Javascript
Week 12
What is the Dom?
- It's an API for HTML pages
- Serves as a "map" to all the elements on the page
- Can use the "map" to locate and manipulate elements and content
- Without the DOM, JavaScript wouldn't be able to locate and manipulate items on an HTML page
Nodes
Each HTML element on a page is considered a node
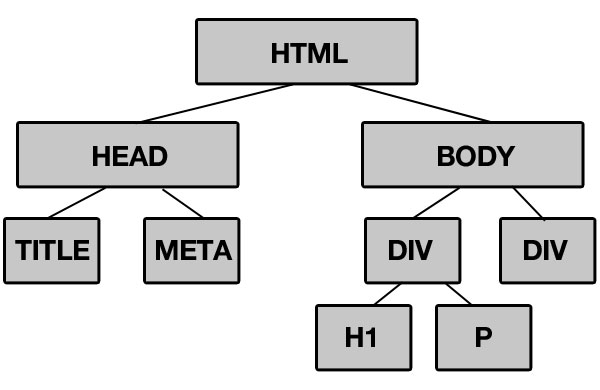
Nodes
- You can access all the nodes on an HTML page
- document object identifies the page and is the starting point for DOM crawling
- There are a number of standard built-in properties that you can use to access collections of elements
Nodes
var time=document.getElementbyId ("clock")innerHTML
Accessing DOM NODES
- document.getElementByTagName()
- The above retrieves element(s) you specify in the argument
- document.getElementByTagName("h1") returns every H1 tag on the page using a collection (or nodeList) starting with the number 0
- 0 is the first H1 on the page with the highest number being the last H1 that appears on the page
- Example h1 [0], h1 [1], h1[2]
Accessing Dom Nodes
- document.getElementById()
- The above returns a single element based on the ID called in theattribute
Accessing Dom Nodes
<img src="images/kitten.jpg" id="kittenPhoto"/>
var photo=getElementById("kittenPhoto.jpg")
Accessing Dom nodes
- document.getElementByClassName()
- Allows you to access a node based on it's class value
var firstColumn=document.getElementByClassName("colA")
If there is more than one class on the page with the name, it will put them in a Node List like getElementByTagName
Accessing Dom Nodes
- document.querySelectorAll()
- The above access a node based on the CSS style selector
- var paragraph="document.querySelectorAll(".col H1")
- the above also returns a Node List
Accessing dom nodes
- document.getAttribute()
- The above accesses an attribute in an HTML tag
<img src="kittens.jpg" id="catPhoto"/>
var catImage= document.getAttribute ("catPhoto");
alert (catPhoto.getAttribute("src"));
Manipulating nodes
- setAttribute() can set a previous attribute to a new attribute
var catImage="document.getElementByID("kittens.jpg");
catImage.setAttribute ("src", "kittens2.jpg");
Manipulating nodes
- innerHTML(); accesses and changes text and markup inside an element
varColLeft=document.getElementByClassName("intro");
ColLeft.inner.HTML="<p>this is the intro</p>"
Manipulating nodes
- style() - adds, modifies or removes styles
document.getElementByID("intro").style.color="#000;"
Adding and removing elements
- createElement(); creates a new element
var newCol=document.createElement("div");
- createTextNode(); enters text in an element
var newText=document.createTextNode("Wow!");
- appendChild(); takes the above and adds them to the HTML
var newDiv=document.getElementbyID("col1")
var newText=document.createTextNode("Wow!");
newDiv.appendChild(newText)
adding and removing elements
- insertBefore(); inserts an element before another element
var ourDiv=document.getElementByID("ourDiv");
var para = document.getElementByID("newPara");
var newHeading=document.getElementById("h1");
var hText=document.createTextNode("New Heading");
newHeading.appendChild(hText);
ourDiv.insertBefore(newHeading, para)
Adding and removing Elements
- replaceChild(); replaces one node with another
- Takes 2 arguments (new child, node that gets replaced by the first)
var ourDiv=document.getElementById("col1");
var swap=document.getElementById("swapMe");
var newImg=document.createElement("img");
newImg.setAttribute("src", "images/kitten2.jpg");
ourDiv.replaceChild(newImg, swap);
adding and remove elements
- removeChild(); removes a node
var parentDiv=document.getElementById("parent");
var remove=document.getElementById("removeMe");
parentDiv.removeChild(remove);
PolyfilLs
Normalizes behavior across all browsers using JavaScript
Used to help create HTML5 functionality that a browser may not support
- HTML 5 shiv (shim)
- Modernizr
- Selectivzr
- Repond.js
Polyfills:HTML5 Shiv
- enable IE8 and earlier to recognize and style newer HTML5 tags such as header, footer, article, section and nav
-
http://code.google.com/p/html5shim/
- Download JS file, place in your website folder, then reference JS file in the head tag using an IE conditional
<!--[if lt IE9]>
<script src="html5shim.js"></script>
<![endif]-->
POLYFILLS:Modernizr
- tests to see if a browser doesn't support certain features and loads polyfills to correct them
-
http://modernizr.com/
POLYFILLS:MODERNIZR
- Allows older versions of IE to understand CSS3 selectors that it currently doesn't support
-
http://selectivizr.com/
POLYFILLS: REPSPOND.JS
- Allows older browsers to support max and min width CSS properties and media queries
- you can download respond.js and reference it in the head tag
-
https://github.com/scottjehl/Respond
Javascript libraries
Javascript Week 12
LWD: week 12
By shadow4611
LWD: week 12
- 411