "
print(f"Original string: |{s}|")
print(f"After strip(): |{s.strip()}|")
print(f"Capitalize: |{s.strip().capitalize()}|")
print(f"Title case: |{s.strip().title()}|")
print(f"To uppercase: |{s.upper()}|")
print(f"To lowercase: |{s.lower()}|")
print(f"Center with '*' upto 30: |{s.center(30, '*')}|")
start_str = ''.join('*' if i % 5 == 0 else '-' for i in range(1, 31))
position_str = ' ' * len("Center with '*': ") + "|"+start_str+"|"
print(position_str)
print(f"Starts with 'hello': {s.startswith('hello')}")
print(f"Ends with 'world.' : {s.endswith('world.')}")
print(f"Position of 'world': {s.find('world')}")
print(f"Last position of 'o': {s.rfind('o')}")
print(f"Count of 'l': {s.count('l')}")
print(f"Is alphabetic: {s.isalpha()}")
print(f"Is digit: {'12345'.isdigit()}")
print(f"Is space: {' '.isspace()}")
Demo I of String Method
Original string: | hello WORLD. |
After strip(): |hello WORLD.|
Capitalize: |Hello world.|
Title case: |Hello World.|
To uppercase: | HELLO WORLD. |
To lowercase: | hello world. |
Center with '*' upto 30: |****** hello WORLD. ******|
|----*----*----*----*----*----*|
Starts with 'hello': False
Ends with 'world.' : False
Position of 'world': -1
Last position of 'o': 7
Count of 'l': 2
Is alphabetic: False
Is digit: True
Is space: True
Demo II of String Method
s = "hello world."
print(f"Original string: {s}\n")
# Group: "Is" functions
print("=== Checking Properties ===")
print(f"Is alphanumeric: {s.isalnum()}")
print(f"Is lowercase: {s.islower()}")
print(f"Is uppercase: {s.swapcase().isupper()}")
print(f"Is digit: {'12345'.isdigit()}")
print(f"Is alphabetic: {s.isalpha()}")
print(f"Is space: {' '.isspace()}\n")
# Group: Positional & Search functions
print("=== Positional & Search ===")
print(f"Starts with 'hello': {s.startswith('hello')}")
print(f"Ends with 'world.': {s.endswith('world.')}")
print(f"Position of 'world': {s.find('world')}")
print(f"Index of 'o': {s.index('o')}\n")
# Group: Transformation functions
print("=== Transformations ===")
print(f"Swap case: {s.swapcase()}")
print(f"Join list with ',': {','.join(['a', 'b', 'c'])}")
splitlines_example = 'Hello\nWorld'.splitlines()
print("Split lines: ", splitlines_example)
Demo II of String Method
Original string: hello world.
=== Checking Properties ===
Is alphanumeric: False
Is lowercase: True
Is uppercase: True
Is digit: True
Is alphabetic: False
Is space: True
=== Positional & Search ===
Starts with 'hello': True
Ends with 'world.': True
Position of 'world': 6
Index of 'o': 4
=== Transformations ===
Swap case: HELLO WORLD.
Join list with ',': a,b,c
Split lines: ['Hello', 'World']
String Slicing in Python (Python 字串分割)
- Definition: Extracting a portion of a string.
- Example String:
s = "HELLO WORLD"

Retrieving Characters by Index (透過索引值取得字元)
-
Syntax:
s[index]
-
Access the character at a specific position in string
s
. - Indexing starts from 0 in Python.
-
H | E | L | L | O |
---|---|---|---|---|
0 | 1 | 2 | 3 | 4 |
-11 | -10 | -9 | -8 | -7 |
, | W | O | R | L | D |
---|---|---|---|---|---|
5 | 6 | 7 | 8 | 9 | 10 |
-6 | -5 | -4 | -3 | -2 | -1 |
s[0] = s[-11]
s[4] = s[-7]
s[10] = s[-1]
s = "HELLO,WORLD"
print('s :', s)
print('s[0] ', s[0]) # 取 index = 0 第一個字 H
print('s[-11]', s[-11]) # 取 index = -11 第一個字 H
print('s[4] ', s[4]) # 取 index = 4 第五個字 O
print('s[-1] ', s[-1]) # 取 index = -1 最後一個字 D
s : HELLO,WORLD
s[0] H
s[-11] H
s[4] O
s[-1] D
Retrieving Characters by Index Example
👆
String Slice (如何分割字串)
- Syntax:
s[start_index:stop_index]
-
includes the
start_index
but -
excludes the
stop_index
.
-
includes the
H | E | L | L | O |
---|---|---|---|---|
0 | 1 | 2 | 3 | 4 |
-11 | -10 | -9 | -8 | -7 |
, | W | O | R | L | D |
---|---|---|---|---|---|
5 | 6 | 7 | 8 | 9 | 10 |
-6 | -5 | -4 | -3 | -2 | -1 |
s[0:3]
s[6:10]
s[-9:-4]
s = "HELLO,WORLD"
no = "01234567890"
no1 = "10987654321"
print('s :', ' '.join(s))
print('+no :', ' '.join(no))
print('-no :', ' '.join(no1), '\n')
print('s[2:5] ', s[2:5]) # 取 index 2-5 [2,3,4] 第2到第4
print('s[-10:-6] ', s[-10:-6]) # 從 -10 取到 -5
print('s[2:] ', s[2:]) # 從 index 2到底
print('s[:5] ', s[:5]) # 從 開頭取到第5
print('s[:-2] ', s[:-2]) # 從 開頭取到倒數第2
s : H E L L O , W O R L D
+no : 0 1 2 3 4 5 6 7 8 9 0
-no : 1 0 9 8 7 6 5 4 3 2 1
s[2:5] E L
s[-10:-6] W O
s[2:] E L L O , W O R L D
s[:5] H E L
s[:-2] H E L L O , W O R L
Example: How to Slice a String
👆
Variable (變數)
A variable is a symbolic name for a storage location where data can be held and referenced.
- Data Storage: Variables allow us to store and manage data for later use.
