Week 6
Agenda
- LD_PRELOAD
- Symbolic Execution
- DLL Injection
LD_PRELOAD
Remember API calls?
The GOT and PLT are tables for which a program gets linked.
In Linux there is a way to get in between this linking called LD_PRELOAD

We want our library here!
LD_PRELOAD example
#define _GNU_SOURCE
/* Most imports for things you might need, Do Not Delete any */
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <errno.h>
#include <sys/fcntl.h>
#include <dlfcn.h>
#include <dirent.h>
/* Compile: gcc -Wall -fPIC -shared -o evil.so framework.c -ldl */
/* -ldl must be last!!! */
static int (*o_open)(const char *, int);
static void init (void) __attribute__ ((constructor));
/* loaded and executed when ld runs */
void init(void){
/* Get handle on original open */
o_open=dlsym(RTLD_NEXT, "open");
}
/* Try: LD_PRELOAD=./evil.so cat framework.c */
int open(const char *pathname, int flags, ...){
/* example */
printf("Hello world\n");
/* Your code here */
/* Preserve functionality */
return o_open(pathname, flags);
}
Imports for libraries we want to use or hook
SO compile flags
Dynamic set up to best mask our presence
The Hook:
- Do what we want
- Call original
How can we find cool things to hook in our favorite programs?
Trace them! Last week we talked about Ltrace and Strace!
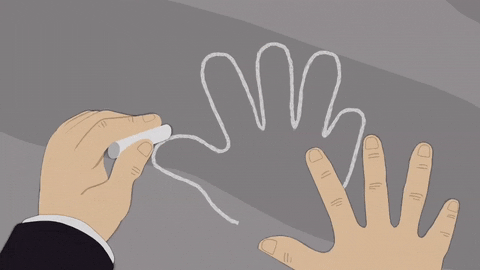
Ltrace is going be great, its output goes to STDERR or we can use the `-o FILE` option. Programs like Vim or Emacs will have long traces, and so we might want to grep for certain functions we hope to find. This is why I mention `-o FILE` so we can save output while running the program separately
Tracing slows the process a LOT, give it time to execute!
Symbolic Execution
What is Symbolic Execution?
a means of analyzing a program to determine what inputs cause each part of a program to execute. An interpreter follows the program, assuming symbolic values for inputs rather than obtaining actual inputs as normal execution of the program would, a case of abstract interpretation. It thus arrives at expressions in terms of those symbols for expressions and variables in the program, and constraints in terms of those symbols for the possible outcomes of each conditional branch. -wiki
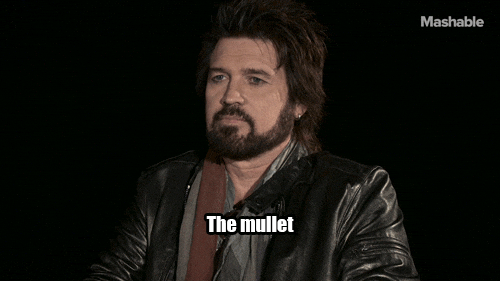
After Break, we will work with Angr
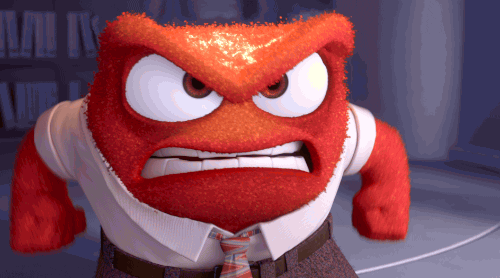
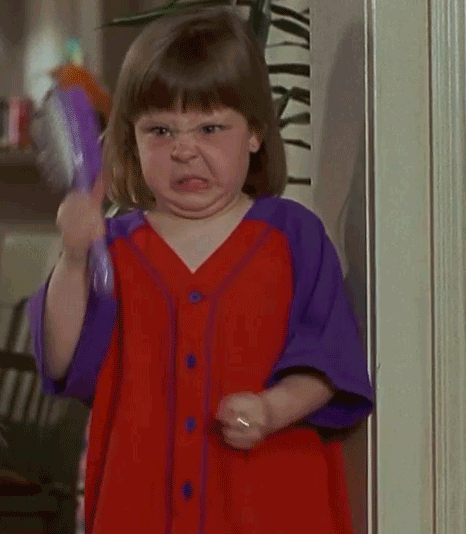

DLL Injection
But first we need to talk about Windows internals
Windows Objects
- File
- Thread
- Process
- Etc.
A Windows object is a data structure that represents a system resource
Windows objects are created and accessed using the Win32 API
- Kernel32.dll
- User32.dll
- GDI32.dll
Windows Handles
- Application accesses Windows Objects through a handle
- Each handle refers to an entry in an internal object table that contains the address of a resource and means to identify the resource type
Handles
Injection time
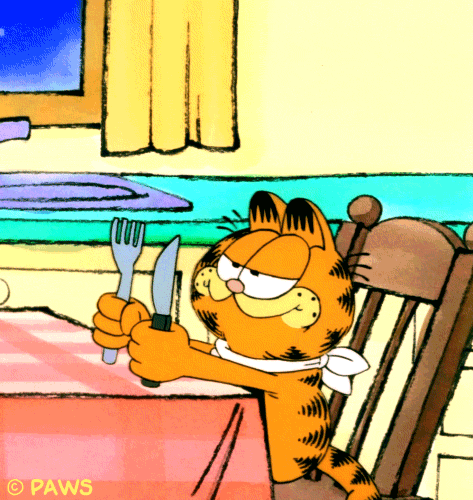
- Attach to target process
- Allocate Memory
- Write DLL to memory
- Execute the DLL
In general this can be done in four steps
Attaching
In order to "attach" to a process we need to get a handle to the target process

OpenProcess to get a handle!
Alocating Memory
First we need to get the length of the path to our DLL
We can then directly request memory from the process we opened (remember the permissions we requested)

Writing DLL to memory
First we need to get LoadLibraryA from kernell32.dll

We're not actually going to load the DLL into memory directly. We're going to tell the process to do it for us
Executing the DLL
First we need to get LoadLibraryA from kernell32.dll
With this method we tell the process handle to create a remote thread and start the thread with LoadLibraryA, with the DLL name as the argument

Week 6
By Drake P
Week 6
LD_PRELOAD, Symbolic execution
- 179