- Flexibility: We can change, update, or manipulate data through its variable.
- Readability: Variables give meaning to data, making code more understandable.
- Reusability: Variables let us use and reuse data without repeating it.
- Control: By using variables, we can dynamically control program behavior based on changing data values.
Understanding and Utilizing Variables
- variable as a name attached to a particular object
- variables also act as pointers, directing us to the exact location of our data in memory.
-
how to use
- assign it a value
- using it
Variables: Naming Objects
3
variable
Variables: Pointers to Data Locations
variable
3
Variable Assignment and Usage
- Assign a Value:
Use the " = " symbol to assign a value to a variable, e.g., `x = 10`.
- Utilize the Variable:
Once assigned, use the variable in calculations, functions, or any other operations, e.g., `print(x * 2)` 'x = x +2'.
Variable Assignment
x = 10
variable name
assign
value
10
x
10
x
Python Tutor Objects Render option
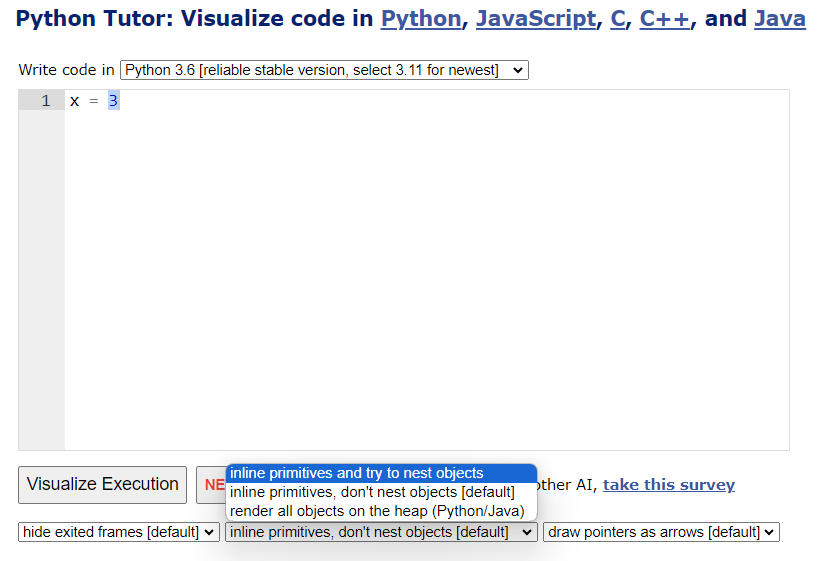
Naming Objects
Objects
Pointer
Python Tutor Naming Objects
Python Tutor Pointer to Data Locations
Example of Variable Assignment and Usage
# Variable Assignment in Python
# Assign a value to a variable using the "=" symbol
x = 10 # Here, 'x' is a variable that is assigned the value 10.
# Variables act as pointers to memory locations
# The variable 'x' points to a memory location holding the value 10.
# Utilize the variable in operations
double_x = x * 2 # Multiply the value of 'x' by 2 and assign to 'double_x'.
# Display the result using the variable
print(double_x) # This will print 20, which is 10 multiplied by 2.
20
Two variables have the same value
x = 3
y = 3
3
x
3
x
3
y
y
Two variables have the same value
Python Tutor -- inline primitive
Two variables have the same value
Python Tutor -- render all objects
Reassignment of Variables
- Key Points:
- Reassignment:
Variables can be reassigned to new values at any point - Dynamic Typing:
A variable can be assigned to one data type, and then be reassigned to another.
- Reassignment:
In Python, variables are not just tied to a specific value but also aren't bound to a specific data type.
Example of Reassignment of Variables
x = 10
print("x is an integer:", x)
x = 'Hello'
print("x is a string:", x)
x is an integer: 10
x is a string: Hello
10
x
'Hello'
x
Python Tutor
Chained Assignment
Allows you to assign a single value to multiple variables simultaneously
a = b = c = 300 # Chained assignment of value 300 to variables a, b, and c
print(a, b, c) # This will print 300 300 300
300, 300, 300
Python Tutor
Augmented Operations
performing an operation and assignment in one step
variable <operator>= expression
x += 5
Augmented Operations
x += 5 --> x = x + 5
x −= 3 --> x = x − 3
x ∗= 4 --> x = x * 4
x /= 2 --> x = x / 2
x %= 4 --> x = x % 4
x ∗∗= 3 --> x = x ** 3
x //= 3 --> x = x // 3
Augmented Operations Example
# Initialize variables
total_items = 0
price_per_item = 50 # in TWD
discount = 0.1 # 10% discount
# User buys 6 items
total_items += 6
total_cost = total_items * price_per_item
total_cost -= total_cost * discount # Apply discount
print(f"物品數量: {total_items}")
print(f"每件價格: {price_per_item} TWD")
print(f"總價格: {total_cost} TWD")
物品數量: 6
每件價格: 50 TWD
總價格: 270.0 TWD
Variable Naming Rules
-
Use only letters, numbers, and underscores.
-
Start with a letter or underscore, not a number.
-
Names are case-sensitive (variable ≠ Variable).
-
Avoid Python keywords (e.g., if, else, for).
-
No spaces; use underscores for separation (user_name).
-
Prefer descriptive names (word_list vs wl).
-
No special symbols like !, @, #, etc.
-
Chinese characters are permissible but not encouraged.
Variable Naming Examples
Variable Name | Result | Remarks |
---|---|---|
username | ✅ | |
_user_name | ✅❌ | python Internal use (by convention) |
user9name | ✅ | |
9username | ❌ | Starts with a number |
user name | ❌ | Contains a space |
user@name | ❌ | Contains a special symbol |
if | ❌ | Python keyword |
用户 | ✅❌ | Chinese characters (not recommended) |
user-name | ❌ | Contains a hyphen |
userName | ✅ | camelCase format |
Variable Naming Examples
# Traditional Chinese character in variable name (not recommended but valid)
學生 = "Alice" # Assigning Alice's name to the variable 學生
user_age = 25
user_email = "alice@example.com"
# Using numbers in variable names
order_1 = "Book"
order_2 = "Laptop"
# List of users (demonstrating snake_case)
user_list = [學生, "Bob", "Charlie"]
# Calculating average age of users (example of a descriptive variable name)
total_age = user_age + 30 + 28 # Ages of Alice, Bob, and Charlie
average_age = total_age / 3
# Translation: Alice's email is {user_email}, and she ordered a {order_1}.
print(f"{學生} 的電子郵件是 {user_email},她訂購了 {order_1}。")
# Translation: The average age of users is {average_age} years.
print(f"使用者的平均年齡是 {average_age:.2f} 歲。")
Variable Naming Examples
Alice 的電子郵件是 alice@example.com,她訂購了 Book。
使用者的平均年齡是 27.67 歲。
Immutability (不可變性)
- An inherent trait where an object, once established, remains unaltered.
- "不可變性" 指的是一個對象一旦創建,就不能被更改的特性
- int (整數)
- float (浮點數)
- bool (布林值)
- string (字符串)
- tuple (元組)
Memory Address (記憶體位址)
print(f"5,記憶體位址是 {hex(id(5))}")
print(f"5.0,記憶體位址是 {hex(id(5.0))}")
print(f"'hello',記憶體位址是 {hex(id('hello'))}")
print(f"(1, 2, 3),記憶體位址是 {hex(id((1, 2, 3)))}")
print(f"True,記憶體位址是 {hex(id(True))}")
5,記憶體位址是 0x7885b0880170
5.0,記憶體位址是 0x788596f9edd0
'hello',記憶體位址是 0x7885973310b0
(1, 2, 3),記憶體位址是 0x78857af16e40
True,記憶體位址是 0x57d94cfcf420
frozenset([1, 2, 3]),記憶體位址是 0x7885971cedc0
int Memory Address
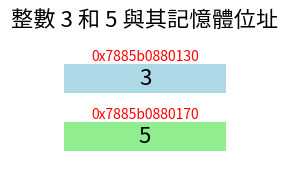
print(f"3,記憶體位址是 {hex(id(3))}")
print(f"5,記憶體位址是 {hex(id(5))}")
3,記憶體位址是 0x7885b0880130
5,記憶體位址是 0x7885b0880170
int Variable & Memory Address
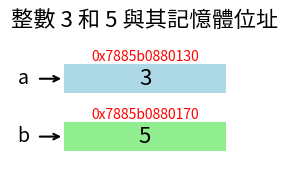
a = 3
print(f"a 的值是 {a},記憶體位址是 {hex(id(a))}")
b = 5
print(f"b 的值是 {b},記憶體位址是 {hex(id(b))}")
a 的值是 3,記憶體位址是 0x7885b0880130
b 的值是 5,記憶體位址是 0x7885b0880170
Immutability of int
a = 3
print(f"a 的值是 {a},記憶體位址是 {hex(id(a))}") # 這會顯示 'a' 的記憶體位址。
b = 5
print(f"b 的值是 {a},記憶體位址是 {hex(id(b))}") # 這會顯示 'b' 的記憶體位址。
a = 8
print(f"a 的值是 {a},記憶體位址是 {hex(id(a))}") # 新的記憶體位址,因為 a 現在參考到一個不同的整數。
b = 8
print(f"b 的值是 {a},記憶體位址是 {hex(id(b))}") # 新的記憶體位址,因為 b 現在參考到一個不同的整數。
a 的值是 3,記憶體位址是 0x7c165bd38130
b 的值是 3,記憶體位址是 0x7c165bd38170
a 的值是 8,記憶體位址是 0x7c165bd381d0
b 的值是 8,記憶體位址是 0x7c165bd381d0
Python Tutor 🔗
Python Strings: Immutable, Only Reassignable
(Python 字串: 不可變,僅可重新指定
String Immutable(字串不可變)
Example: String Immutable
word = 'Python'
word[0] = 'J'
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-74-ad89e228b316> in <cell line: 2>()
1 word = 'Python'
----> 2 word[0] = 'J'
TypeError: 'str' object does not support item assignment
String Reassign
word = 'Python'
new_word = 'J' + word[1:]
print(f"word {word}")
print(f"new word: {new_word}")
# Recreating new_word using join method
new_word_join = ''.join(['J', word[1:]])
print(f"new word: {new_word}")
word Python
new word: Jython
new word: Jython
Data Type Mismatches in Operations
(不同資料型態的運算不匹配)
Direct operations between different data types can result in errors.
不同的資料形態不能運算
123 + 'aaa'
'aaa' - 12
Example of Data Type Mismatches
a = 123 # a is int
b = '456' # b is str
print(a+b) # a+b ==> int + str has error
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-3-d3484c5e35e9> in <cell line: 3>()
1 a = 123 # a is int
2 b = '456' # b is str
----> 3 print(a+b) # a+b ==> int + str has error
TypeError: unsupported operand type(s) for +: 'int' and 'str'
Type Convert
OperationResult
int(x) | x converted to integer |
float(x) | x converted to floating point |
str(x) | x coverted to string |
a = 123 # a is int
b = '456' # b is str
print(a+int(b)) # int(b) ==> int + int
print(str(a)+b) # str(a) ==> str + str
579
123456
input Type str
x = input("What's x? ")
y = input("What's y? ")
z = x + y
print(z)
What's x? 3
What's y? 5
35
input Type Convert
x = int(input("What's x? "))
y = int(input("What's y? "))
z = x + y
print(z)
What's x? 3
What's y? 5
8
Calculate Grades Example
student_name = '李大中' # 學生名稱
ch_score = 98 # 國文成績
en_score = 95 # 英文成績
math_score = 90 # 數學成績
# 計算總分和平均
total = ch_score + math_score + en_score # 總分
average = total / 3 # 平均
# 顯示學生姓名和成績
print('學生姓名:', student_name)
print('國英數成績:', ch_score, en_score, math_score)
print('平均成績:', average)
學生姓名: 李大中
國英數成績: 98 95 90
平均成績: 94.33333333333333
round()
returns a floating point number that is a rounded version of the specified number
round(number) # 預設無小數
round(number , 小數後幾位)
print('round(59.4)', round(59.4))
print('round(59.5)', round(59.5))
sc = 5954/100
print('sc', sc, 'round(sc)', round(sc))
print('sc', sc, 'round(sc,1)', round(sc, 1))
print('sc', sc, 'round(sc,2)', round(sc, 2))
round(59.4) 59
round(59.5) 60
sc 59.54 round(sc) 60
sc 59.54 round(sc,1) 59.5
sc 59.54 round(sc,2) 59.54
Practice - Arithmetic Operations
請寫一支Python程式,能讀取兩個整數,並把他們的四則運算印出來。四捨五入取到小數點第二位
Practice - Calculate BMI
身體質量指數(Body Mass Index, BMI)
BMI = 體重(公斤)除以身高(公尺)的平方
---------------------------------------------------------------------------
請使用者輸入體重(公斤)和身高 (公分),協助計算使用者的BMI並輸出 (input(), int(x), print())
程式請宣告體重,身高,BMI三個變數
BMI請四捨五入取到小數點第二位 (fstring)
Data Structures
-
List
- A dynamic collection of items, ordered and mutable.
-
Tuple
- An ordered collection, similar to a list, but immutable.
-
Dictionary
- A collection of key-value pairs, allowing for efficient data retrieval.
-
Set
- An unordered collection of unique items.
Lists
A list is one of the fundamental data structures in Python. It is like a container that stores a collection of items in a specific order, and these items can be of any type.
Why Use Lists?
-
Store collections of related data.
-
Easily loop through multiple items.
-
Dynamic resizing based on content.
Using Lists
# Shopping List
shopping_list = ["milk", "eggs", "bread", "apples"]
# Bookshelf with Mixed Data Types (Strings and Dictionaries)
bookshelf = [
"Harry Potter",
{"title": "Harry Potter and the Philosopher's Stone", "author": "J. K. Rowling"},
"1984",
{"title": "Nineteen Eighty-Four", "author": "George Orwell"}
]
# Class Scores with Mixed Data Types (Strings and Floats)
class_scores = ["John", 85.5, "Emily", 92.0, "Michael", "Sarah", 89.0]
# Queue at a Bank with Mixed Data Types (Strings and Tuples)
bank_queue = ["Person1", ("Person2", "09:05 AM"), "Person3", ("Person4", "09:15 AM")]
Lists Key Features
-
Ordered:
- Items in a list have a defined order.
-
Mutable:
- You can add, remove, and modify items after the list is created.
-
Flexible:
- A list can contain items of different data types, including other lists!
Create a List
mixed = ['apple', 1, [2, 3], 3.1416]
print(f"mixed type: {type(mixed)}")
for i, item in enumerate(mixed):
print(f"Item {i} : {str(item):<10} - Type: {type(item)}")
mixed type: <class 'list'>
Item 0 : apple - Type: <class 'str'>
Item 1 : 1 - Type: <class 'int'>
Item 2 : [2, 3] - Type: <class 'list'>
Item 3 : 3.1416 - Type: <class 'float'>
How to Access Items
Python lists are ordered collections. Each item has an index starting from 0.
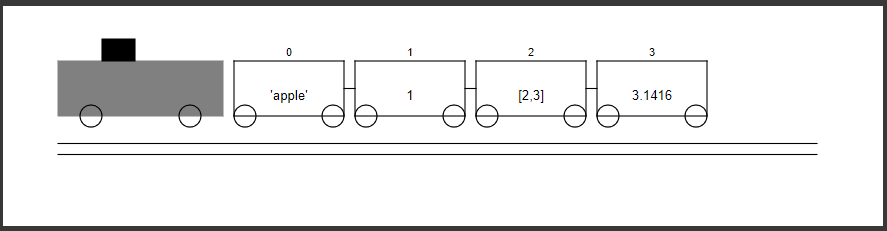
mixed
mixed[0]
mixed[1]
mixed[2]
mixed[3]
Accessing Items by Positive Index
mixed = ['apple', 1, [3, 4], 3.1416]
print(f"mixed : {mixed}\n")
for i in range(len(mixed)):
print(f"mixed[{i}] get {mixed[i]}")
mixed : ['apple', 1, [3, 4], 3.1416]
mixed[0] get apple
mixed[1] get 1
mixed[2] get [3, 4]
mixed[3] get 3.1416
Accessing Items by Negtive Index
mixed = ['apple', 1, [3, 4], 3.1416]
print(f"mixed : {mixed}\n")
for i in range(-1, -len(mixed)-1, -1):
print(f"mixed[{i}] get {mixed[i]}")
mixed : ['apple', 1, [3, 4], 3.1416]
mixed[-1] get 3.1416
mixed[-2] get [3, 4]
mixed[-3] get 1
mixed[-4] get apple
Index Out of Range
Trying to access an index that does not exist in the list will result in an IndexError
.
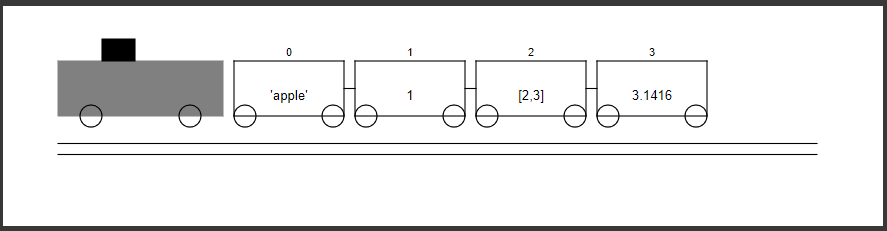
4
The maximum index of a list with N items is N-1
(because indexing starts from 0).
Index Out of Range
mixed = ['apple', 1, [3, 4], 3.1416]
print(mixed[4]) # ???????
---------------------------------------------------------------------------
IndexError Traceback (most recent call last)
<ipython-input-19-67ede96cbb23> in <cell line: 3>()
1 mixed = ['apple', 1, [3, 4], 3.1416]
2
----> 3 print(mixed[4]) # ???????
List Slicing
Slicing allows for extracting a subset of items from a list.
Syntax :
-
start:
- The first desired item (inclusive). Defaults to 0 if omitted.
-
stop:
- The first undesired item (exclusive). Goes to the end if omitted.
-
step:
- The interval between items. Defaults to 1 if omitted.
Index Out of Range
mixed = ['apple', 1, [3, 4], 3.1416]
print(f"mixed : {mixed}")
print("First three items mixed[0:3] :", mixed[0:3])
print("Items from second to third mixed[1:3] :", mixed[1:3])
print("All items except the first mixed[1:] :", mixed[1:])
print("All items except the last mixed[:-1] :", mixed[:-1])
print("Reversed every second item mixed[::-2] :", mixed[::-2])
print("Second to last, reversed mixed[-2::-1] :", mixed[-2::-1])
mixed : ['apple', 1, [3, 4], 3.1416]
First three items mixed[0:3] : ['apple', 1, [3, 4]]
Items from second to third mixed[1:3] : [1, [3, 4]]
All items except the first mixed[1:] : [1, [3, 4], 3.1416]
All items except the last mixed[:-1] : ['apple', 1, [3, 4]]
Reversed every second item mixed[::-2] : [3.1416, 1]
Second to last, reversed mixed[-2::-1] : [[3, 4], 1, 'apple']
List Assignment
- Lists are mutable in Python, which means their contents can be changed after creation.
- Individual items or entire sections of a list can be updated using assignment.
Assigning to an Index
mixed = ['apple', 1, [3, 4], 3.1416]
print(f"{'Before:':<26}", mixed)
mixed[0] = 'orange'
afterstr = "After mixed[0] = 'orange':"
print(f"{afterstr:<26}", mixed)
Before: ['apple', 1, [3, 4], 3.1416]
After mixed[0] = 'orange': ['orange', 1, [3, 4], 3.1416]
Assigning with Slicing
mixed = ['apple', 1, [3, 4], 3.1416]
print(f"{'Before:':<26}", mixed)
mixed[1:3] = [5, 6]
print(f"{'After mixed[1:3] = [5, 6]:':<26}", mixed)
Before: ['apple', 1, [3, 4], 3.1416]
After mixed[1:3] = [5, 6]: ['apple', 5, 6, 3.1416]
Inserting with Slicing
mixed = ['apple', 1, [3, 4], 3.1416]
print(f"{'Before:':<32}", mixed)
mixed[2:2] = ['berry', 7]
afterstr = "After mixed[2:2] = ['berry', 7]:"
print(f"{afterstr:<32}", mixed)
Before: ['apple', 1, [3, 4], 3.1416]
After mixed[2:2] = ['berry', 7]: ['apple', 1, 'berry', 7, [3, 4], 3.1416]
Removing with Slicing
afterstr = "After mixed[2:4] = []:"
width = len(afterstr)+1
mixed = ['apple', 1, [3, 4], 3.1416]
print(f"{'Before:':<{width}}", mixed)
mixed[2:4] = []
print(f"{afterstr:<{width}}", mixed)
Before: ['apple', 1, [3, 4], 3.1416]
After mixed[2:4] = []: ['apple', 1]
List Methods
# Append
# Description: Add an item to the end of the list.
fruits = ['apple', 'banana']
fruits.append('cherry')
print(fruits) # ['apple', 'banana', 'cherry']
# Insert
# Description: Insert an item at a specific position.
fruits.insert(1, 'avocado')
print(fruits) # ['apple', 'avocado', 'banana', 'cherry']
# Remove
# Description: Remove the first occurrence of a value.
fruits.remove('banana')
print(fruits) # ['apple', 'avocado', 'cherry']
More List Methods
# Extend
# Description: Extend the list by appending elements from another list.
veggies = ['carrot', 'broccoli']
fruits.extend(veggies)
print(fruits) # ['apple', 'avocado', 'cherry', 'carrot', 'broccoli']
# Pop
# Description: Remove the item at a specific position and return it.
# By default, it removes and returns the last item.
popped_item = fruits.pop(3)
print(popped_item) # 'carrot'
print(fruits) # ['apple', 'avocado', 'cherry', 'broccoli']
# Index
# Description: Return the index of the first occurrence of a value.
index_of_cherry = fruits.index('cherry')
print(index_of_cherry) # 2
More List Methods
# Count
# Description: Return the number of times a value appears in the list.
count_avocado = fruits.count('avocado')
print(count_avocado) # 1
# Sort
# Description: Sort the list in place.
fruits.sort()
print(fruits) # ['apple', 'avocado', 'broccoli', 'cherry']
# Reverse
# Description: Reverse the list in place.
fruits.reverse()
print(fruits) # ['cherry', 'broccoli', 'avocado', 'apple']
# Clear
# Description: Remove all items from the list.
fruits.clear()
print(fruits) # []
Tuples
-
A tuple is a collection of ordered elements.
-
Unlike lists, tuples are immutable.
This means that once a tuple is created, its elements cannot be modified. -
This immutability makes tuples a perfect choice for representing unchangeable data
Lists vs. Tuples
-
Mutability:
- Tuples are immutable, but Lists are mutable (can be changed).
-
Syntax:
- Tuples use parentheses
(),
while Lists are created using square brackets[]
,.
- Tuples use parentheses
Why Use Tuples?
-
Immutable
- Ensures data cannot be altered, protecting data integrity.
-
Safety
- Reduces bugs in code because you know the data won't change unexpectedly.
-
Use in Dictionaries:
- Tuples can be used as dictionary keys, while lists cannot.
Tuple: A Real-life Example
Consider a birthdate guessing game.
Once a birthdate is set, it remains unchanged.
By representing this data with a tuple, it guarantees constancy throughout its utilization.
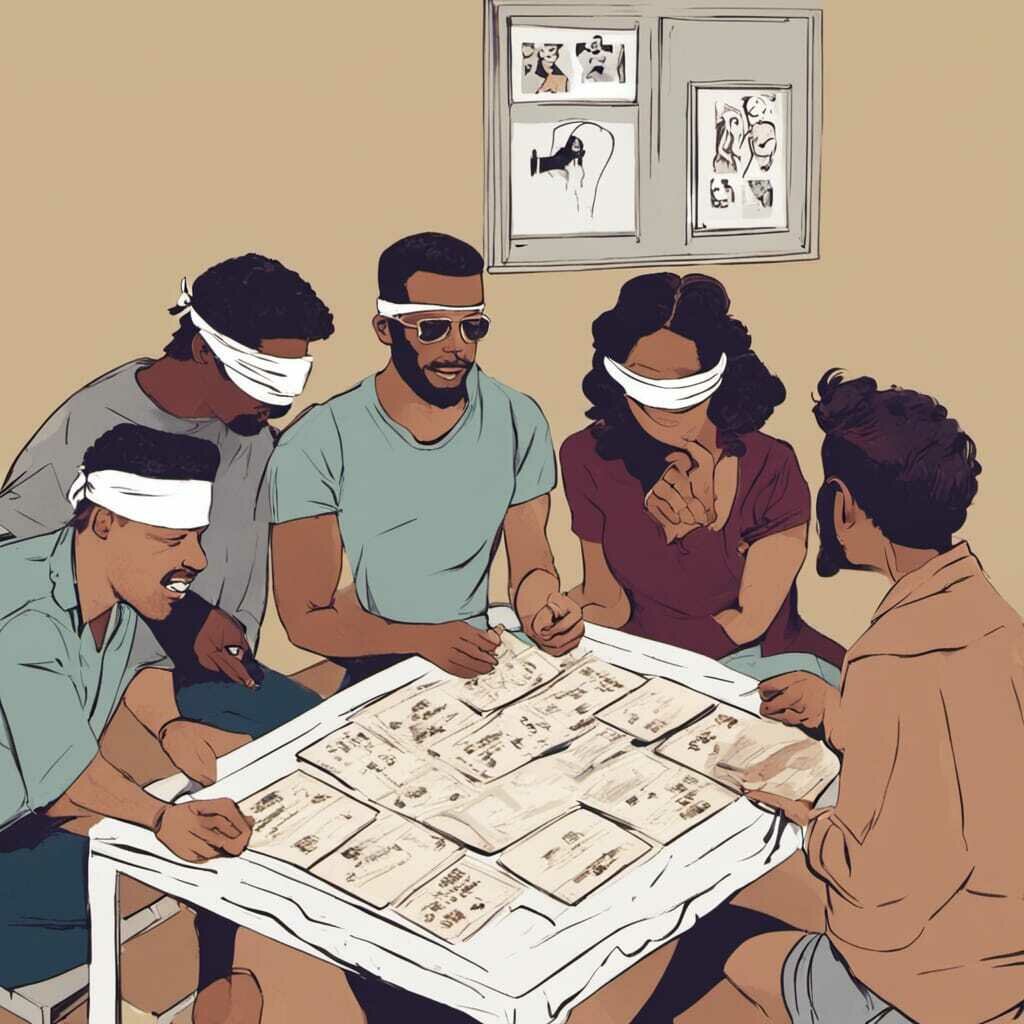
Create Tuples
# Tuples are made by comma-separating values inside parentheses.
birthdate = ("25th", "December", 2000)
print(birthdate, type(birthdate))
# Without Parentheses
birthdate = "25th", "December", 2000
print(birthdate, type(birthdate))
# Using the tuple() Constructor
date_list = ["25th", "December", 2000]
birthdate = tuple(date_list)
print(birthdate, type(birthdate))
A tuple representing a birthdate "25th", "December", 2000
('25th', 'December', 2000) <class 'tuple'>
('25th', 'December', 2000) <class 'tuple'>
('25th', 'December', 2000) <class 'tuple'>
Create Single Element Tuples
# Correct way to create a single-element tuple
correct_single_element_tuple = ('25th',)
print(f"(using '('25th',)') {correct_single_element_tuple} , \
type: {type(correct_single_element_tuple)}")
# Incorrect way to create a single-element tuple
incorrect_single_element_tuple = ("25th")
print(f"(using '('25th')') {incorrect_single_element_tuple} ,\
type: {type(incorrect_single_element_tuple)}")
(using '('25th',)') ('25th',) , type: <class 'tuple'>
(using '('25th')') 25th ,type: <class 'str'>
Create nested Tuples
birthdate_with_time = ('25th', 'December', 2000, ('12:00', 'PM'))
print("using nested tuples)")
print("birthdate_with_time = (25th', 'December', 2000, ('12:00', 'PM')")
print(f"birthdate_with_time {birthdate_with_time}")
print(f"birthdate_with_time type: {type(birthdate_with_time)}")
using nested tuples)
birthdate_with_time = (25th', 'December', 2000, ('12:00', 'PM')
birthdate_with_time ('25th', 'December', 2000, ('12:00', 'PM'))
birthdate_with_time type: <class 'tuple'>
Tuples Immutability
birthdate = ("25th", "December", 2000)
# we attempt to modify it
birthdate[1] = "January"
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-29-812b8ae24954> in <cell line: 4>()
2
3 # we attempt to modify it
----> 4 birthdate[1] = "January"
TypeError: 'tuple' object does not support item assignment
Tuple Concatenation
birthdate = ("25th", "December", 2000)
additional_info = ("Male", "A+")
combined_data = birthdate + additional_info
print(f"Concatenated tuple: {combined_data}")
Concatenated tuple: ('25th', 'December', 2000, 'Male', 'A+')
Tuple checking the existence
birthdate = ("25th", "December", 2000)
# Check if "December" exists in the tuple
exists_december = "December" in birthdate
print(f"Does 'December' exist in the tuple? {exists_december}")
# Check if "January" exists in the tuple
exists_january = "January" in birthdate
print(f"Does 'January' exist in the tuple? {exists_january}")
# Check if a number exists in the tuple
exists_2000 = 2000 in birthdate
print(f"Does the number 2000 exist in the tuple? {exists_2000}")
# Check if a number doesn't exist in the tuple
not_exists_1999 = 1999 not in birthdate
print(f"Does the number 1999 not exist in the tuple? {not_exists_1999}")
Does 'December' exist in the tuple? True
Does 'January' exist in the tuple? False
Does the number 2000 exist in the tuple? True
Does the number 1999 not exist in the tuple? True
Dictionaries
- A collection of data values that is unordered.
- It is used to store data values as "key:value" pairs.
- Unlike lists, which are indexed by a range of numbers, dictionaries are indexed by keys. These keys can be of any immutable type.
- Can‘t contain duplicate keys
Dictionaries
The world of key-value pairs in Python!
Key | Value |
---|---|
Apple | 蘋果 |
Orange | 橘子 |
Papaya | 木瓜 |
Creating a Dictionary
-
Create a dictionary with key:value pairs inside curly braces { }.
-
Dictionary keys are unique.
person = {
"name": "John",
"age": 25,
"is_student": False
}
fruits = {
"Apple" : "蘋果",
"Orange" : "橘子",
"Papaya" : "木瓜"
}
Retrieve values using their respective key
name = person["name"]
Access a value by key with get()
name = person.get("name")
Accessing Items in the Dictionary
# Accessing items from the 'fruits' dictionary
apple_in_chinese = fruits["Apple"]
orange_in_chinese = fruits.get("Orange")
print(f"Translation for Apple: {apple_in_chinese}")
print(f"Translation for Orange: {orange_in_chinese}")
# Accessing items from the 'person' dictionary
person_name = person["name"]
is_student = person.get("is_student")
print(f"\nPerson's Name: {person_name}")
print(f"Is the person a student? {'Yes' if is_student else 'No'}")
Translation for Apple: 蘋果
Translation for Orange: 橙子
Person's Name: John
Is the person a student? No
Accessing Items in the Dictionary
Inserting Items in the Dictionary
Add a new key:value pair
fruits["Banana"] = "香蕉"
fruits["Grape"] = "葡萄"
print(fruits)
# {'Apple': '蘋果', 'Orange': '橘子', 'Papaya': '木瓜', 'Banana': '香蕉', 'Grape': '葡萄'}
person["gender"] = "male"
person["country"] = "USA"
print(person)
# {'name': 'John', 'age': 25, 'is_student': False, 'gender': 'male', 'country': 'USA'}
Modifying Items in the Dictionary
Using a key:value pair with an existing key
fruits["Papaya"] = "大木瓜"
print(fruits)
# {'Apple': '蘋果', 'Orange': '橘子', 'Papaya': '大木瓜', 'Banana': '香蕉', 'Grape': '葡萄'}
person["name"] = "Jane"
person["age"] = 30
print(person)
# {'name': 'Jane', 'age': 30, 'is_student': False, 'gender': 'male', 'country': 'USA'}
Deleting Items in the Dictionary
-
Using
del
keyword-
Removes the item with the specified key name
del fruits["Apple"]
-
-
Using
pop()
method-
Removes the item with the specified key name and returns its value
-
removed_fruit = fruits.pop("Orange")
-
-
Using
popitem()
method-
Removes the last inserted key-value pair
-
last_item = fruits.popitem()
-
Deleting Items in the Dictionary
fruits = {
"Apple" : "蘋果",
"Orange" : "橘子",
"Papaya" : "木瓜",
'Banana': '香蕉'
}
del fruits["Apple"]
print(fruits) # {'Orange': '橘子', 'Papaya': '木瓜', 'Banana': '香蕉'}
removed_fruit = fruits.pop("Orange")
print(removed_fruit) # 橘子
print(fruits) # {'Papaya': '木瓜', 'Banana': '香蕉'}
last_item = fruits.popitem()
print(last_item) # ('Banana', '香蕉')
print(fruits) # {'Papaya': '木瓜'}
Looping Through a Dictionary
person = { "name": "John","age": 25,"is_student": False}
# Looping through keys:
for key in person:
print(f" key: {key:<5}", end=' ')
print()
# Looping through values:
for value in person.values():
print(f"value: {value:<5}", end=" ")
print()
# Looping through both:
for key, value in person.items():
print(f"{key}: {value}")
key: name key: age key: is_student
value: John value: 25 value: 0
name: John
age: 25
is_student: False
Sets
- Definition: In Python, sets are a collection of unique items.
- Characteristics:
- Unordered: The items in a set do not have a defined order.
- Unchangeable: Cannot change the items after the set is created.
- Do not allow duplicate values.
Creating an Empty Set
Using just {}
creates a dictionary, not a set. To create an empty set, you use the set()
constructor.
empty_set = set()
Creating a Set
To create a set, place a comma-separated list of items between curly braces {}
.
fruits = {"apple", "banana", "cherry"}
Creating a Set from a List
You can use the set()
constructor to create a set from a list or any other iterable.
numbers_list = [1, 2, 3, 3, 4]
unique_numbers = set(numbers_list) # This will remove duplicates
Note on Duplicates
Sets automatically remove duplicate values. So, if you create a set with duplicate items, they will be stored only once.
duplicates = {"apple", "banana", "apple"}
print(duplicates) # Outputs: {"apple", "banana"}
Iterating Through a Set
Since sets are unordered, you cannot access items using an index. Instead, you can loop through the set items using a for
loop.
fruits = {"apple", "banana", "cherry"}
for fruit in fruits:
print(fruit)
Checking if an Item Exists
To check if an item is present in a set, use the in
keyword.
if "apple" in fruits:
print("Apple is in the set")
Length of a Set
You can use the len()
function to determine how many items a set has.
num_of_fruits = len(fruits)
print(num_of_fruits) # Outputs: 3
Adding Items Using `add()`
To add a single item to a set, use the `add()` method.
fruits = {"apple", "banana", "cherry"}
fruits.add("orange")
# Output: {'apple', 'banana', 'cherry', 'orange'}
Adding Items Using update()
To add multiple items (from lists, sets, or other iterables), use the update()
method.
fruits = {"apple", "banana", "cherry"}
fruits.update(["mango", "grapes"])
# Output: {'apple', 'banana', 'cherry', 'mango', 'grapes'}
Removing Items Using remove()
This method removes the specified item. If the item is not found, it raises an error.
fruits = {'apple', 'banana', 'cherry', 'mango', 'grapes'}
fruits.remove("banana")
# Output: {'apple', 'cherry', 'mango', 'grapes'}
Removing Items Using discard()
This method removes the specified item. However, if the item is not found, it does NOT raise an error.
fruits = {'apple', 'cherry', 'grapes', 'mango'}
fruits.discard("mango")
# Output: {'apple', 'cherry', 'grapes'}
Removing Items Using pop()
Since sets are unordered, the pop()
method removes a random item. Be cautious when using this.
fruits = {'apple', 'cherry', 'grapes'}
removed_fruit = fruits.pop()
print(f"Removed: {removed_fruit}, Remaining: {fruits}")
# Potential Output: Removed: apple, Remaining: {'cherry', 'grapes'}
Clearing a Set
To remove all items from a set, use the clear()
method.
Example:
fruits.clear()
Removing Duplicates
Sets store unique values, making them ideal for removing duplicates from a list.
items = [1, 2, 2, 3, 4, 4, 5]
unique_items = set(items)
print(unique_items) # Output: {1, 2, 3, 4, 5}
Removing Duplicates
Sets can be used to quickly check for common words, find unique words, or exclude specific words from texts.
text = "apple banana apple orange"
words = set(text.split())
print(words) # Output: {"apple", "banana", "orange"}
What is a Function?
- A block of organized, reusable code that performs a single, related action.
- Makes code more modular and easier to manage.
- Can return values and accept parameters (arguments).
Functions are fundamental in programming. They prevent code repetition and improve code clarity.
Why Use Functions?
- Avoid repetition of code.
- Increase code reusability.
- Improve code readability.
- Simplify complex tasks.
Defining a Function
- Use the
def
keyword. - Followed by the function name.
- Parameters inside parentheses.
- A colon to start the function body.
# Define a function called 'greet' that takes a single parameter 'name'
def greet(name):
# Print a greeting message using the provided name
print(f"Hello, {name}!")
Calling a Function
- Use the function name followed by parentheses.
- If the function accepts arguments, provide them inside the parentheses. Example:
# Define a function called 'greet' that takes a single parameter 'name'
def greet(name):
# Print a greeting message using the provided name
print(f"Hello, {name}!")
# Call the 'greet' function and pass the string "Alice" as an argument
greet("Alice")
No-Parameter Function
define a function that doesn't take any parameters.
# Define a function called 'display_message'
def display_message():
# Print a static message
print("Welcome to Python Programming!")
# Call the 'display_message' function
display_message() # Expected output: Welcome to Python Programming!
Return Values
- Use the
return
keyword to send a result back from the function. - A function without a return statement returns
None
. Example:
# Define a function called 'add' that takes two parameters: 'a' and 'b'
def add(a, b):
# Return the sum of 'a' and 'b'
return a + b
# Call the 'add' function with arguments 5 and 3, and print the result
print(add(5, 3)) # Expected output: 8
Multiple Parameters
- A function can have any number of parameters.
- Parameters are separated by commas. Example:
# Define a function called 'full_name' that takes two parameters: 'first_name' and 'last_name'
def full_name(first_name, last_name):
# Return the concatenated full name using the provided first and last names
return f"{first_name} {last_name}"
# Call the 'full_name' function with "John" as the first name and "Doe" as the last name, and print the result
print(full_name("John", "Doe")) # Expected output: John Doe
Default Parameters
- Assign a default value to a parameter.
- Used when an argument is not provided for that parameter. Example:
# Define a function called 'greet' with a default parameter 'name' set to "Guest"
def greet(name="Guest"):
# Print a greeting message using the provided name or the default value if no name is provided
print(f"Hello, {name}!")
# Call the 'greet' function without providing an argument, which will use the default value "Guest"
greet() # Expected output: Hello, Guest!
# Call the 'greet' function with "Alice" as an argument
greet("Alice") # Expected output: Hello, Alice!
Variable-length Arguments
Use *args
for variable number of positional arguments.
# Define a function called 'print_args' that accepts a variable number of arguments using *args
def print_args(*args):
# Join all arguments with a space and print them in one line
print(' '.join(map(str, args)))
# Call the 'print_args' function with multiple arguments
print_args(1, 2, 3) # Expected output: 1 2 3
print_args("apple", "banana", "cherry") # Expected output: apple banana cherry
Variable-length Arguments
Use **kwargs
for variable number of keyword arguments.
# Define a function that accepts any number of keyword arguments using **kwargs
def print_key_value_pairs(**kwargs):
# Loop through each keyword argument and print the key-value pair
for key, value in kwargs.items():
print(f"{key}: {value}")
# Call the function with two keyword arguments
print_key_value_pairs(brand="Apple", product="iPhone")
# Expected output:
# brand: Apple
# product: iPhone
print_key_value_pairs(subject="Math", grade="A", semester="Spring")
# Expected output:
# subject: Math
# grade: A
# semester: Spring
Detailed Function Description with Docstrings
Python docstrings associate descriptions or documentation with functions, modules, or classes using triple quotes ('''
or """
).
def calculate_area(length, width):
'''
Calculate the area of a rectangle.
Parameters:
- length (float): The length of the rectangle.
- width (float): The width of the rectangle.
Returns:
- float: The area of the rectangle.
'''
return length * width
# Example usage
area = calculate_area(5, 3)
print(area) # Expected output: 15
Recap and Practice
- Functions are a fundamental concept in programming.
- They help organize, modularize, and reuse code.
-
Practice Task:
write a function that calculates the area of a circle.
CS50P 0_Functions, Variables
By wschen
CS50P 0_Functions, Variables
This presentation covers Python basics including functions, variables, and datatypes. It also explores string manipulation and the use of escape characters. Discover how to properly declare strings and handle special characters.
- 746